The C++ `[]` operator is used for array subscript notation, allowing access to elements in an array or a vector by their index.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40};
std::cout << "The second element is: " << numbers[1] << std::endl; // Output: The second element is: 20
return 0;
}
What is the C++ [] Operator?
Definition
The C++ [] operator, commonly known as the subscript operator, is a powerful and versatile tool in the C++ programming language. Its primary function is to allow access to elements within arrays, vectors, and other data structures. This operator simplifies the way programmers retrieve and manipulate individual elements, making it essential for efficient coding.
Syntax of the C++ [] Operator
The syntax for using the [] operator is straightforward. When you want to access an element at a certain index of an array or a similar data structure, you enclose the index in square brackets.
Example Code Snippet:
int arr[5] = {0, 1, 2, 3, 4};
int element = arr[2]; // Accessing the third element
In this example, `arr[2]` accesses the third element of the array `arr`. Remember that indexing in C++ starts at 0, meaning the first element is accessed with `arr[0]`.
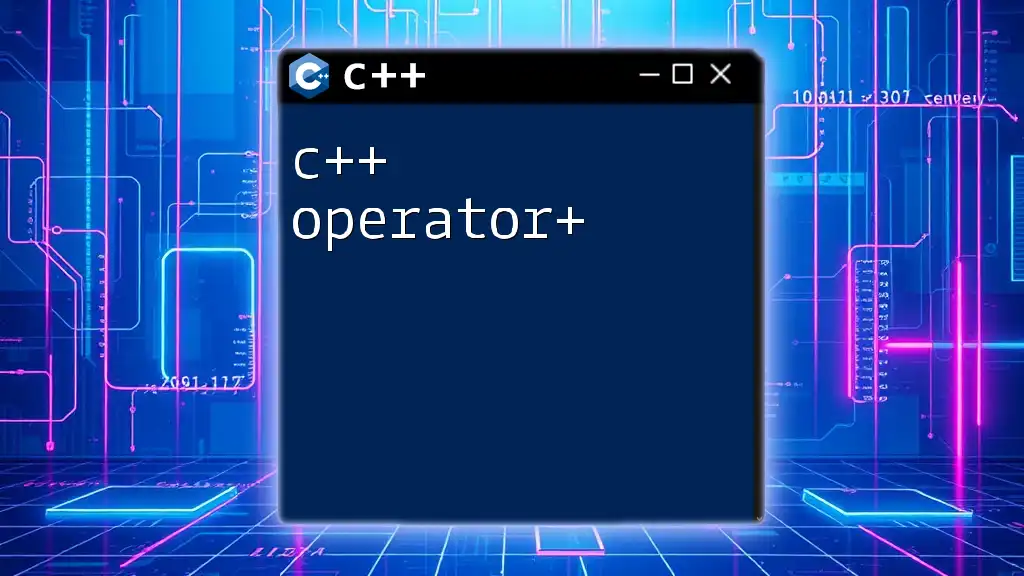
Using the [] Operator with Arrays
Understanding Array Access
Accessing elements via the [] operator in arrays is simple yet powerful. The operator allows you to read values stored at specific positions in memory.
Example with Illustrative Comments:
int numbers[4] = {10, 20, 30, 40};
for (int i = 0; i < 4; ++i) {
std::cout << numbers[i] << " "; // Outputs: 10 20 30 40
}
In this example, the loop iterates over the `numbers` array, outputting each value. The operator lets you access each element through its index, which is crucial for processing data efficiently.
Modifying Array Elements
The [] operator also enables you to modify existing elements. This flexibility allows for dynamic updates, which is beneficial for managing data structures.
Code Example:
numbers[1] = 25; // Changing the second element to 25
In the example above, the second element of the `numbers` array is updated to 25. The ability to alter array data is vital for applications that require real-time data processing.
