Manipulators in C++ are special functions used to format input and output operations, allowing you to control how data is displayed or processed, such as adjusting the precision of floating-point numbers or adding line breaks.
Here’s a code snippet demonstrating the use of the `std::endl` and `std::fixed` manipulators:
#include <iostream>
#include <iomanip> // Required for std::setprecision
int main() {
double value = 123.456789;
// Using manipulators to set precision and print the value
std::cout << std::fixed << std::setprecision(2) << value << std::endl; // Outputs: 123.46
std::cout << "Hello World!" << std::endl; // Outputs: Hello World!
return 0;
}
What Are C++ Manipulators?
Manipulators are functions that influence the behavior of input and output streams in C++. They allow you to format text and data, enhancing the representation of the information displayed on the console or redirected to files. Understanding how to effectively utilize manipulators in C++ can greatly improve the readability and aesthetic of your code output, making it more user-friendly and visually pleasing.
Key Characteristics
- Functionality: Manipulators can change various attributes of output streams, such as width, precision, and formatting style.
- Stream Manipulation: They can be used seamlessly within the context of I/O streams, allowing you to embed them directly in your output statements.
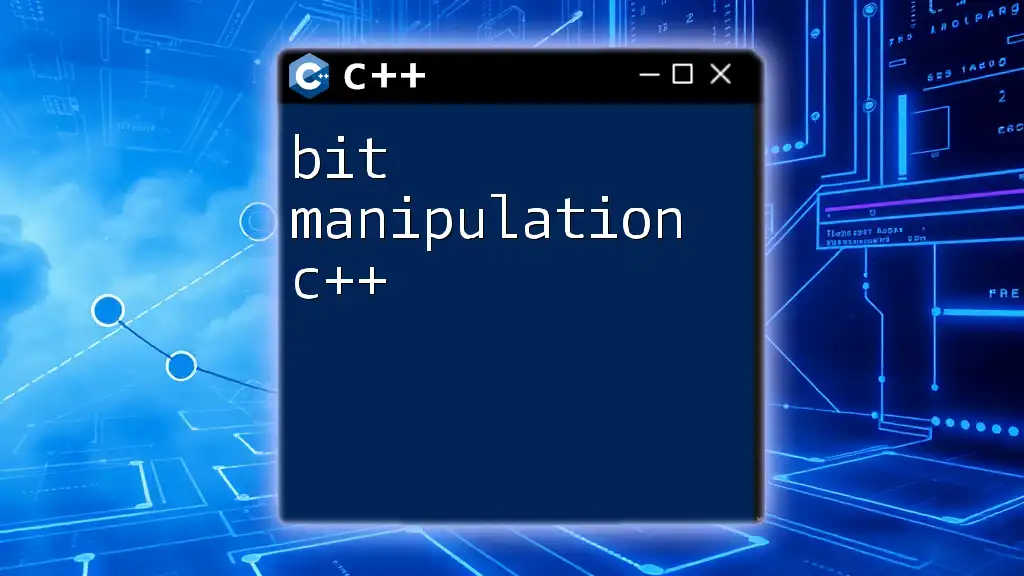
Why Use Manipulators in C++?
Improved Readability
Using manipulators enhances the overall readability of your output. By aligning text and data neatly, your console outputs become easier to interpret for users.
Control Over Output Format
In situations where specific formatting is crucial, such as displaying monetary values or presenting tabular data, manipulators provide fine control. This customization ensures your output is not only accurate but also visually appealing.
Consistency in Output
Employing manipulators helps maintain consistency in the presentation of output across different parts of an application. When outputs are consistently formatted, it keeps user experiences predictable and professional.
