The `perror` function in C++ is used to print a description for the last error that occurred during a library function call, helping developers debug issues related to system calls.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
#include <cerrno>
int main() {
FILE *file = fopen("non_existing_file.txt", "r");
if (file == nullptr) {
perror("Error opening file");
}
return 0;
}
What is perror?
Definition and Purpose
The `perror` function is a standard C library function that provides a simple mechanism for error reporting in C++ programs. It prints a description of the last error that occurred during a library function call, which has set the global variable `errno`. Understanding `perror` is crucial since most C++ applications interact with the operating system, filesystem, or network, where errors are inevitable.
How perror fits into C++ error handling
In C++, several error handling methods exist, from exceptions to error codes. `perror` is specifically designed for use with system calls and standard library functions that do not throw exceptions. When used correctly, it can instantly provide users and developers insights into what went wrong, making it an indispensable tool in debugging.
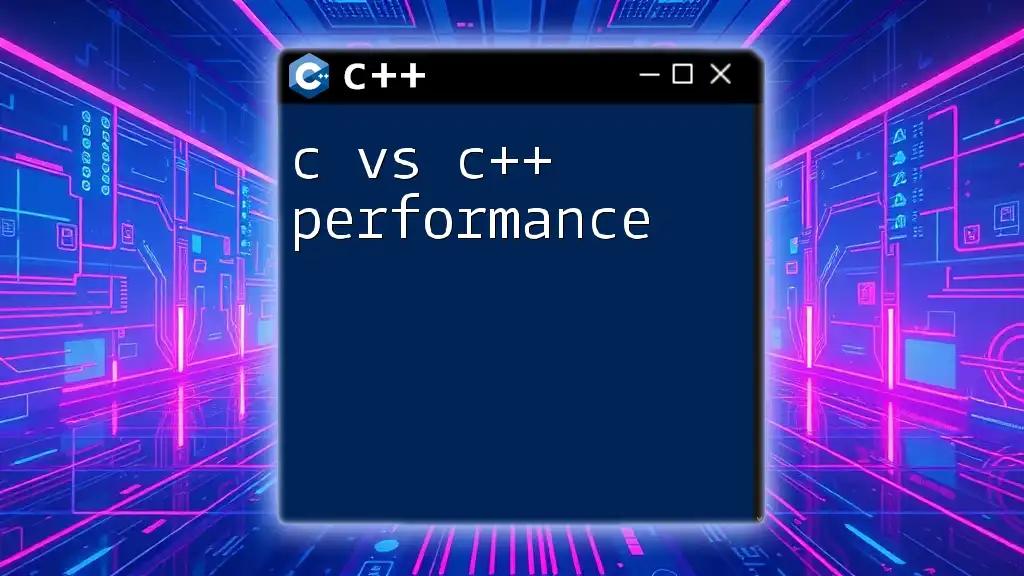
The Basics of perror in C++
Syntax of perror
The syntax for `perror` is as follows:
void perror(const char *s)
- The parameter `s` is a string that is prepended to the error message. This string can help clarify the context of the error for the developer using `perror`.
How perror works
When a function fails and sets `errno`, calling `perror` retrieves the associated error message from the system's error message database and outputs it to the standard error.
The `errno` variable is defined in `<cerrno>` and holds an error code corresponding to the last error encountered by system calls or library functions. Common error codes include `EINVAL`, `EIO`, `ENOMEM`, among others.
