In 2024, C++ introduces several enhancements such as the "std::views" feature to improve range processing and "constexpr" capabilities, allowing for more efficient and expressive code.
#include <vector>
#include <iostream>
#include <ranges>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto evens = numbers | std::views::filter([](int n) { return n % 2 == 0; });
for (int even : evens) {
std::cout << even << " "; // Output: 2 4
}
}
New Features in C++ 2024
Enhanced Language Features
Introduction of Contracts
Contracts are a powerful concept in modern programming, allowing developers to specify preconditions, postconditions, and invariants for functions and classes. This feature enhances code robustness and reliability. For instance, you can define a contract for a function that ensures input parameters meet specific criteria before proceeding with its logic.
Usage: Contracts help ensure that bugs are caught early in the development process. By incorporating contracts into your codebase, you can reduce the likelihood of runtime errors.
Code Snippet:
#include <cassert>
contract requires(bool expression);
contract ensures(bool expression);
void divide(int numerator, int denominator) contract requires(denominator != 0)
ensures(denominator * result == numerator) {
return numerator / denominator;
}
Extended `constexpr` Capabilities
In C++ 2024, the `constexpr` feature has been significantly expanded, allowing for more complex computations to be evaluated at compile time. This means developers can now define intricate data structures, such as classes or arrays, using `constexpr`.
This enhancement not only improves performance by reducing runtime overhead but also allows for cleaner and more expressive code.
Example: You can now compute values such as factorials directly at compile time.
Code Snippet:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
constexpr int value = factorial(5); // value is computed at compile time
Enhanced Standard Library
New Algorithms in std namespace
With the introduction of new algorithms in the standard library, developers can write cleaner and more efficient code. These enhancements aim to make operations on collections and data structures simpler and more intuitive.
Usage: The new algorithms often provide better performance due to optimization and improved patterns.
Code Snippet: Here’s an example of using a new sorting algorithm, such as `std::sorting_abc`.
#include <vector>
#include <algorithm>
std::vector<int> vec = {3, 1, 4, 1, 5};
std::sorting_abc(vec.begin(), vec.end());
Support for More Data Types
The addition of new data types like `std::byte` expands the functionality and versatility of C++. This new data type allows for better type safety and clarity when handling raw memory and data manipulation tasks.
Example: The `std::byte` type is both type-safe and easier to manage, which can lead to fewer errors in systems programming.
Code Snippet:
#include <cstddef>
std::byte b = std::byte{0x01};
Improved Type Inference and Deduction
Reflection in C++
Reflection is becoming a crucial feature in C++ 2024, allowing programs to inspect and modify their own structure at runtime. This feature simplifies many tasks, such as serialization, debugging, and building frameworks or libraries.
Implications: Reflection can drastically change how developers approach code reuse and structure as it provides the necessary tools to handle types dynamically.
Code Snippet: You can explore class properties using reflective capabilities.
class Person {
std::string name;
int age;
};
auto properties = reflect<Person>(); // Generates metadata about the 'Person' class
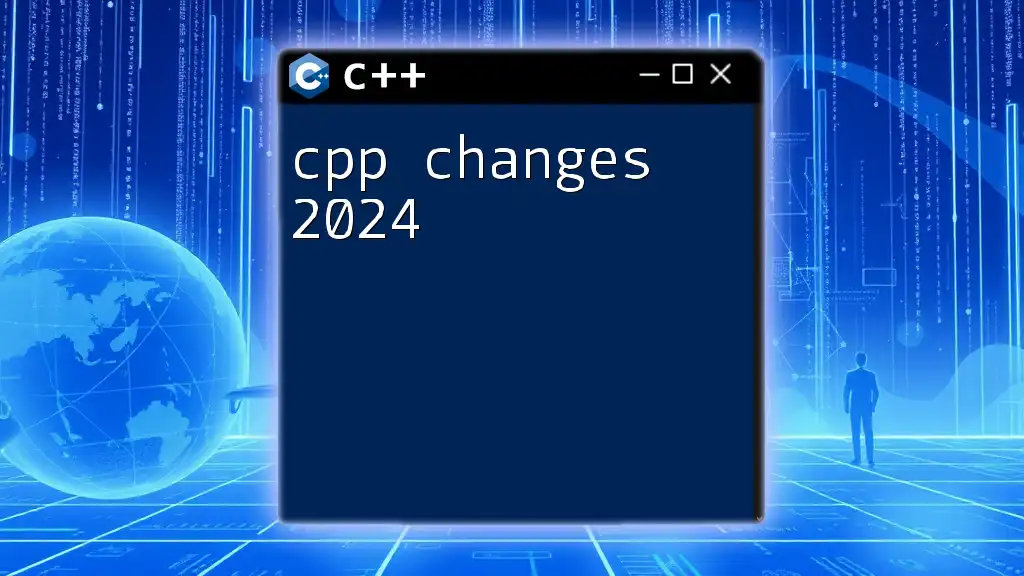
Deprecations and Removals
Deprecated Features
As C++ evolves, some features become outdated and are marked for deprecation. Developers should be aware of these changes to ensure they are using the most efficient and modern practices available.
