In C++, you can reverse sort a collection using the `std::sort` algorithm along with the `std::greater` comparator from the `<algorithm>` header. Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 5, 6};
std::sort(numbers.begin(), numbers.end(), std::greater<int>());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Sorting
Sorting is a fundamental concept in programming, involving the arrangement of data in a specified order. Whether you're managing user data, manipulating large datasets, or implementing algorithms, the ability to sort data efficiently is crucial. Sorting helps improve the performance of certain algorithms, enables better organization, and enhances data retrieval processes.
When using C++, programmers benefit from built-in functions designed to simplify sorting. Understanding how these functions operate allows for more effective coding practices.
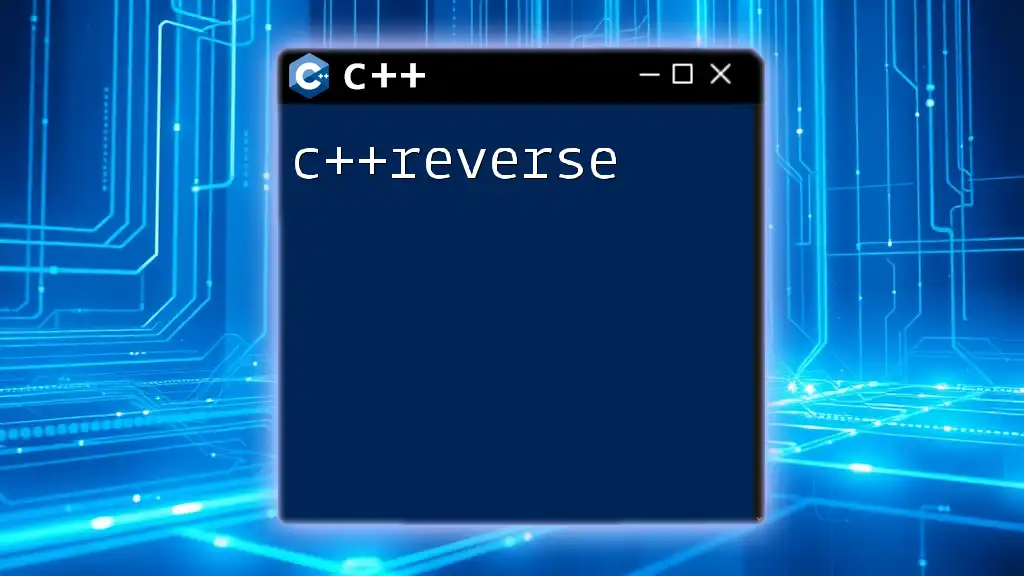
What is Reverse Sorting?
Reverse sorting, or sorting in descending order, is the process of arranging data from the highest value to the lowest, opposite to the standard ascending order. It is particularly useful in scenarios where you want the largest values prioritized, such as ranking scores, organizing financial data, or display preferences.
To understand reverse sorting, it's essential to differentiate it from standard sorting. While the conventional sorting orders elements from smallest to largest, reverse sorting takes the largest elements first.
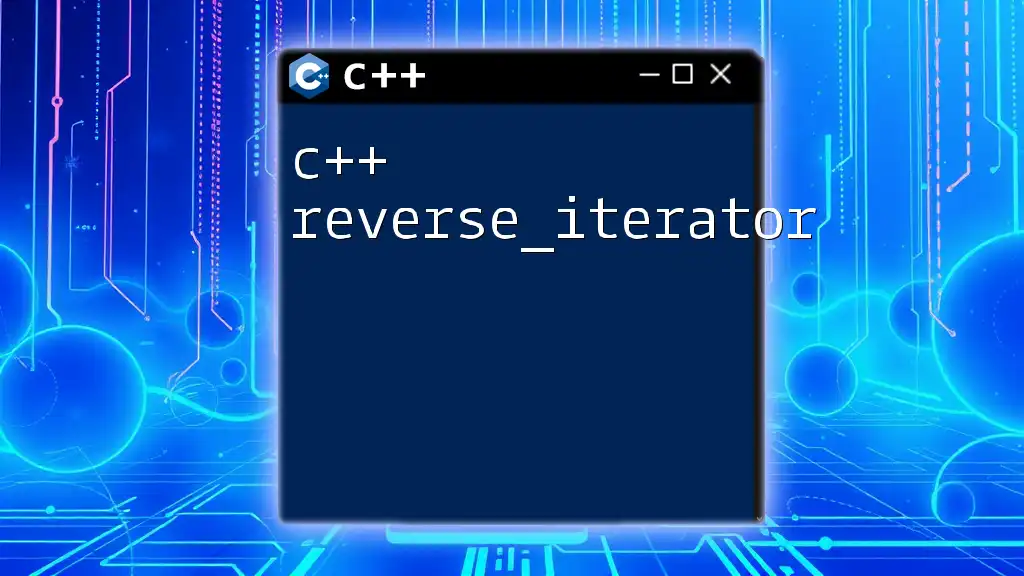
Using `std::sort` for Sorting in C++
The C++ Standard Library includes the `std::sort` function, which is highly regarded for its efficiency and simplicity when sorting collections of data. It provides a versatile way to sort data structures such as arrays and vectors.
