`std::regex_search` is a function in C++ that searches for a regular expression match within a specified string, returning a boolean value indicating whether a match was found.
Here’s a simple example of using `std::regex_search`:
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string text = "The quick brown fox jumps over the lazy dog.";
std::string pattern = "fox";
std::regex regexPattern(pattern);
if (std::regex_search(text, regexPattern)) {
std::cout << "Match found!" << std::endl;
} else {
std::cout << "No match found." << std::endl;
}
return 0;
}
Understanding Regular Expressions
What are Regular Expressions?
Regular expressions, commonly known as regex, are sequences of characters that form a search pattern. They are used primarily for string pattern matching and manipulation. The concept of regex dates back several decades and has evolved significantly to become an essential tool in programming for tasks like string searching, validation, and even data extraction.
Importance of Regex in C++
In the context of C++, regular expressions play a crucial role in simplifying complex string manipulations. Instead of using verbose and inefficient string handling techniques, developers can harness regex to efficiently search, replace, and validate strings. Common applications include validating formats for email addresses, phone numbers, and parsing structured data from text files.
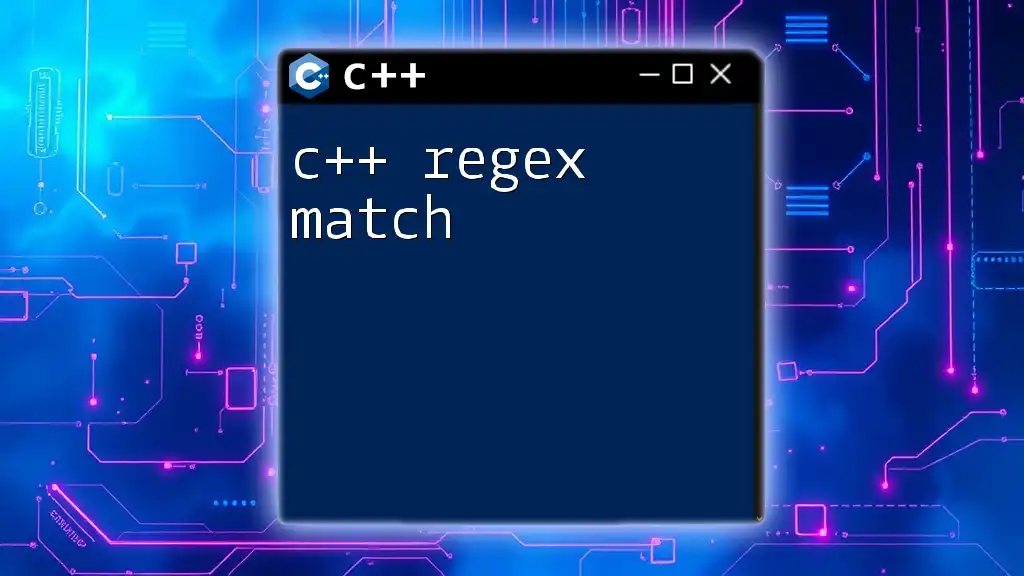
C++ `regex_search`
Definition and Purpose
The `regex_search` function in C++ provides a way to search for a regex pattern within a target string. Unlike `regex_match`, which checks if an entire string matches a pattern, `regex_search` looks for a match of the pattern anywhere in the string, making it ideal for partial string matching tasks.
Syntax of regex_search
The basic syntax for `regex_search` is as follows:
std::regex_search(const std::string& str, std::smatch& matches, const std::regex& pattern);
- Input string (`str`): The string to be searched.
- Output match results (`matches`): A `std::smatch` object that holds matched results.
- Regex pattern (`pattern`): The regex pattern to be searched for.
- Optional flags: These can modify the behavior of the search.
