A `reverse_iterator` in C++ allows you to iterate through a container in reverse order, providing a convenient way to access elements from the end to the beginning.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Using reverse_iterator to print elements in reverse order
for (auto rit = vec.rbegin(); rit != vec.rend(); ++rit) {
std::cout << *rit << " ";
}
return 0;
}
Overview of `reverse_iterator`
Definition of `reverse_iterator`
The `reverse_iterator` in C++ is a special type of iterator that allows you to traverse a container in reverse order, meaning you can access the elements from the last to the first. This can be incredibly useful in various programming scenarios where backward traversal is desirable.
Use Cases for `reverse_iterator`
The `reverse_iterator` is particularly valuable in the following situations:
- Searching from the end: When you want to find elements starting from the tail of a container.
- Reverse operations: When performing operations that require reversing the data processing order.
- Display routines: When you want to show elements in reverse order without modifying the original container.
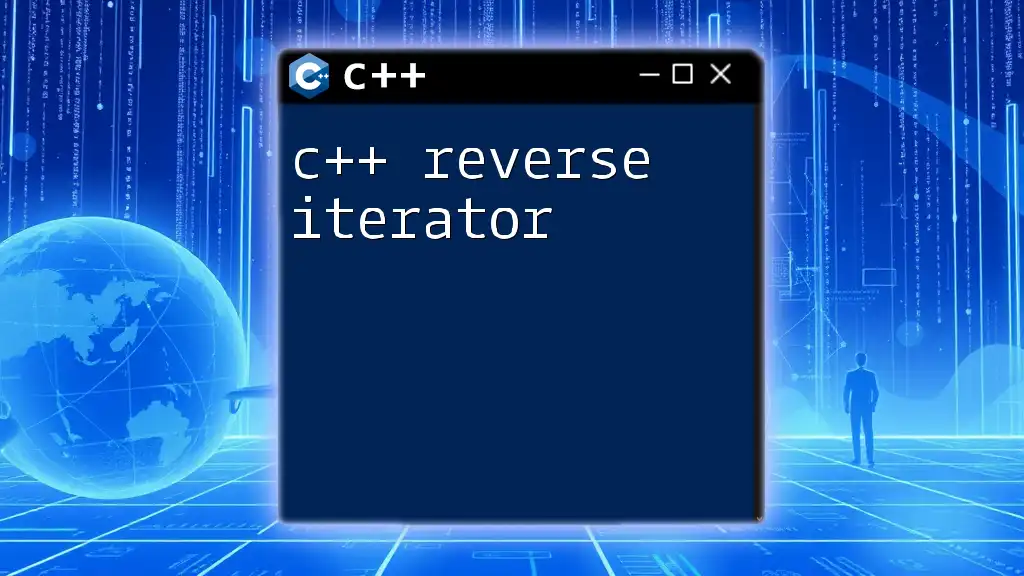
Basic Syntax and Implementation
Including Necessary Headers
Before you can utilize `reverse_iterator`, ensure to include the appropriate headers in your C++ code:
#include <vector>
#include <iostream>
Creating a Simple Reverse Iterator
To create a simple reverse iterator, you can use the `rbegin()` and `rend()` functions provided by STL containers, such as `vector`. Here’s how to do it:
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::reverse_iterator rit = vec.rbegin();
In this snippet:
- `rbegin()` returns a reverse iterator to the last element of the vector.
- `rend()` returns a reverse iterator to one position before the first element.