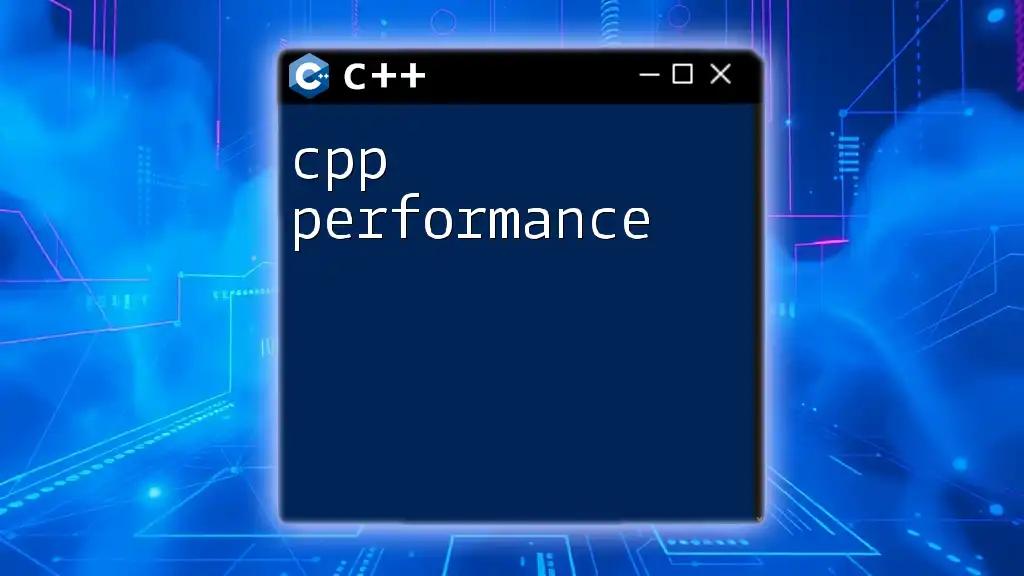
When to Use perror
Common Scenarios
`perror` is particularly useful in scenarios involving:
- File I/O Operations: Opening, reading, or writing to a file can fail due to permissions, non-existing files, or disk errors.
- Network Operations: Functions such as connecting to a socket or sending data might fail due to connectivity issues.
- Memory Allocation Failures: Dynamic memory allocation functions like `malloc` can fail, especially in low-memory conditions.
- System Calls: Calls like `fork()`, `exec()`, and `rmdir()` have inherent risks and can produce errors that require immediate attention.
Inappropriate Use Cases
While `perror` is useful, it may not always be the best choice, especially in contexts where exceptions are favored, as in standard C++ code. Using exceptions allows for cleaner separation of error handling logic and control flow and is more in line with modern C++ practices.
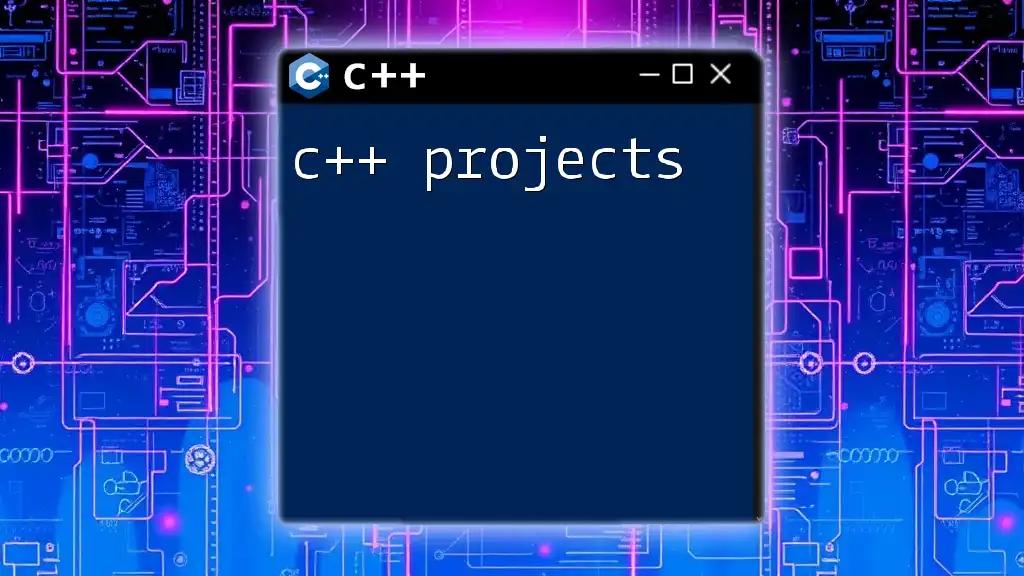
Using perror Effectively
Example Code Snippets
Basic Example
#include <iostream>
#include <cstdio>
#include <cerrno>
int main() {
FILE *file = fopen("nonexistent_file.txt", "r");
if (file == nullptr) {
perror("Error opening file");
}
return 0;
}
In this example, we attempt to open a file that does not exist. The output will look something like:
Error opening file: No such file or directory
Here, the message "No such file or directory" provides immediate insight into the issue.
