Compilation in C++ is the process of transforming source code written in C++ into executable machine code using a compiler.
Here’s a simple code snippet that demonstrates how to compile a C++ program using the g++ compiler:
g++ -o my_program my_program.cpp
Understanding Compilation
What is Compilation?
Compilation is the process of converting high-level source code written in languages like C++ into machine code that the computer can execute. Unlike interpreted languages, where the code is executed line-by-line, C++ requires this initial step of translation.
Stages of Compilation
The compilation process can be broken down into several stages, each with its own specific roles.
Preprocessing
During the preprocessing phase, the compiler handles directives that begin with a `#`. This includes commands like `#include` to import libraries and `#define` to create macros. For example:
#include <iostream>
#define PI 3.14159
In this stage, the preprocessor expands these directives, preparing the code for the next phase, which is the actual compilation.
Compilation
The compilation stage transforms the preprocessed code into object code, which is a binary representation that the machine understands. Here, the compiler checks for syntax errors, type mismatches, and other critical errors.
For instance, consider a simple C++ program saved as `hello.cpp`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
When this file is compiled, the compiler will generate an object file (`hello.o` or similarly) that contains machine-readable code.
Linking
The linking stage combines various object files into a single executable. This process resolves external references, such as function calls from libraries or other object files.
Linking can be static, where all library code is included in the executable, or dynamic, where the libraries remain separate. For example, a program that uses the standard math library may need linking depending on how it’s structured.
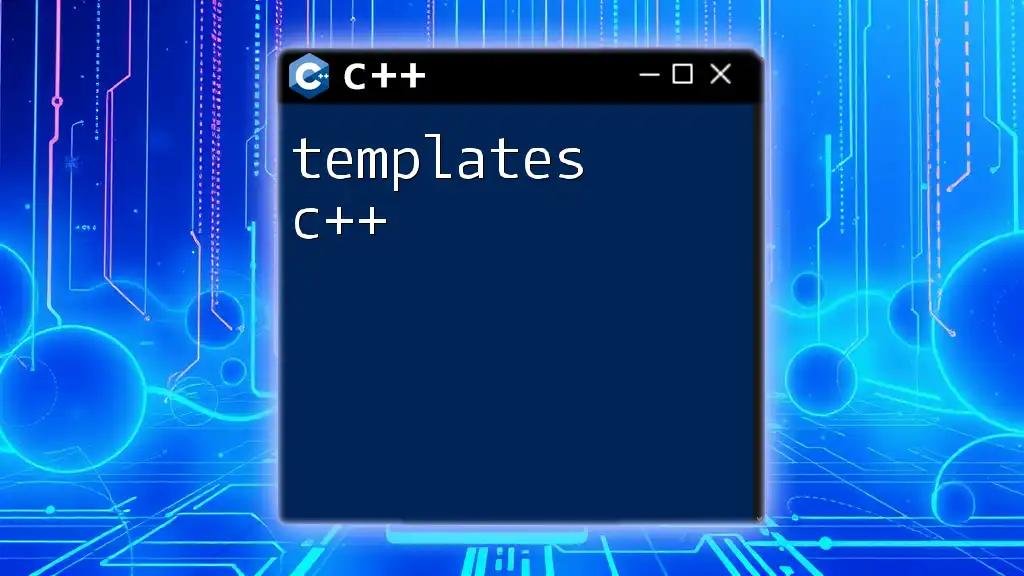
The C++ Compilation Process
The Role of the Compiler
Different C++ compilers offer varied features and performance. Popular choices include GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++). Each compiler has specific command-line flags that can significantly alter the compilation behavior and performance.
For example, using GCC, you might compile with optimization like this:
g++ -O2 -o my_program my_program.cpp
Steps in the Compilation Process
Source Code Writing
Writing clean and well-structured C++ code is crucial. Adopting best practices enhances the compilation process and reduces the likelihood of errors. For instance, using proper naming conventions, indentation, and comments makes your code more readable.
A simple structure would be:
#include <iostream>
int main() {
// This is the entry point of the program
std::cout << "Example of best practices in C++" << std::endl;
return 0;
}
Compilation Command-Line
To compile C++ programs via the command line, using commands is essential. For instance, to compile the `hello.cpp` file, you would use:
g++ -o hello hello.cpp
The command above instructs GCC to compile `hello.cpp` and create an executable named `hello`.
Error Handling during Compilation
Common errors during compilation can range from simple syntax mistakes to complex type mismatches. Understanding compilation error messages is important for debugging. A typical error message might look like this:
hello.cpp: In function 'int main()':
hello.cpp:5:5: error: 'cout' was not declared in this scope
cout << "Hello, World!" << endl;
This indicates that the compiler could not recognize `cout`. In this case, ensuring the proper library is included (like `<iostream>`) resolves the issue.
