A C++ class header defines the structure and behavior of a class, including its member variables and functions, and is typically placed in a separate file for better organization.
// Example of a C++ class header
class MyClass {
public:
MyClass(); // Constructor
void myFunction(); // Member function
private:
int myVariable; // Member variable
};
Understanding C++ Class Header Files
What is a C++ Class Header File?
A C++ class header file is a file that contains the definitions of classes, along with their member variables and function prototypes. Its primary purpose is to declare the structure and interfaces of the classes, allowing other parts of a program to interact with them without needing to see the actual implementation details. Typically, these header files have a `.h` or `.hpp` file extension.
Why Use Header Files in C++?
Using header files provides several advantages:
-
Separation of Interface and Implementation: This allows you to separate how a class works from how it is used. The header file describes the interface (i.e., how to use the class), while the implementation file contains the actual code.
-
Code Reusability and Maintainability: Once you create a header file, you can reuse it across multiple .cpp files. This helps in maintaining your code and reduces redundancy.
-
Reduced Compile Times: Splitting code into headers and implementation files can significantly speed up compile times, especially when working on large projects.
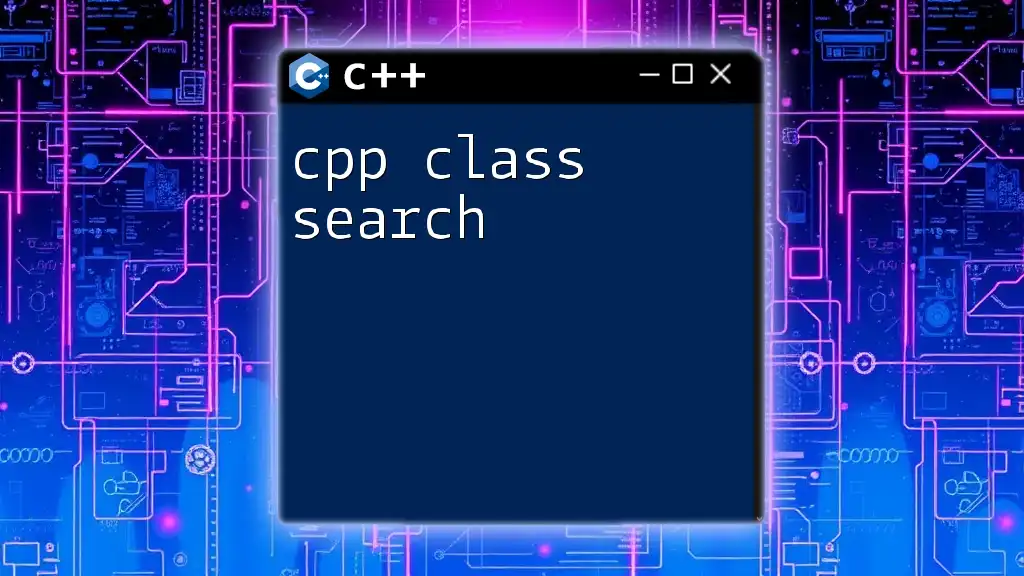
Structure of a C++ Class in a Header File
Basic Components of a C++ Class Header
A C++ class header generally consists of the following components:
-
Class Definition: This defines the class itself.
-
Member Variables: These are the data that belongs to the class.
-
Member Functions: These are the functions that define the capabilities of the class.
-
Access Specifiers: These determine the accessibility of class members (public, private, and protected).
Example of a Simple C++ Class in Header File
Below is a simple example of a class defined in a header file.
// MyClass.h
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClass {
public:
MyClass(int value);
void display();
private:
int number;
};
#endif
In this example:
- The `#ifndef` and `#define` directives prevent double inclusion of the header file.
- The constructor `MyClass(int value)` initializes a member variable, while `void display()` outputs the variable.