Reasons: Features may be deprecated due to issues like poor design, lack of use, or the availability of superior alternatives.
Removed Features
Several features will be removed entirely in C++ 2024.
Feature 1: The old `auto_ptr` smart pointer has been deprecated in favor of `unique_ptr` or `shared_ptr`, which provide better memory management and ownership semantics.
Feature 2: The use of certain legacy input/output functions like `gets()` is also removed for safety and reliability reasons. Developers should adopt safer alternatives like `fgets()` or C++ streams.
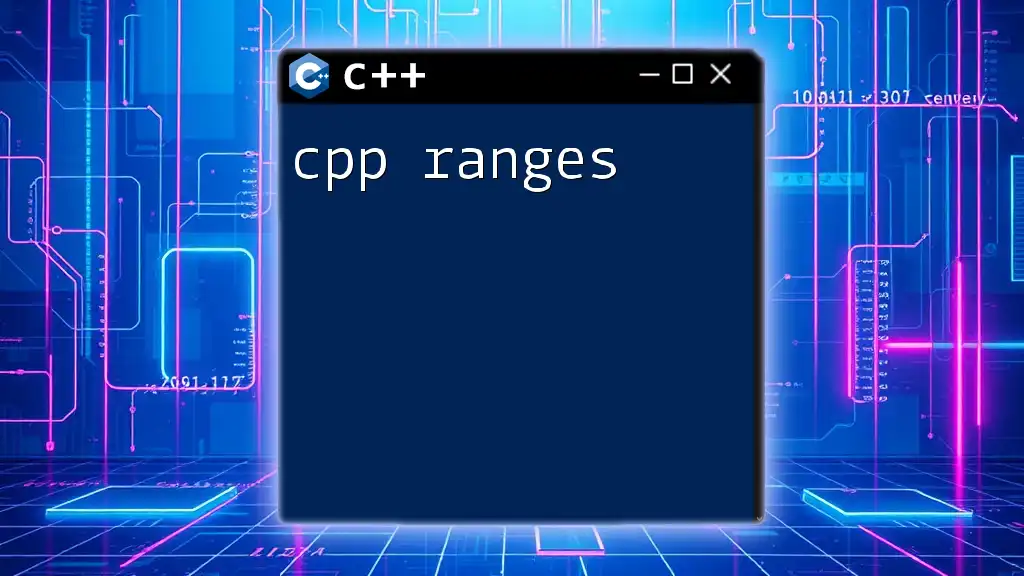
Best Practices with New Changes
Embracing Modern C++ Features
Incorporating the new features of C++ 2024 into your code is not merely a suggestion, but a necessity for forward-looking developers. By leveraging these advancements, you ensure that your code is efficient, readable, and maintainable.
Tips: Start by exploring small, individual features and gradually adopt them into larger projects to see how they can enhance overall performance and clarity.
Code Examples: Here’s a comparison of handling data between older and newer practices.
// Old way
void oldFunc(int* ptr);
// New way using smart pointers
#include <memory>
void newFunc(std::shared_ptr<int> ptr);
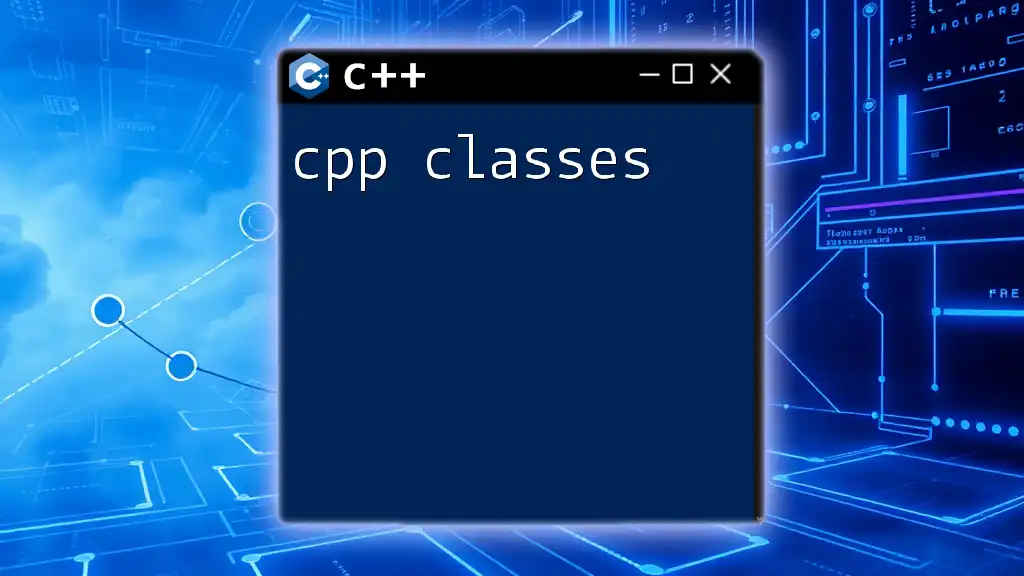
Impact of the Changes on Future Development
Future Trends
The changes introduced with C++ 2024 are expected to have a profound impact on the programming landscape. With increased emphasis on safety, efficiency, and developer experience, the language is positioned to remain relevant in a world rapidly evolving with the demands of software development.