Syntax of `std::sort`
The basic syntax of `std::sort` is as follows:
std::sort(start_iterator, end_iterator);
This function requires two iterators: the starting position and the ending position of the collection.
Example: Basic Sorting
To illustrate the use of `std::sort`, consider this example that demonstrates sorting a vector of integers in ascending order:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {4, 1, 3, 9, 5};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In the example, we initialize a vector with unsorted integers. The `std::sort` function arranges these integers in ascending order before displaying them.
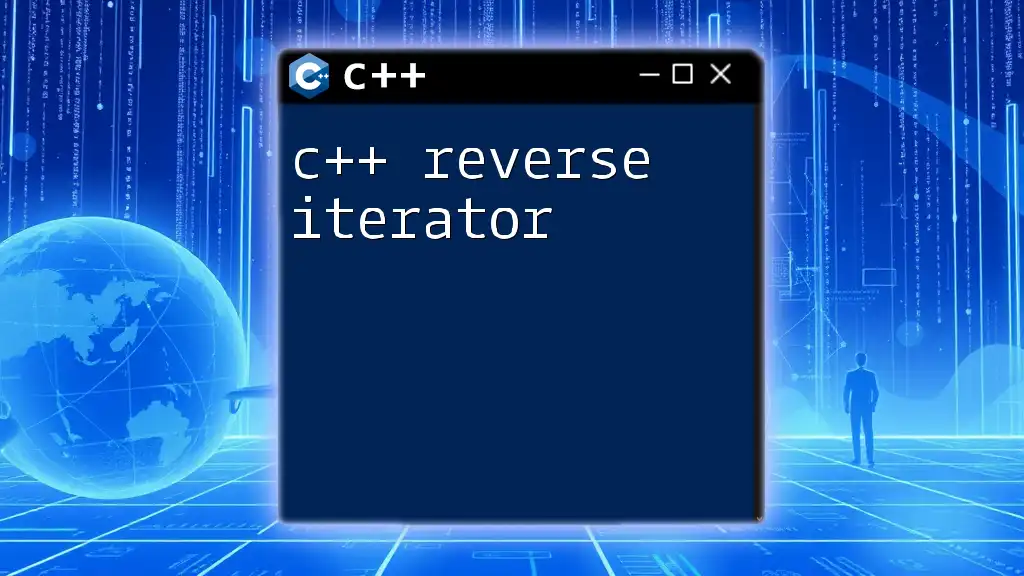
Implementing Reverse Sort in C++
To sort data in descending order in C++, we can leverage the `std::greater` function from the `<functional>` header. This built-in function serves as a predefined comparator ideal for reverse sorting.
Syntax and Practical Use
To implement a reverse sort using `std::greater`, the syntax remains similar, but we need to supply the comparator as an additional argument:
#include <iostream>
#include <algorithm>
#include <vector>
#include <functional> // Required for std::greater
int main() {
std::vector<int> numbers = {4, 1, 3, 9, 5};
std::sort(numbers.begin(), numbers.end(), std::greater<int>());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this code snippet, we include the `<functional>` library and apply `std::greater<int>()` as the third argument in `std::sort`. This effectively sorts the vector `numbers` in descending order, outputting `9 5 4 3 1`.
