In this cpp tutorial, we will cover the basics of a simple C++ program that outputs "Hello, World!" to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
Setting Up Your Development Environment
Before you can dive into C++ programming, you'll need to set up your development environment properly. This includes choosing the right IDE (Integrated Development Environment) and installing a C++ compiler.
Choosing the right IDE: Some of the most popular IDEs for C++ include Visual Studio, Code::Blocks, and CLion. Each has its own set of features, so consider factors such as user interface, debugger capabilities, and community support when making your choice.
Installing a C++ Compiler: A C++ compiler is crucial for converting your code into executable programs. Three renowned compilers are GCC, Clang, and Microsoft C++ Compiler. Make sure to choose one that is compatible with your operating system and IDE.
Writing Your First C++ Program
Once your development environment is set up, it's time to write your first C++ program! A classic starting point is the "Hello, World!" program. This simple program will help you understand the basics of C++ syntax and structure.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, `#include <iostream>` allows you to use input and output streams. The `main` function is the entry point of any C++ program. When executed, it will display "Hello, World!" on the console.
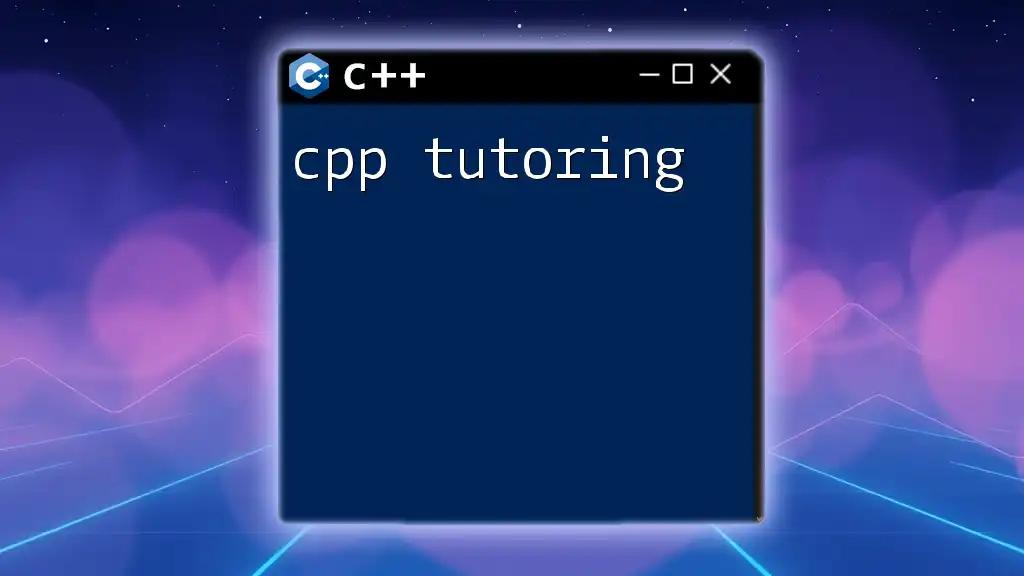
C++ Fundamentals
Variables and Data Types
In C++, variables are used to store data that can be manipulated within your program. Declaring variables involves specifying a data type, which determines the kind of data that can be stored.
Common data types in C++ include:
- int: For integers.
- float: For floating-point decimal numbers.
- char: For single characters.
- string: For strings of text (after including `<string>`).
Here’s a simple example of variable declarations:
int age = 25;
float height = 5.8;
char initial = 'A';
Understanding data types is fundamental to effective programming, as it affects how you can use and manipulate your variables.
Control Structures
Control structures allow you to dictate the flow of the program based on certain conditions. The two primary types are conditional statements and loops.
Conditional Statements
The if-else statement enables you to execute code based on a condition. For example:
if (age >= 18) {
cout << "Adult" << endl;
} else {
cout << "Minor" << endl;
}
This code evaluates whether the variable age is 18 or older, then prints either "Adult" or "Minor" accordingly.
Loops
Loops are used to execute a block of code multiple times. The for loop is particularly common:
for (int i = 0; i < 5; i++) {
cout << "Count " << i << endl;
}
In this example, the loop will print the numbers 0 through 4, making loops a fundamental part of programming.
