The "cpp class search" involves utilizing C++ classes and their members to effectively locate and access data or functionalities within a project.
Here's a simple code snippet demonstrating a class search functionality using a standard C++ class:
#include <iostream>
#include <vector>
#include <algorithm>
class Item {
public:
Item(std::string name, int id) : name(name), id(id) {}
std::string name;
int id;
};
class Inventory {
private:
std::vector<Item> items;
public:
void addItem(const Item& item) { items.push_back(item); }
Item* searchItemById(int id) {
auto it = std::find_if(items.begin(), items.end(), [id](Item& item) { return item.id == id; });
return (it != items.end()) ? &(*it) : nullptr;
}
};
int main() {
Inventory inventory;
inventory.addItem(Item("Laptop", 1));
inventory.addItem(Item("Phone", 2));
Item* foundItem = inventory.searchItemById(1);
if (foundItem) {
std::cout << "Found Item: " << foundItem->name << std::endl;
} else {
std::cout << "Item not found." << std::endl;
}
return 0;
}
Understanding C++ Classes
What is a Class in C++?
In C++, a class acts as a blueprint for creating objects. It encapsulates data members (attributes) and member functions (methods) that operate on those attributes, allowing for better organization and modularity. The basic syntax of a class is as follows:
class ClassName {
public:
// Data members
int data;
// Member function
void memberFunction() {
// Function definition
}
};
Importance of Classes in C++
Classes form the backbone of object-oriented programming in C++. They facilitate key principles such as encapsulation and data hiding, allowing developers to bundle data and behavior together. This improves code reusability, flexibility, and maintainability. By using classes, developers can create more sophisticated systems that model real-world entities.
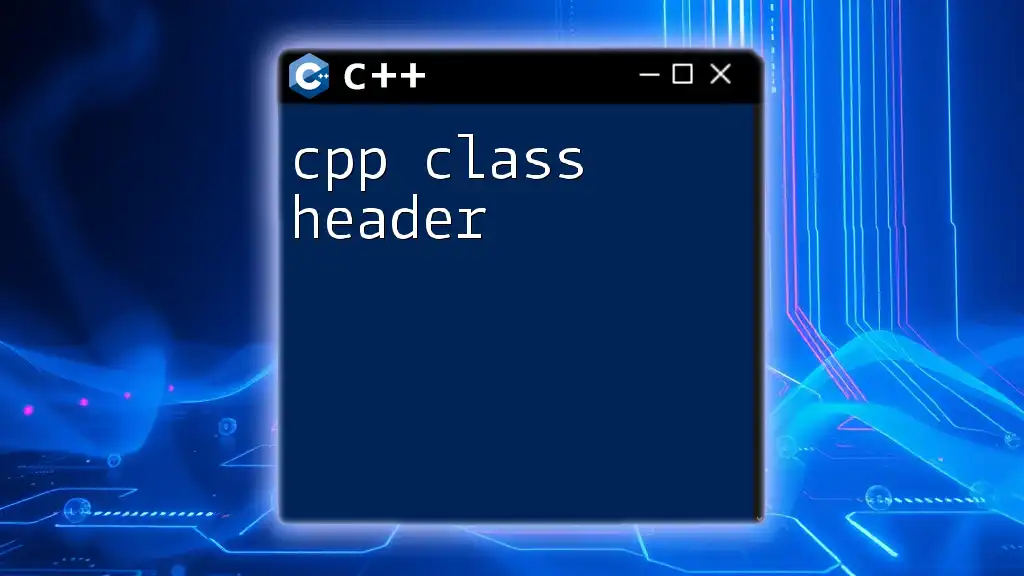
Searching for Classes in C++ Code
Why Search for Classes?
In larger projects, searching for classes becomes crucial for maintaining a seamless workflow. It helps developers navigate through complex codebases, understand dependencies, and efficiently locate functionality. The need arises frequently when modifying a class, debugging issues, or refactoring code. Efficient searching capabilities save time and reduce errors.