Explanation of Code
Each line of the code serves a specific purpose. The `std::greater<int>()` comparator tells the sort function to arrange the integers in descending order instead of the default ascending order. This flexibility allows programmers to tailor the sorting behavior to their specific use cases.
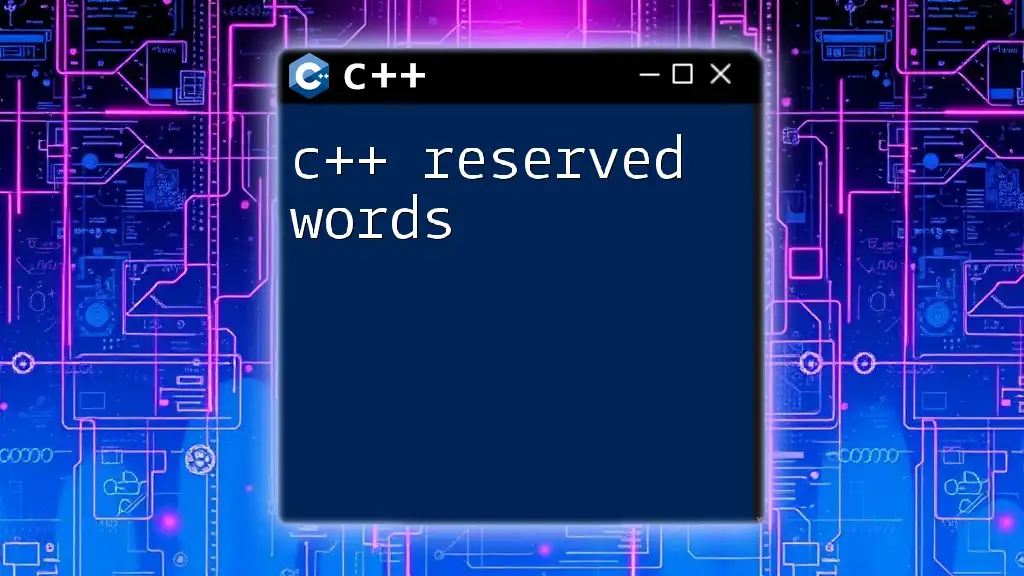
Custom Comparison Functions
In scenarios where predefined functions do not meet specific requirements, you can create custom comparison functions. These functions offer enhanced functionality and flexibility.
Creating Custom Comparison Functions
To implement your unique sorting logic, define a comparison function that returns `true` if the first parameter should precede the second. Here’s an example:
bool customComparator(int a, int b) {
return a > b; // For descending order
}
int main() {
std::vector<int> numbers = {4, 1, 3, 9, 5};
std::sort(numbers.begin(), numbers.end(), customComparator);
// Output logic...
}
In this snippet, `customComparator` is defined to implement a descending order by returning `true` when `a` is greater than `b`. This function can be used similarly to `std::greater`.
Explaining the Custom Function
The custom comparison function grants programmers more control over how the data is sorted, thus allowing sorting criteria specific to their application.
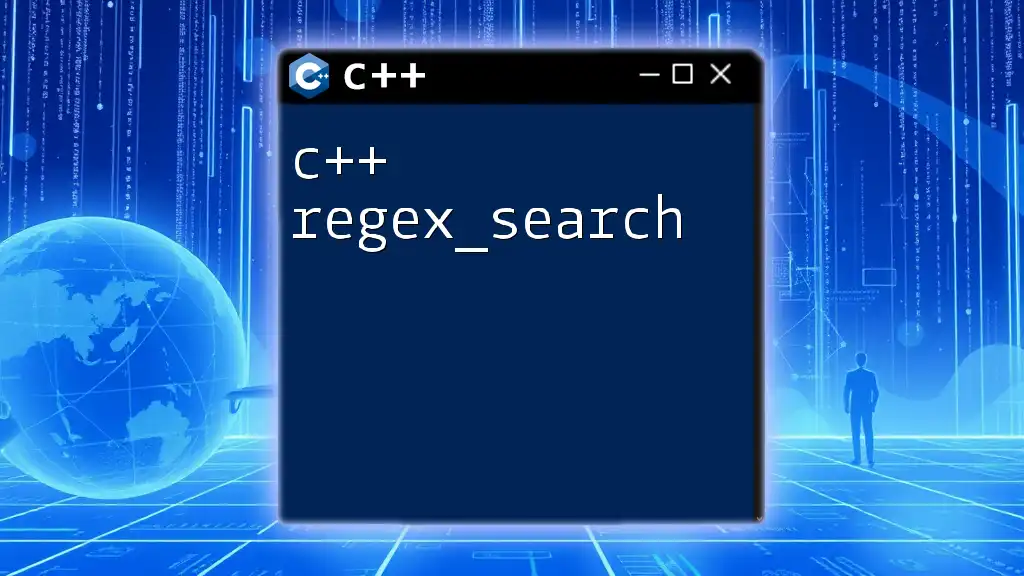
Working with Other Data Types
C++ is a versatile language that enables you to sort various data types, including strings, structures, and user-defined types. Sorting non-integer types doesn't fundamentally change the approach, but you must consider the characteristics of these data types.