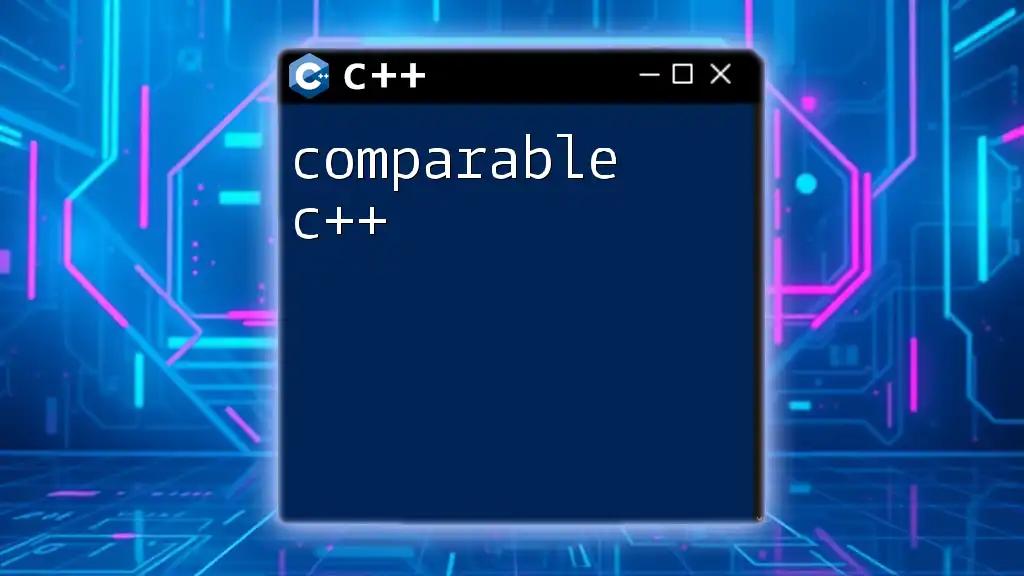
Compilation Tools and Techniques
Integrated Development Environments (IDEs)
Using an IDE can simplify the compilation process significantly. IDEs like Visual Studio, Code::Blocks, and CLion provide features such as syntax highlighting, integrated terminal, debugging tools, and one-click compilation. These tools help you identify errors quickly and streamline your workflow.
Build Systems
Build systems like Makefiles and CMake offer structured ways to manage the compilation of complex projects. They automate the build process, allowing developers to focus on coding instead of managing individual compilation commands.
Here’s an example CMake configuration file (`CMakeLists.txt`):
cmake_minimum_required(VERSION 3.10)
project(MyProgram)
set(CMAKE_CXX_STANDARD 14)
add_executable(my_program main.cpp)
Continuous Integration
Using Continuous Integration (CI) systems enhances the compilation and deployment pipeline. CI tools like Jenkins and GitHub Actions ensure that code is automatically built and tested upon commit. This not only catches errors early but also encourages better coding practices.
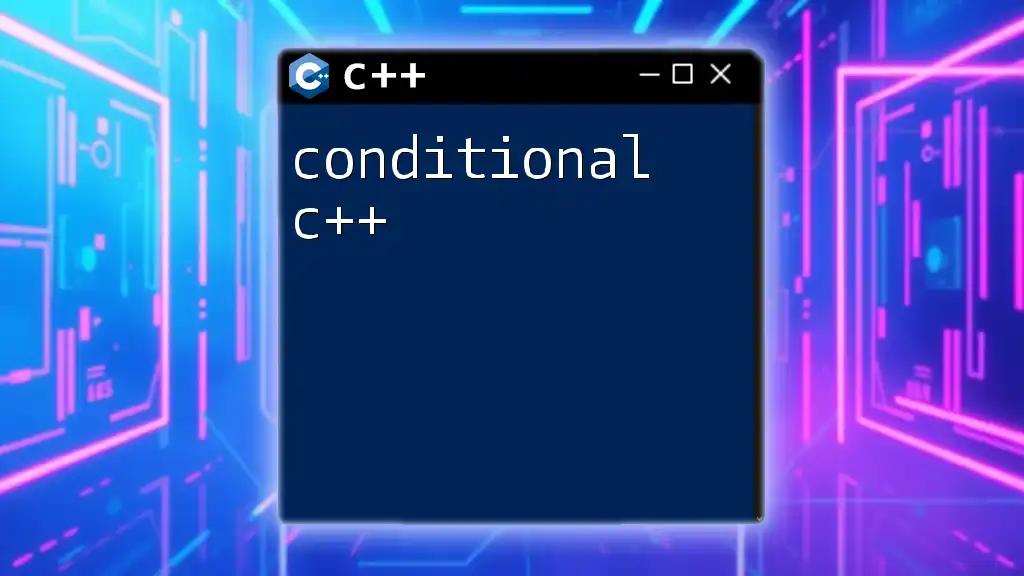
Advanced Compilation Topics
Optimizations during Compilation
Optimizing code during compilation can greatly enhance performance. The compiler provides various optimization levels. For example:
- `-O1`: Basic optimizations
- `-O2`: Further optimizations that are not time-consuming
- `-O3`: Aggressive optimizations, possibly increasing compilation time
Using these flags allows developers to strike a balance between compilation time and runtime efficiency:
g++ -O3 -o optimized_program main.cpp
Cross-Compilation
Cross-compilation refers to the process of compiling code on one platform to run on another. This is useful for developing software intended for different operating systems or architectures. For instance, compiling a C++ program on a Windows PC to run on an ARM-based Raspberry Pi can be achieved with the right toolchain.