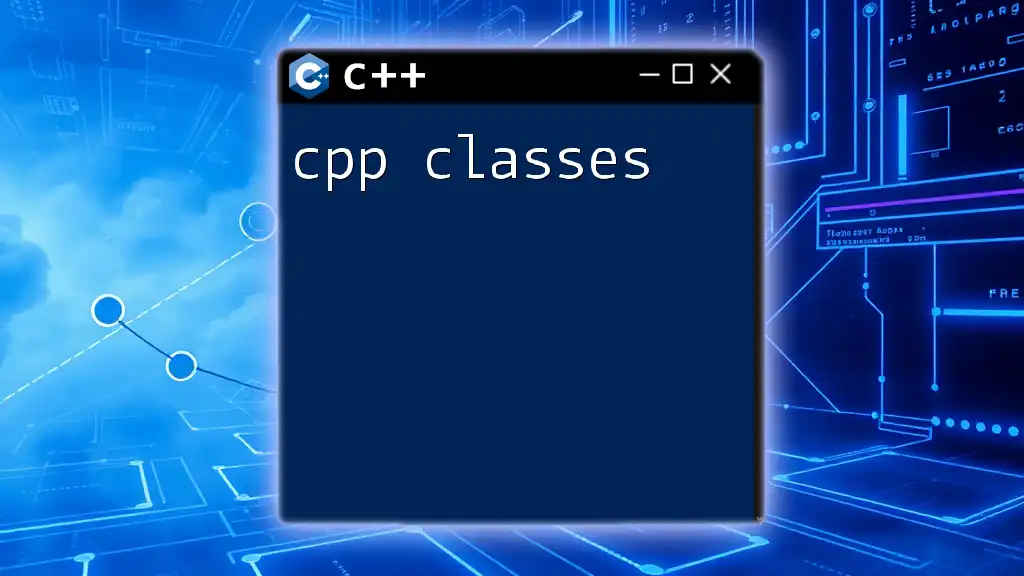
Creating a C++ Class Header: A Step-by-Step Guide
Step 1: Define the Class
When writing a class header file, start with defining the class itself. The syntax looks like this:
class Example {
// Members will go here
};
Step 2: Add Member Variables
Next, declare the member variables that will hold the data of your class.
private:
int value; // This is a member variable
Step 3: Implement Member Functions
Now, declare the functions that define how the class operates.
public:
void setValue(int v); // Declare a function
Step 4: Use Include Guards
Include guards are essential to prevent multiple inclusions of the same header file, which can lead to errors.
#ifndef EXAMPLE_H
#define EXAMPLE_H
// Class code
#endif
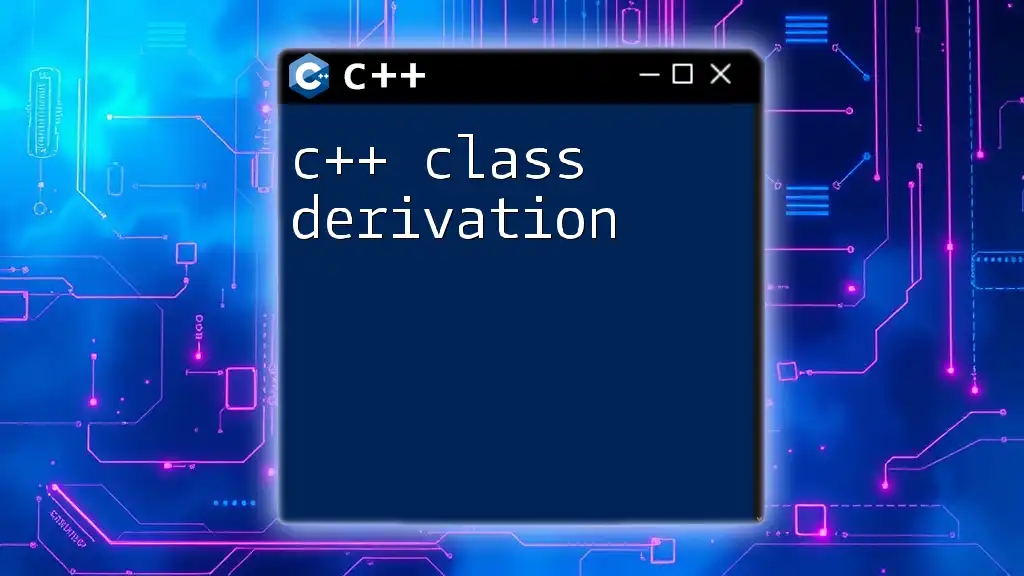
Using C++ Classes with Header Files
How to Include Header Files in C++ Implementation Files
To use a C++ class defined in a header file, you need to include that header file in your implementation (.cpp) file using the `#include` directive.