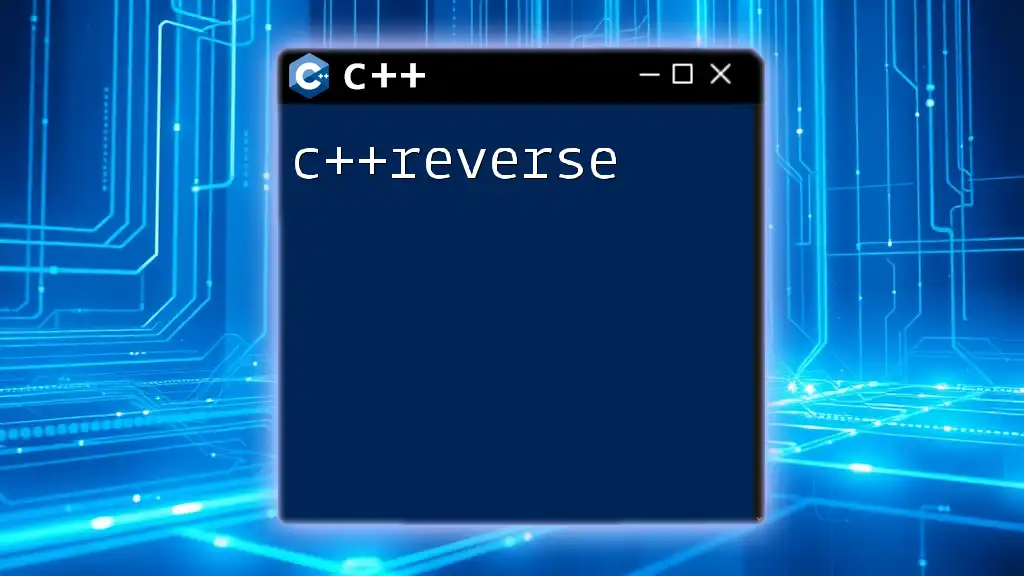
Setting Up Your Environment
Required Headers
To use `regex_search`, you need to include the following headers:
#include <regex>
#include <string>
#include <iostream>
- `<regex>`: Contains all the regex functionalities.
- `<string>`: Provides support for string data types.
- `<iostream>`: Needed for input and output operations.
Compiling with Regex Support
Make sure that your compiler supports C++11 or later, as regex was introduced in the C++11 standard. Use flags like `-std=c++11` for GCC or Clang when compiling your code.
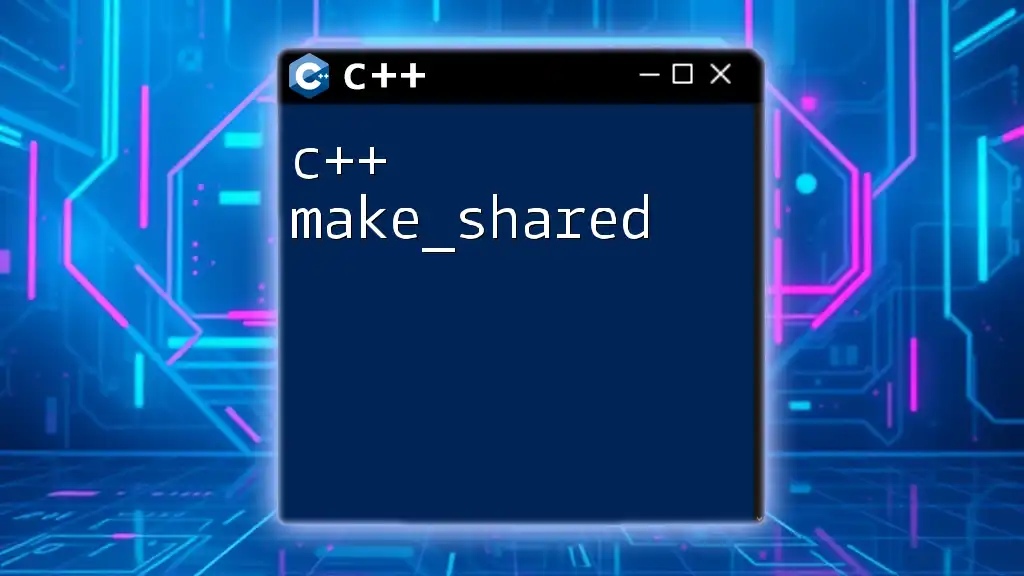
Basic Usage of `regex_search`
Simple Example
Here's a straightforward example demonstrating the use of `regex_search`:
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string text = "Hello, my email is example@mail.com";
std::regex pattern(R"((\w+)(@\w+\.\w+))");
std::smatch matches;
if (std::regex_search(text, matches, pattern)) {
std::cout << "Email found: " << matches[0] << std::endl;
} else {
std::cout << "No match found." << std::endl;
}
return 0;
}
In this example:
- We define a string, `text`, containing a phrase with an email address.