Sorting Strings in Reverse Order
For example, reverse sorting a vector of strings follows the same principle. Here’s how you can do it:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<std::string> names = {"Alice", "Bob", "Charlie", "David"};
std::sort(names.begin(), names.end(), std::greater<std::string>());
for(const std::string& name : names) {
std::cout << name << " ";
}
return 0;
}
This code sorts the `names` vector in descending alphabetical order, producing the output: `David Charlie Bob Alice`.
Considerations for Other Types
When reverse sorting other types, it’s vital to ensure that the comparison logic respects the data's nature. For instance, when sorting custom objects, you might need to define how to access the properties that will be sorted.
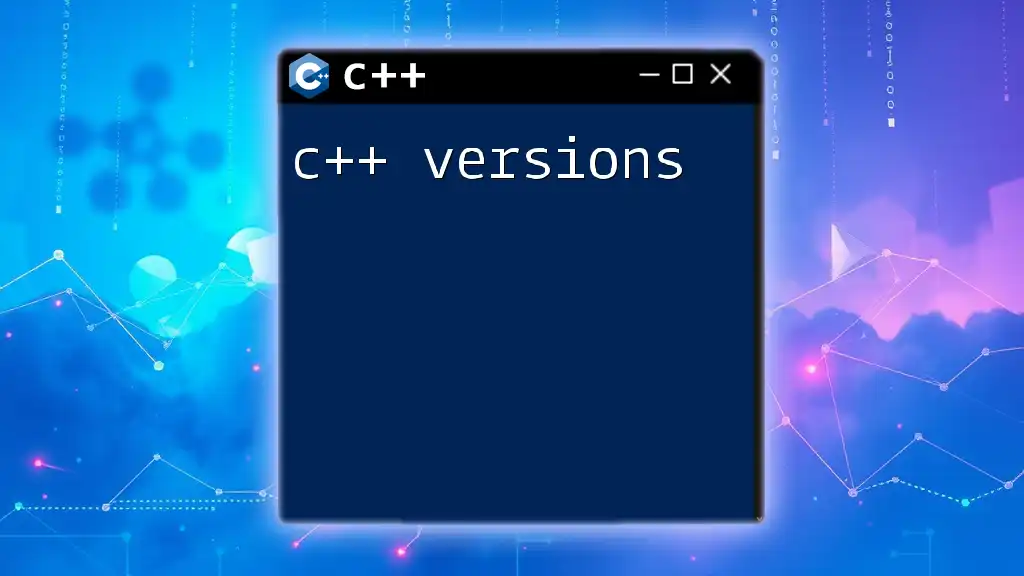
Challenges in Reverse Sorting
While reverse sorting can be straightforward, programmers may face common challenges. Some of these include:
- Incorrect Comparator Logic: Misunderstanding how the comparator functions may lead to unexpected sort orders.
- Performance Issues: Sorting large datasets may impose efficiency concerns, particularly if a naive approach is used.
Best Practices
To avoid potential issues, consider the following best practices:
- Validate Comparison Functions: Ensure that your comparison functions return consistent outcomes.
- Benchmark Performance: For large datasets, test the performance of different sorting implementations.
- Read Documentation: Familiarize yourself with library functions and their capabilities.
