A free C++ compiler for Windows, such as MinGW or Microsoft Visual Studio Community, allows you to write, compile, and execute C++ code efficiently on your system.
Here's a simple "Hello, World!" program example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Compiler?
A C++ compiler is a specialized tool that converts C++ code into machine-readable instructions. At its core, a compiler interprets human-written code and translates it into binary code, allowing the CPU to execute it. Compilers vary in functionality and can include additional features such as debugging tools, optimizations, and code analysis.
While many programmers often conflate interpreters with compilers, they serve different functions. An interpreter executes code line-by-line in real-time, while a compiler translates the entire code ahead of execution, allowing it to run faster. Recognizing these distinctions is vital, especially when selecting a free C++ compiler for Windows.
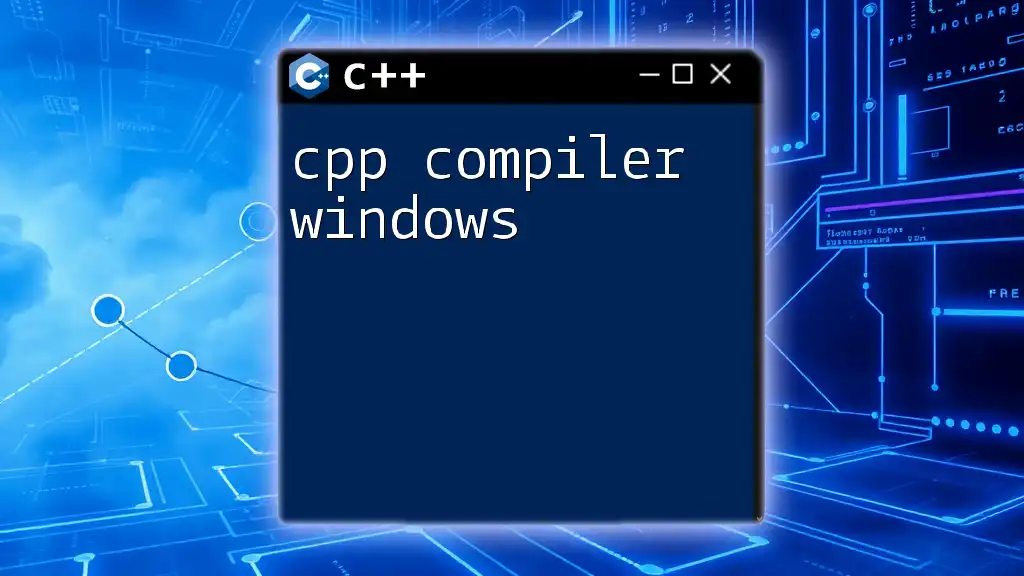
Why Use a Free C++ Compiler?
The benefits of free C++ compilers are extensive. Below are some reasons you should consider using one:
Cost-effectiveness: Many robust compilers are available for free, ensuring that beginners and those on tight budgets can still learn and develop without financial constraints.
Accessibility: Free compilers open the door for anyone interested in learning C++. This democratization of knowledge empowers more individuals to explore software development and programming.
Community support: Free compilers often have large user communities. This means you can find tutorials, forums, and documentation to help you troubleshoot issues and enhance your learning experience.
