A C++ developer is a programmer proficient in utilizing the C++ programming language to create efficient software applications, leveraging its features like object-oriented programming and low-level memory manipulation.
Here's a simple code snippet illustrating a basic class definition in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Understanding the Role of a C++ Developer
Overview of a C++ Developer's Responsibilities
A C++ Developer plays a crucial role in the software development lifecycle by crafting and optimizing code for various applications. This role is multifaceted, involving tasks such as code development, debugging, and collaboration within teams.
Code Development
At the heart of a C++ Developer's responsibilities is writing and optimizing C++ code. This includes developing software that can range from games and graphics engines to complex systems and embedded software. Developers use their knowledge to implement features effectively and ensure the software runs smoothly.
Debugging and Troubleshooting
Debugging is another critical component of a C++ Developer's job. They must be adept at identifying coding errors or bugs in their applications and fixing them efficiently. With tools like gdb (GNU Debugger), they can perform step-by-step execution of their code to analyze and diagnose issues.
Collaboration
Collaboration is essential within development teams, as C++ Developers work with cross-functional groups, including designers, testers, and project managers. Effective communication helps ensure that all team members are aligned on project goals, timelines, and deliverables.
Essential Skills for a C++ Developer
To excel as a C++ Developer, one must master several essential skills:
Proficiency in C++
A deep understanding of C++ is fundamental. Developers should be familiar with its syntax, control structures, and standard libraries. Mastery of C++ allows them to write efficient code and leverage its powerful features.
Knowledge of Object-Oriented Programming (OOP)
C++ is an object-oriented programming language, which means that familiarity with OOP principles is vital. Key concepts include:
- Classes: Blueprints for creating objects.
- Inheritance: Mechanism to create new classes based on existing ones.
- Encapsulation: Hiding the internal state of an object and requiring all interaction to be performed through methods.
Algorithms and Data Structures
A proficient C++ Developer needs to understand common algorithms and data structures, as they significantly impact code performance. Knowledge of how to implement and optimize these structures will enhance the efficiency and speed of software applications.
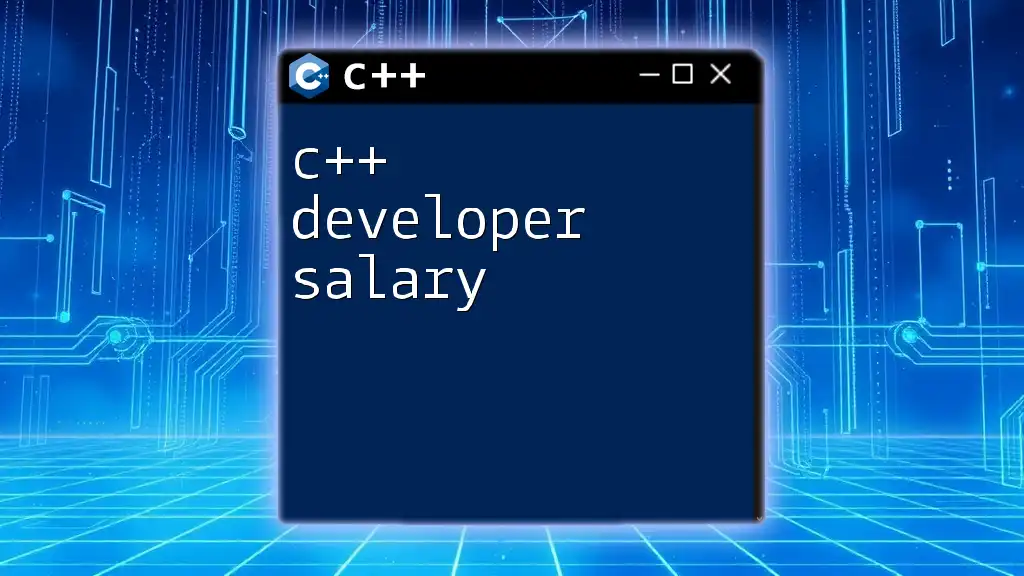
Getting Started with C++
Setting Up Your Development Environment
Choosing the Right IDE
When beginning as a C++ Developer, selecting an Integrated Development Environment (IDE) is crucial. Popular options include:
- Visual Studio: Comprehensive features for writing, debugging, and compiling C++ code.
- Code::Blocks: Lightweight and customizable IDE that's great for beginners.
- Eclipse: An open-source IDE with extensive plugins for C++ development.
Each IDE offers unique features such as code auto-completion and debugging tools that streamline the coding process.
Installing a C++ Compiler
A C++ compiler is necessary for compiling your written code into executable programs. Common compilers include GCC (GNU Compiler Collection) and Clang. Installation varies by operating system, but typically involves downloading a package and following installation instructions.