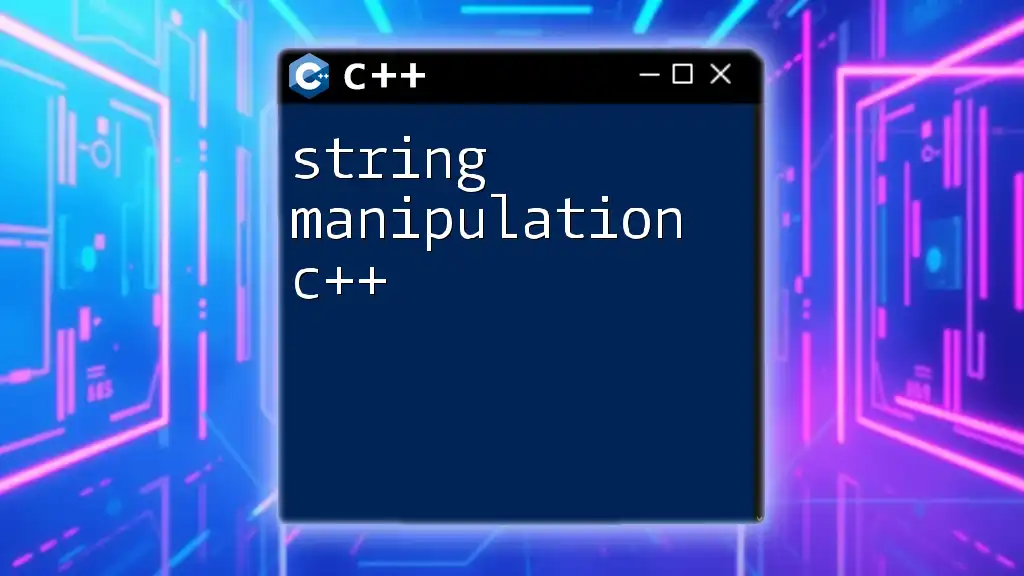
Types of C++ Manipulators
Standard Manipulators
std::endl
The `std::endl` manipulator is used to insert a new line in the output stream and to flush the output buffer. This is particularly useful for ensuring that data written to the console is immediately visible.
Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, "Hello, World!" is printed, followed by a line break. Flushing the output buffer ensures that no data is left buffered, which can be crucial in real-time applications.
std::setw
The `std::setw` manipulator sets the width of the next output field. This is useful when you want to align output in a tabular form.
Example:
#include <iostream>
#include <iomanip> // For std::setw
int main() {
std::cout << std::setw(10) << "Name" << std::setw(10) << "Age" << std::endl;
std::cout << std::setw(10) << "Alice" << std::setw(10) << 30 << std::endl;
return 0;
}
In this example, the names and ages align nicely, making it easy to read. The setw manipulator provides a meaningfully structured output.
std::setprecision
The `std::setprecision` manipulator is primarily used for controlling the number of digits displayed after the decimal point when dealing with floating-point numbers.
Example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::setprecision(3) << pi << std::endl;
return 0;
}
With this code, "3.14" is outputted, showcasing how precision affects numerical representation. Setting precision is critical in applications involving finance or scientific calculations.
User-Defined Manipulators
Creating custom manipulators can be beneficial for specific output needs. They encapsulate frequently repeated formatting logic into reusable functions, promoting cleaner code.
Example of a User-Defined Manipulator
Example:
#include <iostream>
#include <iomanip>
// Custom manipulator to display a header
std::ostream& header(std::ostream& os) {
return os << "=== Custom Header ===" << std::endl;
}
int main() {
std::cout << header; // Using the custom manipulator
return 0;
}
In this example, the `header` manipulator inserts a custom formatted string at the point it's called. Such manipulators can encapsulate branding or context-specific formatting requirements.
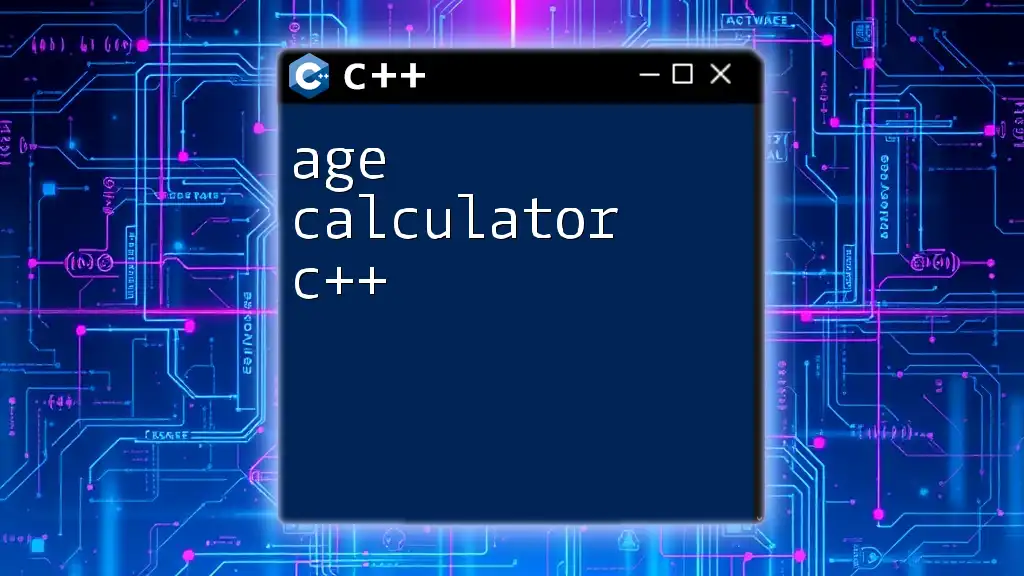
Combining Multiple Manipulators
Chaining manipulators allows you to combine their effects on a single output, creating sophisticated formatting in a single line.
