The g++ compiler is a key tool in C++ programming that allows developers to compile their C++ source code into executable programs.
Here's an example of a simple C++ program and how to compile it using g++:
// hello.cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile the above program, use the following g++ command in your terminal:
g++ hello.cpp -o hello
This command compiles `hello.cpp` and generates an executable named `hello`.
What is g++?
g++ is the GNU C++ Compiler, a crucial part of the GNU Compiler Collection (GCC). It is a free and open-source compiler widely used for compiling C++ programs. Developed under the GNU Project, g++ supports various C++ standards, including C++11, C++14, C++17, and C++20.
The history of g++ dates back to the late 1980s when it was introduced to facilitate the development of C++ programs on UNIX platforms. As C++ evolved, so did g++, which continually adapted to incorporate new features and ensure compliance with the evolving language standards.
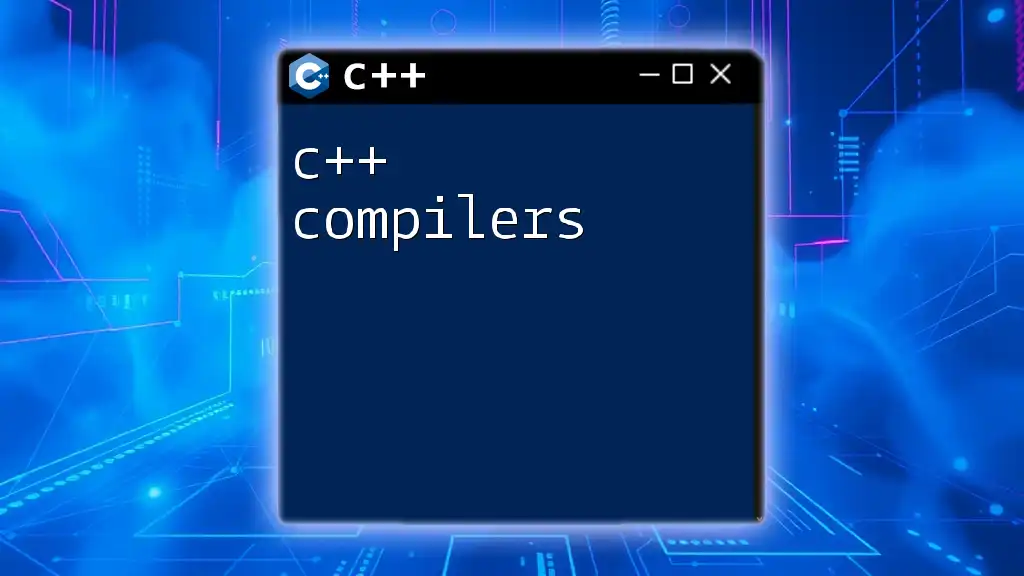
Why Use g++?
Open-source Benefits
One of the main reasons developers prefer the g++ compiler is its open-source nature. This makes it a cost-effective solution since there are no licensing fees, allowing developers to focus on coding rather than financial hurdles. The collaborative community behind g++ furthers this benefit, providing extensive documentation, tutorials, and support.
Cross-platform Compatibility
g++ boasts impressive cross-platform compatibility, making it available on various operating systems such as Linux, Windows, and macOS. This capability allows developers to write code on one platform and compile it on another, ensuring versatility and ease of collaboration. For instance, a developer can write and compile a C++ project on Linux and then share it with a Windows user without facing significant integration issues.