Advanced Example with System Calls
#include <iostream>
#include <unistd.h>
#include <cerrno>
int main() {
if (rmdir("nonexistent_directory") != 0) {
perror("Error removing directory");
}
return 0;
}
In this situation, we attempt to remove a directory that is not present. `perror` will print a relevant error message, revealing why the operation failed.
Combining perror with errno
It is also beneficial to print the `errno` value before calling `perror` to understand the specific error code indicated.
#include <iostream>
#include <cstdio>
#include <cerrno>
int main() {
FILE *file = fopen("file.txt", "r");
if (file == nullptr) {
std::cerr << "Error number: " << errno << std::endl;
perror("Error opening file");
}
return 0;
}
By outputting both the error number and the descriptive string, you can gain deeper insights into the nature of the error and debug more effectively.
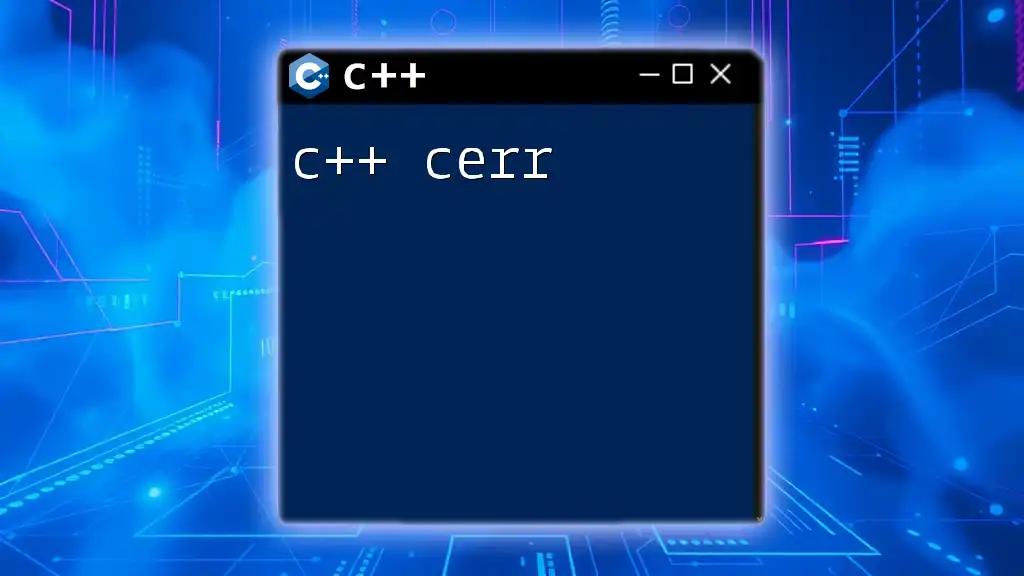
Limitations of perror
Key Limitations
One limitation of `perror` is that it may not provide adequate context for every error. While the error message itself is informative, it may lack specificity regarding the operations leading to the failure. Additionally, in certain locales (language settings), `perror` outputs error messages in the local language, which may not be universally understandable.
Alternatives to perror
If sophisticated error handling is necessary, or if you need to structure your error reporting more complexly, alternatives include:
- Using `std::cerr`: For more customized error messages.