Example:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << std::setprecision(2) << std::setw(10) << num << std::endl;
return 0;
}
Here, `std::fixed`, `std::setprecision(2)`, and `std::setw(10)` are all applied concurrently, ensuring that the output is a fixed-point decimal rounded to two digits with assigned width for neat alignment. The order of manipulators can significantly influence output formatting, so it’s crucial to apply them wisely.
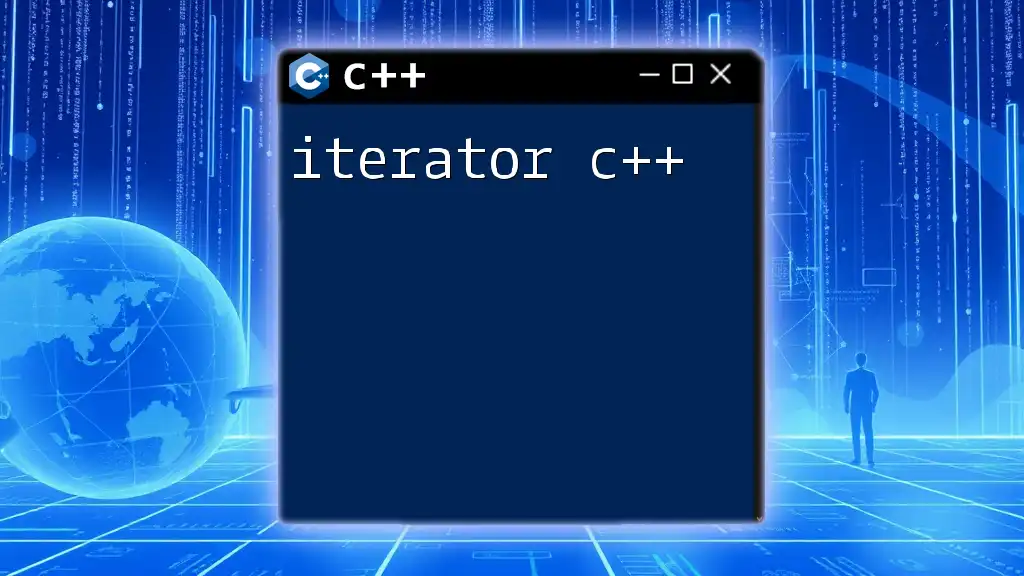
Manipulator Limitations and Considerations
While manipulators are powerful tools, there are limitations and considerations to keep in mind:
- Context-Specific Application: Manipulators affect only the stream they are directly applied to, meaning their effects do not extend beyond that single output statement.
- Performance: In performance-critical applications, excessive or unnecessary use of manipulators could slow down output operations, especially in high-frequency print cycles.
- Compiler Variations: There might be slight differences in behavior across various compilers or standards (such as C++11, C++14, etc.), so testing across different environments is wise.
