The RF24 library for Arduino allows users to set the communication channel for NRF24L01 modules, enabling multiple devices to communicate simultaneously without interference by using the `setChannel()` function.
Here's a code snippet demonstrating how to set the RF24 channel to 76:
#include <SPI.h>
#include <RF24.h>
RF24 radio(9, 10); // CE, CSN pins
void setup() {
radio.begin();
radio.setChannel(76); // Set the channel to 76
radio.openWritingPipe(0xF0F0F0F0E1LL);
radio.openReadingPipe(1, 0xF0F0F0F0D2LL);
}
Understanding RF24 and Its Applications
What is RF24?
The RF24 library is a powerful tool designed for wireless communication, particularly in the Internet of Things (IoT) realm. It facilitates the use of Nordic Semiconductor's nRF24L01 series of transceivers, providing a straightforward way to implement low-power, reliable wireless communication between devices. The library supports features like automatic acknowledgment, dynamic payloads, and multi-channel communication, making it an excellent choice for various applications.
Applications of RF24 in Real-World Scenarios
RF24 is widely adopted in devices such as remote controls, wireless sensors, and automation systems. Its ability to provide long-range communication while consuming minimal power makes it particularly advantageous for battery-operated devices. The RF24 library's flexibility allows developers to create applications ranging from home automation systems to sensitive environmental monitoring setups.

Hop Channels Explained
What Are Hop Channels?
Hop channels refer to the technique of changing communication frequencies during data transmission to decrease the likelihood of interference and enhance reliability. This method, known as frequency hopping, allows devices to switch between different channels quickly, making it difficult for potential eavesdroppers to intercept communication and reducing the impact of interference from other devices.
How Hop Channels Work
Frequency hopping works by rapidly changing the transmission frequency according to a predefined sequence. By using multiple channels, devices can maintain a stable communication link even in environments with high electromagnetic interference. This technique not only ensures data integrity but also increases the overall throughput of the communication.

Setting Up RF24 for Hop Channels
Required Hardware
To start using RF24 with hop channels, you need specific hardware, including:
- nRF24L01+ transceivers: These are the wireless communication modules.
- Microcontroller (e.g., Arduino, Raspberry Pi): This will serve as the brain of your project, controlling the RF24 modules.
For efficient performance, select low-power microcontrollers and consider additional components like antennas to enhance range.
Installing the RF24 Library
To utilize RF24, you must install its library on your platform. The installation process varies slightly depending on your environment:
For Arduino:
- Open the Arduino IDE.
- Go to `Sketch` > `Include Library` > `Manage Libraries`.
- Search for "RF24" and click `Install`.
For Raspberry Pi:
- Access the terminal.
- Install the library using the command:
git clone https://github.com/nRF24/RF24.git cd RF24 make sudo make install
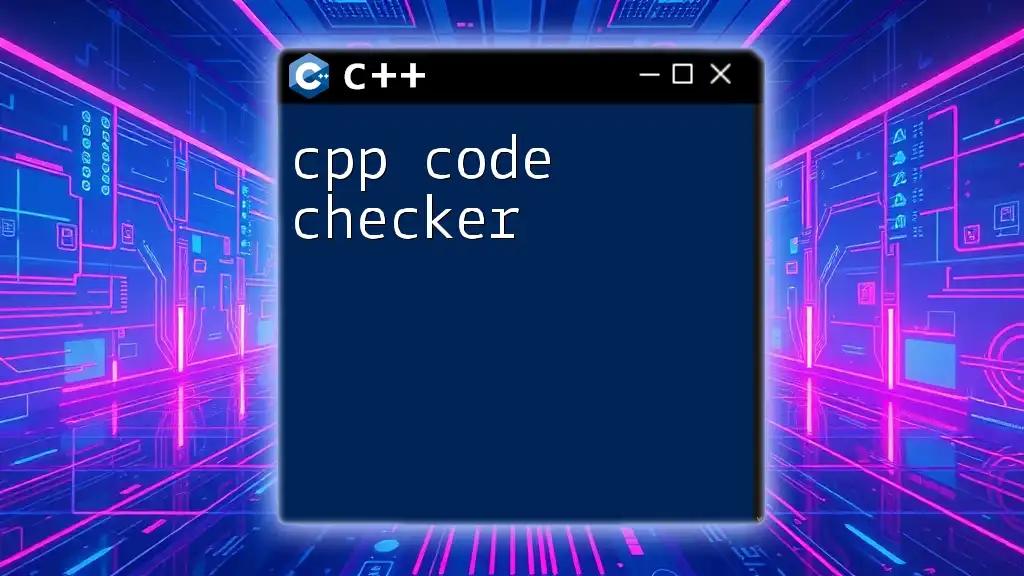
Implementing Hop Channels in Your RF24 Project
Code Overview
To successfully implement hop channels, you must first establish a basic RF24 setup to facilitate communication. This involves importing the necessary libraries and initializing the RF24 object.