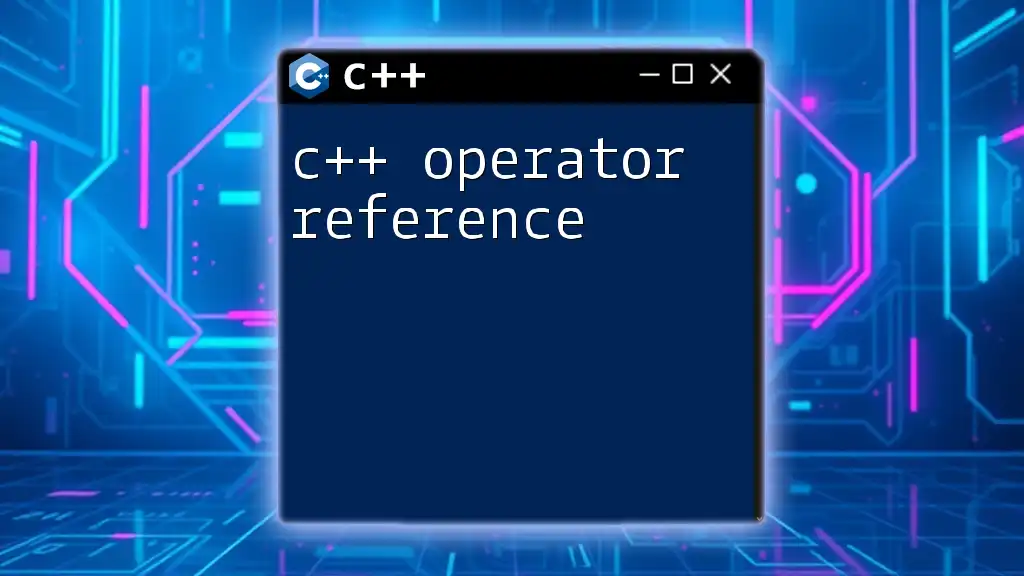
The C++ [] Operator with Other Data Structures
Using the [] Operator with Vectors
C++ Standard Template Library (STL) vectors provide dynamic array capabilities, and the [] operator integrates seamlessly with them.
Vector Access Example:
#include <vector>
#include <iostream>
std::vector<int> vec = {1, 2, 3, 4};
vec[1] = 5; // Changing the second element to 5
std::cout << vec[1]; // Outputs: 5
Here, the operator allows for the modification of elements in a vector just like it does for a traditional array. This feature is crucial for developers needing flexible and resizable data storage.
Using the [] Operator with Maps
The [] operator can also be used with the `std::map` container, allowing easy access to key-value pairs.
Example:
#include <map>
#include <string>
#include <iostream>
std::map<std::string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
std::cout << age["Alice"]; // Outputs: 30
In this case, accessing `age["Alice"]` retrieves the integer value associated with the key "Alice". The operator simplifies interactions with complex data structures such as maps.
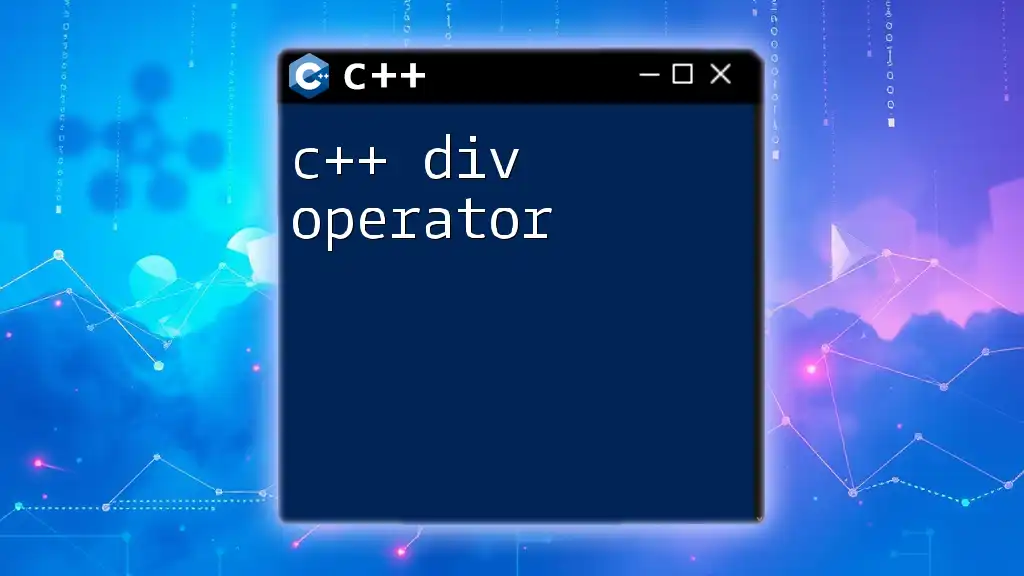
Operator Overloading: Custom Implementation of the [] Operator
What is Operator Overloading?