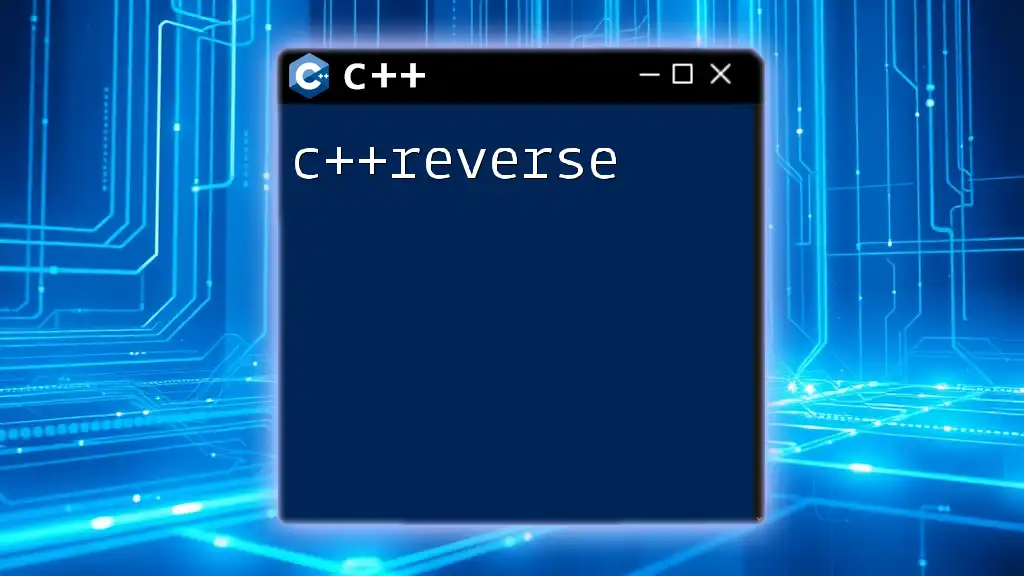
Characteristics of `reverse_iterator`
How `reverse_iterator` Works
The real power of the `reverse_iterator` lies in its ability to smoothly transition through the container in reverse order. The iterator starts at `rbegin()` and stops at `rend()`. Here’s a simple loop demonstrating reverse iteration:
for (auto rit = vec.rbegin(); rit != vec.rend(); ++rit) {
std::cout << *rit << " ";
}
When executed, this will output:
5 4 3 2 1
This output confirms that we are indeed traversing the list in reverse order.
Incrementing and Decrementing
When you use `reverse_iterator`, the increment operator (`++`) moves the iterator to the preceding element in the original container. Conversely, the decrement operator (`--`) moves it to the next element, which follows a different logic than with regular iterators. Keeping this in mind can save you from confusion when manipulating iterators.
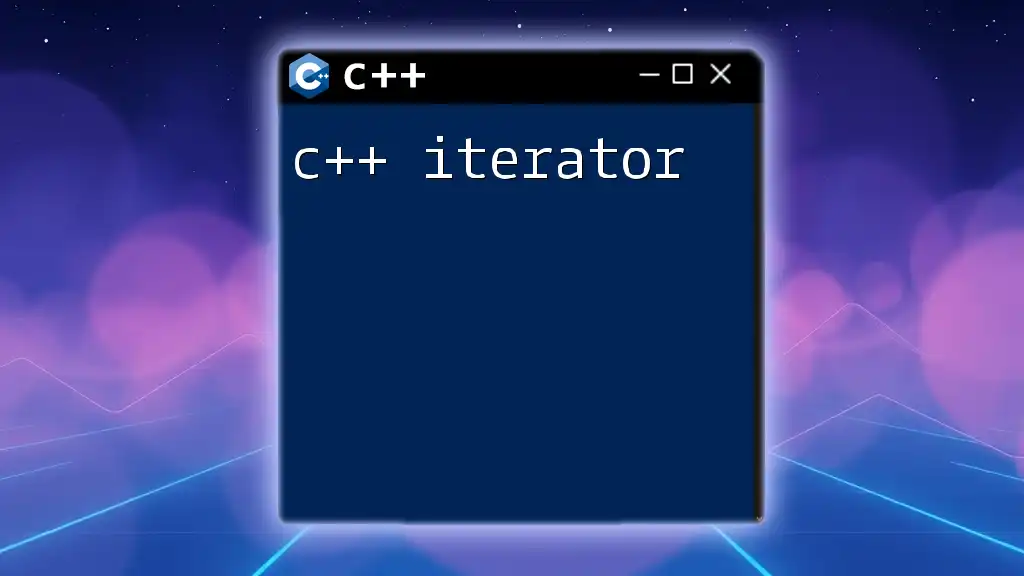
Advanced Usage
Modifying Elements Using `reverse_iterator`
`reverse_iterator` isn’t just for reading — it can also be used for modifying elements within the container. Consider the following example where we double each element:
for (auto rit = vec.rbegin(); rit != vec.rend(); ++rit) {
*rit *= 2; // Duplicates the value
}
After running this code, the original vector `vec` will change from `{1, 2, 3, 4, 5}` to `{2, 4, 6, 8, 10}`. This showcases how `reverse_iterator` can effectively modify container elements within a reverse traversal.