#include <RF24.h>
RF24 radio(9, 10); // CE, CSN pins
uint8_t channel = 76; // Default RF channel
The channel variable represents the current communication channel being utilized. You can adjust it during program execution to hop between channels.
Writing Your First RF24 Hop Channel Program
Setting Up the Code
Initialize your RF24 transceiver as demonstrated above. Once the RF24 library is set up, you'll implement logic for frequency hopping.
Implementing Frequency Hopping
To enable frequency hopping, define a function that modifies the channel during communication.
void hopChannel() {
channel = (channel + 1) % 127; // Change channel every time
radio.setChannel(channel); // Set the new channel
}
In this function, the channel increments by one for each iteration in your main loop. The modulo operation `% 127` ensures that the channel stays within the valid range of 0-127.
Example of Frequency Hop in Action
Here's a simple program that demonstrates how to incorporate frequency hopping in a typical RF24 setup:
void setup() {
radio.begin();
radio.setChannel(channel);
// Additional setup code
}
void loop() {
hopChannel();
// Code to send and receive data
}
In this loop, the `hopChannel()` function will get called on every iteration, effectively changing the communication channel and reducing potential interference.
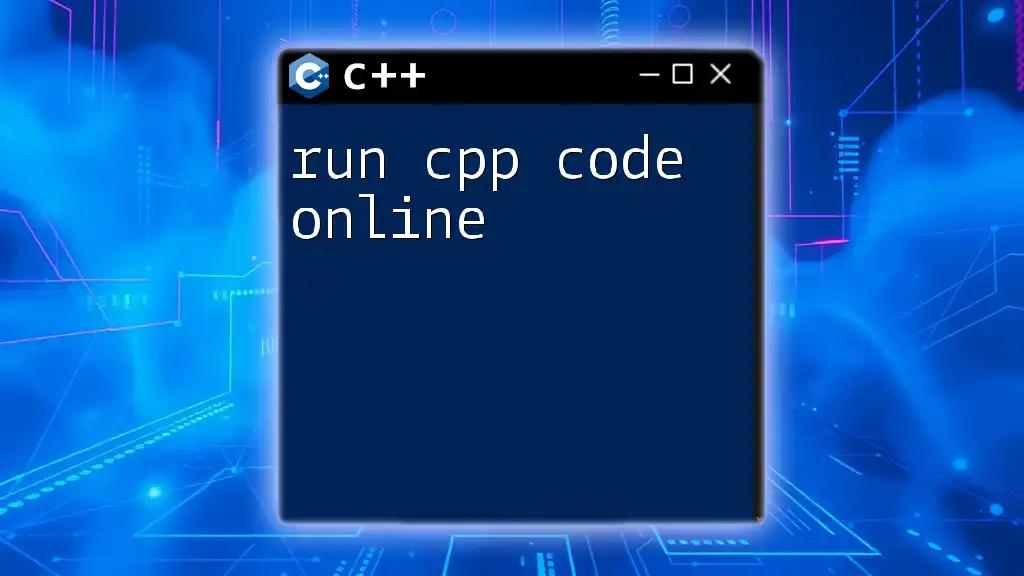
Best Practices for Using Hop Channels in RF24
Troubleshooting Common Issues
When implementing hop channels, developers may encounter specific issues like dropped packets or low throughput. Ensure your RF24 transceivers are configured correctly and that the channels you are using are not crowded. Consider adjusting the data rate or power level for optimal performance.
Optimizing Performance
To maximize data transmission efficiency, it's essential to:
- Choose less congested channels: Monitor the presence of other devices operating within the frequency range.
- Implement error handling: Use acknowledgment features provided by the RF24 library to ensure reliable communication.
- Adjust timing: Manipulating the frequency hopping rate can improve communication success, especially in dynamic environments.