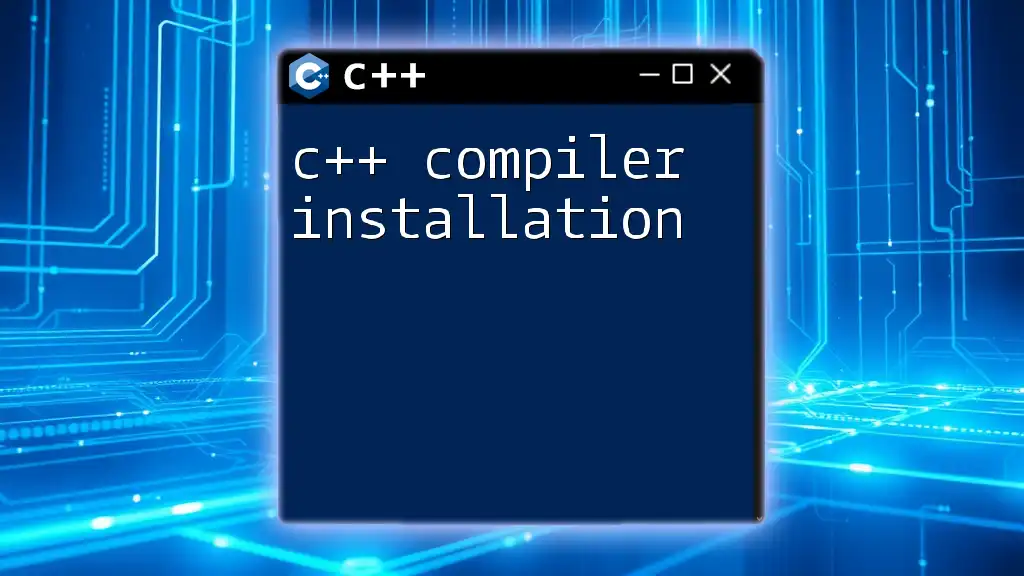
Popular Free C++ Compilers for Windows
GCC (GNU Compiler Collection)
Overview: GCC is a powerful open-source compiler offering broad compatibility with various programming languages, including C++. GCC is versatile and widely used in professional environments.
Installation Guide:
- To install GCC on Windows, you can use MinGW or MSYS2.
- For MinGW, download the installer, select the C++ compiler option during setup, and add the binary directory to your system's PATH.
Code Example: Here’s how to compile and run a simple C++ program with GCC:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this program, save it as `hello.cpp`, then run the following command:
g++ -o hello hello.cpp
./hello
Clang
Overview: Clang is a modern compiler designed to offer a user-friendly experience and excellent diagnostics. It’s known for its speed and is often more informative in error reporting compared to other compilers.
Installation Guide:
- Download the Clang installer from the official website and run it. For users integrating with Visual Studio, Clang can be included as a toolchain option.
Code Example: Below is a simple program to demonstrate Clang's usage:
#include <iostream>
int main() {
std::cout << "Hello, Clang World!" << std::endl;
return 0;
}
To compile and run the above code, use:
clang++ -o hello hello.cpp
./hello
Microsoft Visual C++ Community Edition
Overview: Part of Microsoft Visual Studio, this edition is comprehensive and provides an excellent development environment integrated with C++ tools. It's particularly suitable for Windows developers.
Installation Guide:
- Download the Visual Studio installer from Microsoft's website. During installation, select the "Desktop development with C++" workload.
Code Example: Once set up, you can create a new C++ project and use the IDE to write and execute code. Here is a simple program:
#include <iostream>
int main() {
std::cout << "Hello, Visual Studio World!" << std::endl;
return 0;
}
Use the built-in tools to build and run this application effortlessly.
Code::Blocks
Overview: Code::Blocks is an open-source IDE that can integrate various compilers, including GCC, MinGW, and others. Its flexibility and user-friendly interface make it an excellent choice for beginners.
Installation Guide:
- Download the setup file from the official Code::Blocks website and ensure that you choose a version that comes bundled with MinGW for a complete installation.
Code Example: Once installed, create a new project, and you can write and run your C++ program within the IDE. For instance:
#include <iostream>
int main() {
std::cout << "Hello, Code::Blocks World!" << std::endl;
return 0;
}
Simply hit the run button to execute the code.
Dev-C++
Overview: Dev-C++ is a lightweight IDE with a built-in MinGW compiler. While it's less frequently updated than some other options, its simplicity is appealing for newcomers.
Installation Guide:
- Download it from the official website, run the installer, and begin programming with this straightforward interface.
Code Example: Here’s a simple demonstration of writing C++ code in Dev-C++:
#include <iostream>
int main() {
std::cout << "Hello, Dev-C++ World!" << std::endl;
return 0;
}
Compile and run directly by clicking the appropriate buttons in the IDE.
