Running C++ code online allows you to execute and test your C++ programs directly from a web browser without the need for a local development environment. Here's a simple example to demonstrate how you can print "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Benefits of Running C++ Code Online
Convenience of Online Editors
One of the most significant advantages of online C++ editors is accessibility. Unlike traditional local environments that require setup, online editors can be accessed from any device—be it a laptop, tablet, or smartphone. This flexibility means you can code and compile from anywhere, whether at home, at college, or even in a café.
Moreover, not having to install a local development environment can save time, especially for beginners. Setting up tools like compilers and IDEs can sometimes be complex; thus, using online platforms streamlines this process, allowing you to focus solely on coding.
Instant Feedback
When you run C++ code online, you receive immediate feedback. This instant response facilitates a practical coding environment, enabling you to test ideas quickly, debug on the fly, and make adjustments in real-time. For example, if a mistake occurs, you can see the specific error and its location, helping you learn and correct your coding errors effectively.
Collaborative Learning
Online C++ editors lend themselves well to collaborative learning. Sharing code snippets becomes a breeze, allowing friends or classmates to work together on projects or review each other’s code. This communal approach not only enhances learning opportunities but also builds problem-solving skills as users benefit from diverse coding styles and methodologies.
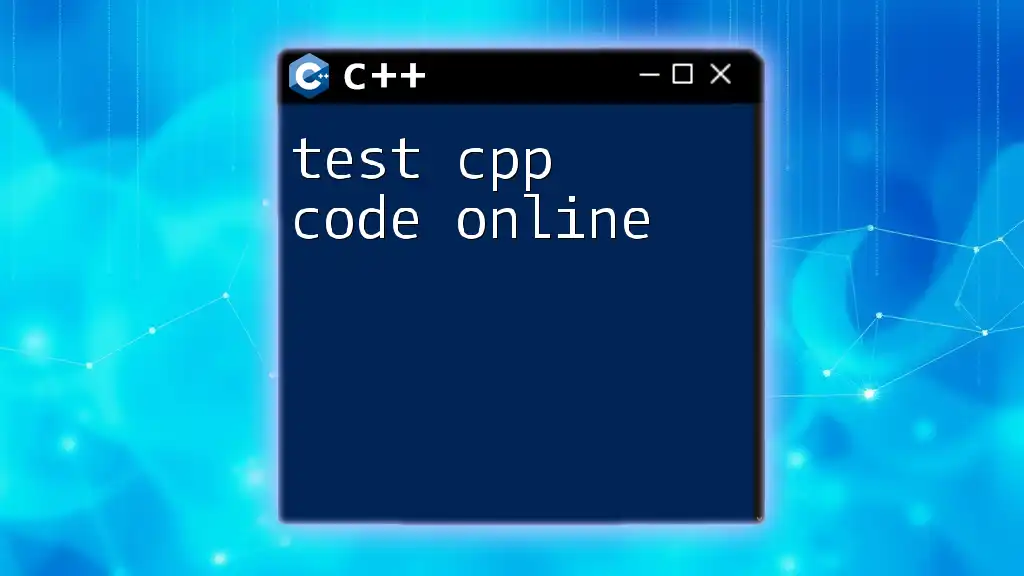
Popular Online C++ Editors
Overview of Top Platforms
Several online C++ editors stand out due to their user-friendly interfaces, powerful features, and robust functionality. Some of the most popular platforms include:
- Repl.it
- Ideone
- W3Schools
- CoderPad
- CPP.sh
Detailed Review of Each Editor
Repl.it
Repl.it provides an interactive and intuitive platform for coding in C++. Its clean interface makes it easy to write, run, and debug code. With built-in collaboration features, multiple users can work on the same codebase in real time.
To run code on Repl.it, simply create a new C++ project, enter your code, and click the "Run" button. Here's an example of a simple C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code prints "Hello, World!" to the console, demonstrating how quick and easy it is to launch a simple program.
Ideone
Ideone is another excellent online compiler that supports various programming languages, including C++. Its straightforward interface allows users to input code, compile it, and view output quickly.
With Ideone, you can run slightly more complex C++ functions. For example, if you want to implement a simple addition function, your code may look like this:
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl;
return 0;
}
Just paste this code into Ideone, and you can see the result instantly.
W3Schools
W3Schools caters not only to programmers but also to complete beginners. Its C++ online editor allows novice users to grasp C++ syntax and concepts effortlessly. One of the unique features of W3Schools is the built-in tutorials, which guide you as you write code.
Here’s an example of a simple for loop:
#include <iostream>
using namespace std;
int main() {
for(int i = 1; i <= 5; i++) {
cout << "Number: " << i << endl;
}
return 0;
}
W3Schools is particularly beneficial for users unfamiliar with C++.