Common Compilation Issues and Fixes
Compilation can often lead to frustrations, particularly with linking errors or conflicting definitions.
-
Linking Errors: Usually arise from unresolved references. Ensuring that all necessary libraries are included during linking can resolve these issues.
-
Multiple Definitions: Result from including the same header file multiple times without proper guards. The solution is to use header guards or `#pragma once`.
Adhering to best practices for managing dependencies and structuring code helps minimize these problems.
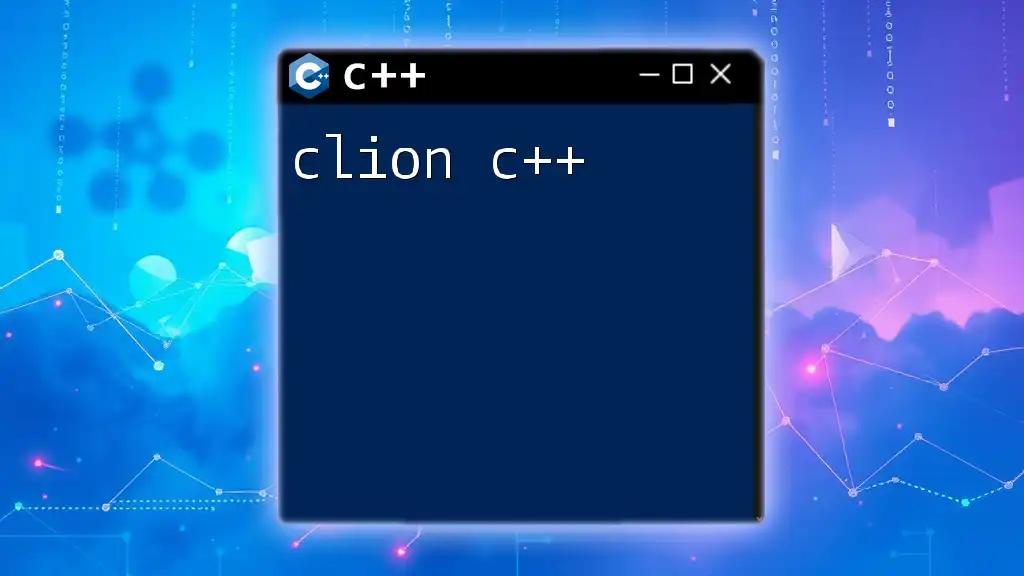
Conclusion
The compilation c++ process is a multi-faceted journey that plays a crucial role in turning source code into executable programs. Understanding the stages of compilation—preprocessing, compiling, and linking—equips programmers with knowledge that can significantly enhance their coding efficiency.
By practicing the outlined topics and tools, developers can streamline their workflows, resolve errors more effectively, and write better C++ code. As you continue to explore and practice, remember that familiarity with compilation will empower you to become a more proficient C++ programmer.
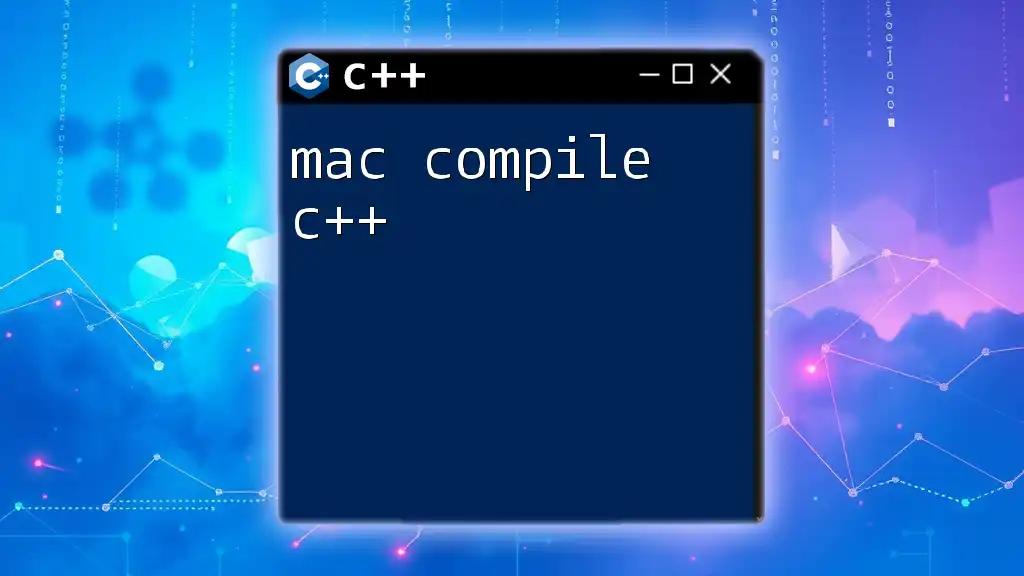
Additional Resources
For further reading and resources, consider exploring books and online tutorials specifically focused on C++ programming and compilation. Joining community forums can also provide additional support as you delve deeper into the world of C++.