In this OpenGL tutorial for C++, you'll learn how to create a simple window and render a triangle using essential OpenGL commands.
#include <GL/glew.h>
#include <GLFW/glfw3.h>
void render() {
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glVertex2f(-0.5f, -0.5f);
glVertex2f(0.5f, -0.5f);
glVertex2f(0.0f, 0.5f);
glEnd();
}
int main() {
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL Tutorial", NULL, NULL);
glfwMakeContextCurrent(window);
glewInit();
while (!glfwWindowShouldClose(window)) {
render();
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwDestroyWindow(window);
glfwTerminate();
return 0;
}
What is OpenGL?
OpenGL stands for Open Graphics Library. It is a cross-platform graphics API that enables developers to render 2D and 3D graphics. Established in the early 1990s, OpenGL has become a key tool in the game development and computer graphics industry. Its flexibility and efficiency make it an essential component for applications that require high-performance graphics rendering.
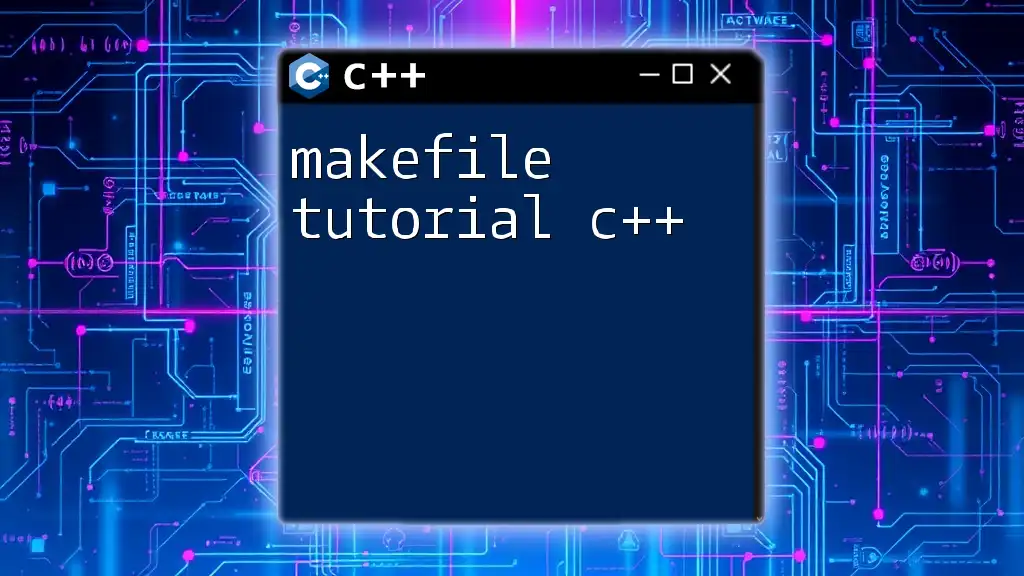
Why Use OpenGL with C++?
OpenGL is especially popular among C++ developers for several reasons:
- Performance: OpenGL provides direct hardware access, allowing for optimized rendering and performance.
- Cross-Platform Compatibility: OpenGL can run on various operating systems, including Windows, macOS, and Linux, making it a flexible choice for cross-platform game development.
- Wide Adoption: Many resources, tutorials, and community support are available for OpenGL, making it easier for developers to find help and documentation.
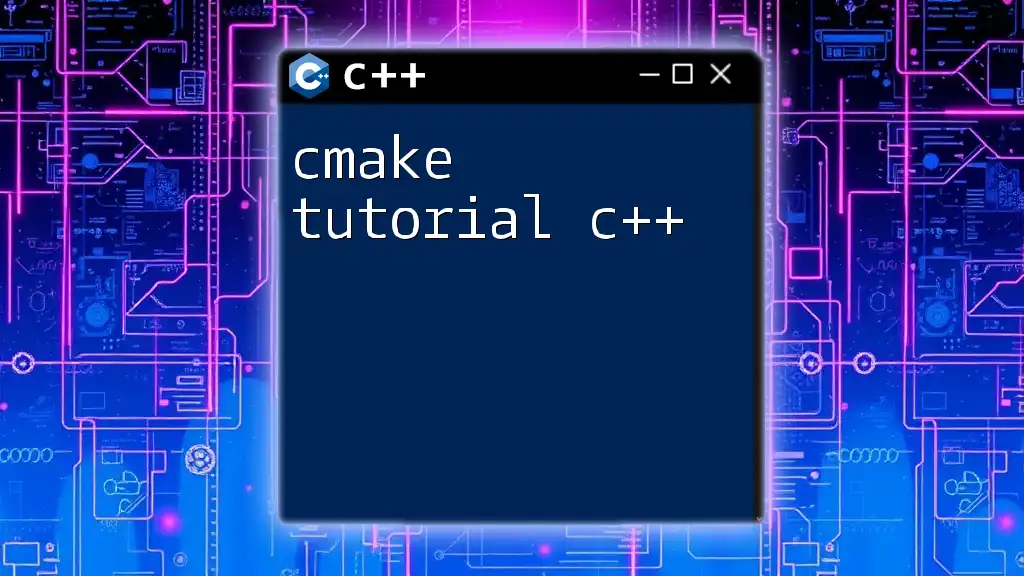
Setting Up Your Environment
System Requirements
Before diving into development, ensure your system meets the following requirements:
- Operating Systems: Supports Windows, macOS, and Linux.