CoderPad
CoderPad is primarily used in tech interviews, allowing users to demonstrate their coding skills. It provides a clean interface and immediate feedback, making it ideal for executing sample code and analyzing output during coding assessments.
For example, you could run a basic sorting algorithm on CoderPad to showcase your skill in handling data structures.
CPP.sh
CPP.sh offers a straightforward online C++ environment that focuses on simplicity. It’s great for quick tests without any unnecessary features. Users appreciate its clean interface for running simple scripts.
Using CPP.sh, you could try out a simple conditional statement:
#include <iostream>
using namespace std;
int main() {
int a = 10;
if (a > 5) {
cout << "a is greater than 5" << endl;
} else {
cout << "a is not greater than 5" << endl;
}
return 0;
}
This snippet will produce immediate output based on the boolean condition.
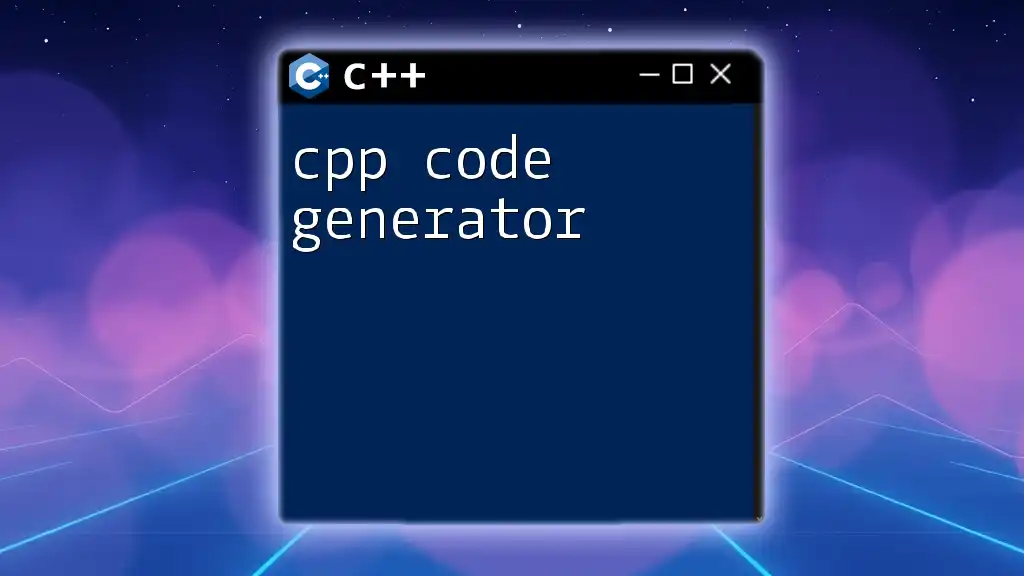
How to Use an Online C++ Editor
Step-by-Step Guide
Using an online C++ editor typically follows a straightforward process. Here’s how you can write, compile, and execute C++ code online:
-
Choose Your Editor: Select one of the popular online C++ editors mentioned earlier.
-
Write Your Code: Begin by entering your C++ code in the provided code editor.
-
Compile the Code: Most editors have a "Run" or "Compile" button that you can easily click to compile your code.
-
View the Output: The console output displays the results of your execution, providing you with immediate feedback on your code.
Common Features to Explore
Syntax Highlighting
Syntax highlighting is a crucial feature in online C++ editors. It enhances code readability by visually differentiating keywords, variables, and strings. This makes identifying errors or necessary edits much easier.
Error Highlighting
Many online editors provide error highlights in real-time. This feature is essential for diagnosing issues quickly, allowing you to see where the problem lies and how to fix it without sifting through code for long periods.
File Management
While coding online, consider how you can save, load, and share your projects. Some platforms enable file management features that facilitate easy access to your past projects, helping you revisit and refine your code when necessary.