- The regex pattern is defined to capture sequences of word characters followed by an '@' symbol and a domain.
- The `std::smatch matches;` object holds any matches found during the search.
Output Explanation
If the email is found in the string, `matches[0]` will contain the entire matched portion (e.g., `example@mail.com`). The program will print "Email found: example@mail.com" to the console. If no match is found, it will simply state "No match found."
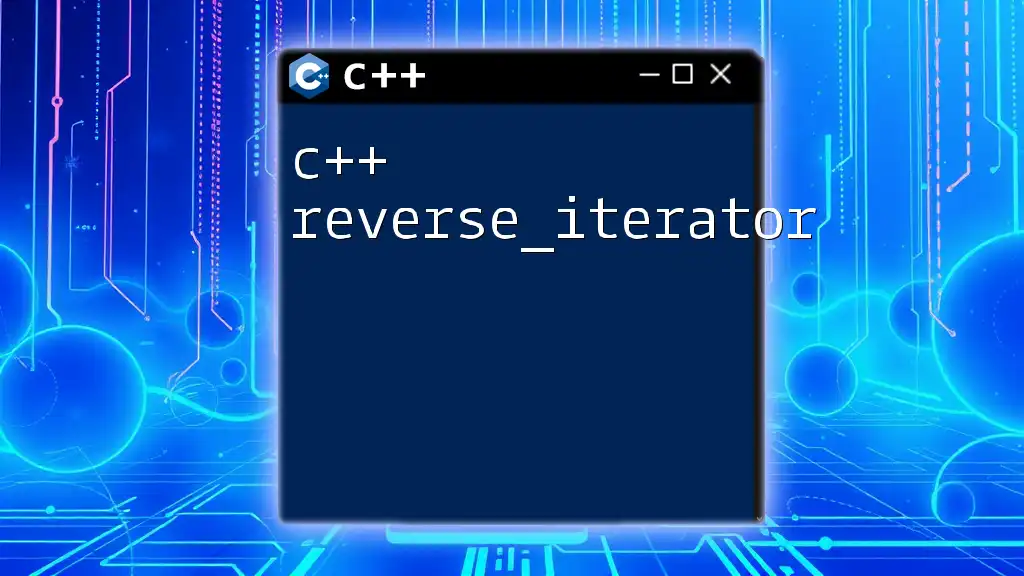
Advanced Patterns in regex_search
Special Characters and Escaping
Regular expressions use special characters to define their patterns. Characters like `.` (dot), `*` (asterisk), and `+` (plus) have specific meanings and need to be escaped with a backslash (`\`) when you want to use them literally in a pattern. For instance, to match a period, you would use `\.` in your regex.
Using Flags with regex_search
You can modify how `regex_search` behaves through flags. For example, using `std::regex_constants::icase` makes the search case-insensitive:
std::regex pattern(R"((hello|Hello))", std::regex_constants::icase);
This allows you to find matches regardless of their case, making your search more flexible and user-friendly.
