In C++, `errno` is a global variable that is set by system calls and some library functions to indicate what error occurred during the operation, allowing developers to check for and handle errors effectively.
Here's a code snippet demonstrating the use of `errno`:
#include <iostream>
#include <errno.h>
#include <cstring>
#include <cstdlib>
int main() {
FILE *file = fopen("non_existing_file.txt", "r");
if (!file) {
std::cerr << "Error opening file: " << strerror(errno) << std::endl;
}
return 0;
}
What is `errno`?
The `errno` variable is a global integer variable defined in the `<cerrno>` header in C++. It is used to indicate the error number when a function fails. Understanding `errno` is crucial for properly handling errors in C++ programs. Many standard library functions set `errno` to a positive error code when they encounter an issue. It’s important to note that `errno` is not cleared automatically; thus, utilizing it effectively requires careful attention to the sequence of function calls.
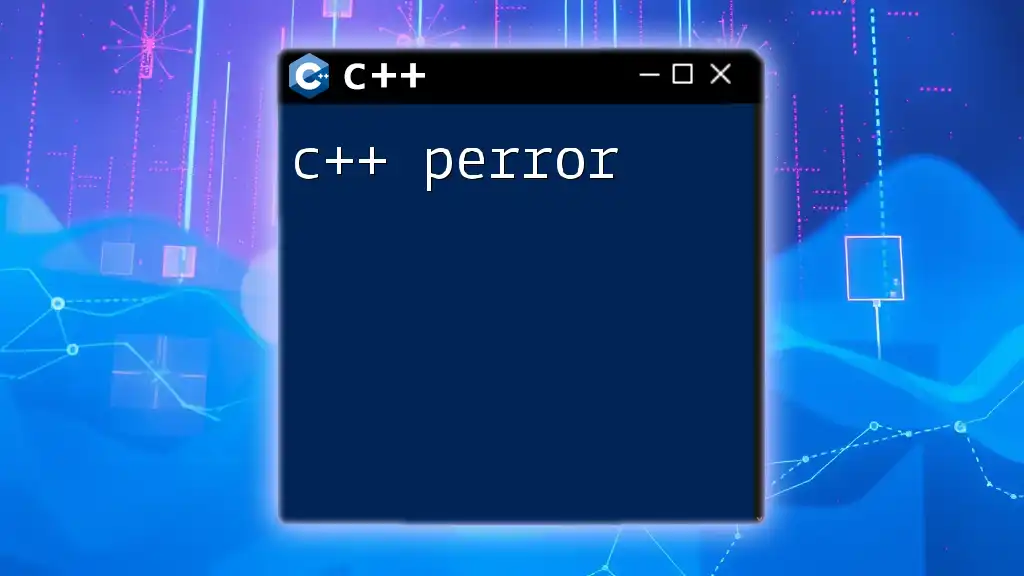
The `errno` Variable
Explanation of the `errno` Variable
`errno` is defined in such a way that its value gives insights on what computational failure occurred during the execution of a standard library function.
Thread Safety: One might wonder about `errno` in multi-threaded environments. In such cases, `errno` behaves as a non-thread-safe variable. This means that if multiple threads modify `errno` simultaneously, problems may arise. For thread-safe error indication, consider using thread-local storage, accessible using `thread_local`.
Understanding `errno` Values and Significance
The `<cerrno>` header file defines a set of macros that correspond to common error codes. Some of these include:
- `EINVAL`: Invalid argument
- `ENOMEM`: Out of memory
- `EFAULT`: Bad address
Each of these defined error codes helps developers quickly identify the specific issue that occurred.