- Creating custom error handling classes: This allows for advanced logging and error categorization instead of relying on `perror`.

Best Practices for Using perror in C++
Guidelines for Effective Error Handling
- Keep error messages informative but concise: Ensure that users understand the issue without overwhelming them with information.
- Use `perror` in tandem with appropriate logging mechanisms: Combining `perror` with logging aids in maintaining error records for future analysis.
- Always check `errno` before using `perror`: Since `errno` can be altered by numerous other function calls, always verify its value contextually relevant to the function of interest.
Error Message Standardization
Creating a consistent error reporting function is advisable for larger projects. Such a function could wrap calls to `perror` and standardize the output format, making it easier to maintain throughout the application.

Conclusion
In summary, `perror` serves as a vital tool in C++ error handling, particularly when dealing with system-level operations. Familiarizing yourself with this function can enhance your debugging skills significantly. By practicing different scenarios of `perror` and understanding its usage, you can make your applications more robust and user-friendly.

Additional Resources
For further reading, consider exploring:
- Error Handling in C++: Online documentation and tutorials.
- C++ Standard Library Functions: Comprehensive references on standard functions that use `errno`.
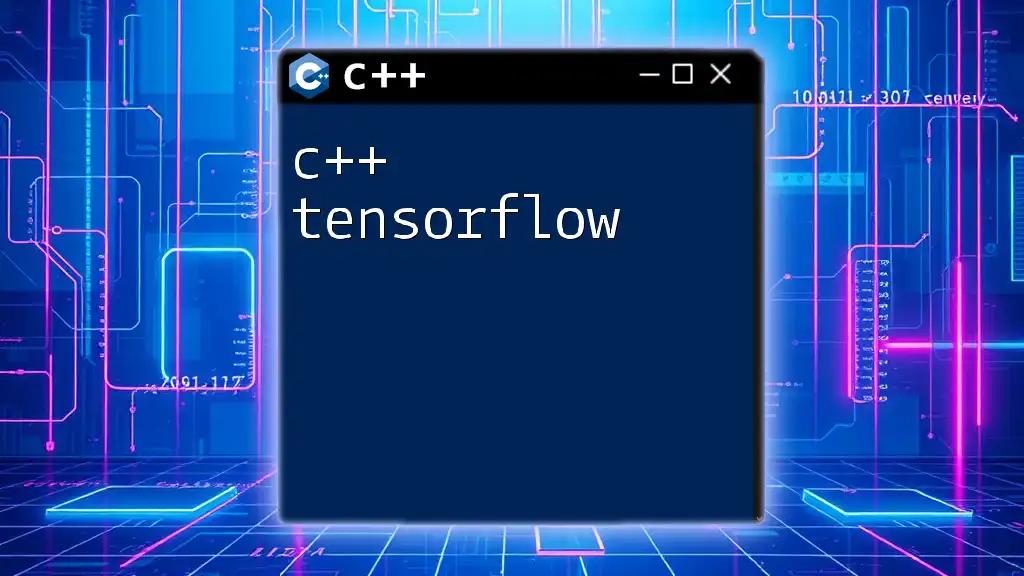
FAQs
What is the difference between perror and std::cerr?
While `perror` specifically deals with errors tied to system calls and library functions, `std::cerr` can be used for any kind of error reporting in C++. The former provides standardized error messages associated with `errno`, while the latter allows for a broader range of custom messages.
Can perror be used in multi-threaded applications?
Yes, you can use `perror` in multi-threaded applications, but it’s important to note that `errno` is thread-local in C++. Each thread maintains its own store of `errno`, making the usage of `perror` safe within threaded contexts.
How do I format the error messages returned by perror?
The messages returned by `perror` cannot be formatted because they are predefined system error strings. However, you can control what you prepend by passing your custom string as an argument, giving you flexibility in defining the context of the error.