In C++, a CPP equation typically refers to mathematical expressions or calculations implemented using C++ syntax to achieve specific outcomes. Here's a simple example:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 10;
int sum = a + b; // Simple equation: sum of a and b
cout << "The sum is: " << sum << endl;
return 0;
}
Understanding Basic Mathematical Concepts in C++
Equations are fundamental components of programming, allowing developers to perform calculations and manipulate data effectively. In C++, equations can be represented using various operators and constructs that enable complex decision-making and problem-solving.
Importance of Operators in Equations
In C++, operators play a significant role in how equations are executed. They are symbols that perform specific operations on variables and constants, forming the backbone of mathematical manipulations.
Arithmetic Operators: These are the most basic operators, used to perform standard mathematical operations:
- Addition (+): Combines two values.
- Subtraction (-): Finds the difference between two values.
- Multiplication (*): Calculates the product of two values.
- Division (/): Divides one value by another.
- Modulus (%): Returns the remainder of a division operation.
Example: Basic Arithmetic Operations in C++
int a = 10, b = 20;
int sum = a + b; // 30
int difference = a - b; // -10
int product = a * b; // 200
int quotient = b / a; // 2
int remainder = b % a; // 0
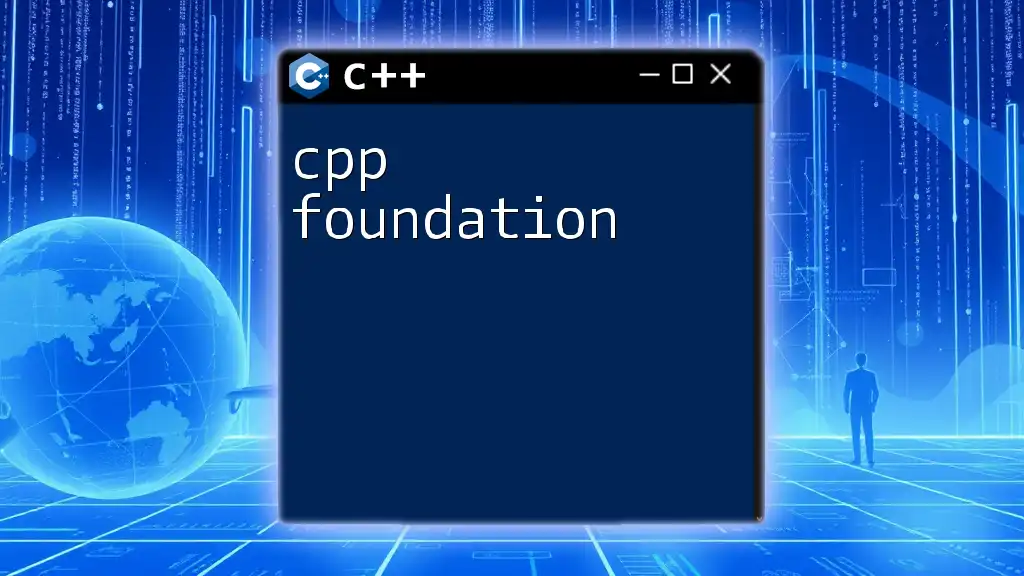
Working with Variables and Constants
In C++, variables and constants allow you to store and manipulate data for your equations.
Defining Variables and Constants
Variables are data holders that can change during program execution, while constants hold fixed values. Understanding data types—such as `int`, `float`, and `double`—is crucial as they influence how equations compute.
Example: Using Variables and Constants in Equations
const float PI = 3.14; // Fixed value
int radius = 5;
float area = PI * radius * radius; // Area of a circle
In this example, the value of `PI` is constant, ensuring accuracy in calculations involving circular shapes.