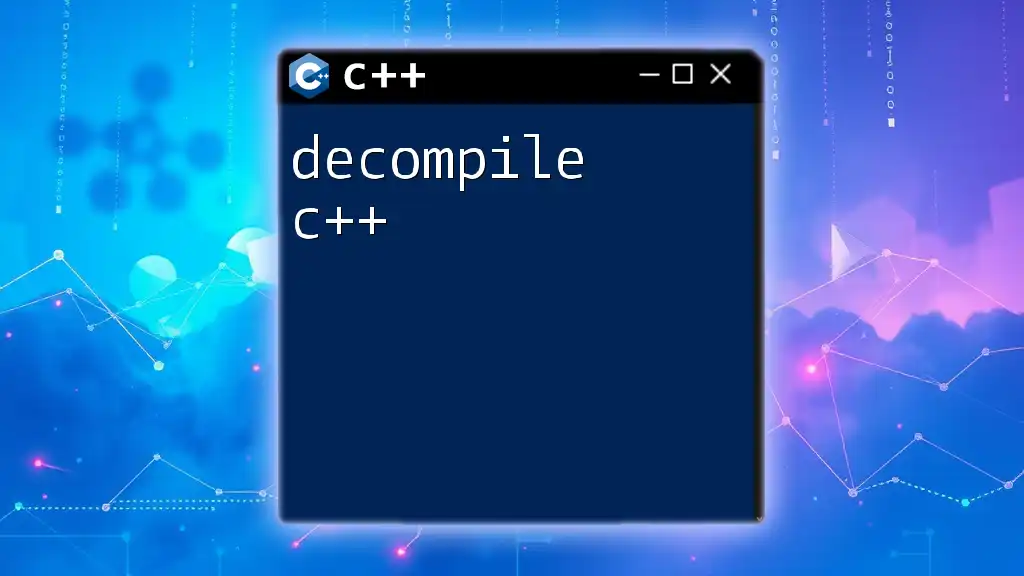
Installation of g++
Requirements
Before installing g++, you need to ensure that your system meets the necessary requirements. Generally, g++ can be easily installed on Linux distributions, Windows (via MinGW or Cygwin), and macOS (using Homebrew).
Installation Steps
Linux
On most Linux distributions, you can install g++ using the package manager with the following command:
sudo apt-get install g++
Windows
To install g++ on Windows, you can use MinGW. Here's a simplified rundown:
- Download and install MinGW from the official website.
- During installation, select the "g++" component.
- Add MinGW's bin directory to your system's PATH.
After installation, verify it using:
g++ --version
macOS
If you are using macOS, you can install g++ easily through Homebrew. Simply run:
brew install gcc
Check the installation by running:
g++ --version
Verifying Installation
To confirm that g++ is correctly installed, use the following command:
g++ --version
You should see the version number and other details about the installation. If not, revisit your installation steps.
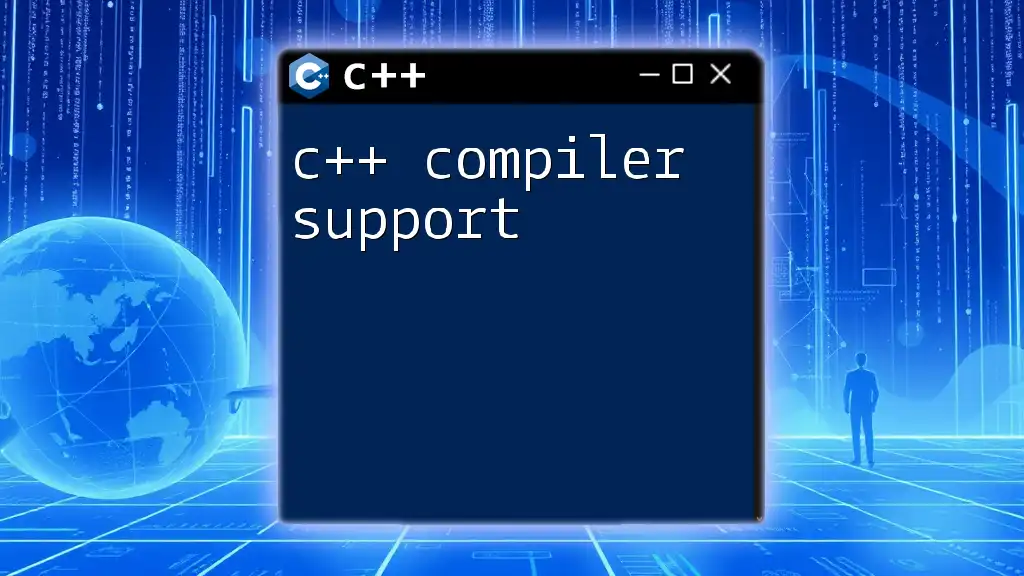
Basic g++ Commands
Compiling a Simple C++ Program
To illustrate the power of g++, let’s compile a simple "Hello, World!" program. Below is a basic C++ program sample:
#include<iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this program, save it in a file named `hello.cpp` and execute the following command:
g++ hello.cpp -o hello
Running the Compiled Program
After a successful compilation, you can run the program using:
./hello
You should see the output: Hello, World!