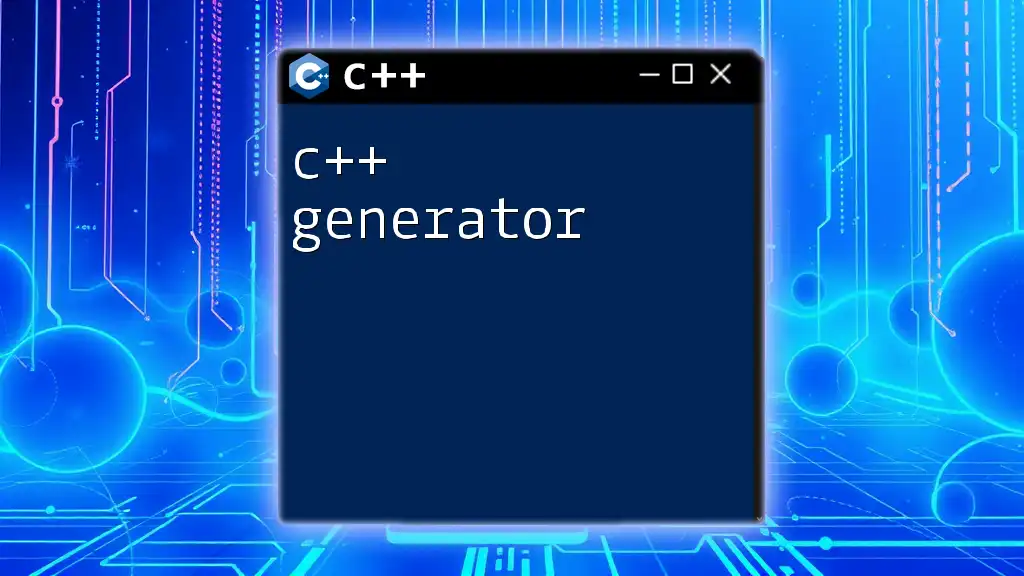
Conclusion
In summary, mastering `C++ reverse sort` enhances your ability to manage complex data structures efficiently. From utilizing `std::sort` and built-in comparators to crafting custom comparison functions, C++ provides a comprehensive framework for sorting. Embracing these techniques will empower you to manipulate data effectively in your programming endeavors.
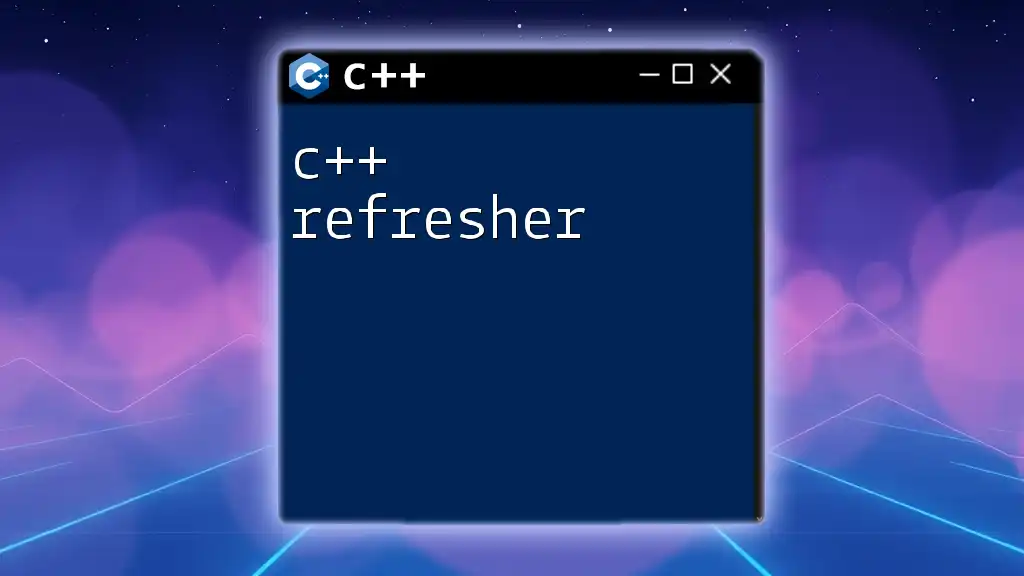
Additional Resources
To further your understanding of C++ and sorting techniques, it's beneficial to explore recommended learning materials. Look for online courses, books, and tutorials that delve deeper into data manipulation in C++. Additionally, consider branching out to related topics, such as algorithm optimization and data structures, to broaden your skill set.