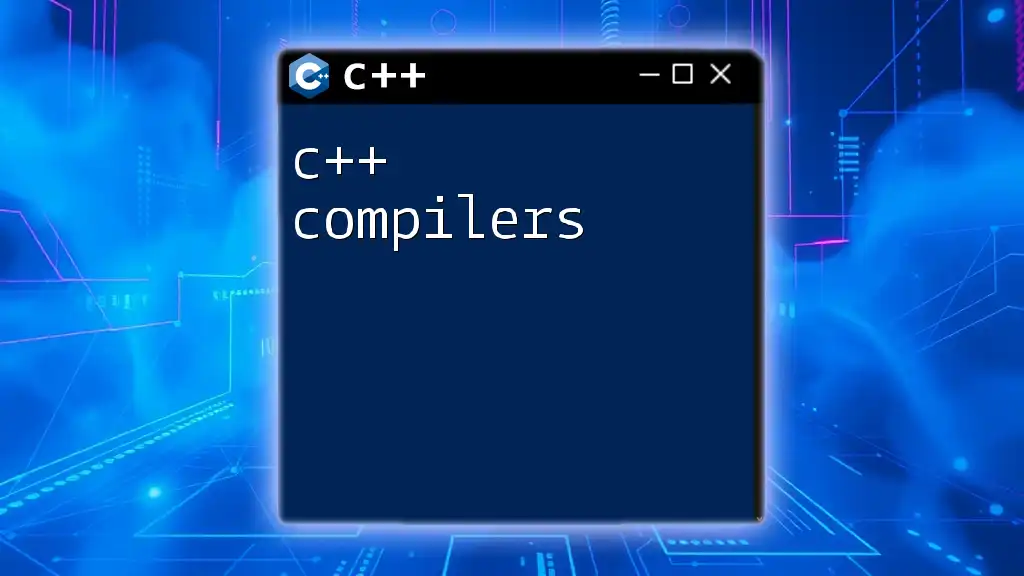
Comparison of Free C++ Compilers
Ease of Use
Each compiler has its unique features. For example, Microsoft Visual C++ provides a complete development environment, while GCC and Clang operate well from the command line, appealing to those who prefer that interface. Meanwhile, IDEs like Code::Blocks and Dev-C++ are designed for ease of access, making them favorites among beginners.
Performance
In general, GCC is known for producing highly optimized code, while Clang excels in faster compilation times and informed diagnostics. Keep in mind that performance may vary depending on the complexity and specifics of the project you're working on.
Community Support and Documentation
Every compiler mentioned has a strong community backing, with forums, documentation, and tutorials readily available. This accessibility makes troubleshooting much simpler, providing resources for fixing common errors and enhancing learning.
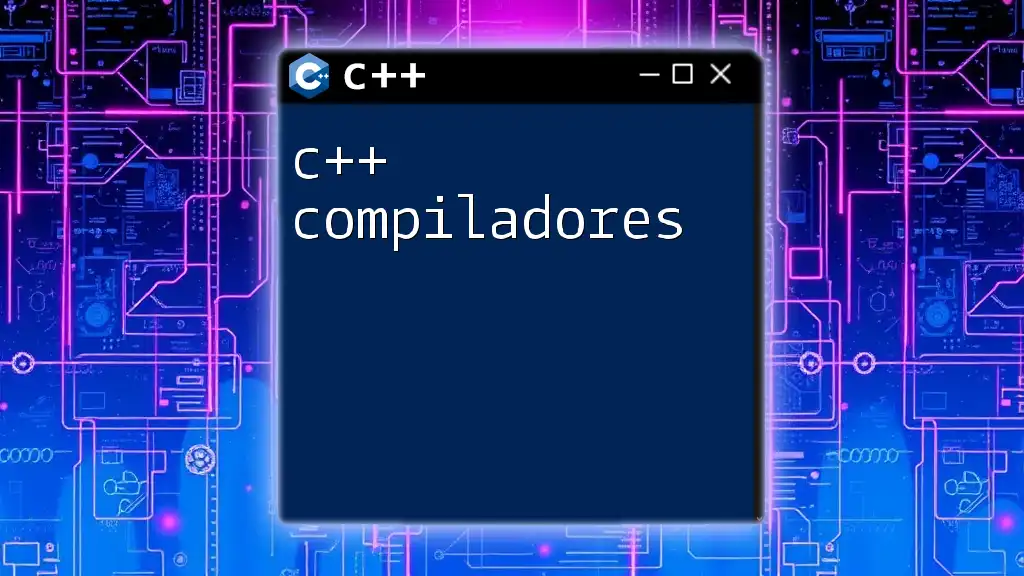
How to Choose the Right C++ Compiler for You
When selecting a free C++ compiler for Windows, consider your project’s requirements, your current skill level, and your preferred programming style.
