A C++ decompiler online is a tool that translates compiled C++ binaries back into human-readable source code, allowing developers to analyze and understand the original program.
Here's a simple example of how C++ code looks before and after compilation:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This C++ code snippet would get compiled into a binary, which can then be processed by a C++ decompiler to recover the source code.
What is C++ Decompilation?
Understanding Decompilation
Decompilation is the process of converting compiled code (binary form) back into a human-readable source code format. In simple terms, it allows developers to analyze and study how a program works by reversing the compilation process. This is especially useful for debugging, recovering lost source code, or understanding third-party libraries when source code is not available.
The purpose of decompilation is multifaceted, with developers often seeking to:
- Recover lost or misplaced source code.
- Analyze how external libraries work for better integration.
- Debug applications when source code is no longer accessible.
It's important to note that decompilation is significantly different from compilation. While compilation transforms high-level source code into machine code that a computer can execute, decompilation attempts to reconstruct that source code from the machine code. This process can be complex, and reconstructed code may not exactingly match the original due to optimizations made by the compiler.
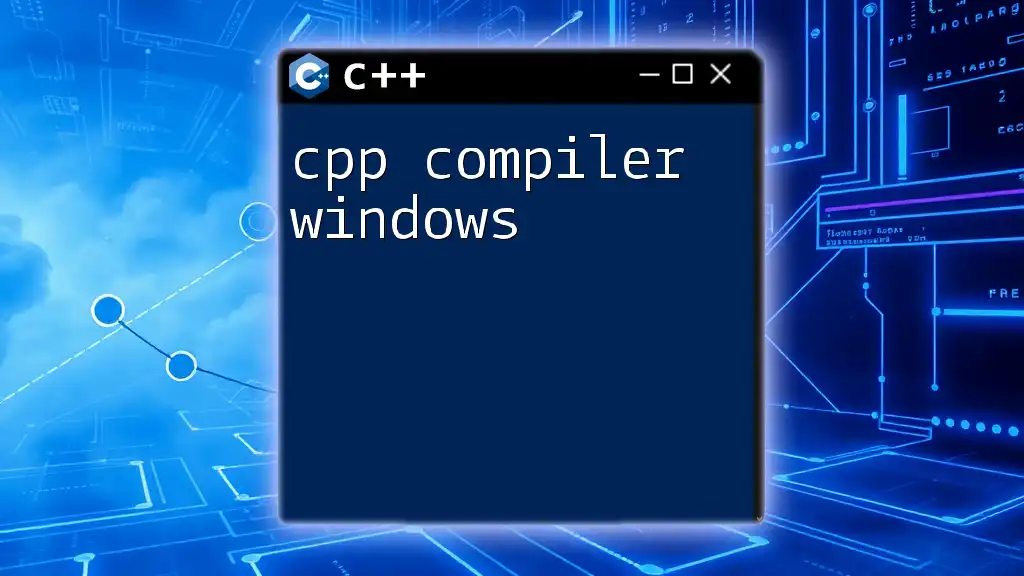
Types of C++ Decompilers
Online vs Offline Decompilers
When it comes to decompilation, developers have the choice between online and offline tools.
- Online Decompilers: These are web-based tools that allow users to upload their C++ files directly through a browser. They are convenient for quick tasks without needing software installation. Examples include Decompiler.com and Ghidra Online.
- Offline Decompilers: These are software programs installed on a local machine, providing more privacy and greater control over the decompilation process. They're often preferred for larger projects or sensitive codebases.
Advantages of Online Decompilers:
- No installation required, making them accessible from any device.
- Often have intuitive user interfaces that are easy to navigate.
Disadvantages:
- Potential security risks when uploading sensitive code.
- Limited functionality compared to their offline counterparts.
Popular C++ Decompilers
Several decompilers stand out within the realm of C++ development:
-
Ghidra: An open-source tool developed by the NSA that allows for advanced reverse engineering. Ghidra supports a variety of architectures and provides an extensive suite of analysis tools, making it an excellent choice for in-depth examination of binary files.
-
Decompiler.com: This web-based decompiler is user-friendly and allows for straightforward uploading and analysis of C++ source code. It’s great for quick lookups and offers a hassle-free experience.
-
RetDec: A versatile open-source decompiler that can handle multiple programming languages, including C++. It provides both a web interface and command line version, catering to various user preferences.
-
Other Notable Mentions: There are other options like Hex-Rays and IDA Pro, which are robust but often come at a steep cost.