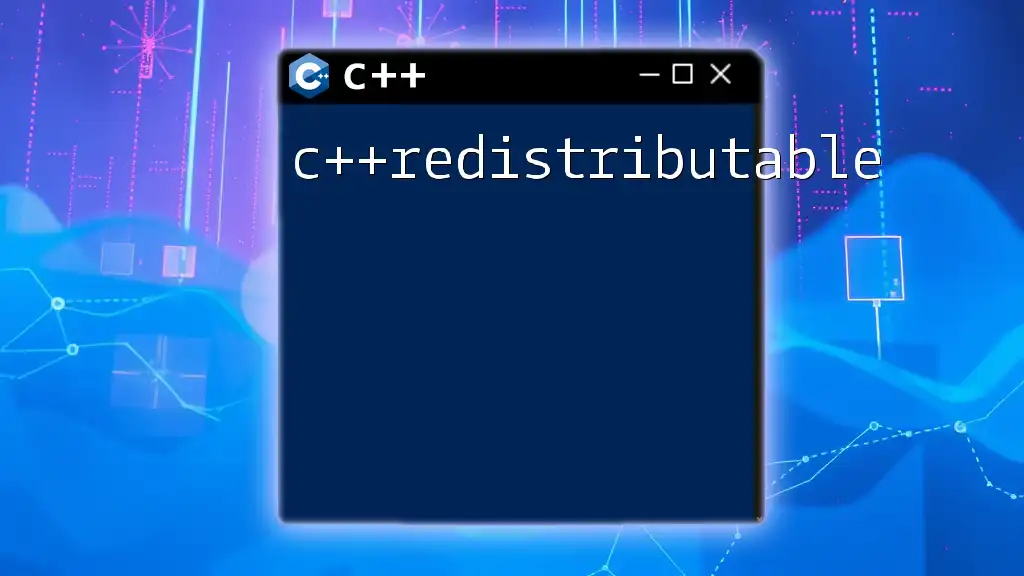
Handling Complex Patterns
Anchors and Boundaries
Anchors such as `^` (beginning of a line) and `$` (end of a line) can be incorporated into your regex expressions to assert positions within your string. For example:
std::regex pattern(R"(^Hello)");
This pattern matches any string that starts with "Hello".
Quantifiers
Quantifiers in regex allow you to specify the number of occurrences of a pattern. The symbols `*`, `+`, `?`, and `{n,m}` provide valuable ways to express frequency. For instance:
- `*` matches zero or more occurrences.
- `+` matches one or more occurrences.
- `?` matches zero or one occurrence.
- `{n,m}` specifies between n and m occurrences.
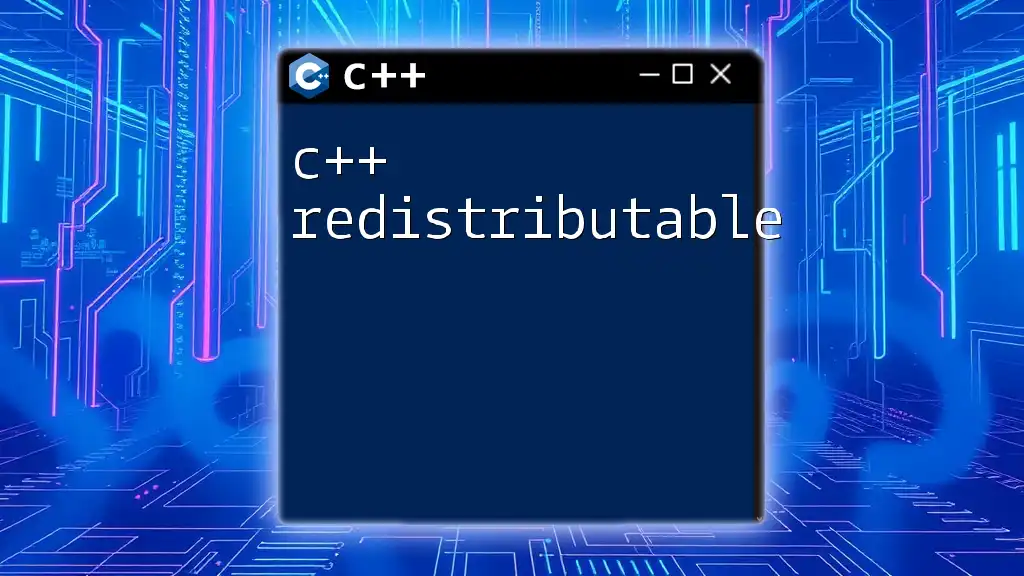
Performance Considerations
Evaluating the Performance of regex_search
While `regex_search` is a powerful tool for string manipulation, it can be less efficient compared to more traditional methods in certain scenarios. It's essential to assess when to use regex versus simple string functions, such as `std::string::find()` or `std::string::substr()`, especially in performance-critical applications. Profile your code to understand the impact.