- Minimum Hardware: Graphics card with OpenGL support (most modern graphics cards will suffice).
Installing OpenGL
Here’s how to install OpenGL on different platforms:
- Windows: Download the GLEW and GLFW libraries and use an installer like vcpkg to manage dependencies.
- macOS: OpenGL comes pre-installed, but you can manage dependencies using Homebrew.
- Linux: Install OpenGL using your package manager, often bundled with Mesa libraries.
Setting Up a C++ Development Environment
Choose your preferred Integrated Development Environment (IDE) such as:
- Visual Studio (Windows)
- Code::Blocks (Cross-platform)
- CLion (Cross-platform with CMake)
To utilize OpenGL libraries effectively, you need to link the GLEW and GLFW libraries to your projects. Most IDEs have steps for adding these dependencies in their project settings.
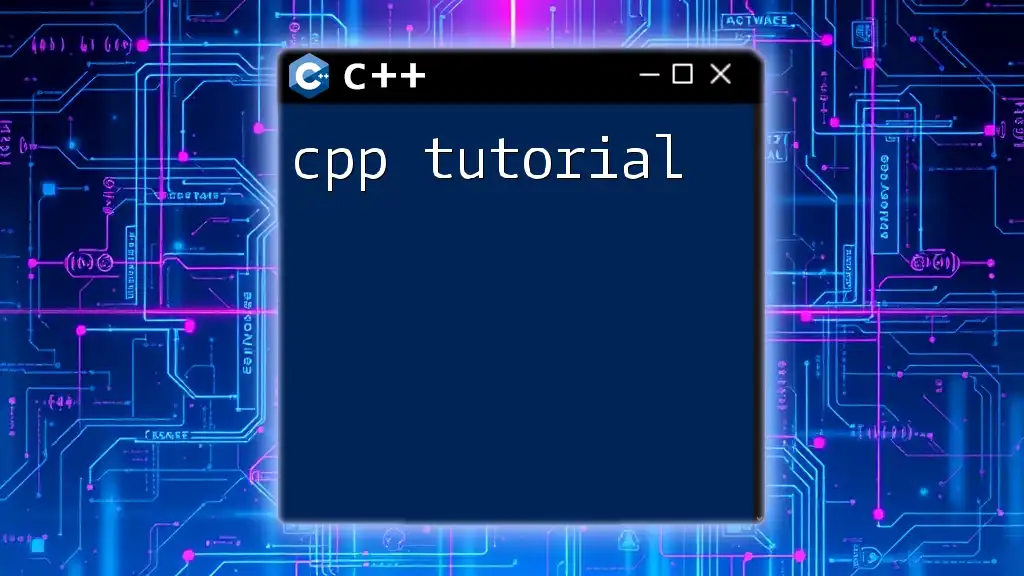
Getting Started with OpenGL
Your First OpenGL Program
Creating your first window with OpenGL is a monumental step. Below is an example code snippet that demonstrates how to create a basic OpenGL window using GLFW:
#include <GL/glew.h>
#include <GLFW/glfw3.h>
int main() {
glfwInit();
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL", NULL, NULL);
if (window == NULL) {
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
return 0;
}
In this code, we initialize GLFW, create a window, and enter a loop that keeps the window open until it’s closed. The main rendering occurs inside the loop, where we clear the color buffer.
Understanding the Rendering Pipeline
OpenGL operates under a structured rendering pipeline composed of several stages:
- Vertex Processing: This stage processes vertices, transforming them from object space to screen space.
- Primitive Assembly: Vertices are grouped into shapes like points, lines, or triangles.