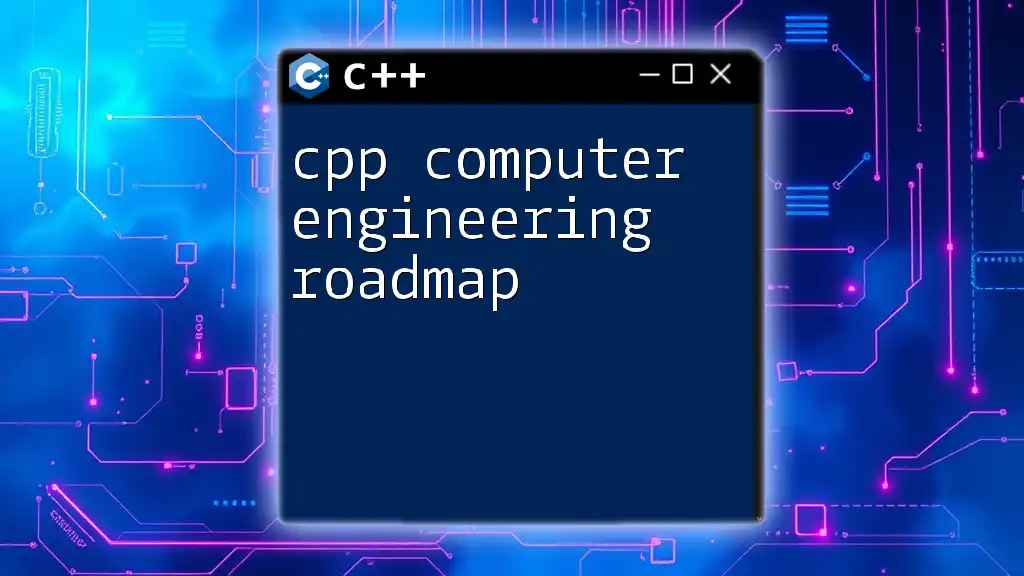
How to Use Online C++ Decompilers
Step-by-Step Guide
Accessing an Online Decompiler: Start by finding a reliable online C++ decompiler. Ensure that it has positive reviews and offers clear functionality.
Uploading Your C++ File: Most online decompilers accept various file formats (.cpp, .obj, .exe), but always check the specific requirements. After uploading your file, the decompiler will begin its analysis.
Analyzing Decompiled Output: Once the analysis is complete, the tool will present the results, transforming the binary code back into C++ source code. Take your time to understand this output — it may not be fully optimized and can contain unexpected results.
Example of Decompiling a Simple C++ Program
To illustrate the decompilation process, let's look at a simple C++ program:
// Original C++ Code
#include<iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
When decompiled, this code might produce something akin to:
#include <iostream>
using namespace std;
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
While the decompiled output largely resembles the original source code, minor discrepancies may arise, showing how some code constructs may be less descriptive or overly simplified.
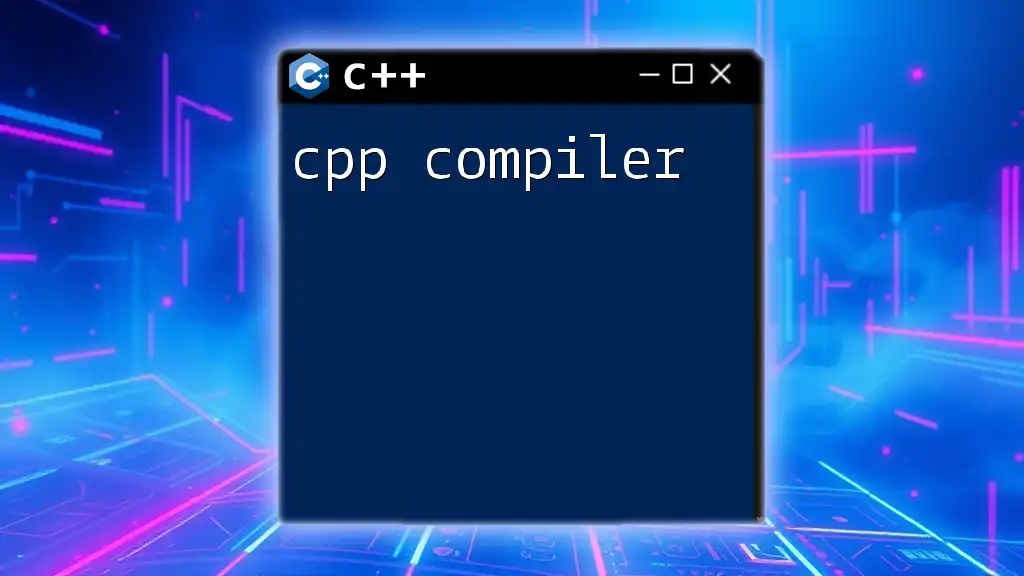
Limitations of Online C++ Decompilation
Legal and Ethical Considerations
Before diving into decompilation, it's crucial to acknowledge copyright issues. Decompiling software that you do not own or have explicit permission to analyze can lead to legal repercussions. It's generally acceptable to decompile only your own code or software where you’ve secured permissions or licenses.