- If you're a beginner, IDEs like Code::Blocks or Microsoft Visual C++ might be more suitable.
- Intermediate users may appreciate the flexibility of GCC or Clang.
- Advanced programmers might prefer command-line interfaces for more control and efficiency.
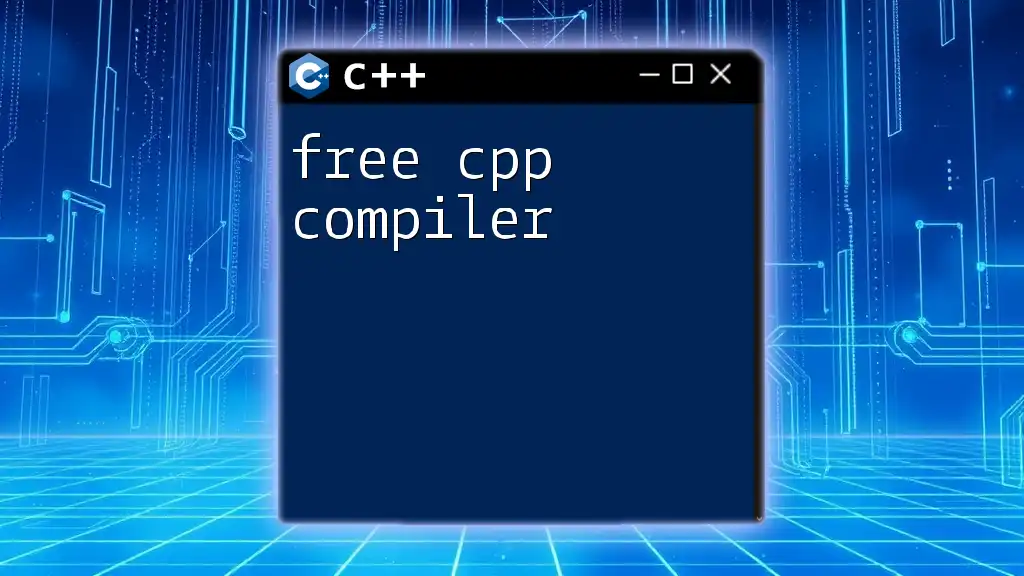
Conclusion
Having a good C++ compiler is indispensable for anyone serious about programming in C++. With numerous options available, from GCC and Clang to Microsoft Visual C++ and Dev-C++, you can find a compiler that fits your style and needs. We encourage you to explore different compilers and engage with the community. Practice your coding skills to build a solid foundation in C++ programming.
