The C++ divide operator (/) is used to perform division between two operands, returning the quotient of the division.
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 2;
int result = a / b; // result will be 5
cout << "The result of dividing " << a << " by " << b << " is " << result << endl;
return 0;
}
Understanding the C++ Divide Operator
What is the Division Operator?
The C++ divide operator is a fundamental arithmetic operator used to perform division in C++ programming. Its primary role is to divide one number by another, returning the quotient of the two operands. The operator is represented by the symbol `/`. The syntax for using the divide operator is straightforward:
result = numerator / denominator;
In this statement, `numerator` is the number being divided, while `denominator` is the number you are dividing by.
Types of Division in C++
Integer Division
In C++, if both operands are integers, the division operation performs integer division. In integer division, the result is also an integer; any fractional part is truncated. This means that when you divide two integers, the result is the largest whole number less than or equal to the actual quotient.
For example:
int a = 7;
int b = 3;
int result = a / b; // result is 2
In this case, `7 / 3` results in `2`, not `2.33`, because the fractional part is discarded.
Floating-Point Division
If at least one of the operands is a floating-point number (i.e., either `float` or `double`), the division operation conducts floating-point division. This means the result will be a floating-point number, preserving any decimal components.
For instance:
double x = 7.0;
double y = 3.0;
double result = x / y; // result is 2.33333
In this example, `7.0 / 3.0` accurately gives you `2.33333`, demonstrating the precision that floating-point division offers.
How the Division Operator Works in C++
Basic Usage of the Division Operator
Utilizing the C++ divide operator is simple. Here’s a basic illustration:
int a = 20;
int b = 4;
int result = a / b; // result will be 5
In this code snippet, we are dividing `20` by `4`, which gives us a quotient of `5`.
Operator Precedence
C++ follows a specific order of operations (operator precedence) when evaluating expressions. The division operator has a higher precedence than many other arithmetic operators, such as addition (`+`) and subtraction (`-`). This means that divisions will be performed before additions or subtractions unless parentheses dictate otherwise.
For example:
int result = 10 + 20 / 5; // result is 14, not 16
In this case, `20 / 5` is evaluated first, giving `4`, and then `10 + 4` is computed, resulting in `14`.
Advanced Topics with the Division Operator
Division by Zero
One of the most critical aspects to consider when utilizing the C++ divide operator is how the program handles division by zero. Attempting to divide a number by zero leads to undefined behavior and can cause your program to crash.
It is essential to check for a zero denominator before performing any division:
if (b != 0) {
result = a / b;
} else {
// Handle division by zero error
std::cout << "Error: Cannot divide by zero." << std::endl;
}
By validating the denominator, you prevent potential runtime errors and enable your program to handle such situations gracefully.
Casting and Type Conversion
The result of the division operation in C++ can be affected by the data types of the operands being used. If both operands are integers, the result is also an integer. However, you can use casting to ensure that floating-point division takes place.
Here's how you achieve that:
int a = 5;
int b = 2;
double result = static_cast<double>(a) / b; // result is 2.5
In this instance, by casting `a` to `double`, we force the division operation to work with floating-point values, ensuring the division yields a result that includes the decimal component.
Common Mistakes with the Division Operator
Errors in Integer Division
A frequent pitfall when working with the C++ divide operator is misunderstanding how integer division behaves. As previously noted, integer division truncates the result, which may lead to unexpected outcomes if you're not prepared for it.
For example:
int a = 7;
int b = 2;
int result = a / b; // result is 3, not 3.5
The truncation of the decimal component can lead to significant logical errors in your program if not correctly accounted for.
Debugging Division Mistakes
When debugging division operations, consider adding comprehensive error-checking mechanisms and logging the values involved in calculations. This approach can help identify unexpected results resulting from improper handling of integer division or division by zero errors.
Best Practices for Using the Division Operator
Ensuring Precision in Calculations
To ensure precision in your calculations, be mindful of the data types you are using. When dealing with fractions or numbers needing precision, prefer floating-point types to store your results and perform the calculations.
Avoiding Division by Zero
Always validate that your denominator is not zero before performing a division. This practice will help maintain program stability and prevent errors in runtime execution. You can also create streamlined functions to handle division operations neatly:
double safeDivide(int numerator, int denominator) {
if (denominator == 0) {
throw std::invalid_argument("Denominator cannot be zero.");
}
return static_cast<double>(numerator) / denominator;
}
This function ensures that any calls to divide will safely handle zero denominators and provide a clear error if encountered.