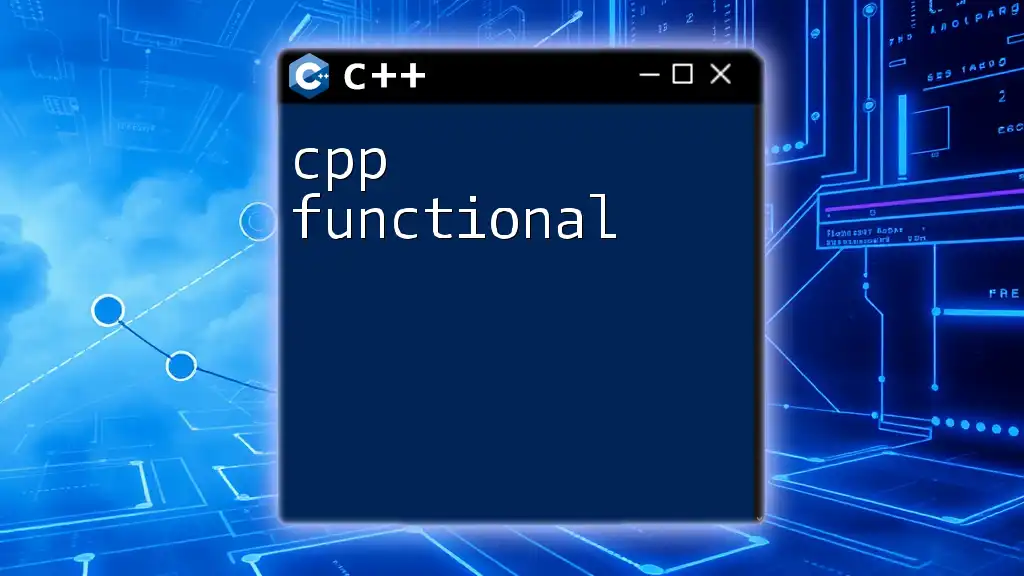
Control Structures for Equations
Control structures like `if`, `else`, and loops help manage the flow of your program, especially when calculations require conditions to be met.
Utilizing Conditionals and Loops
When creating equations that depend on specific criteria, control structures allow you to execute different code paths. For instance, you might want a function to compute the factorial of a number based on user input.
Example: Calculating the Factorial of a Number
int n = 5; // Example input
int factorial = 1;
for (int i = 1; i <= n; ++i) {
factorial *= i; // 120
}
This loop multiplies numbers from 1 to `n`, yielding the factorial result through repeated multiplications.
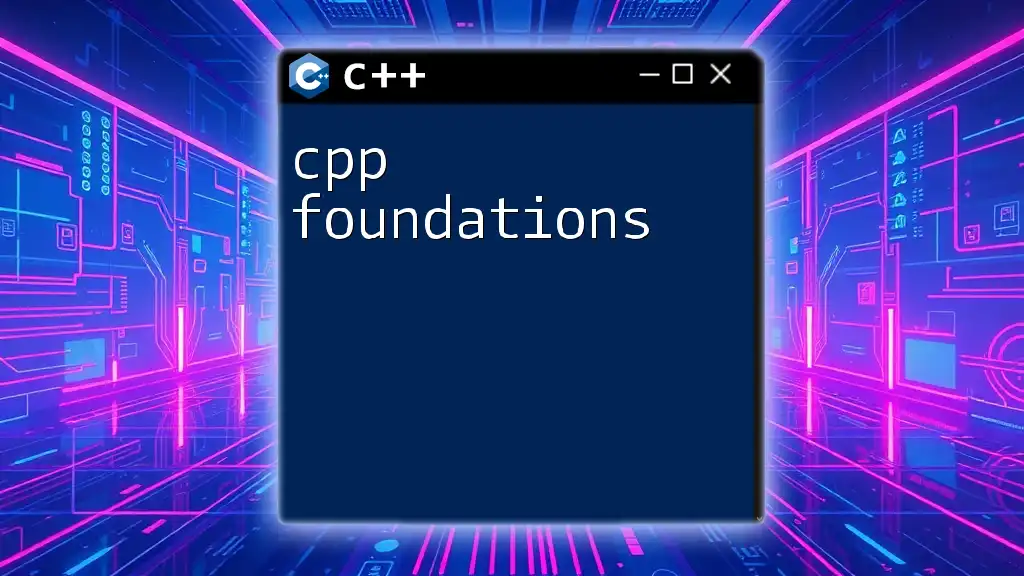
Functions and Equations
Functions encapsulate equations, allowing for reusable and organized code. They simplify complex calculations and enhance readability.