- Rasterization: Converts geometric shapes into pixels.
- Fragment Processing: Colors are assigned to pixels, considering texture and lighting details.
Understanding this pipeline is crucial for optimizing your OpenGL applications effectively.
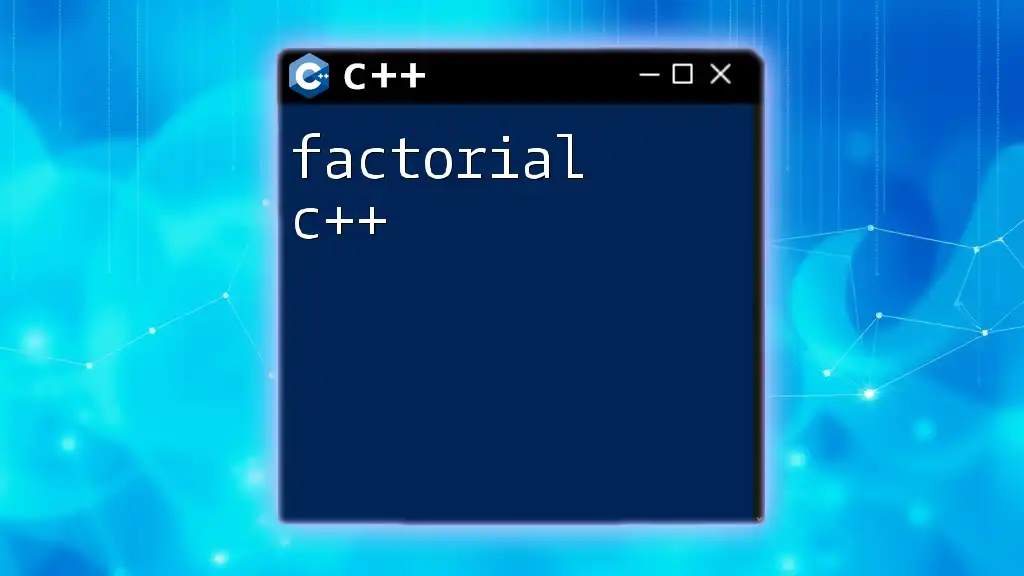
Drawing 2D Shapes
Creating 2D Shapes with OpenGL
Drawing 2D shapes requires defining vertex buffers. Here’s how to draw a simple triangle:
GLfloat vertices[] = {
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
0.0f, 0.5f, 0.0f
}; // Define vertices
GLuint VBO, VAO;
glGenVertexArrays(1, &VAO);
glGenBuffers(1, &VBO);
glBindVertexArray(VAO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(GLfloat), (GLvoid*)0);
glEnableVertexAttribArray(0);
In this snippet, we define the vertices of a triangle and set up Vertex Array Object (VAO) and Vertex Buffer Object (VBO). This setup is crucial for rendering the shapes efficiently.
Texturing in OpenGL
Textures can bring your 2D shapes to life. Applying a texture involves uploading texture data as a 2D image and using texture coordinates. Learn how to create a texture object and map it to your shapes through specific texture functions and shader manipulation.
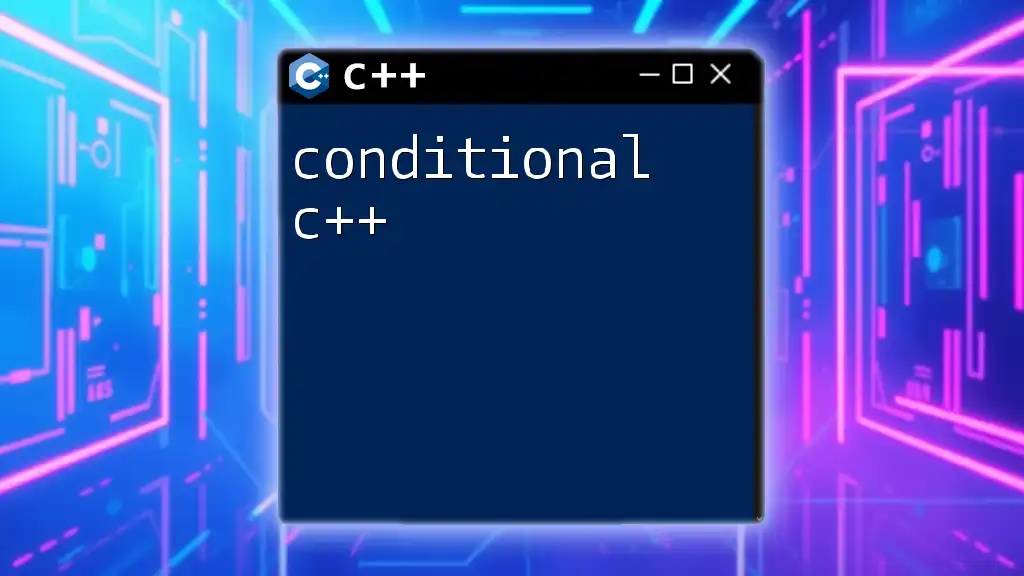
Introduction to 3D Graphics
Basics of 3D Rendering
Moving into 3D graphics requires you to understand the importance of depth. Unlike in 2D graphics, 3D rendering utilizes z-coordinates to create depth perception, relying on a depth buffer to manage visibility.