Technical Limitations
Online C++ decompilers do have some technical limitations:
- Incomplete Output: Decompiled code may not retain the original logic or structure due to optimizations and transformations applied during compilation. As a result, the analysis might not provide the clarity developers hope for.
- Performance Concerns: The complexity and size of the code can significantly impact the quality and accuracy of decompilation, with larger binaries often yielding less reliable results.
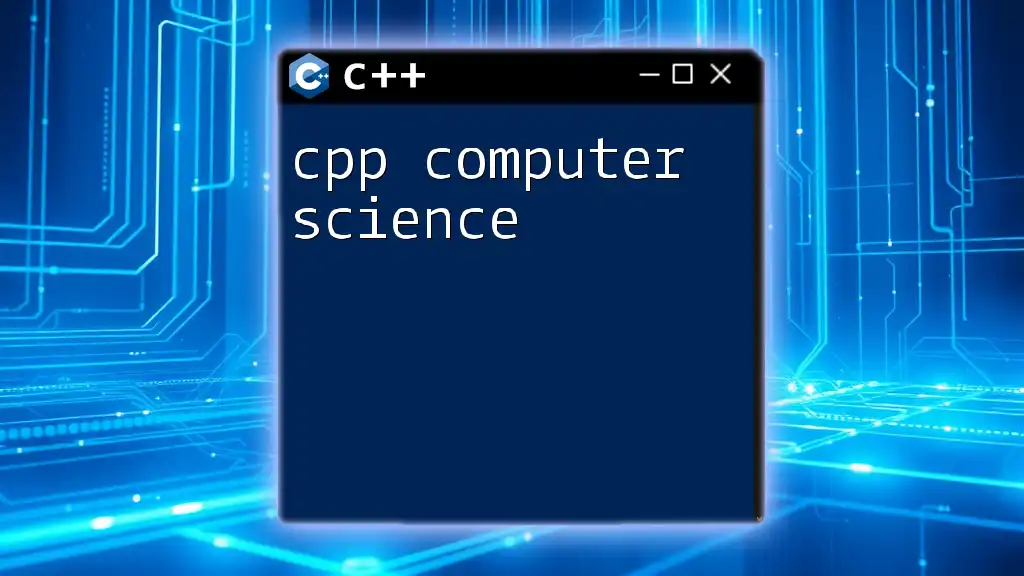
Best Practices for Using Online Decompilers
Ensuring Code Safety
When using online decompilers, ensuring the safety of your code is paramount. Always be mindful of the sensitivity of the data you are uploading. Avoid uploading confidential information or proprietary code.
Choosing Reputable Sites: Carefully choose decompilation services with positive feedback and robust security measures in place for data handling.
Maximizing Results
To achieve the best decompilation results:
- Maintain a clear and well-structured codebase. Simple, logically coherent code tends to yield better decompilation outcomes.
- Prioritize commenting and documentation in your original C++ code. Comments and documentation can improve understandability when the code is decompiled.
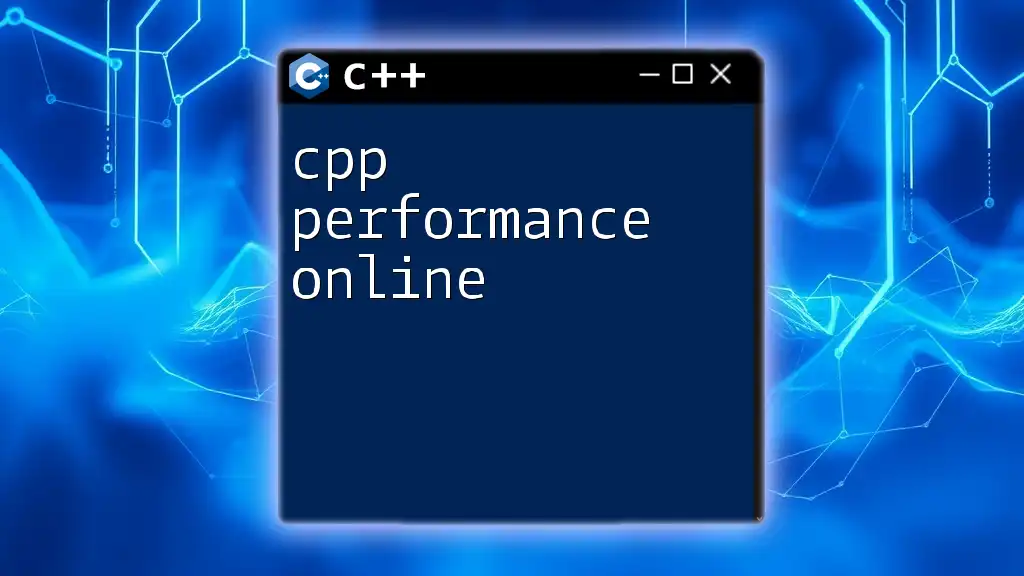
Conclusion
C++ decompilers serve as powerful tools for developers seeking to reverse engineer or analyze code. Whether you're recovering lost source files or integrating third-party libraries, understanding how to utilize online C++ decompilers effectively is essential. However, it is important to remain ethical and responsible in your usage.