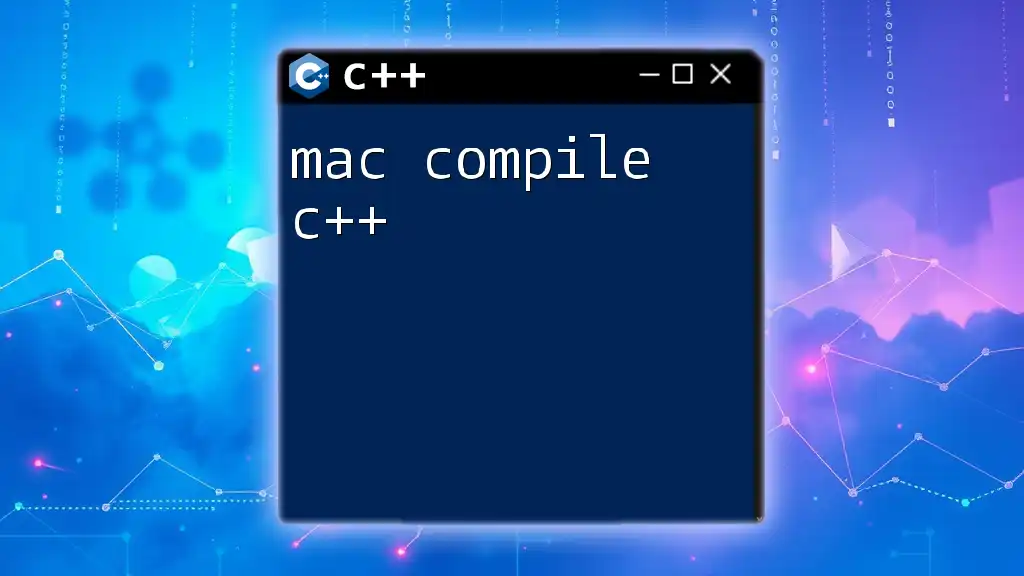
Advanced g++ Compile Options
Using Flags and Options
g++ provides various flags and options that enhance the functionality of the compilation process. For example:
- `-Wall`: Enables all warnings.
- `-O2`: Optimizes the code for speed.
You can use these flags together while compiling:
g++ -Wall -O2 hello.cpp -o hello
Debugging with g++
If you encounter issues while running your program, it is helpful to compile it with the `-g` option, which includes debugging information. This can be crucial for stepping through code:
g++ -g hello.cpp -o hello
You can then use debugging tools like gdb to identify issues in your code.