Using `reverse_iterator` with Other STL Containers
The versatility of `reverse_iterator` extends beyond vectors. Here’s how you can apply it to a `list`:
std::list<int> myList = {10, 20, 30};
for (auto rit = myList.rbegin(); rit != myList.rend(); ++rit) {
std::cout << *rit << " ";
}
Running the above code will output:
30 20 10
This demonstrates that `reverse_iterator` can be efficiently used with various STL containers, maintaining consistency in your codebase.
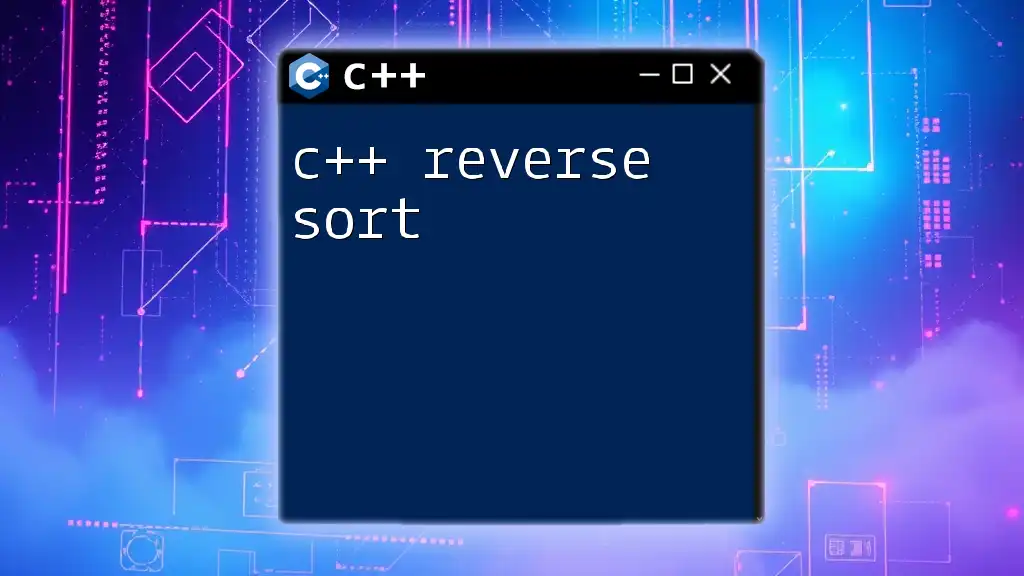
Performance Considerations
Efficiency of `reverse_iterator`
In general, `reverse_iterator` provides the same efficiency as standard iterators since it primarily relies on existing operations. However, when working with complex data structures, you may encounter situations where the inefficiency could arise from how the STL implements that specific container.
Understanding Time Complexity
The time complexity when traversing a container with `reverse_iterator` is `O(n)` for `n` elements, the same as forward iteration. However, the absence of additional data movements or operations makes it efficient for most applications.