Operator overloading in C++ allows developers to extend the functionality of operators for custom classes. This enables programmers to define how operators like the [] operator behave with their own objects, making code more intuitive and readable.
Implementing the [] Operator in a Custom Class
To overload the [] operator in a custom class, you define a member function that specifies its behavior. This is essential for classes that mimic array-like behavior.
Code Example:
class MyArray {
private:
int arr[5];
public:
int& operator[](int index) {
return arr[index]; // Return reference to allow modification
}
};
In this example, the `MyArray` class defines how the [] operator behaves. It returns a reference to the array element, allowing for direct modifications.
Example Usage of the Custom Overloaded Operator
The overloaded operator can now be employed to access and manipulate elements conveniently.
Example:
MyArray myArr;
myArr[0] = 10; // Using the overloaded operator to set value
std::cout << myArr[0]; // Outputs: 10
This demonstrates that the operator behaves just like it does for built-in arrays, enhancing usability in custom data structures.
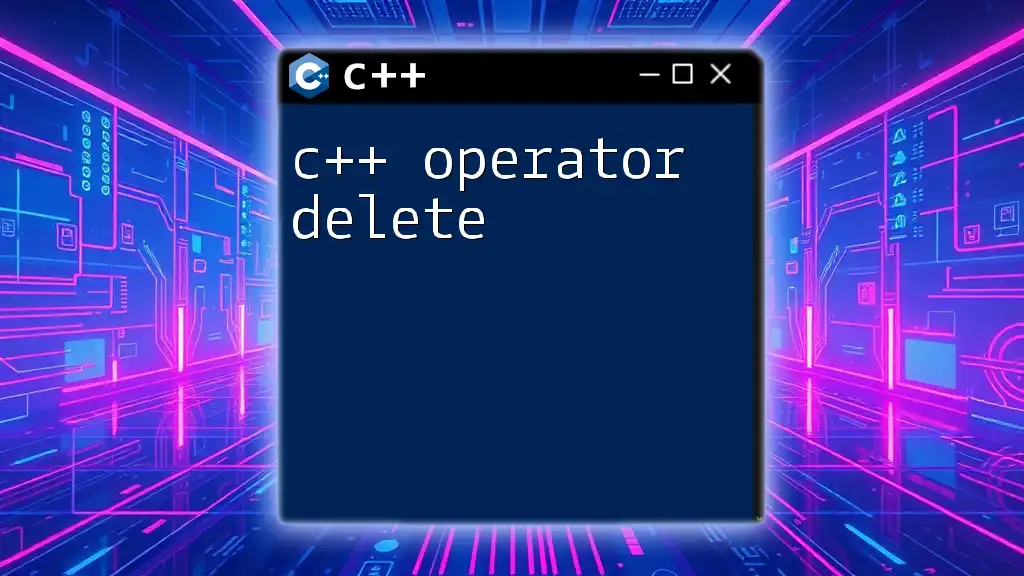
Safety Considerations with the [] Operator
Bounds Checking
While the [] operator is powerful, it does not inherently check for out-of-bounds indices, which can lead to undefined behavior. Implementing bounds checking is vital for creating robust applications.
Implementing Bounds Checking (Code Example):
int& MyArray::operator[](int index) {
if (index < 0 || index >= 5) {
throw std::out_of_range("Index out of bounds");
}
return arr[index];
}
In this implementation, an exception is thrown if an invalid index is accessed, enhancing the safety of your class and making debugging easier.