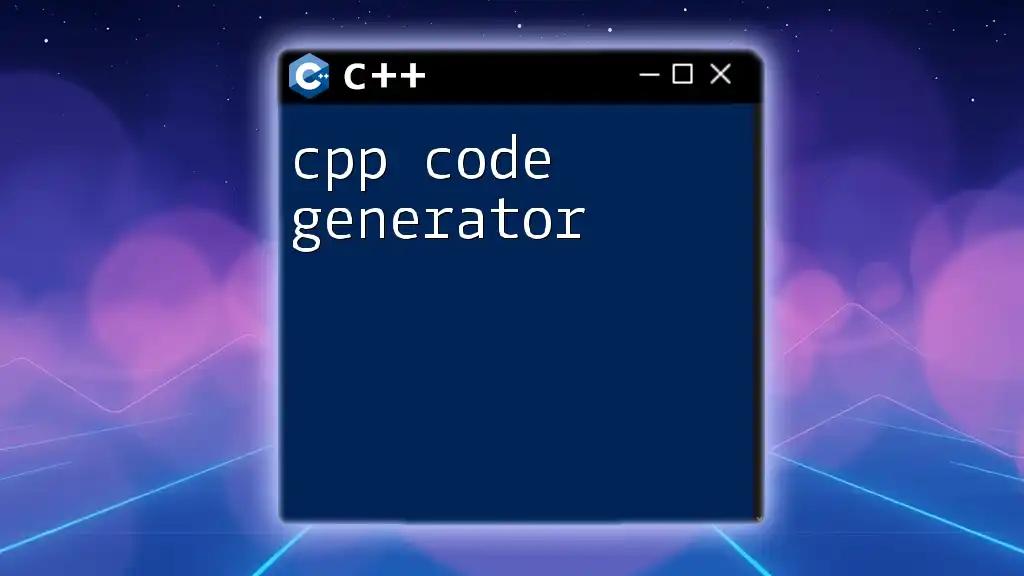
Advanced Techniques with RF24 Hop Channels
Customizing Hop Patterns
Creating specific hop patterns can optimize communications for particular scenarios. You can define a set sequence of channels to be utilized based on application needs, which can help ensure that communication remains consistent in known interference-prone areas.
Leveraging Advanced Features of RF24
In addition to hop channels, RF24 supports several advanced features that can enhance your application:
- Dynamic payloads: Cases where the amount of data transmitted varies can benefit significantly from this feature.
- Auto acknowledgment: Ensures that messages sent have been received successfully, which is critical when using hop channels.

Conclusion
Summary of Key Takeaways
In summary, implementing RF24 C++ hop channels allows for more reliable and flexible wireless communication. By utilizing frequency hopping, you can minimize interference, maximize throughput, and create robust IoT applications.
Call to Action
Explore the potential of hop channels by experimenting with your own RF24 projects. Consider enrolling in our courses to gain a deeper understanding of RF24 and C++ programming tailored to real-world applications.
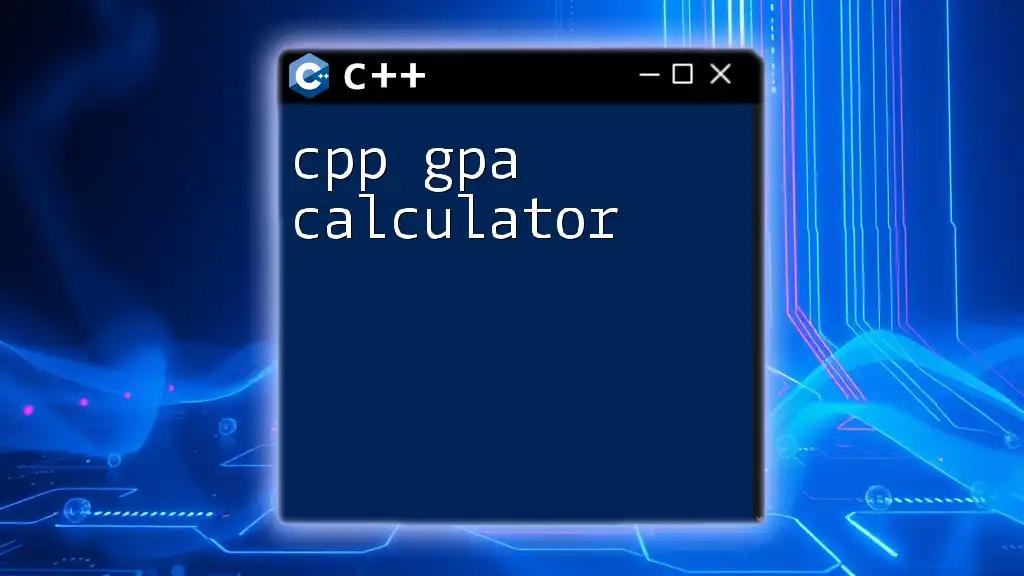
Additional Resources
Recommended Reading
- Books and articles focused on IoT communication, RF technology, and C++ programming can provide valuable insights into improving your skills.
Online Communities and Forums
Joining forums and communities dedicated to RF24 development can open up invaluable opportunities for learning, networking, and problem-solving. Participate actively to stay updated on the latest trends and solutions.