Creating a Function for Solving a Quadratic Equation
Quadratic equations represent various real-world phenomena. Implementing the quadratic formula in C++ showcases the power of functions.
Example: Solving a Quadratic Equation
The quadratic formula is given by:
\[x = \frac{-b \pm \sqrt{b^2 - 4ac}}{2a}\]
Here's a C++ function that uses this formula:
void solveQuadratic(float a, float b, float c) {
float discriminant = b * b - 4 * a * c; // Checking for real roots
if (discriminant >= 0) {
float root1 = (-b + sqrt(discriminant)) / (2 * a);
float root2 = (-b - sqrt(discriminant)) / (2 * a);
cout << "Roots are: " << root1 << " and " << root2;
} else {
cout << "No real roots.";
}
}
This function calculates the discriminant to determine the nature of the roots and computes them if they are real.
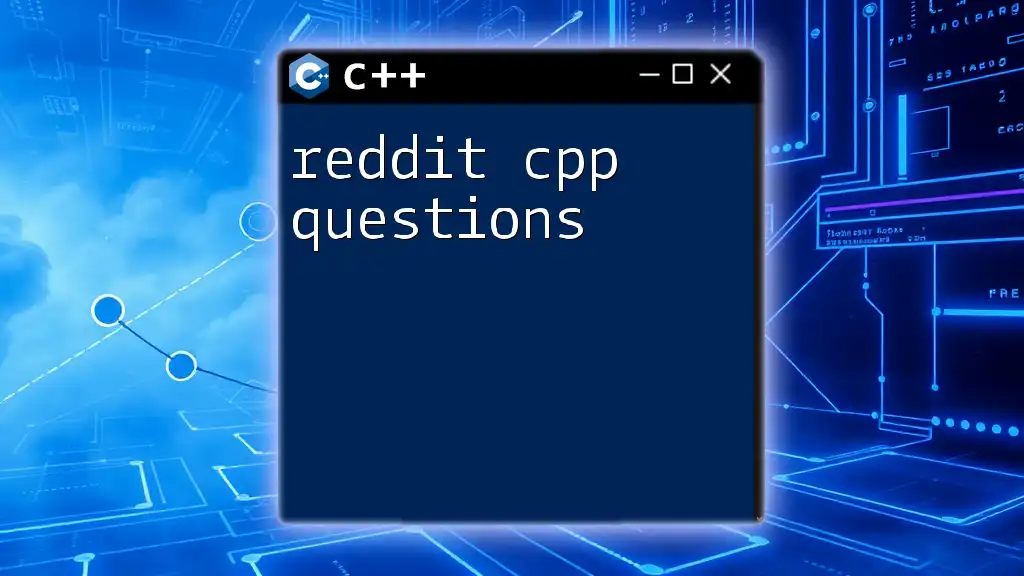
Advanced Equation Techniques
For more complex calculations, C++ provides libraries that can handle intricate mathematical functions, such as `cmath`.
Example: Calculating the Sine of an Angle
Using library functions can streamline operations that would otherwise require significant effort in coding.
Example: Calculating Sine
#include <cmath> // Include the cmath library
double angle = 45.0; // Angle in degrees
double radians = angle * (M_PI / 180); // Conversion to radians
double sineValue = sin(radians); // Calculate sine
In this example, the sine function from the `cmath` library efficiently computes the sine of an angle after converting it from degrees to radians.