As technology evolves, so does the realm of decompilation, making it an exciting field to watch for future developments and advancements.
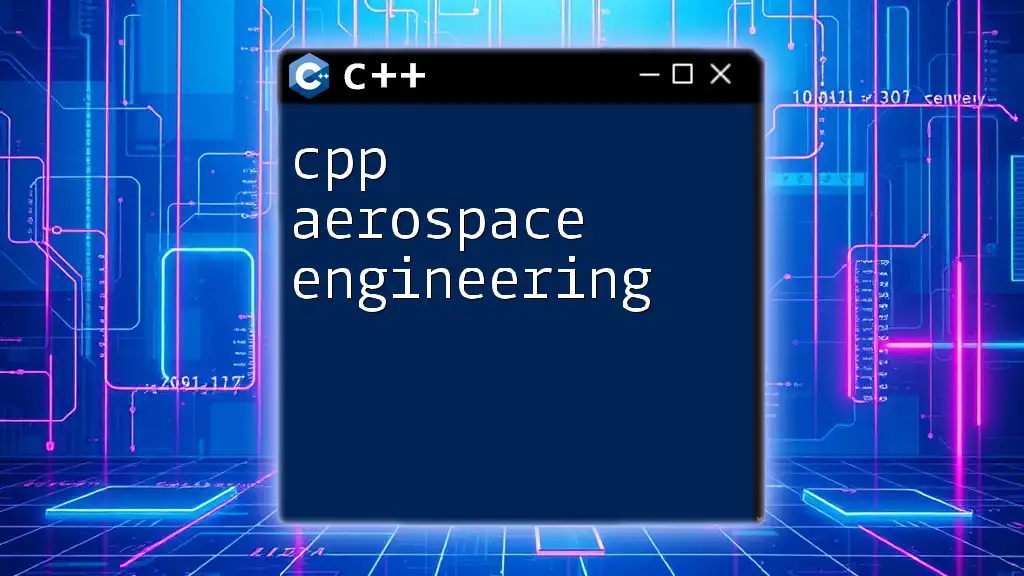
Additional Resources
For those looking to further their understanding of C++ and decompilers, consider exploring established C++ documentation, online forums, and communities where enthusiasts and professionals share insights and experiences.
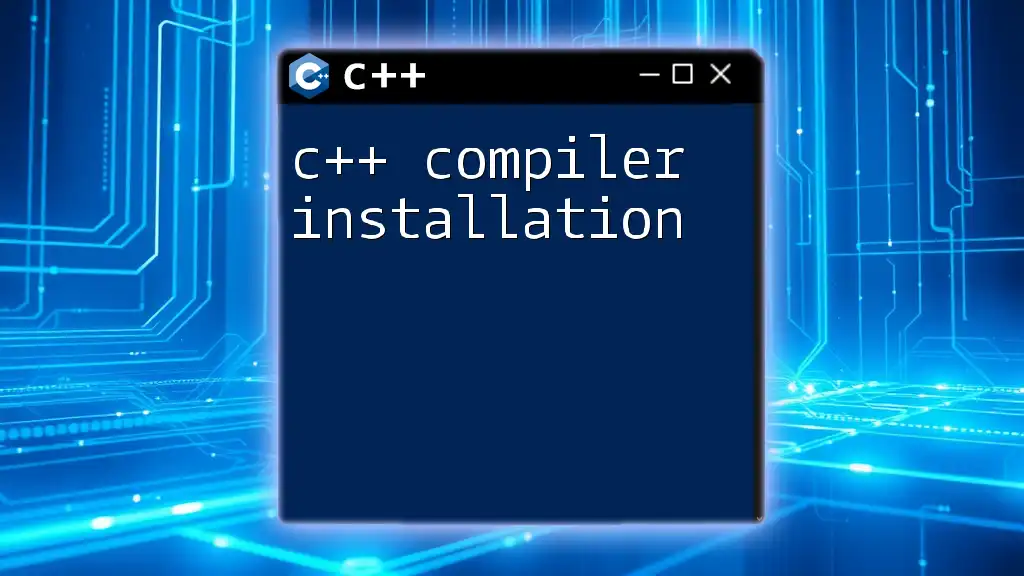
Call to Action
Have you used a C++ decompiler online? Share your experiences, tips, or questions in the comments section below! Your insights could help others in the community navigate the complexities of C++ decompilation.