Common Pitfalls and Optimizations
When working with regex, keep an eye out for overly complex patterns that can lead to performance bottlenecks. Always test your expressions with various inputs to ensure that they perform optimally. Additionally, simplifying your regex when possible and using non-capturing groups (using `(?:...)`) can improve performance.
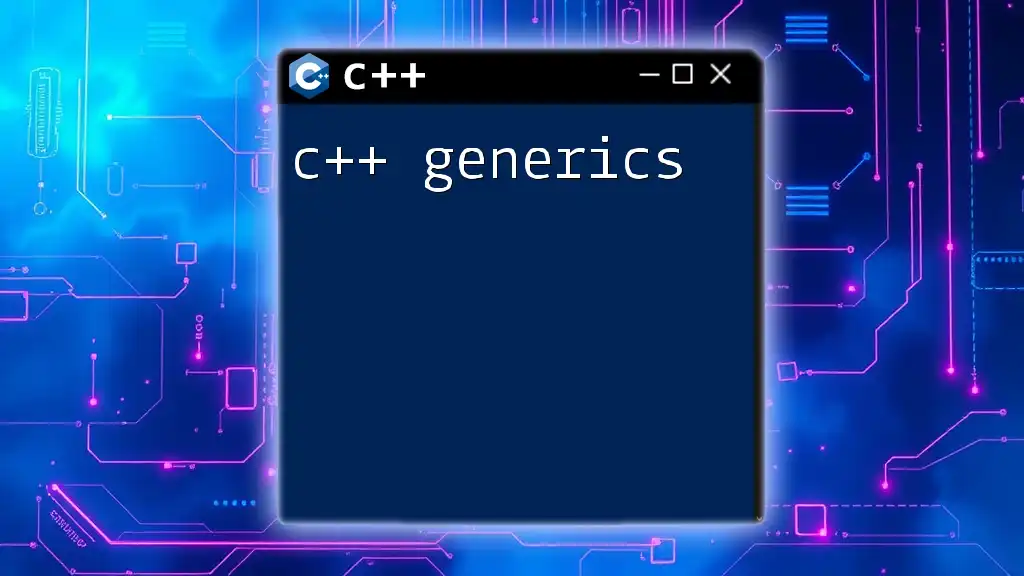
Practical Applications of regex_search
Input Validation
You can employ `regex_search` for input validation. For example, to ensure user inputs like email addresses are in the correct format, a regex pattern can be crafted specifically for that purpose. Here’s a simple email validation example:
std::regex email_pattern(R"((\w+)(@\w+\.\w+))");
This pattern can be used to validate user-provided email addresses effectively.
Text Processing and Extraction
Using `regex_search`, you can extract specific pieces of information from larger texts. For instance, scraping emails from a document involves defining an appropriate regex pattern to identify and capture email addresses efficiently. This cannot only save time but also reduce errors compared to manual extraction methods.