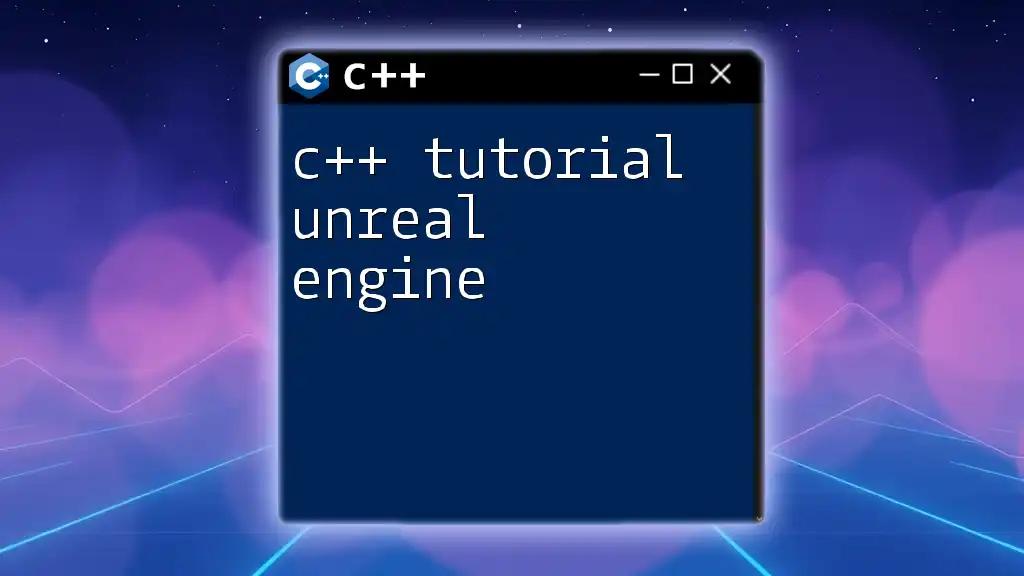
Functions in C++
Defining and Calling Functions
Functions are essential in programming, providing a way to encapsulate code that can be reused throughout your program. You can define a function and call it using its name.
Here’s a simple example:
void greet() {
cout << "Hello!" << endl;
}
int main() {
greet(); // Call the function
return 0;
}
The greet() function is defined to print "Hello!" when called, showcasing the concept of function reuse.
Function Parameters and Return Types
Functions can accept parameters and return values, making them versatile tools in your programming toolbox. Here’s how to define a function that takes parameters:
int sum(int a, int b) {
return a + b;
}
This function, sum, takes two integers and returns their sum, allowing for dynamic calculations based on user input or computations.
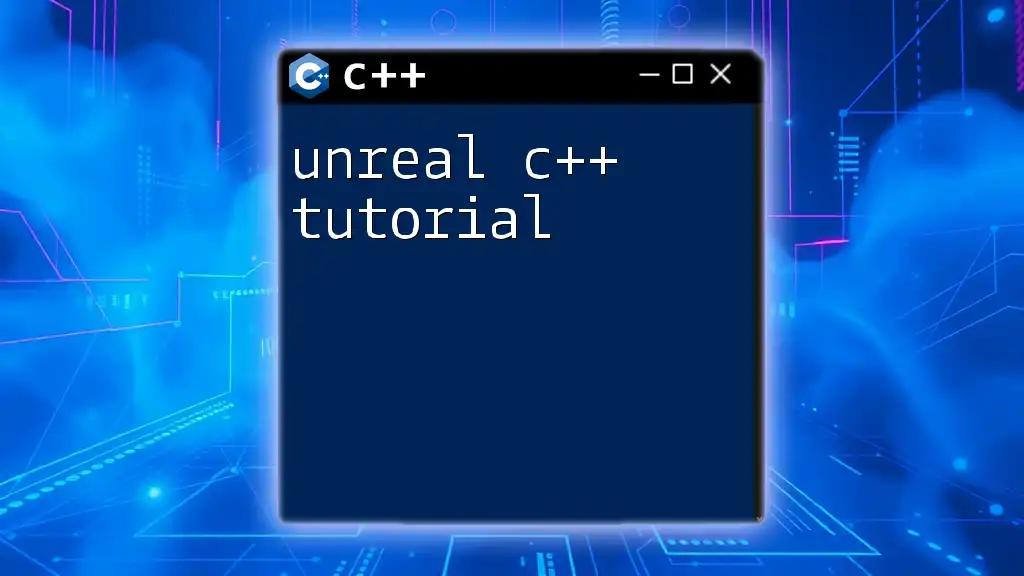
Object-Oriented Programming in C++
Understanding Classes and Objects
C++ is an object-oriented programming language, which means it encourages the use of classes and objects to organize code.