Tools for Class Search
Integrated Development Environment (IDE)
Modern IDEs like Visual Studio and Code::Blocks include powerful searching tools. These environments often allow you to search files directly, facilitating quick access to class definitions.
- Open the search feature in your IDE.
- Enter the keyword “class” followed by the class name (e.g., `class MyClass`).
- Access and edit the definitions as needed.
Command Line Tools
For developers who prefer the command line, tools like grep can be tremendously helpful. The following command recursively searches for class definitions within your codebase:
grep -r "class " /path/to/your/code/
This command will return all occurrences of class declarations in your specified directory, streamlining your search process.
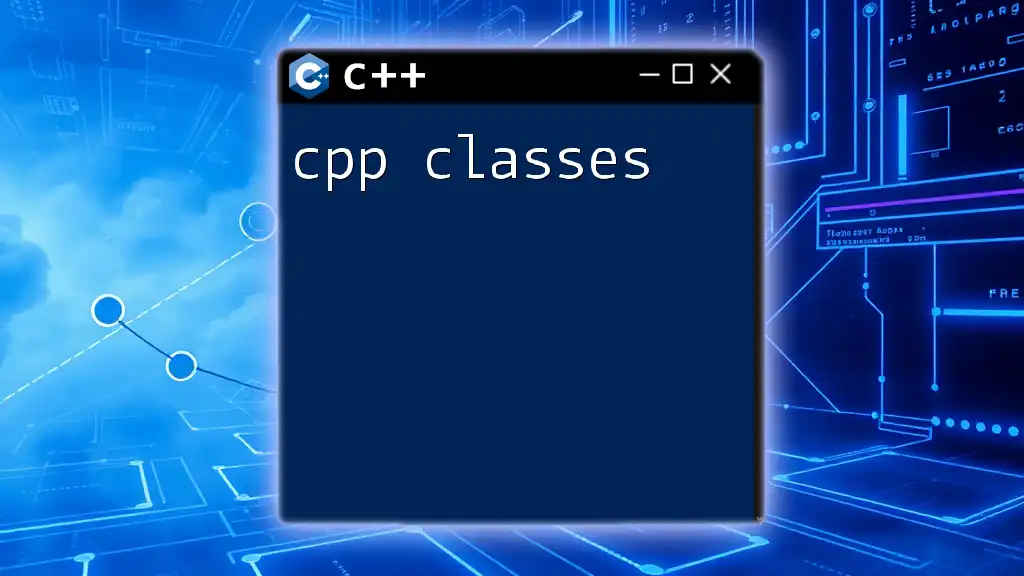
Best Practices for Class Organization
Naming Conventions
When naming classes, clarity is paramount. Names should be descriptive to reflect the purpose of the class, following the typical convention of capitalizing the first letter of each word:
class EmployeeRecord {
// class members
};
Consistent naming conventions enhance readability, making it easier for developers to search for specific classes within a codebase.
Documentation and Comments
To further aid in class searchability, utilize comments and documentation:
- Inline comments should explain complex sections of the code.
- Docstrings can provide a top-level overview of class functionality.
Example docstring:
/**
* Class representing an Employee.
* Contains attributes and methods related to employee management.
*/
class Employee {
// class definition
};
Such documentation allows anyone reading the code to get context quickly and enhances the searchability of related functionalities.