Creating a 3D Object
To draw a 3D cube, define its vertices appropriately. Here’s a basic starter snippet:
GLfloat cubeVertices[] = {
// Define cube vertices here
};
Every face of the cube must be defined through the vertices in 3D space, ensuring all six faces are accounted for to project the cube correctly on screen.
Camera and Projection Matrices
Camera positions and projections significantly impact the rendered scene. You'll need to establish both perspective and orthographic projections:
- Perspective Projection: Mimics the human eye's perception, making distant objects smaller.
- Orthographic Projection: Provides a more technical rendering without perspective distortion.
Create a view matrix that represents the camera's position and orientation in the 3D space.
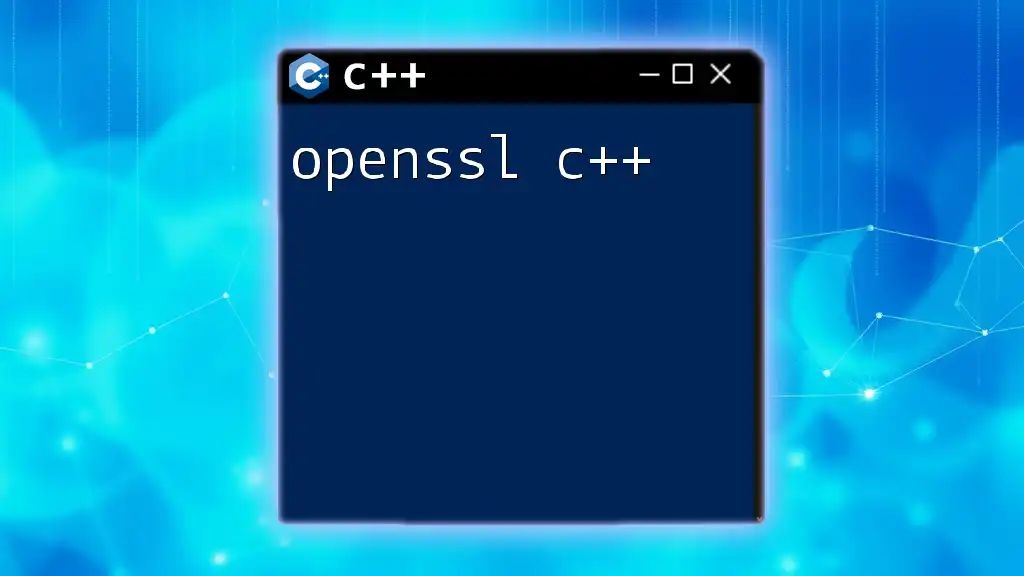
Advanced OpenGL Techniques
Shaders in OpenGL
Shaders are small programs that run on the GPU. They play a crucial role in customizing the graphics processing pipeline. You will primarily work with two types:
- Vertex Shaders: Handle vertex transformation.
- Fragment Shaders: Responsible for pixel coloring.
Here’s a simple vertex shader example:
#version 330 core
layout(location = 0) in vec3 position;
void main() {
gl_Position = vec4(position, 1.0);
}
This shader receives vertex positions and transforms them for rasterization.
Handling Input
Keyboard and mouse inputs are crucial for interactive applications. Using GLFW, you can handle inputs with ease. Set up keyboard callbacks to respond to user inputs effectively, enhancing the interactivity of your graphics.