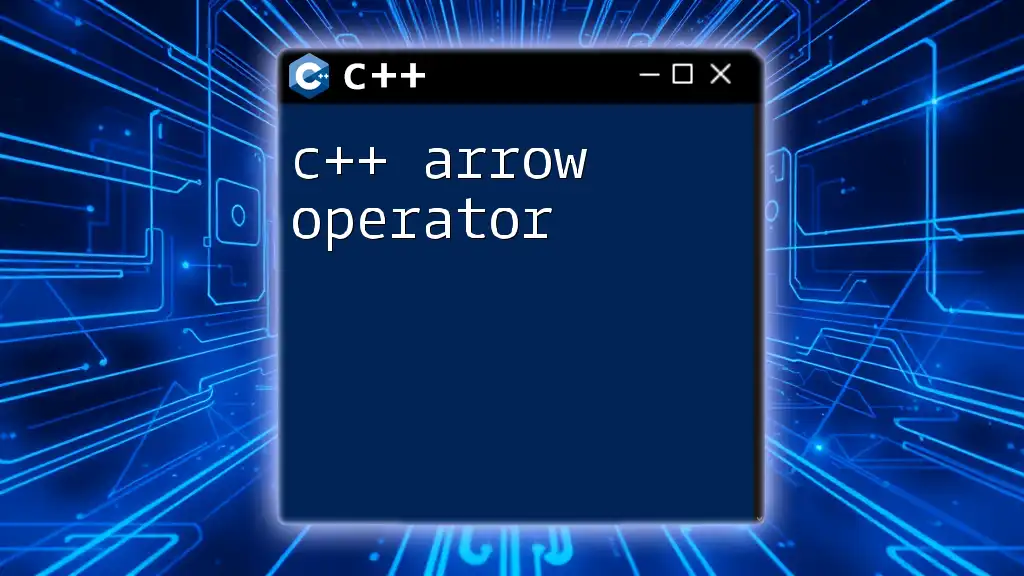
How to Use `errno` in C++
Setting and Checking `errno`
It is crucial to check the value of `errno` immediately after a function call that can potentially fail.
Example: When using `fopen` to open a file, if it fails, `errno` will be set. It’s imperative to inspect `errno` right away:
#include <iostream>
#include <cstring> // For std::strerror
#include <cerrno> // For errno and error numbers
int main() {
FILE* file = fopen("non_existing_file.txt", "r");
if (!file) {
std::cout << "Error opening file: " << std::strerror(errno) << std::endl;
}
return 0;
}
Common Functions that Set `errno`
Many standard library functions set `errno` when they fail. Understanding these functions allows you to handle errors effectively.
- `malloc`: Allocates memory dynamically. If it fails to allocate, it sets `errno` to `ENOMEM`.
int* arr = (int*)malloc(size);
if (arr == nullptr) {
std::cerr << "Memory allocation failed: " << std::strerror(errno) << std::endl;
}
- `strtol`: Converts a string to a long integer. If conversion fails, it sets `errno` to `ERANGE`.
const char* str = "abc"; // Invalid number
errno = 0; // Reset errno
long value = strtol(str, nullptr, 10);
if (errno == ERANGE) {
std::cerr << "Conversion error: " << std::strerror(errno) << std::endl;
}
These examples highlight how various functions can be monitored closely through `errno`.
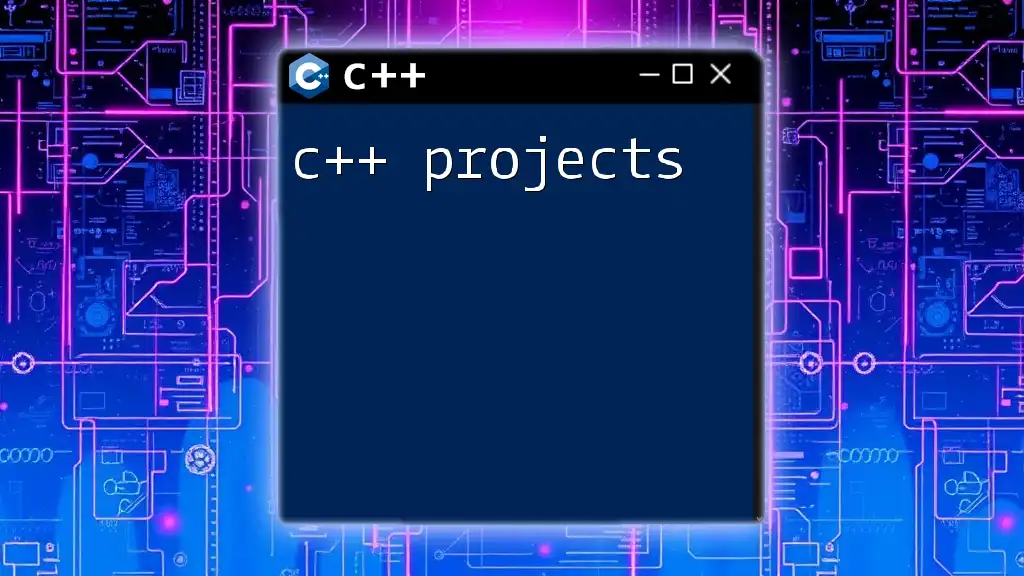
Common Error Codes
It helps to be familiar with common error codes that `errno` may be set to. Here’s a quick reference of some prevalent error codes:
- `EINVAL`: Indicates that an invalid argument was provided.