Your First C++ Program
Embarking on your journey as a C++ Developer typically starts with writing a simple "Hello World" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the Code:
- `#include <iostream>` includes the input-output stream library.
- `int main()` defines the entry point of the program.
- `std::cout` outputs text to the console.
This basic program introduces C++ syntax and structure, laying a foundation for more complex coding.
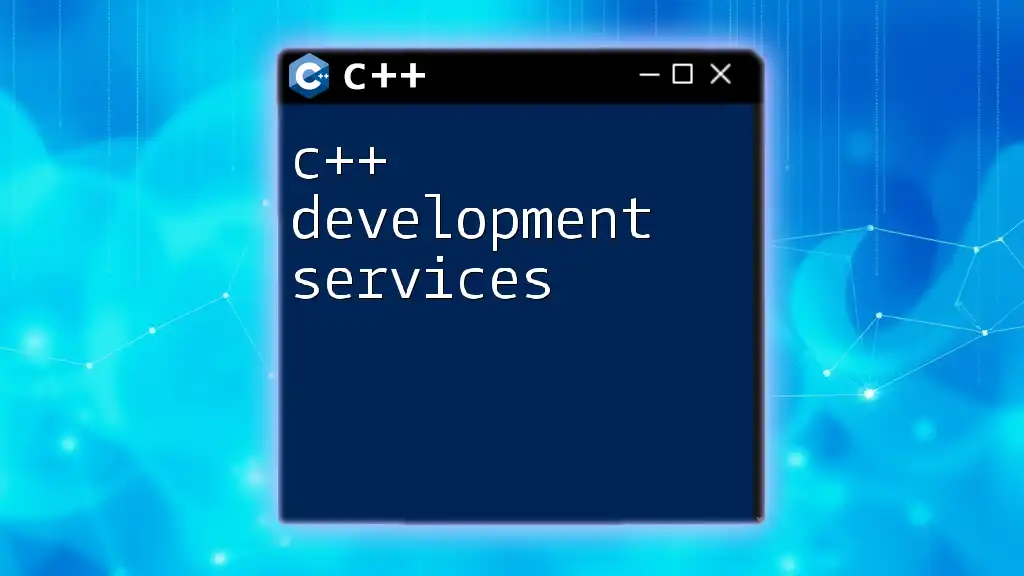
Core Concepts in C++
Understanding Variables and Data Types
Basics of Variables
In C++, a variable is a storage location identified by a name. There are several fundamental data types to understand:
- Integer (`int`): Represents whole numbers.
- Double (`double`): Represents numbers with decimal points.
- String (`std::string`): Represents text.
Example of declaring and initializing variables:
int age = 30;
double salary = 50000.99;
std::string name = "John Doe";
Scope and Lifetime
Variables have a scope and lifetime, determining where and how long they can be accessed. Local variables are defined within functions and are destroyed after the function exits, while global variables exist for the duration of the program.
Control Structures
Conditional Statements
Conditional statements allow developers to execute code based on whether an expression evaluates to true or false. For instance:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
This snippet checks if the variable `age` meets a certain condition and prints an appropriate message.
Loops
Loops facilitate repetitive actions within code. The `for` loop and `while` loop are common constructs that optimize tasks that require iteration:
for (int i = 0; i < 5; ++i) {
std::cout << i << std::endl;
}
This code prints numbers from 0 to 4, showcasing a simple iteration.
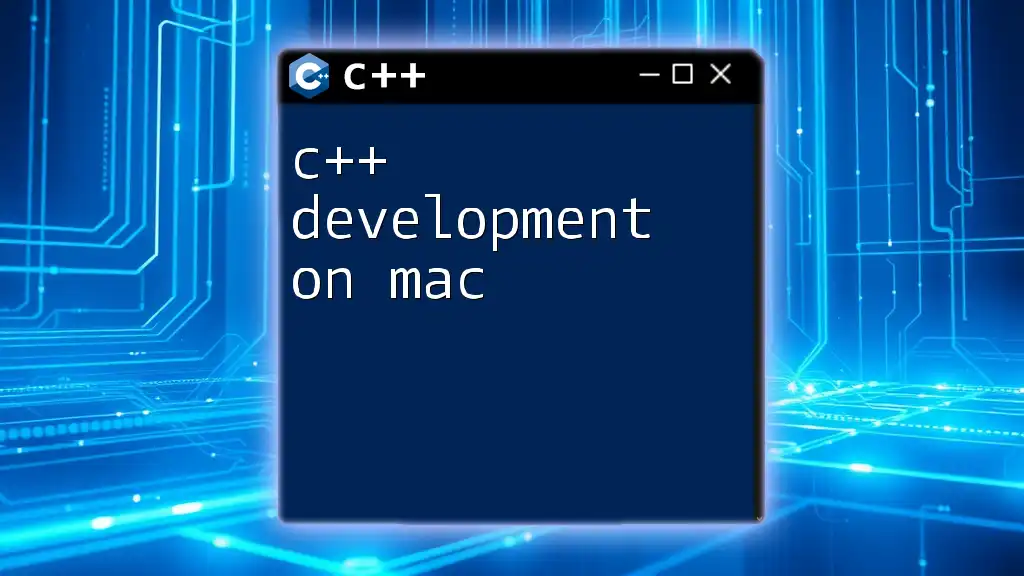
Advanced C++ Techniques
Object-Oriented Programming (OOP)
Classes and Objects
C++ is predominantly an object-oriented language. Classes serve as blueprints for creating objects. Here’s how to define a class:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep beep!" << std::endl;
}
};
In this example, the `Car` class contains a public member variable and a method to simulate a car horn.