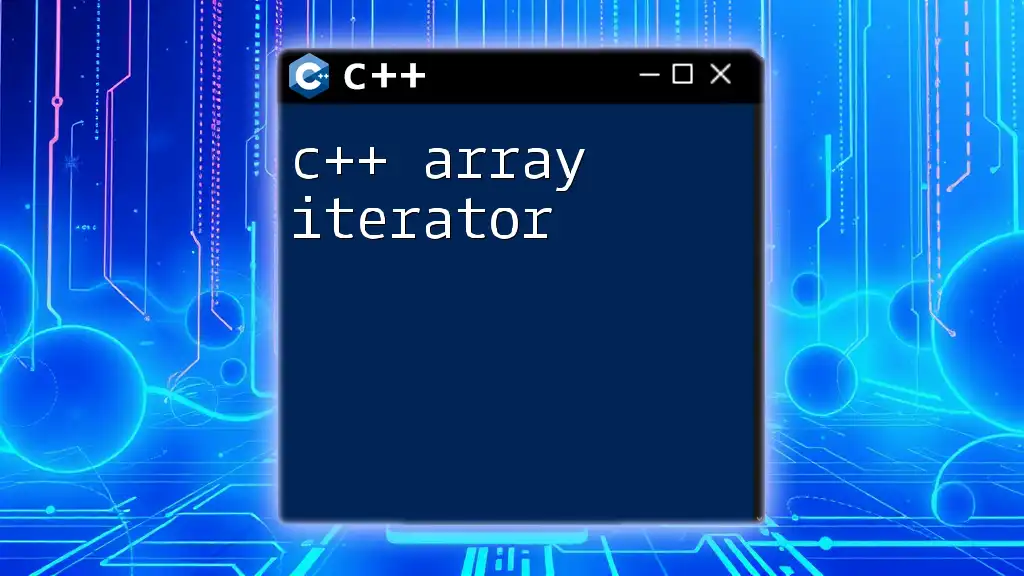
Common Pitfalls and Best Practices
Avoiding Segmentation Faults
Using `reverse_iterator` incorrectly, such as incrementing past `rend()`, can lead to undefined behavior. Always ensure that your loops are properly bound to prevent segmentation faults.
Best Practices for Effective Use
- Readability: Use descriptive variable names for your iterators. `rit` might be too cryptic; consider using `reverseIter` instead.
- Combine with Algorithms: Explore functions and algorithms that may allow you to work with reverse iterators effortlessly, such as `std::reverse`, to help keep your code clean.
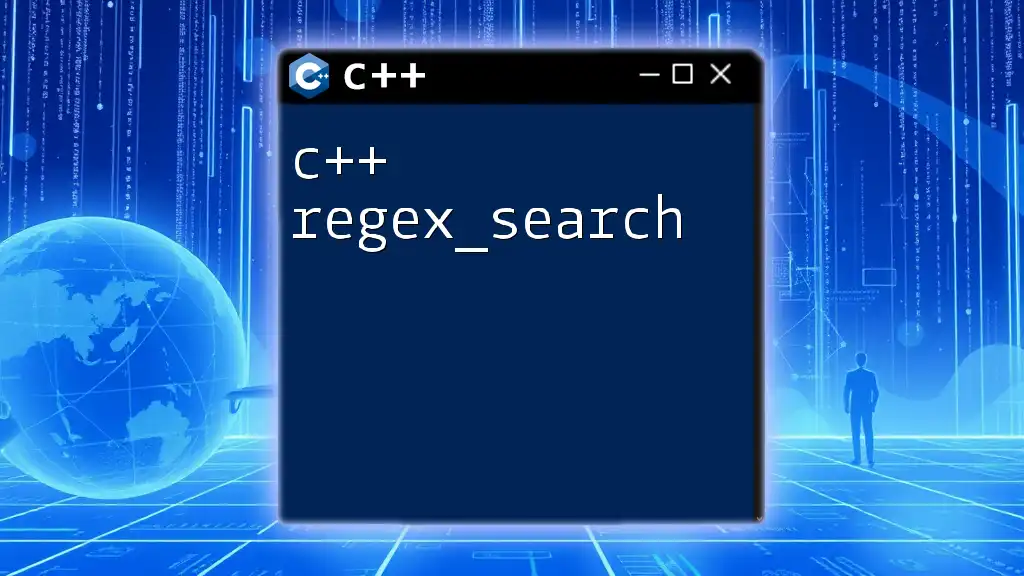
Conclusion
In summary, the C++ `reverse_iterator` is a powerful tool that allows developers to traverse containers in reverse order easily. By understanding its syntax, characteristics, and advanced usage, you can effectively implement it in various scenarios. Mastering `reverse_iterator` will enhance your coding efficiency and versatility.