- `ENOMEM`: Signifies that the program ran out of memory.
- `EFAULT`: Implies a bad memory address was accessed.
Familiarity with these error codes can facilitate quicker debugging and error handling processes.
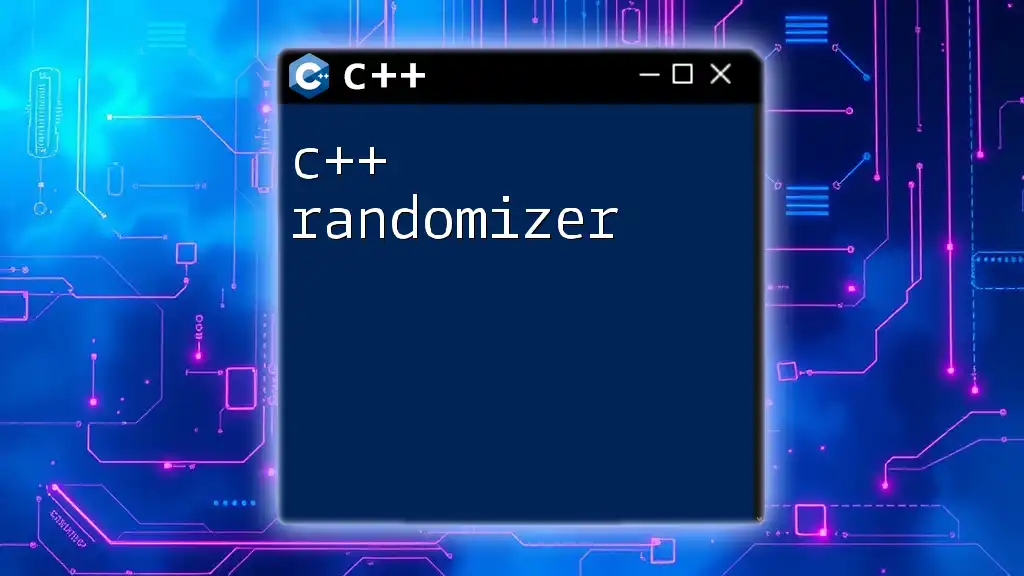
Best Practices for Using `errno`
Do Not Rely Solely on `errno`
It is crucial to understand that you should not depend solely on `errno`; always check the return values of functions. Functions often return an explicit indication of failure, and combining these checks with `errno` can provide more context.
Resetting `errno` Before Function Calls
Before calling a function that sets `errno`, ensure that you reset `errno` to zero. This practice helps distinguish between an actual error and a lingering previous value:
errno = 0; // Resetting errno
Error Handling in Functions
Incorporating error checks directly into function implementations is a good coding practice. Here’s an example function that performs safe division and sets `errno` for a division by zero scenario:
#include <iostream>
#include <cerrno>
#include <cstring>
void safeDivision(int a, int b) {
if (b == 0) {
errno = EINVAL; // Setting errno for division by zero
std::cerr << "Error: " << std::strerror(errno) << std::endl;
return;
}
std::cout << "Result: " << (a / b) << std::endl;
}
This function demonstrates how you can handle potential divides by zero and signal the user appropriately.