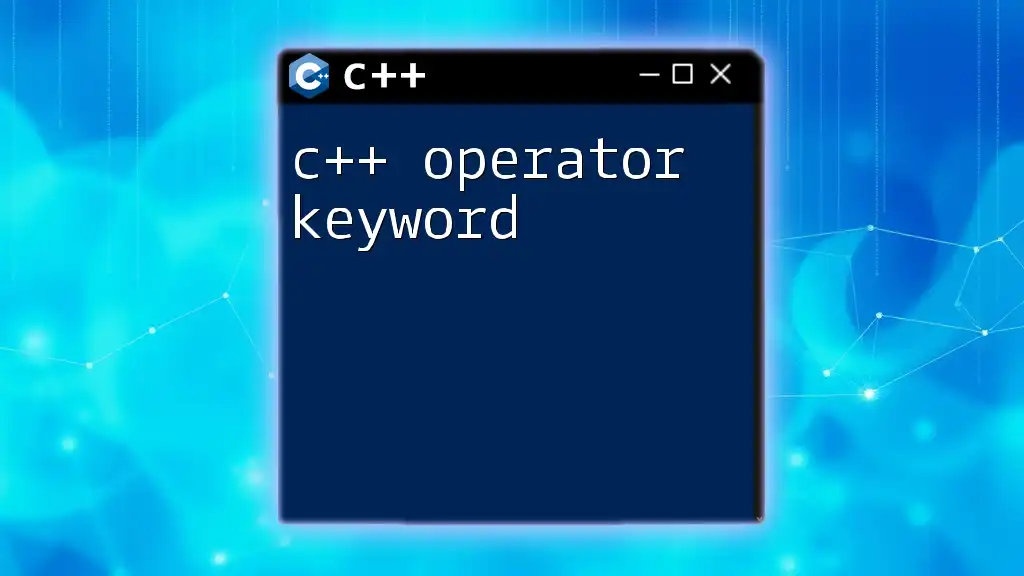
Conclusion
The C++ [] operator is an indispensable tool in the C++ programmer’s toolkit, facilitating efficient access and manipulation of data across various container types. From arrays to vectors and maps, the operator streamlines coding and elevates performance by allowing direct index-based access. Overloading this operator in custom classes further exemplifies its versatility, enabling developers to create more intuitive data structures. Understanding the intricacies of the [] operator not only enriches your programming skills but also leads to better and safer code in your C++ projects.
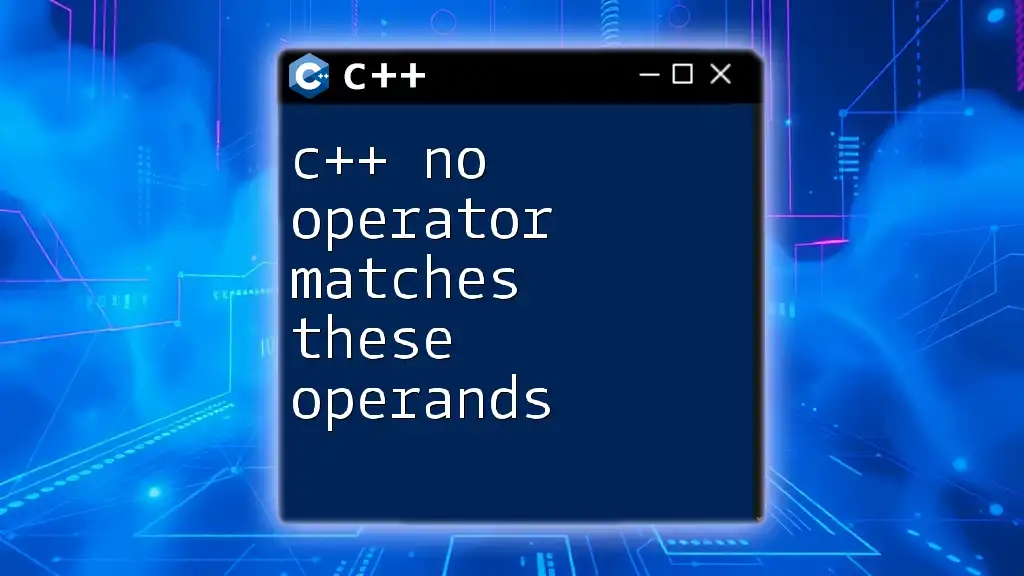
Additional Resources
For further reading and deepening your understanding of the C++ [] operator, consider exploring resources such as [cppreference.com](https://www.cppreference.com/) for detailed documentation and practice exercises to enhance your skills.