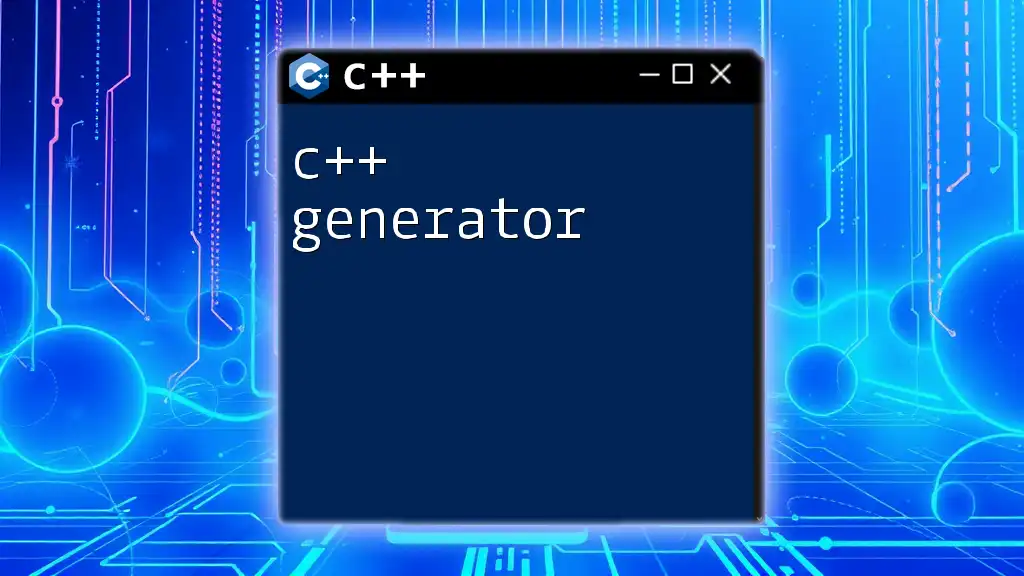
Further Reading and Resources
For deeper insights into `reverse_iterator`, consider exploring various programming books focused on C++, as well as online courses. Additionally, the official C++ documentation provides critical information that can guide you through advanced use cases and best practices while using iterators.
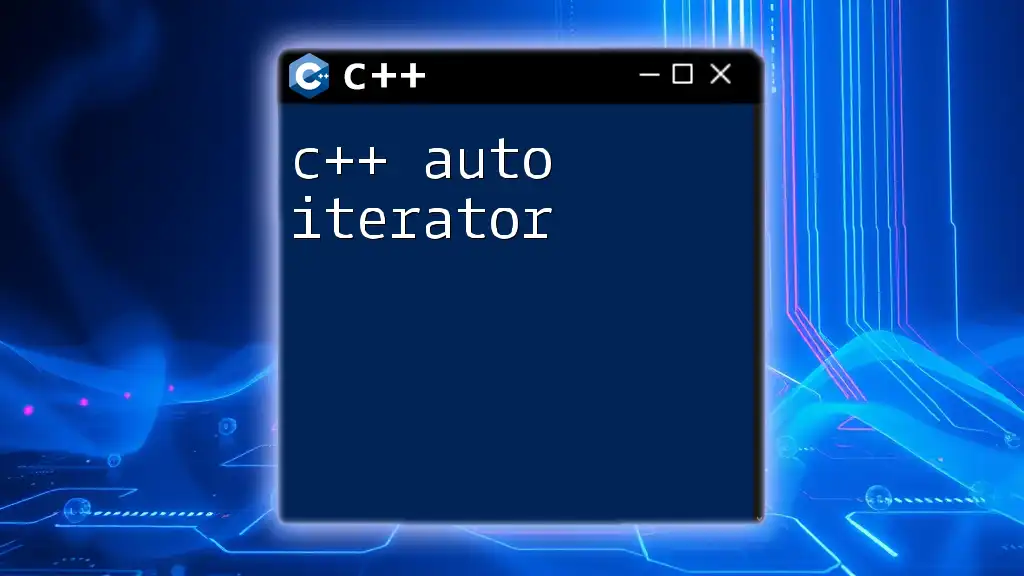
Interactive Example Section
Explore hands-on coding challenges centered on `reverse_iterator` using online coding platforms. Such interactive exercises will allow you to reinforce your understanding and share your solutions with others for collaborative learning.