A class is a blueprint for creating objects. Here’s a basic example:
class Car {
public:
string color;
void honk() {
cout << "Beep Beep!" << endl;
}
};
In this snippet, the Car class has a color attribute and a honk method. You create an object of Car and use it as follows:
Car myCar;
myCar.color = "Red";
myCar.honk(); // Outputs: Beep Beep!
Inheritance and Polymorphism
Inheritance is a key aspect of object-oriented programming, allowing classes to inherit attributes and methods from other classes. This supports code reusability and organization.
Here’s an example of inheritance:
class Vehicle {
public:
string brand;
void honk() {
cout << "Honk! Honk!" << endl;
}
};
class Car : public Vehicle {
public:
string model;
};
In this example, Car inherits from Vehicle, making it easy to extend functionality without rewriting code.
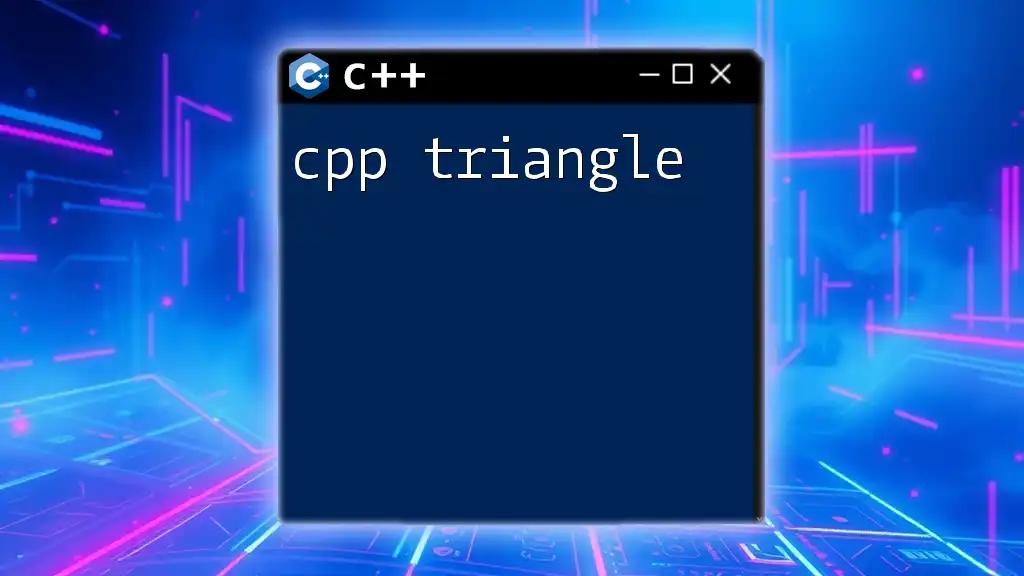
Advanced C++ Concepts
Pointers and Dynamic Memory
Pointers, which store the memory address of a variable, are critical for dynamic memory management.
For instance, you can allocate memory dynamically using new:
int* p = new int(10); // dynamic allocation
delete p; // deallocating memory
This code creates a new integer and assigns it the value 10, before deallocating the memory when it’s no longer needed. Understanding pointers is vital for effective C++ programming.
Templates and the Standard Template Library (STL)
C++ supports templates, allowing developers to write generic and reusable code. For instance, templates enable you to create functions or classes that work with any data type.
Consider this simple template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, the add function can accept any data type (int, float, etc.), enhancing its flexibility.
The Standard Template Library (STL) is a powerful feature of C++ that provides a rich set of functions and classes, such as lists, vectors, and algorithms, to facilitate efficient programming.