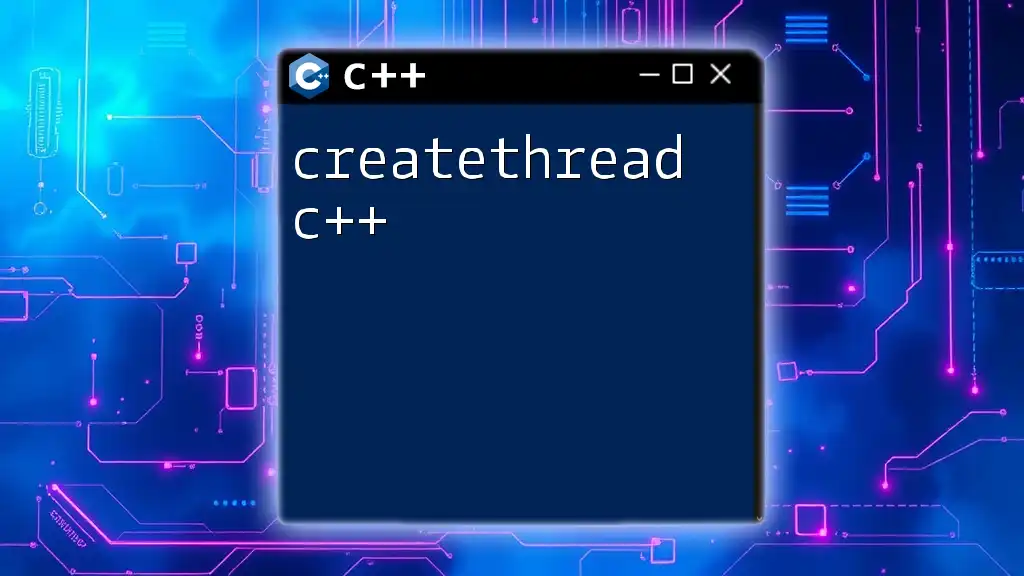
Troubleshooting Common Issues
Common Errors in OpenGL
As you code, you might encounter some common mistakes:
- Shader Compilation Errors: Always check error logs when shaders fail to compile.
- Incorrect Matrix Transforms: Double-check your matrix multiplications; they drastically affect rendering results.
Optimization Techniques
Optimize your OpenGL applications by:
- Profiling performance to identify bottlenecks.
- Reducing state changes during rendering.
- Utilizing instanced rendering for multiple objects with the same data.
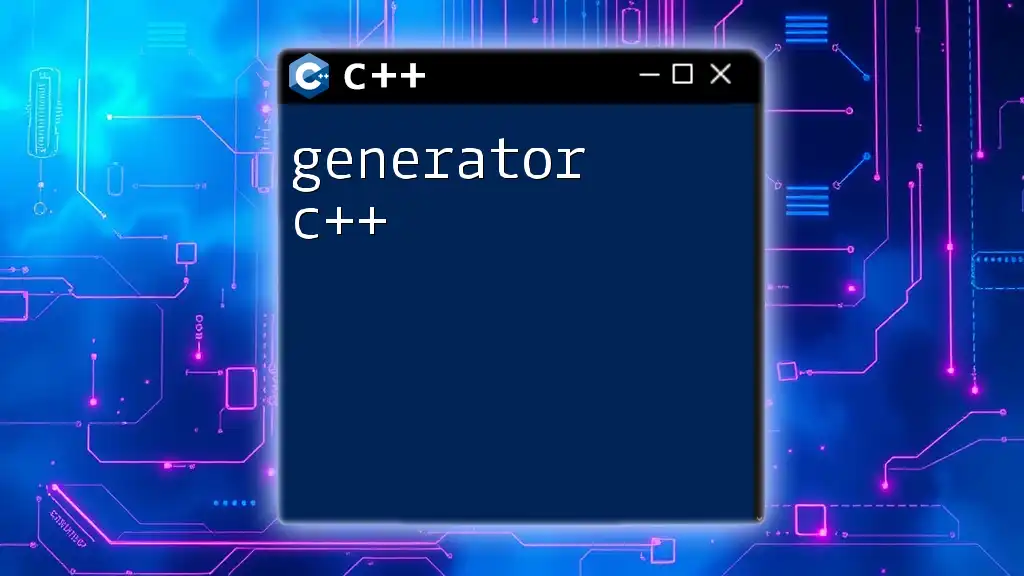
Conclusion
The world of OpenGL and C++ is vast, filled with endless possibilities. Understanding the fundamental concepts outlined in this tutorial allows you to embark on your journey into graphics programming. Experiment with shaders, 3D models, and more to expand your skills further. With commitment and practice, OpenGL will undoubtedly become an invaluable tool in your development arsenal.
Additional Resources
Explore further learning through recommended books, websites, and community forums. Search GitHub repositories for sample projects to solidify your understanding of OpenGL Tutorial C++ and enhance your practical skills in real-world scenarios.