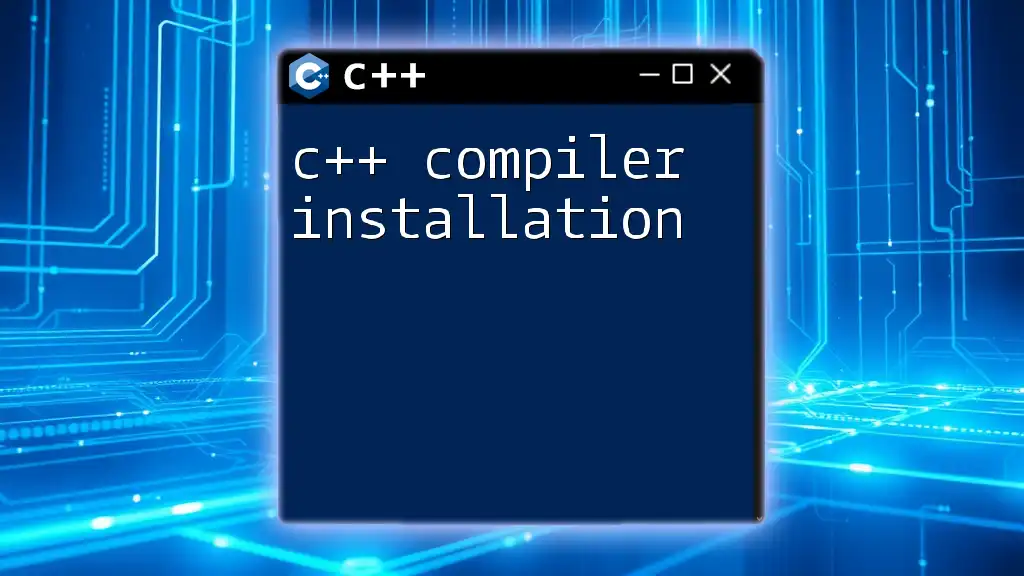
Linker and Libraries in g++
Linking External Libraries
When working with external libraries, understanding the concepts of static and dynamic linking is vital. For instance, a common library used is the math library. To link to it, your command would look like this:
g++ main.cpp -o main -lm
Here, `-lm` tells the compiler to link against the math library. Proper linking ensures that any required external functions can work seamlessly with your code.
Using C++ Standard Libraries
Utilizing C++ standard libraries can significantly enhance the functionality of your programs. Incorporating the `<vector>` library can improve data management:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
To compile this program, you would simply use:
g++ main.cpp -o main
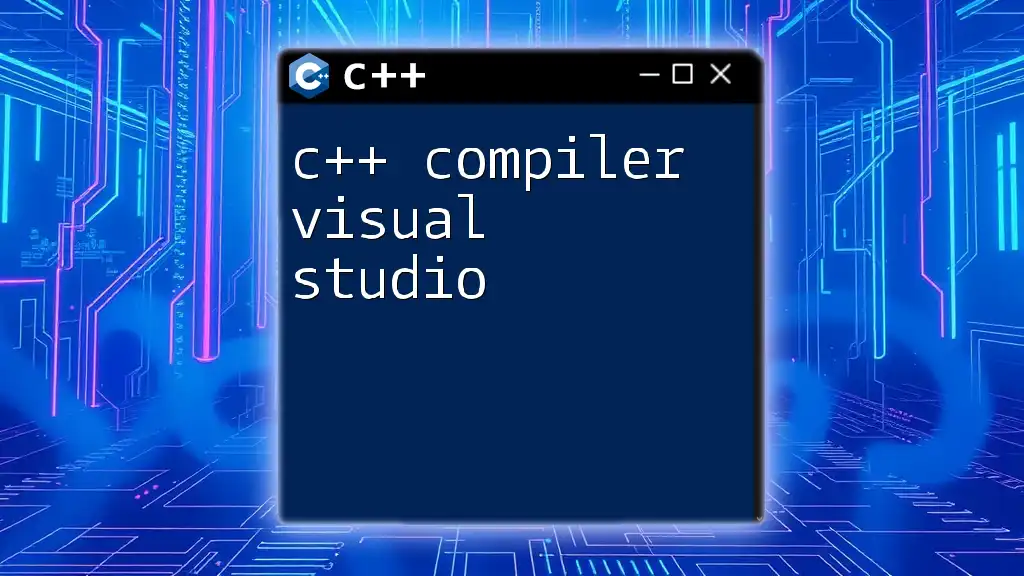
Common g++ Compiler Errors and Solutions
Compilation Errors
As with any programming languages, you may encounter compilation errors. Common errors include:
- Syntax errors: Check for missing semicolons or unmatched braces.