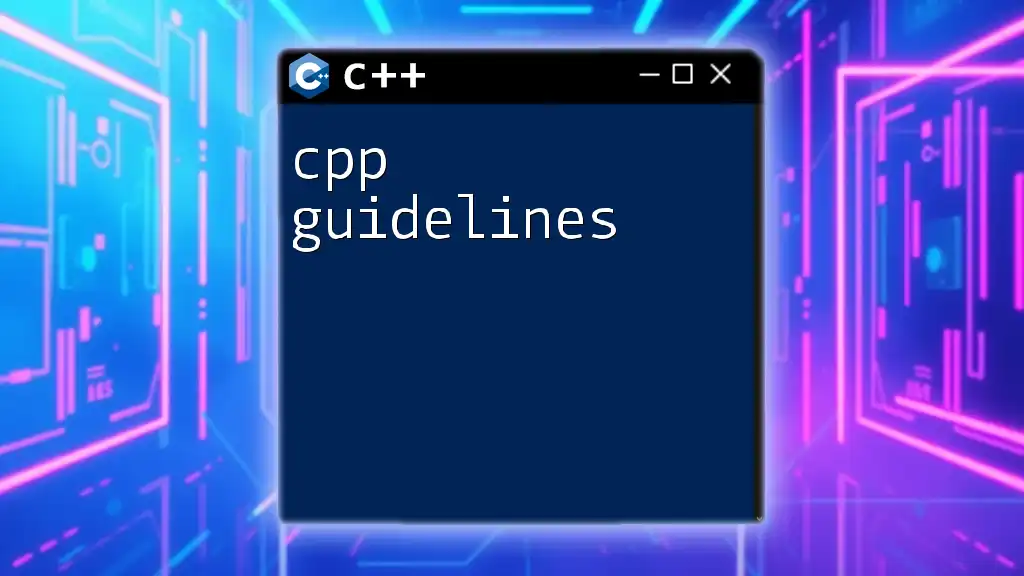
Tips for Effective Use of Online C++ Editors
Optimize for Learning
To maximize learning, embrace best practices for coding. Write clear and purposeful code; include comments where needed to explain your thought process. This not only helps you but also illustrates your coding approach if you share your work with others.
Experiment with Different Features
Don’t hesitate to explore different tools and settings within the editors. Experimenting can lead to discovering new functionalities and coding techniques that improve your coding skills and efficiency.
Collaborate and Share
Engaging in collaboration can significantly enhance your learning experience. Whether you're working on a group project or simply exchanging ideas with peers, the ability to share code snippets and receive feedback can lead to deeper insights and improved coding practices.
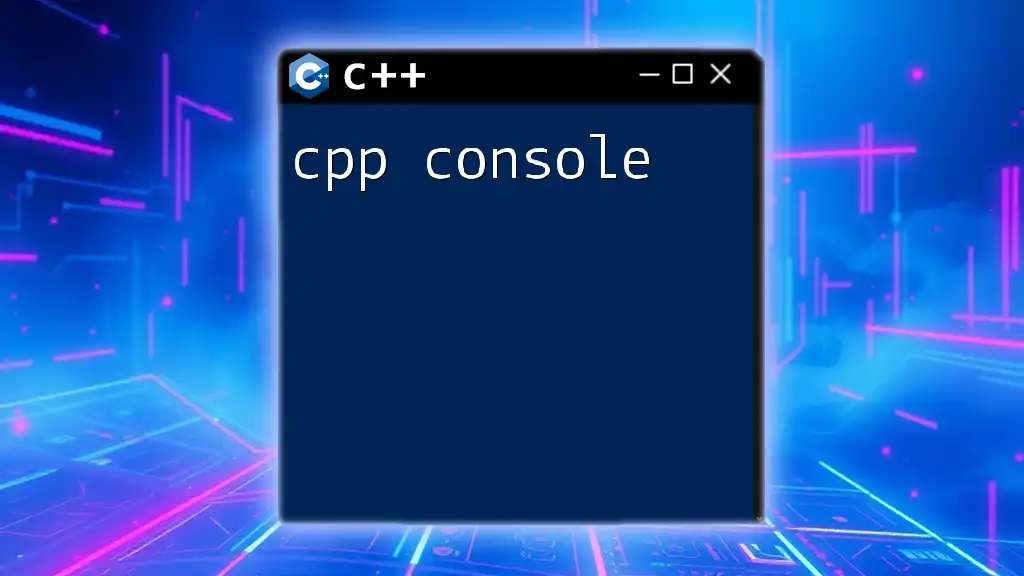
Limitations of Running C++ Code Online
Performance Constraints
While running C++ code online is convenient, it often comes with performance constraints. Online compilers may limit memory usage and computational resources, meaning very large or complex programs might not execute as seamlessly as they would in a local environment.
Internet Dependency
Using online editors requires a stable internet connection. If your connection drops, you might lose unsaved changes in your code or hinder your ability to run programs entirely. Hence, it’s essential to save your progress frequently or work in an offline editor when possible.
Privacy and Security Concerns
When running code on online platforms, consider your privacy and security. Avoid sharing sensitive code that might expose personal information or proprietary algorithms. It’s crucial to be mindful of what you’re uploading to these platforms.