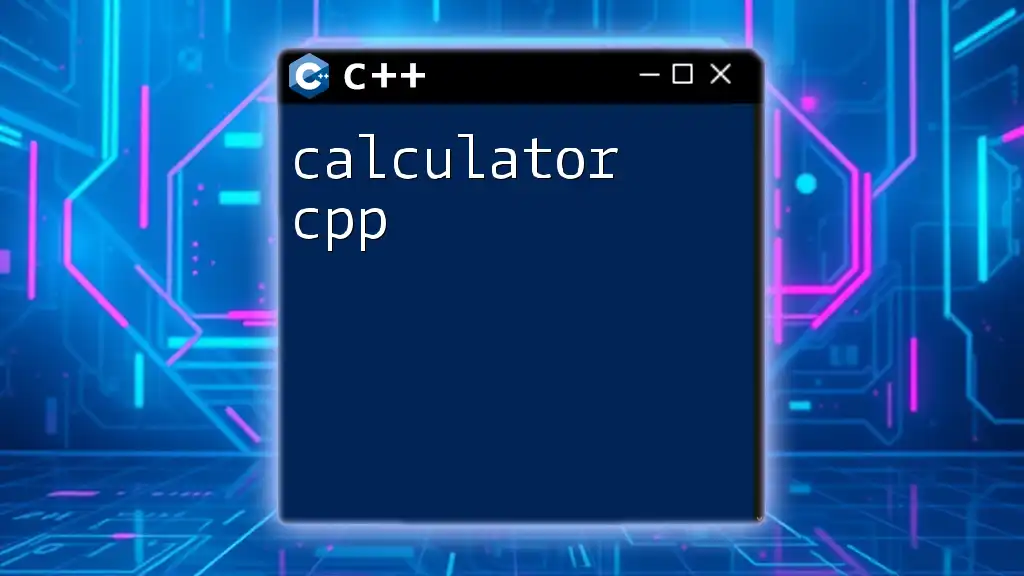
Best Practices for Using Manipulators in C++
To ensure the effective use of manipulators in your code:
- Emphasize Readability: Keep output formatting consistent and intuitive, promoting better comprehension for the end user.
- Comment Thoroughly: When using complex manipulator combinations, clarify your intention in comments. This will help anyone reading your code understand the formatting logic.
- Use Sparingly: While manipulators are helpful, don’t overuse them; maintain a balance between functionality and readability. Avoid convoluted lines of code with nested manipulators.
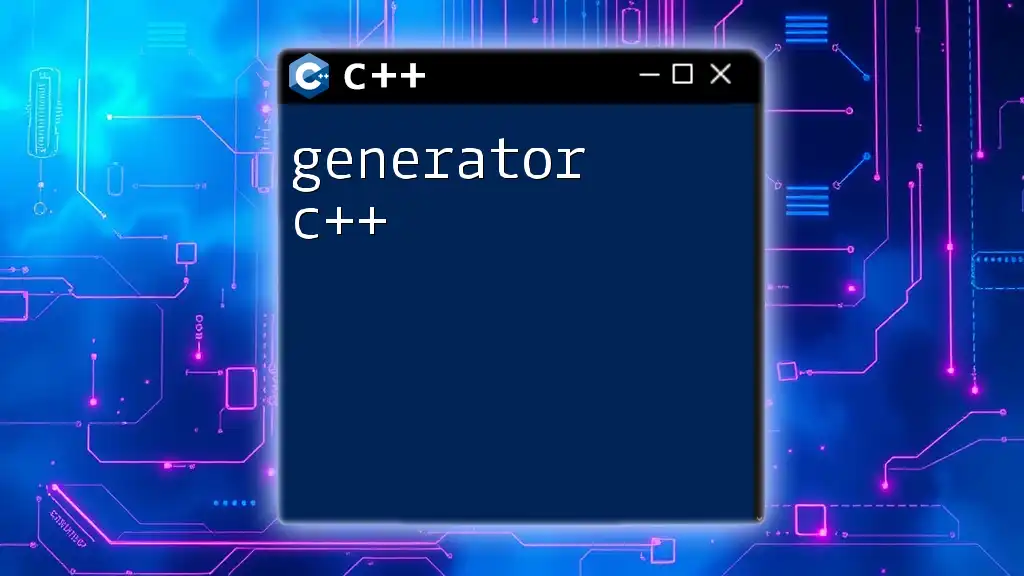
Conclusion
Manipulators in C++ are invaluable for customizing output, maintaining consistency, and enhancing the overall readability of your code. By practicing their usage, you increase your efficiency in C++ programming, laying the groundwork for writing clearer and more professional applications. Embrace manipulators as a tool to streamline your output management and enhance the user experience.