#include "MyClass.h"
Example of Implementing a Class Defined in a Header File
Here’s an example of how to implement a class defined in a header file.
// MyClass.cpp
#include "MyClass.h"
#include <iostream>
MyClass::MyClass(int value) : number(value) {} // Constructor implementation
void MyClass::display() {
std::cout << "Value: " << number << std::endl; // Display the value
}
In this example:
- The constructor initializes `number` using the member initializer list.
- The `display` function outputs the value of `number` to the console.
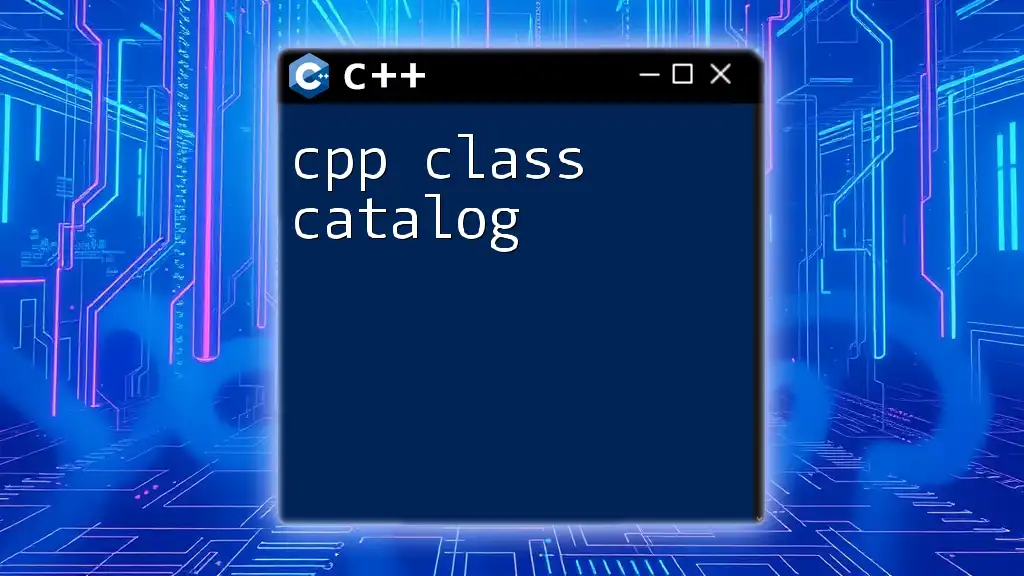
Common Mistakes When Using C++ Class Header Files
Forgetting to Include Guards
One common mistake is neglecting to use include guards. Not using them can result in compilation errors due to multiple definitions.
Incorrect Member Function Declarations
Another issue is making wrong declarations for member functions. For instance, forgetting the `class` scope can lead to confusion and errors during linking.
Circular Dependencies
Circular dependencies occur when two header files include each other, leading to compilation failures. To mitigate this, ensure that your header files are structured cleanly and use forward declarations where applicable.