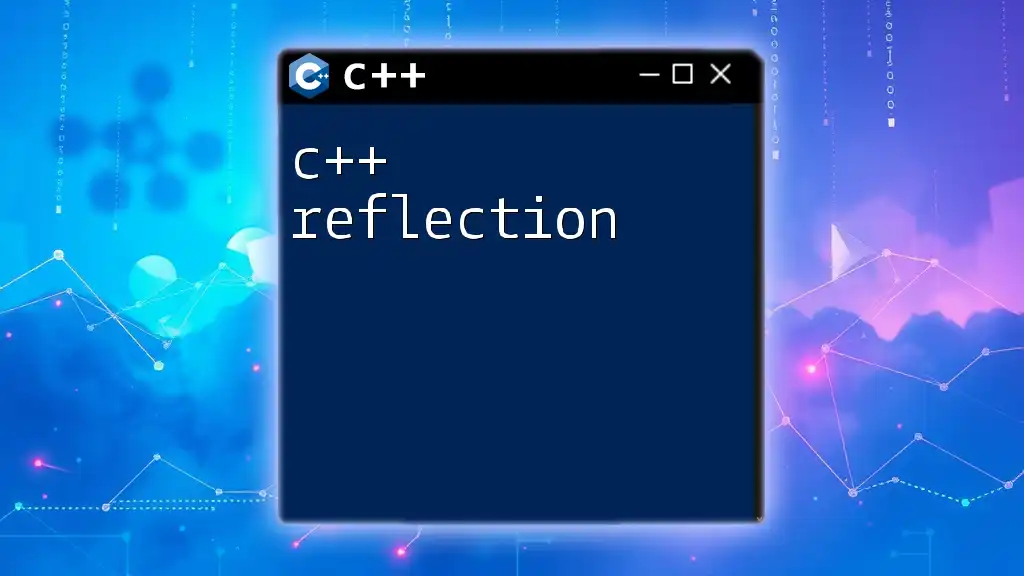
Conclusion
Summary of Key Points
This guide has explored the depth of `c++ regex_search`, covering its syntax, use cases, advanced features, and performance considerations. We've seen how powerful regex can be for string manipulation and pattern matching.
Encouragement for Further Learning
As you continue your journey with C++ and regex, don't hesitate to experiment with different regex patterns. Review additional resources on regex and consider diving deeper into its endless applications in programming.
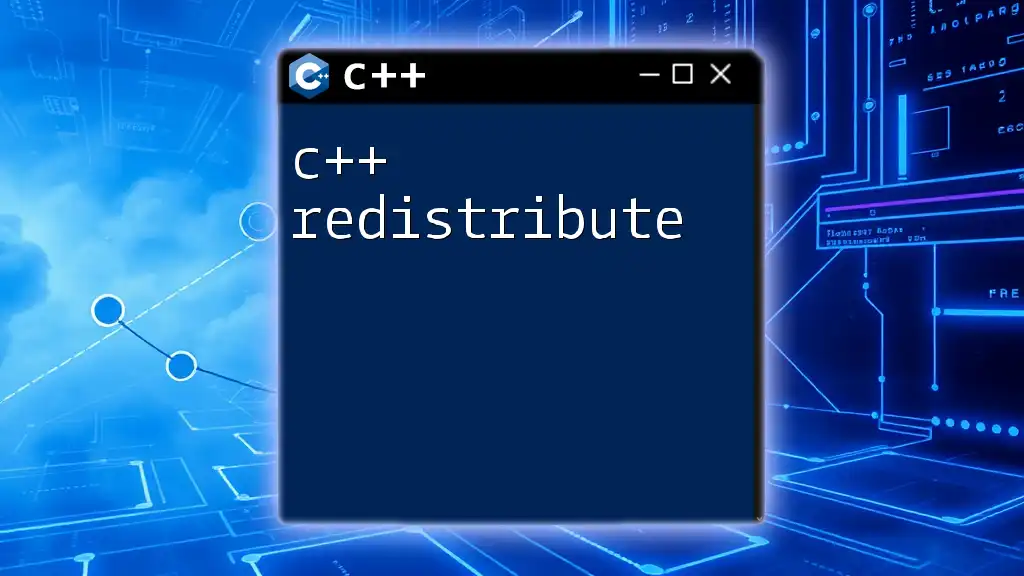
Additional Resources
References and Further Reading
For further exploration, consider checking reputable documentation sites like cppreference.com or online courses that focus on C++ programming and regex functionalities.
Example Code Repository
You can find the complete example code in repositories like GitHub, where similar regex applications in C++ are shared for learning and experimentation.