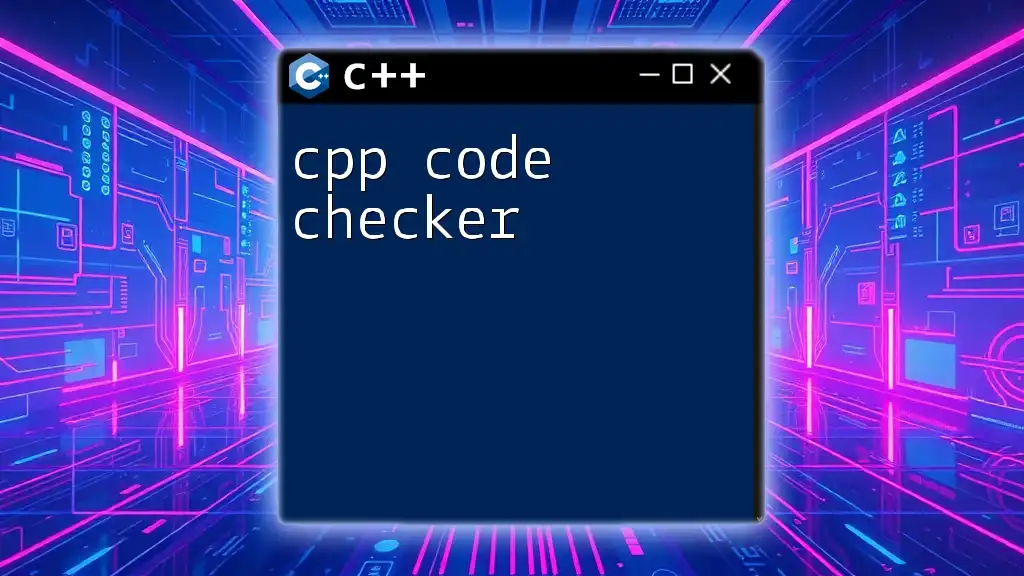
Conclusion
In summary, the ability to run C++ code online opens up a world of convenience and immediate feedback. The variety of online C++ editors available makes it easy for anyone to start coding, regardless of experience level. Whether you're a beginner eager to learn or an experienced coder looking for a quick platform to test your ideas, there’s an online tool suited for your needs.
By exploring different platforms and embracing collaborative learning, you’ll enhance your skills and understanding of C++ programming. Don’t hesitate to dive in, practice, and maximize the potential of online C++ editors. Happy coding!
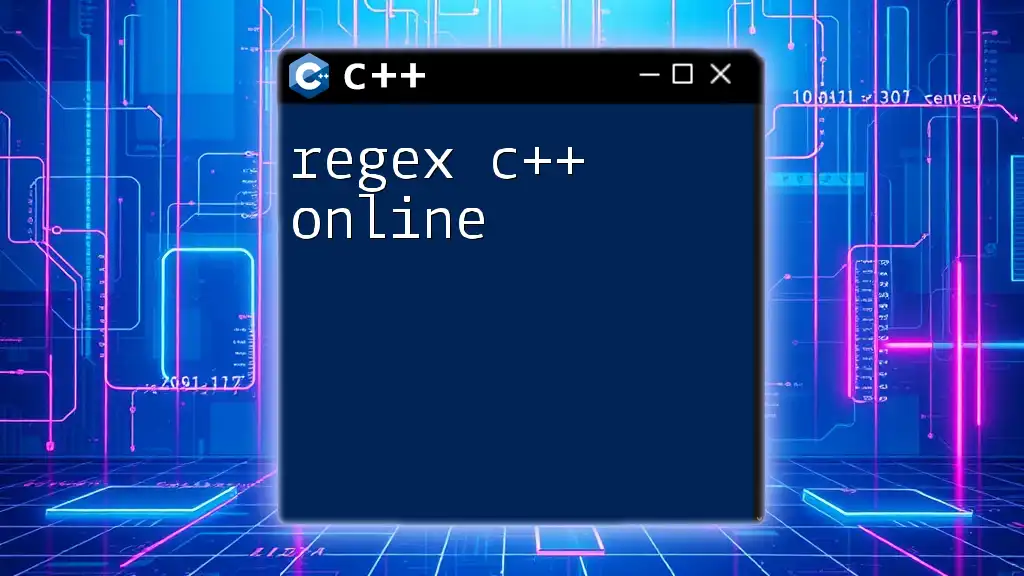
Additional Resources
Explore C++ tutorials, coding courses, and community forums to continue your programming journey. Engaging with resources beyond online editors can significantly bolster your knowledge and skills in C++. Recommended readings and community connections can further fuel your learning process and network.