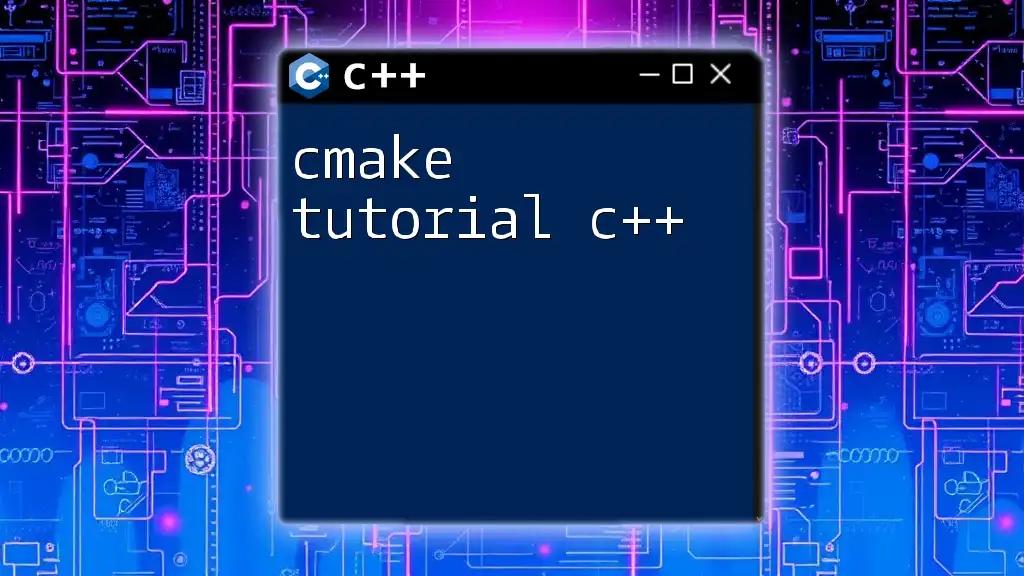
Conclusion
Recap of Key C++ Concepts
In this CPP tutorial, we've covered fundamental concepts of C++ programming. We explored writing your first program, understanding variables and data types, utilizing control structures, defining and calling functions, and exploring object-oriented features such as classes and inheritance.
Next Steps for Learning C++
As you continue your journey into C++, consider utilizing various resources. Books, online courses, and active programming forums are invaluable for deepening your understanding. Regular practice will solidify your skills, allowing you to tackle more complex programming challenges effectively.
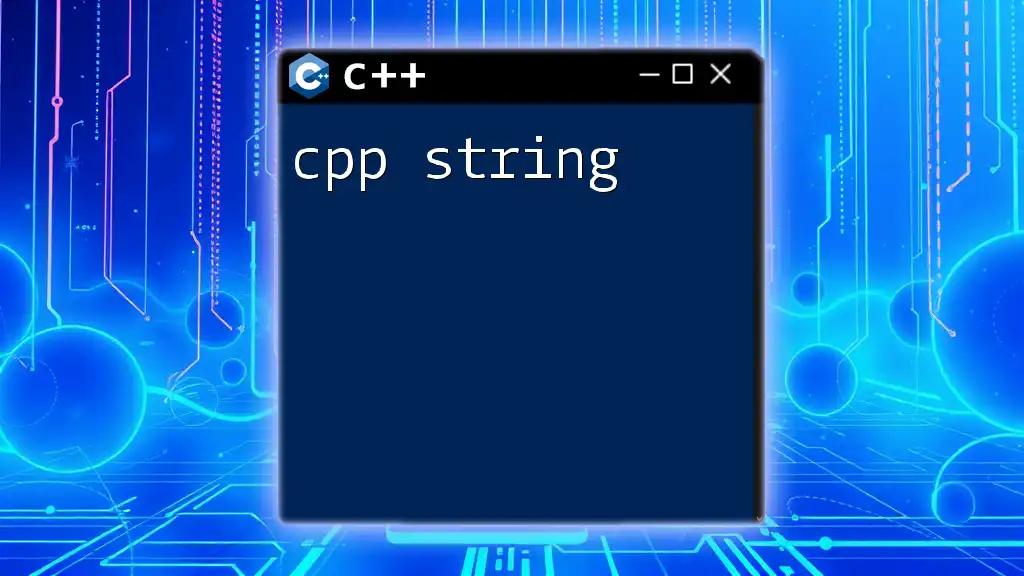
FAQs About C++ Programming
In this section, we’ll address some common questions that beginners often have to clarify any confusion regarding C++ programming fundamentals. Remember, consistent practice and engagement with the C++ community will ensure your growth as a proficient programmer.