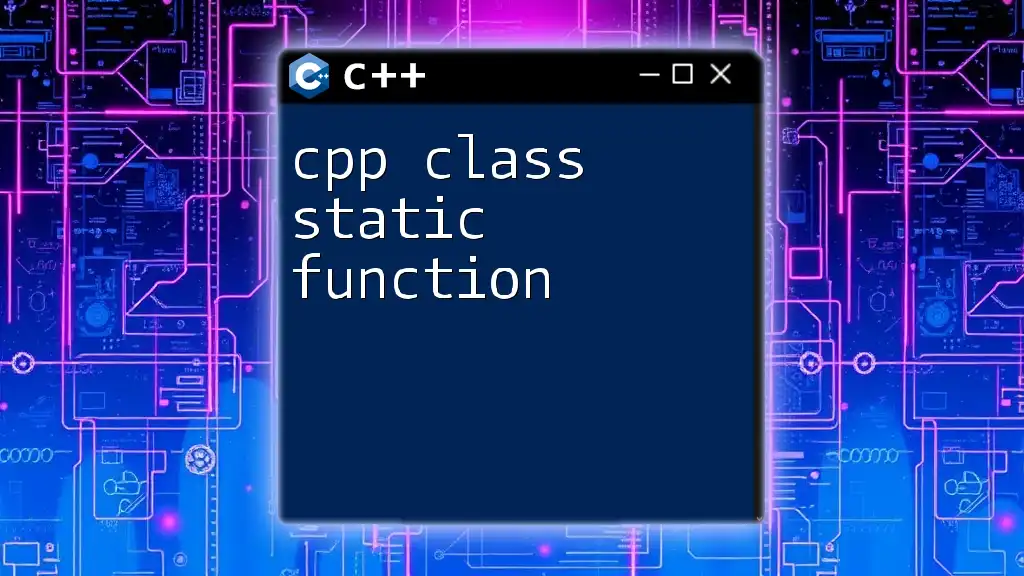
Leveraging C++ Features for Efficient Class Search
Forward Declarations and the `#include` Directive
Using forward declarations effectively can impact how you search for classes. By declaring a class with a forward declaration before its usage, you can manage dependencies better and minimize the need for unnecessary includes in header files, which speeds up your compilation times.
Example of a forward declaration:
class MyClass; // Forward declaration
class AnotherClass {
MyClass* myClassPointer;
};
Utilizing Namespaces
Namespaces are essential for organizing classes and preventing naming collisions. When searching for classes, you can quickly narrow down your search by specifying namespaces.
Example:
namespace MyNamespace {
class MyClass {
// class definition
};
}
When searching through files, you can now perform searches like `MyNamespace::MyClass` to pinpoint the exact class you need.
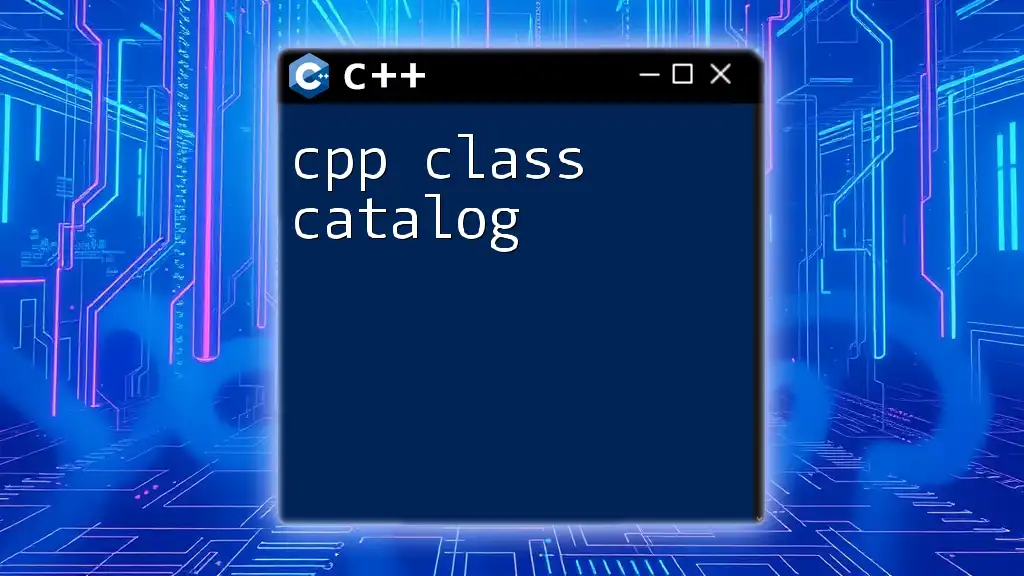
Advanced Searching Techniques
Utilizing Reflection (if applicable)
In modern C++ (from C++17 onwards), some features allow the use of reflection, meaning you can programmatically inspect the structure and attributes of classes. This can be a powerful tool in understanding your code and its components without delving into every file manually.