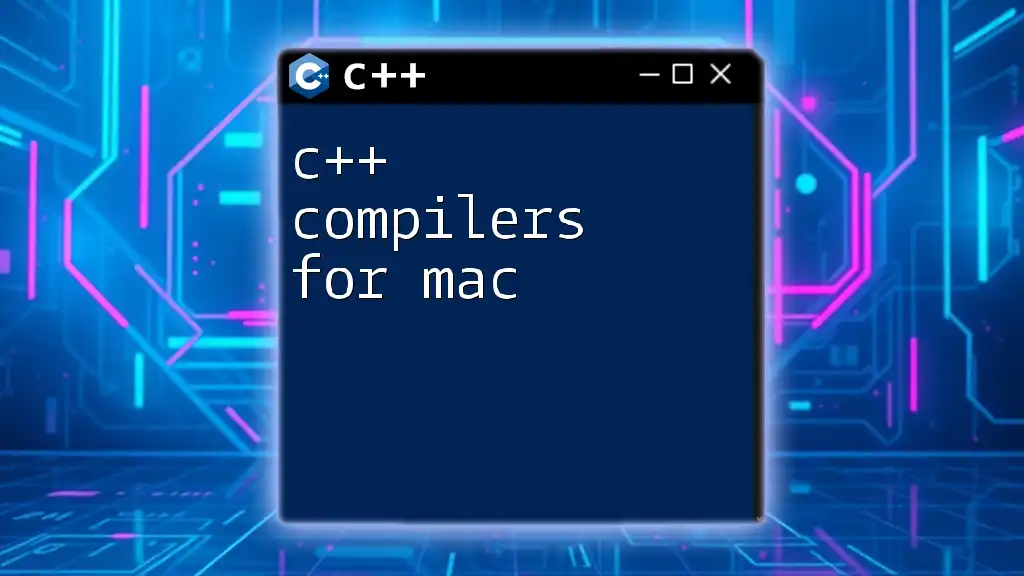
Additional Resources
Links to official websites:
- [GCC](https://gcc.gnu.org/)
- [Clang](https://clang.llvm.org/)
- [Microsoft Visual Studio](https://visualstudio.microsoft.com/)
- [Code::Blocks](http://www.codeblocks.org/)
- [Dev-C++](https://sourceforge.net/projects/orwelldevcpp/)
Recommended books and courses: Search online for beginner to advanced C++ programming books and consider enrolling in online courses for structured learning.
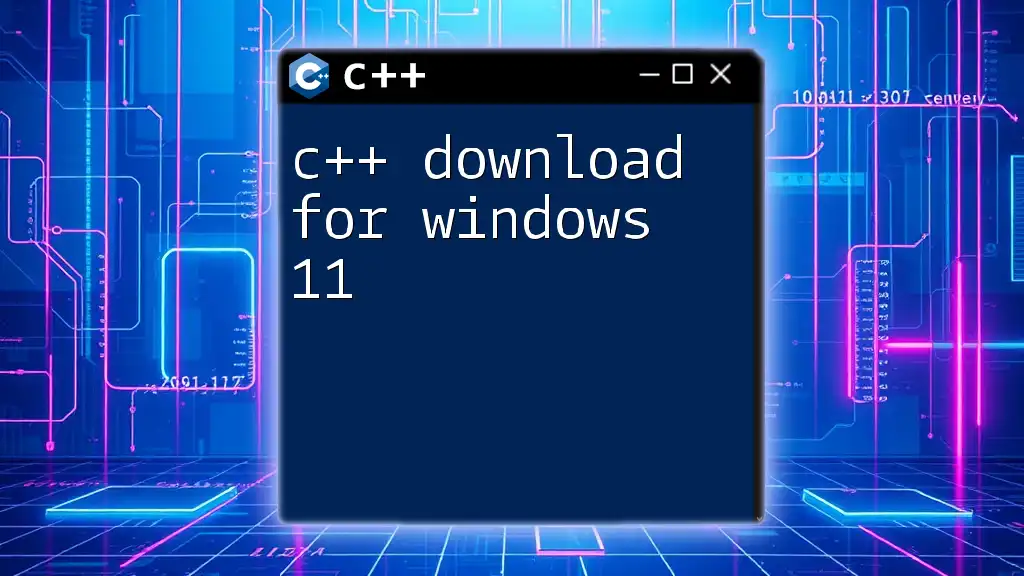
FAQ Section
What is the best free C++ compiler for Windows?
Your choice depends on your needs; however, both GCC and Microsoft Visual C++ Community Edition are widely regarded as excellent options.
Can I use multiple compilers on the same machine?
Absolutely! You can install multiple compilers on your Windows system and use them according to your preferences.
What are the common errors during installation, and how to fix them?
Common issues may include path configuration errors or missing dependencies. Ensure you refer to the official documentation and community forums for specific troubleshooting steps.