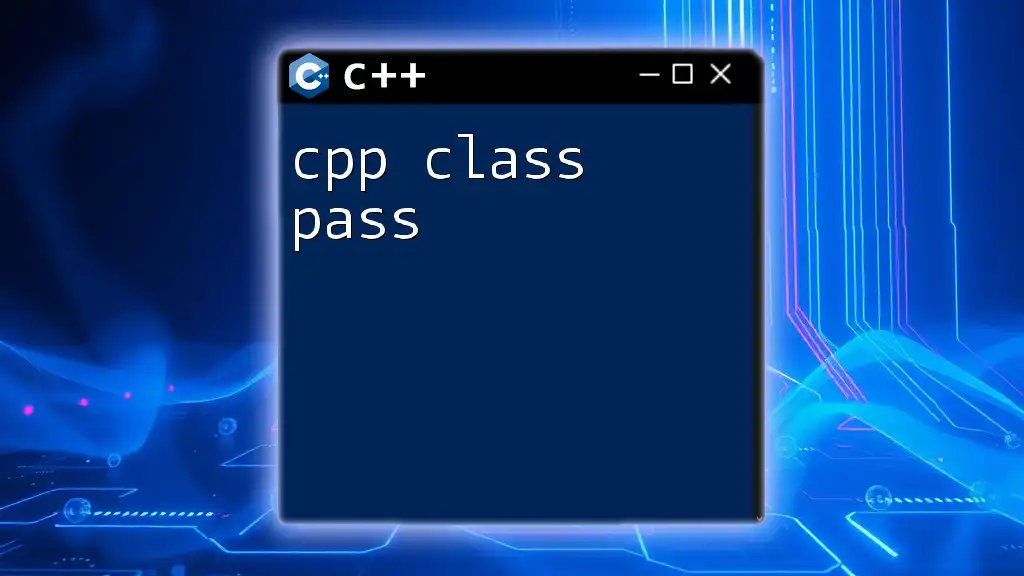
Best Practices for C++ Classes and Header Files
Organizing Code Effectively
It's important to group related classes and keep header files concise. This makes it easy for others to read and use your code. Try to limit each header file to a single class or closely-related classes.
Consistency in Naming Conventions
Adopt clear naming conventions. For example, use the same prefix or suffix for related classes to easily identify them in your codebase.
Documenting Your Classes
Documentation is key to maintaining your code. Write clear comments within your header files to explain the role of each class, its member variables, and functions. This is particularly useful for teams and can greatly enhance code readability.
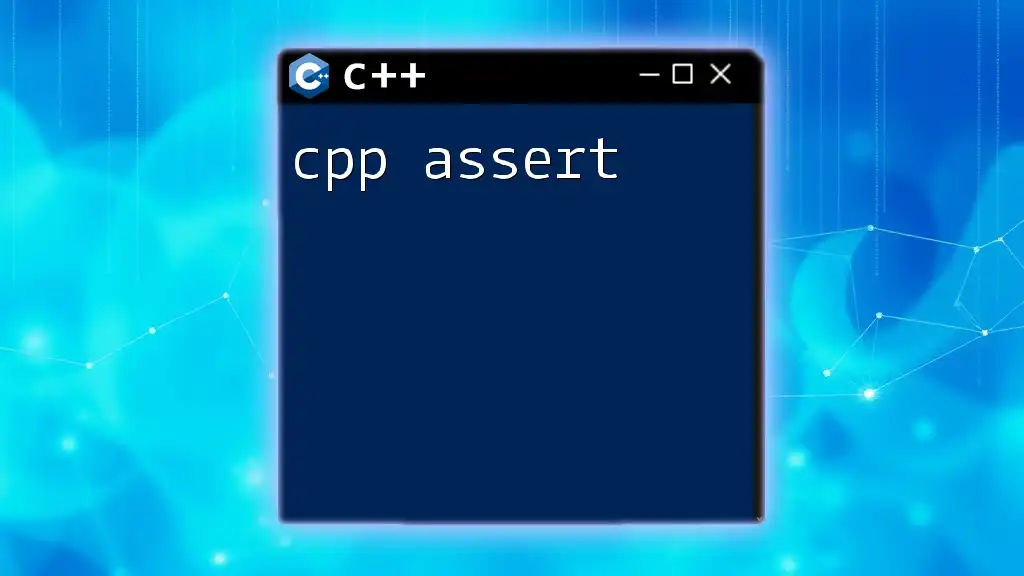
Conclusion
In this article, we've explored the concept of a C++ class header extensively. From understanding the structure and purpose of class header files to creating and implementing them, knowing how to correctly use class headers is fundamental to mastering C++. We encourage you to practice by creating your classes and header files. If you have questions or feedback, don't hesitate to reach out and explore more resources to enhance your C++ programming skills.