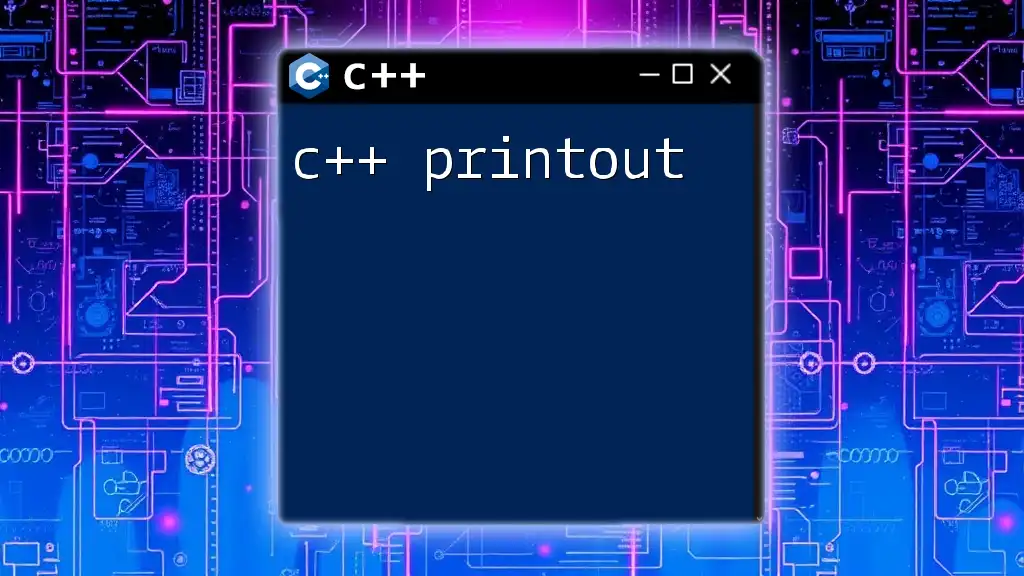
Understanding the Importance of `errno` in Modern C++
While C++ has incredibly robust exception handling mechanisms, `errno` remains relevant and practical in certain contexts. The use of `errno` is still valuable because:
- It provides a quick and lightweight mechanism for error reporting, especially in C-style APIs.
- In performance-critical applications, checking `errno` might yield better performance compared to handling exceptions, which can incur overhead.
Performance Considerations
When choosing between `errno` and exceptions, consider the trade-offs in performance and clarity. Using `errno` gives straightforward error feedback with minimal overhead, while exceptions provide richer contexts for error handling.
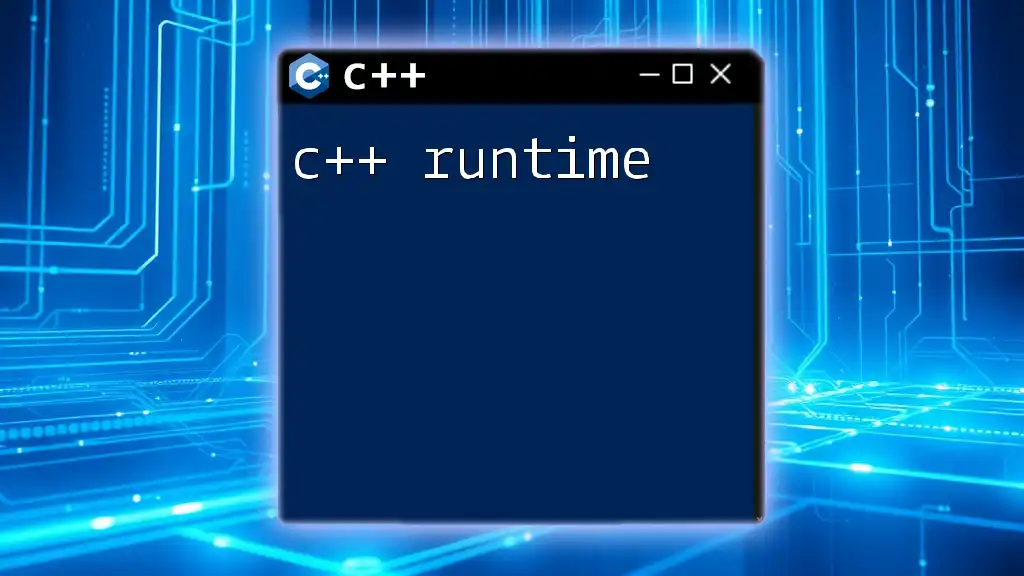
Conclusion
Throughout this guide, we explored the role of `errno` in C++, including its definition, usage, related error codes, best practices, and significance in modern software development. By effectively utilizing `errno`, you can enhance the robustness of your error handling strategies.
Embrace the practices outlined, and make `errno` an integral part of your C++ coding toolkit!