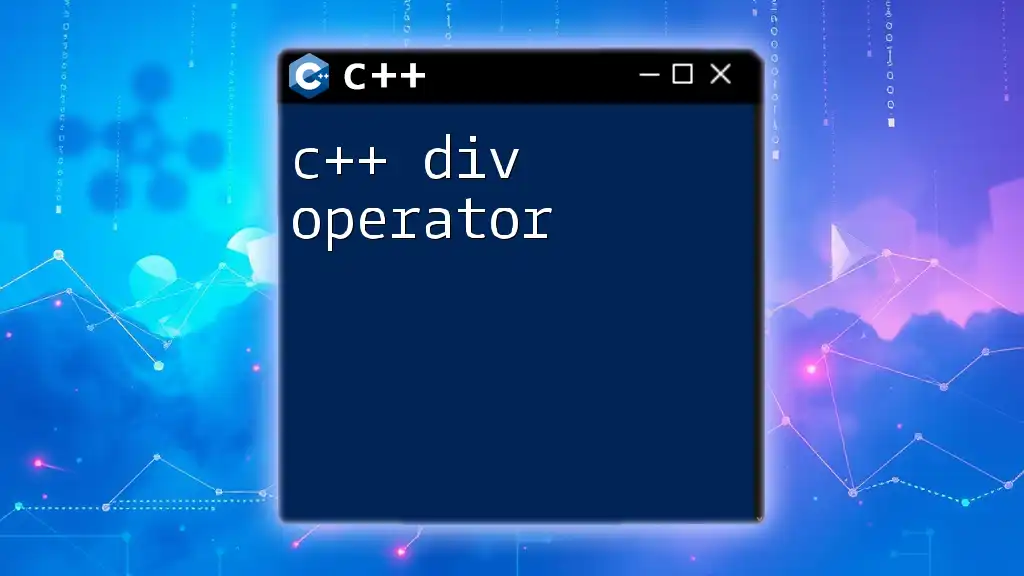
Conclusion
Understanding the C++ divide operator is essential for effective programming in C++. Whether working with integers or floating-point numbers, mastering how division operates will enhance your coding skills and lead to more reliable software development. Always remember to handle special cases, such as division by zero, and consider the implications of data types on your results. With these principles in mind, you'll be well-equipped to use the division operator effectively in your C++ projects.
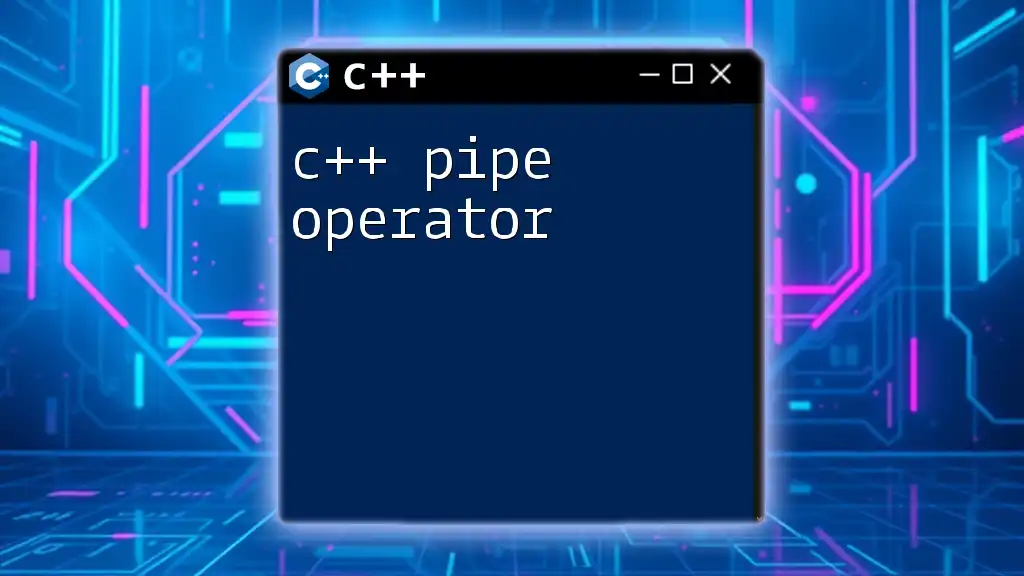
Additional Resources
For those looking to deepen their understanding, consider exploring comprehensive programming textbooks, online tutorials, and practice exercises focusing on arithmetic operations in C++. Expanding your coding knowledge will make you a more proficient and effective programmer.