Community Feedback
The C++ community plays an essential role in shaping the language's future. By voicing opinions and suggestions, developers influence the evolution of features. Engaging in community discussions and contributing to forums can provide valuable insights into best practices and the latest trends.
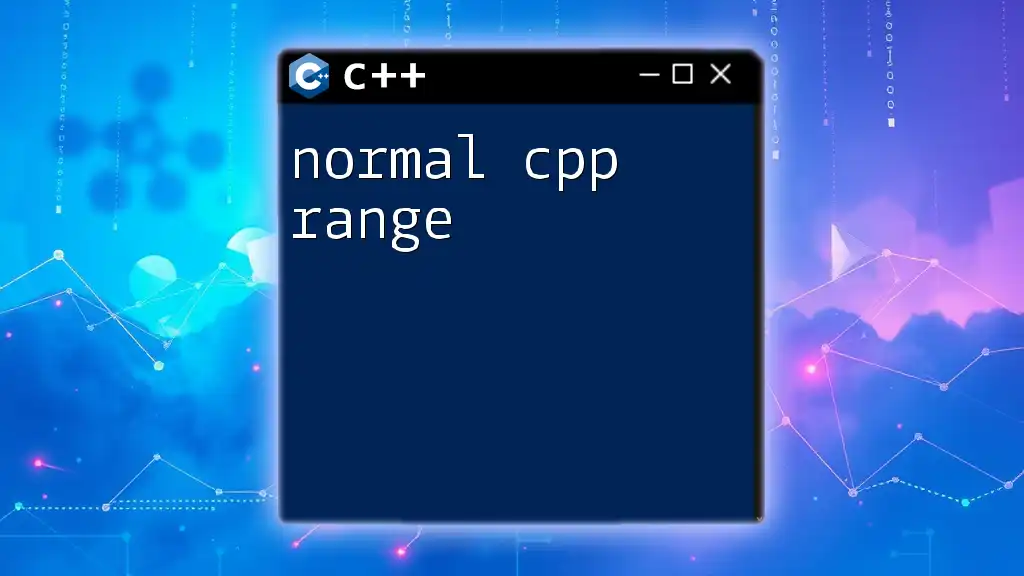
Conclusion
In summary, the 2024 C++ changes introduce significant advancements that enhance the language's capabilities and usability. By embracing features like contracts, expanded `constexpr`, and reflection, developers can produce more reliable and efficient code. Additionally, staying informed about deprecated features ensures that your coding practices remain modern and effective. As always, learning and adapting to these changes will put you at the forefront of C++ programming excellence.
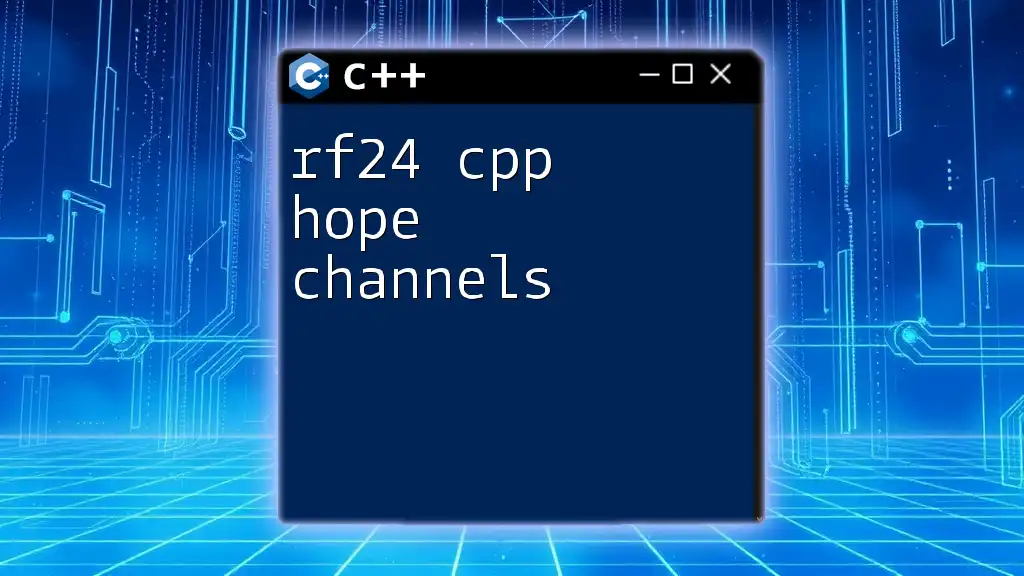
Additional Resources
To dive deeper into these changes, look for books on C++ that focus on the latest updates or enroll in online courses that emphasize C++ 2024. Engaging with C++ communities and forums can also be an excellent way to stay up-to-date and seek support.