- Type mismatches: Ensure that variables are properly declared before use.
A common error message might look like:
error: 'cout' was not declared in this scope
This usually signifies that the appropriate header files, like `#include<iostream>`, are missing.
Linker Errors
Linker errors often occur when you reference variables or functions without defining them. For example, if you forget to link against a library, you may see messages such as:
undefined reference to 'sqrt'
The solution here is to include the appropriate libraries in your compilation command.
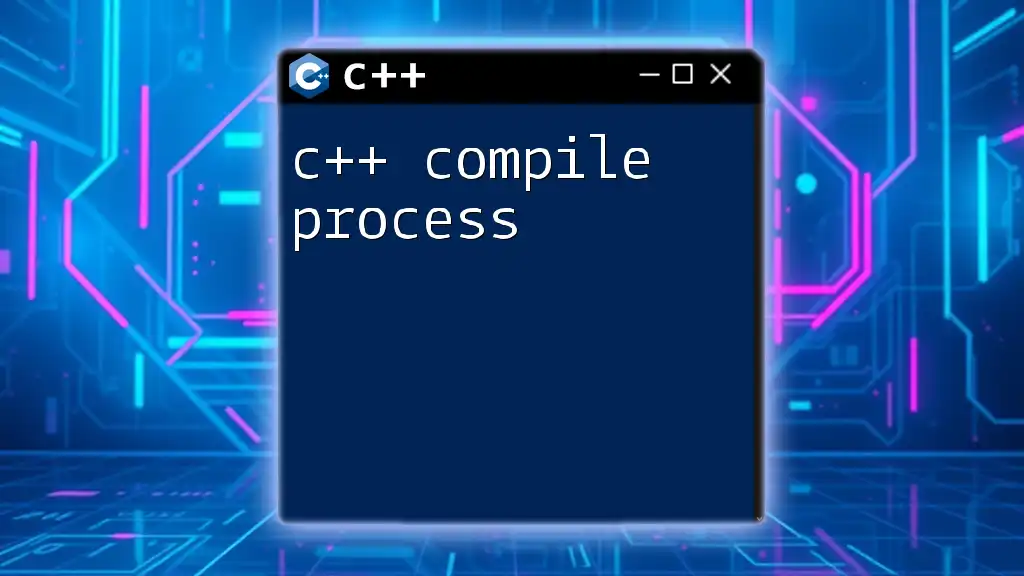
Best Practices for g++ Usage
Organizing Project Files
Organizing your C++ project files is fundamental for maintainability and collaboration. Here’s a recommended folder structure:
/ProjectRoot
│
├── src # Source files
├── include # Header files
├── lib # External libraries
├── bin # Compiled binary executables
└── README.md # Project documentation
Version Control Integration
Integrating git with your g++ projects allows for effective teamwork and version tracking. To initialize a new git repository in your project folder, use:
git init
To add your files and commit, you would run:
git add .
git commit -m "Initial commit"
Using version control helps track changes and collaborate more effectively.