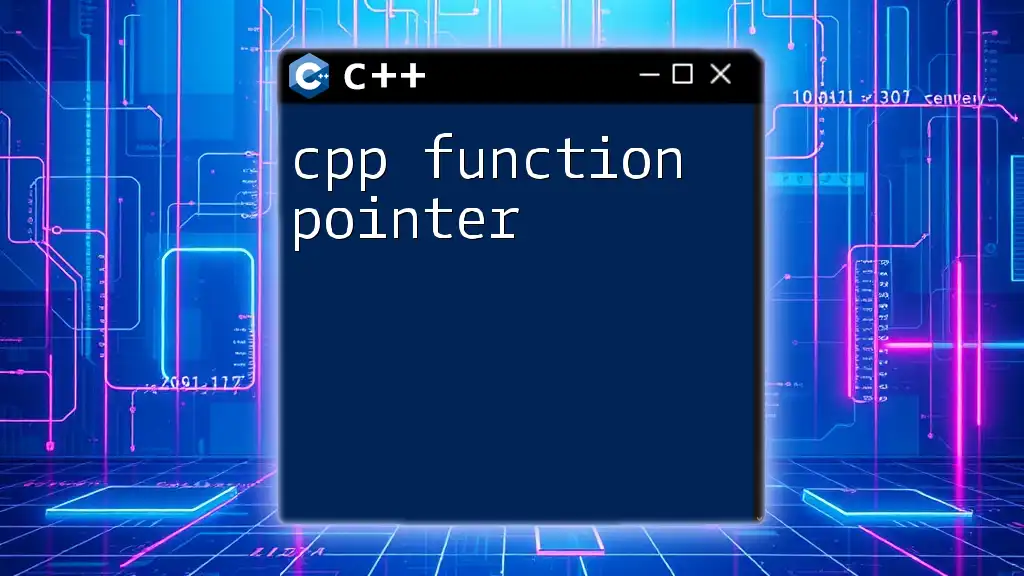
Practical Applications of Equations in C++
Equations in C++ have real-world applications across various fields, such as engineering, finance, and data analysis. Understanding how to implement these concepts can significantly enhance your programming skills.
Case Study: Building a Simple Calculator
A calculator is an excellent example of how to integrate equations effectively. Here's a basic function that carries out arithmetic operations based on user input.
Example: Simple Calculator Function
float calculate(float a, float b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/':
if (b != 0) return a / b; // Prevent division by zero
else throw runtime_error("Division by zero error!");
default: return 0; // Default case for unrecognized operator
}
}
This function leverages a `switch` statement to determine the operation, effectively using equations to produce results based on user input.
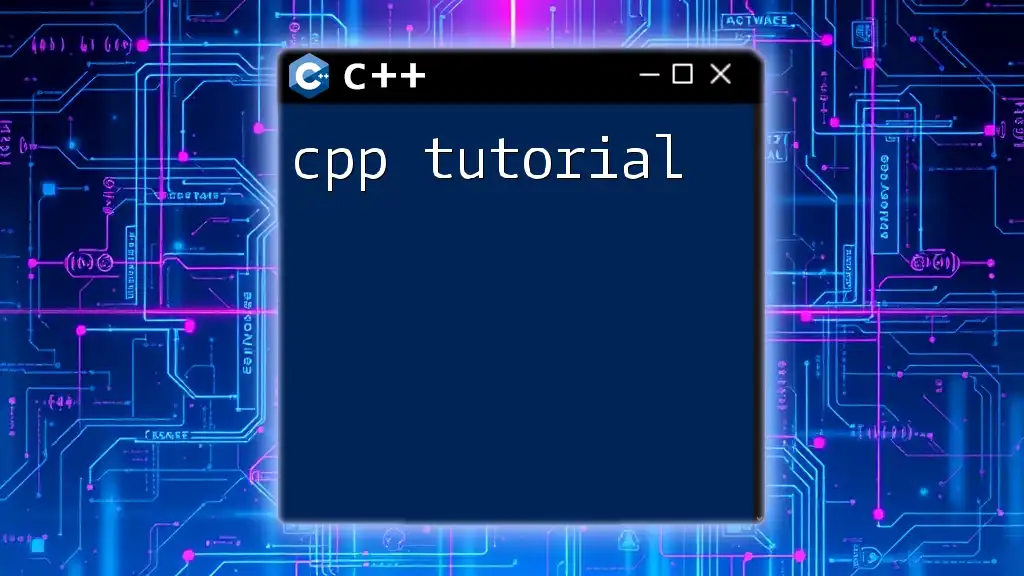
Conclusion
In this guide, we've explored various aspects of the CPP equation through arithmetic operations, control structures, functions, and libraries. As you continue your journey in C++, practicing these concepts will empower you to write robust programs that perform essential calculations.
Call to Action
We encourage you to delve deeper into these topics through hands-on exercises and practical applications. Keep experimenting with equations in C++ to build a strong programming foundation!