Inheritance
Inheritance is a core principle of OOP allowing classes to acquire properties from other classes. Here’s an example of a derived class inheriting from a base class:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started" << std::endl;
}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Ring ring!" << std::endl;
}
};
In this snippet, `Bike` inherits from `Vehicle`, gaining access to its `start` method.
Understanding Pointers and Memory Management
Introduction to Pointers
Pointers are variables that store memory addresses. They are powerful tools in C++ for managing memory:
int value = 42;
int* pointer = &value;
std::cout << *pointer; // Outputs: 42
This example shows how to declare a pointer and dereference it to access the value it points to.
Dynamic Memory Allocation
C++ allows for dynamic memory management using `new` and `delete`. This functionality lets developers allocate memory during program runtime:
int* arr = new int[10]; // dynamically allocating an array
delete[] arr; // deallocating memory
Properly managing memory is crucial to prevent leaks that can slow down or crash applications.
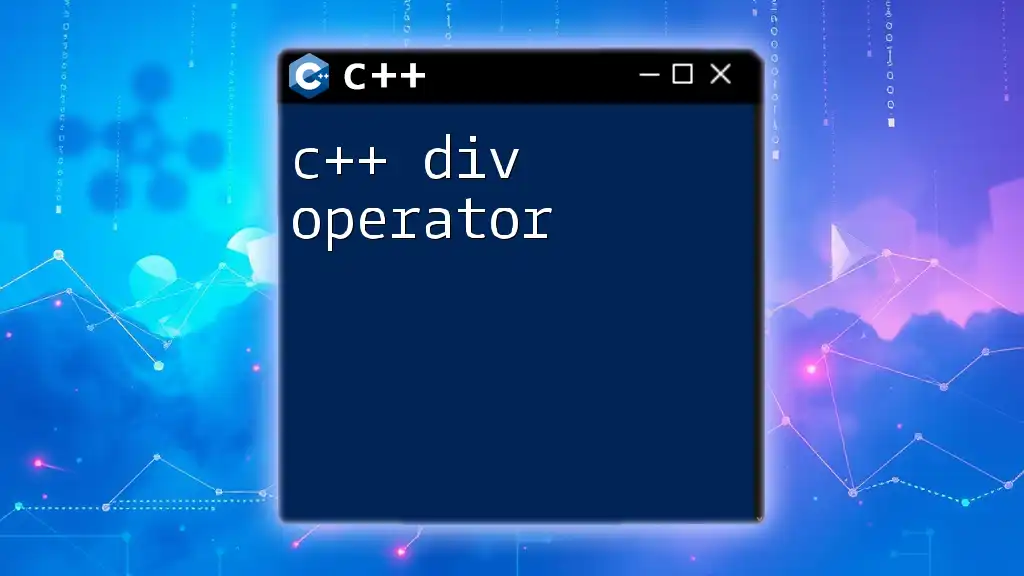
Best Practices for C++ Development
Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for any C++ Developer. Developers should focus on commenting and documenting their code effectively. Clear and concise comments can elucidate complex sections of code, making them easier to understand for both the original coder and future collaborators.
Performance Optimization Techniques
Optimizing performance is vital in C++ development. Using references instead of pointers in function parameters can increase efficiency by avoiding unnecessary copies of large data structures. Additionally, understanding how to avoid memory leaks, such as forgetting to deallocate memory, is vital for creating robust software.