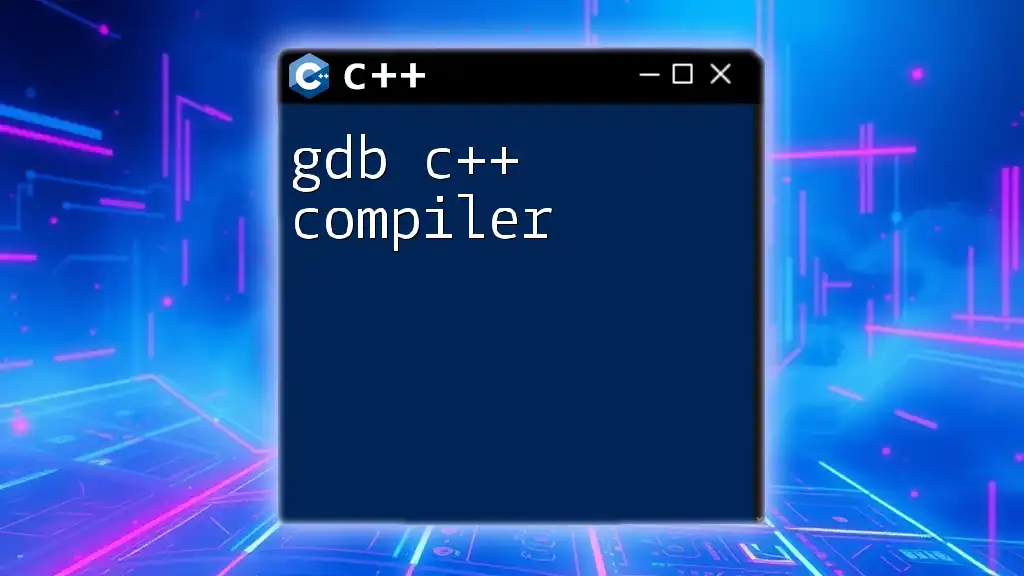
Conclusion
The g++ compiler is a powerful tool for anyone looking to develop C++ applications. Its open-source nature, compatibility across platforms, and robust functionality make it a favorite among developers worldwide. By mastering g++, you'll unlock your potential to create efficient, portable, and maintainable C++ code.
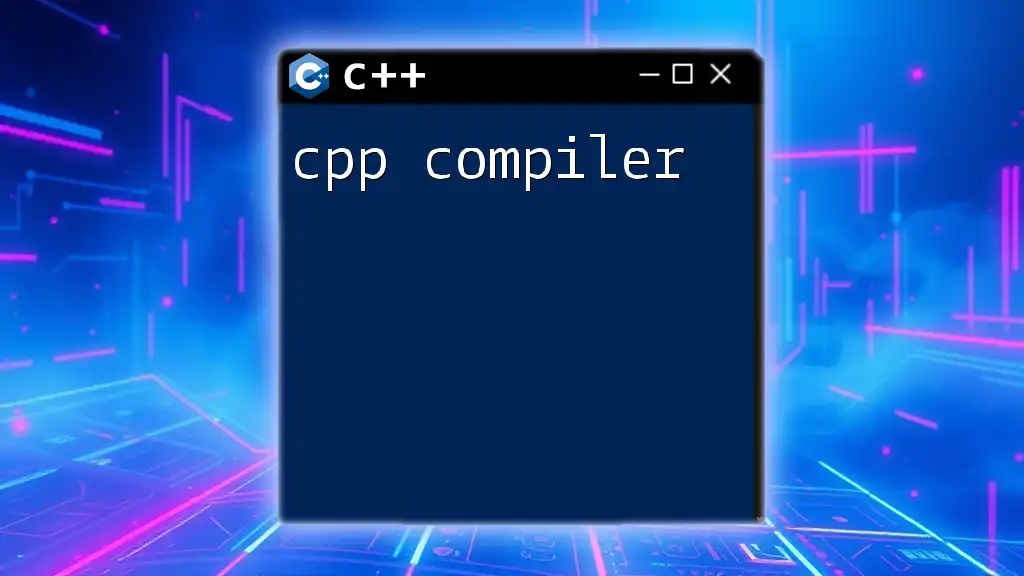
Additional Resources
For further exploration, dive into online communities like Stack Overflow and GitHub, where you can gain insights from fellow developers. Recommended readings include widely acclaimed tutorials and books on C++ and g++. Happy coding!