Static Analysis Tools
Static analysis tools, such as CPPCheck or Clang-Tidy, can be integrated into your workflow to help identify class usage and dependencies. These tools enhance code quality and allow you to search through your codebase for issues related to class relationships, improving your overall development process.
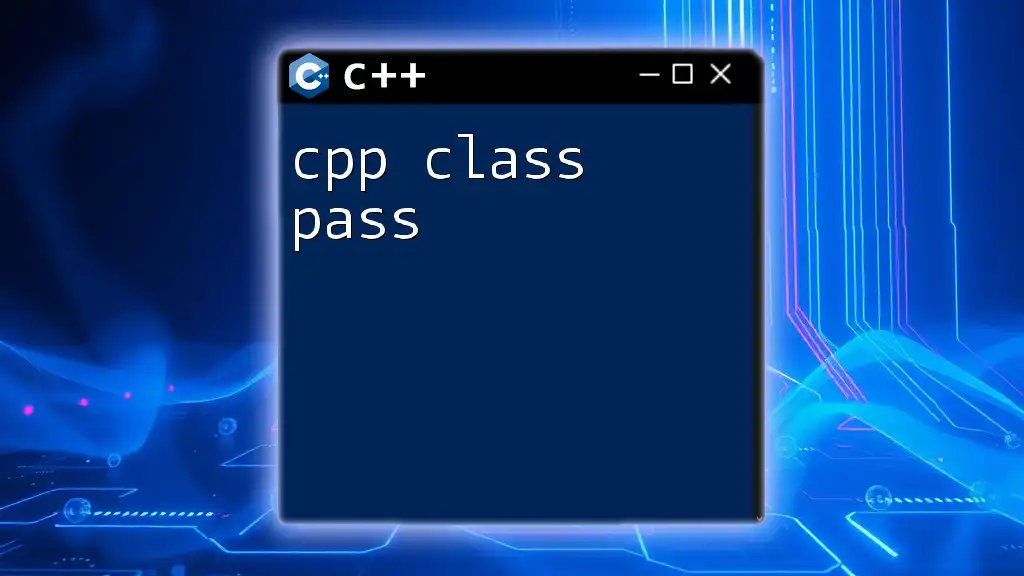
Searching for Class Inheritance and Relationships
Understanding Class Hierarchies
Class hierarchies illustrate how classes relate to one another through inheritance. Understanding these relationships is crucial, especially when modifying parent or derived classes. Searching for inheritance manifests as a means to explore how properties and behaviors are shared across classes.
Tools for Visual Representation of Class Structure
Using tools like UML, you can create visual representations of class hierarchies. Visual aids enhance your understanding of how classes interact, making it simpler to track down relationships and dependencies in your searching efforts.