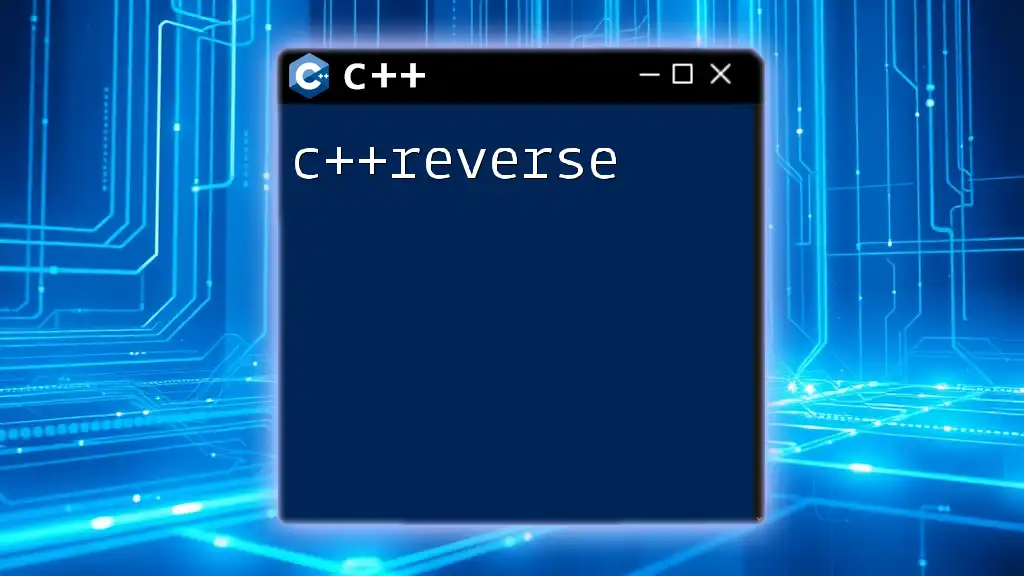
Career Paths in C++ Development
Job Opportunities
The demand for skilled C++ Developers spans various industries. Prominent sectors include gaming, finance, and embedded systems. Each of these sectors requires developers to leverage C++ for performance-critical applications.
Potential Roles
C++ Developers can pursue diverse roles, such as:
- Game Developer: Creating interactive gaming experiences.
- Systems Programmer: Developing low-level system software and tools.
- Software Engineer: Building applications across various domains.
Building Your Portfolio
Having a solid portfolio is key for aspiring C++ Developers. Engaging in personal projects, open-source contributions, or showcasing specific C++ applications can demonstrate skills effectively to potential employers.
Networking within C++ developer communities and forums can also provide insights and opportunities, helping developers stay updated with the latest trends and technologies in the field.
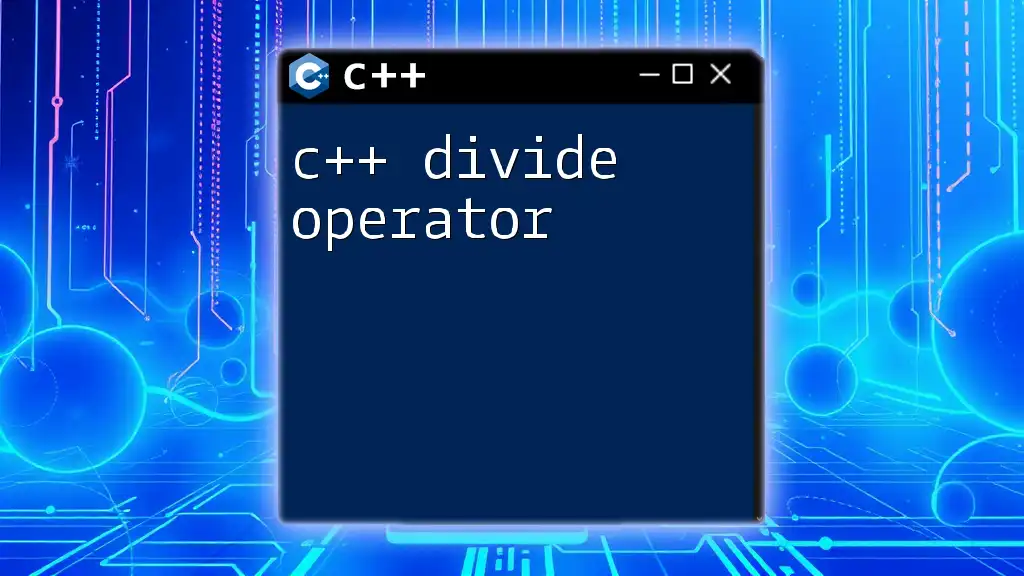
Conclusion
Summary of Key Takeaways
As a C++ Developer, mastering the language and its advanced features opens up numerous opportunities in the tech industry. It's critical to become proficient in core concepts, best practices, and effective memory management to excel in this field. Seeking continuous improvement through projects, learning resources, and community engagement will help developers thrive.
Further Resources
To further your understanding of C++, consider exploring books, online courses, and active forums that specialize in C++ programming. Engaging with these resources will develop your skills and prepare you for a successful career as a C++ Developer.