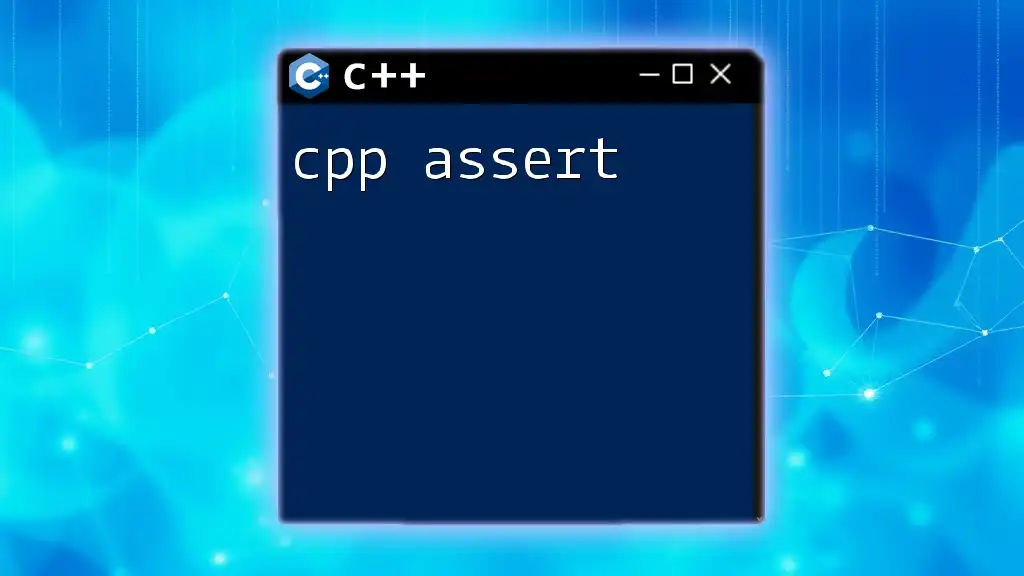
Conclusion
Efficient `cpp class search` is essential for maintaining well-organized and navigable codebases. By leveraging IDE features, command line tools, and adhering to best practices, you can significantly enhance your ability to manage classes effectively. Whether you’re a beginner or an experienced developer, implementing these strategies will optimize your workflow and save you precious development time.
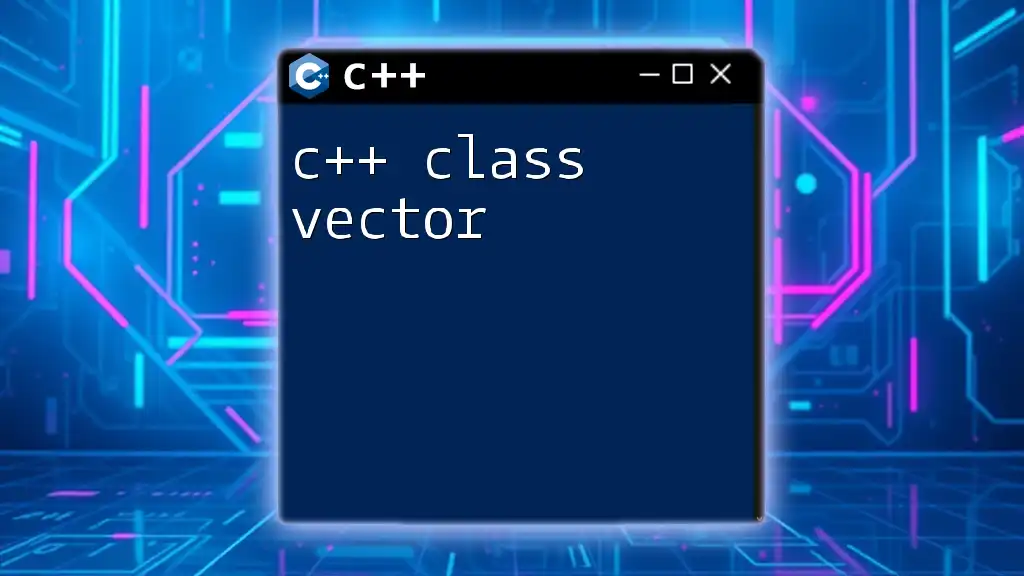
Additional Resources
To further your understanding of searching and working with classes in C++, consider exploring recommended readings, tutorials, and community forums dedicated to C++. Engaging with these resources will deepen your mastery of `cpp class search` and improve your coding practices overall.
By applying the knowledge gained from this guide, you will be better equipped to navigate the complexities of C++ class structures and ultimately become a more proficient developer.