In C++, `cerr` is a predefined output stream used to display error messages to the standard error device, typically the console.
Here's a simple example to illustrate its usage:
#include <iostream>
int main() {
int num = -1;
if (num < 0) {
std::cerr << "Error: Negative number input!" << std::endl;
}
return 0;
}
What is cerr?
`cerr` is a predefined object in C++ that serves as the standard error output stream. It's part of the `<iostream>` header file and is primarily used for reporting errors and diagnostic information to the user. Understanding the difference between `cout`, `cerr`, and `clog` is crucial for effective output management in your applications:
-
cout: This is the standard output stream used for general display of information. It is buffered, meaning that output is stored temporarily before being displayed. This buffering can sometimes cause delays, especially if you're writing a significant amount of data or encountering errors frequently.
-
cerr: Unlike `cout`, `cerr` is an unbuffered stream, which means that data sent to this stream is immediately flushed and displayed. This property makes `cerr` particularly useful for error messages, ensuring that users see error information right away.
-
clog: Similar to `cout`, `clog` is a buffered stream used for logging. While you can use it for error messages, it might not be as immediate as using `cerr`.

How to Use cerr
Basic Syntax
Using `cerr` in your C++ program is straightforward. The basic syntax involves using the insertion operator (`<<`) to send messages to the `cerr` stream, just like you would with `cout`. Here's a simple example:
#include <iostream>
int main() {
std::cerr << "An error occurred!" << std::endl;
return 0;
}
In this code snippet, the message "An error occurred!" is sent to the standard error stream, making it more evident to the user that an issue has arisen.
Output Characteristics
One of the key characteristics of `cerr` is that it is always flushed after each output operation. This immediate flushing means that error messages won’t be delayed by buffering, which is crucial for debugging during runtime. Using `cerr` is suitable for logging errors, warnings, and important notes that need to be communicated to the user as quickly as possible.
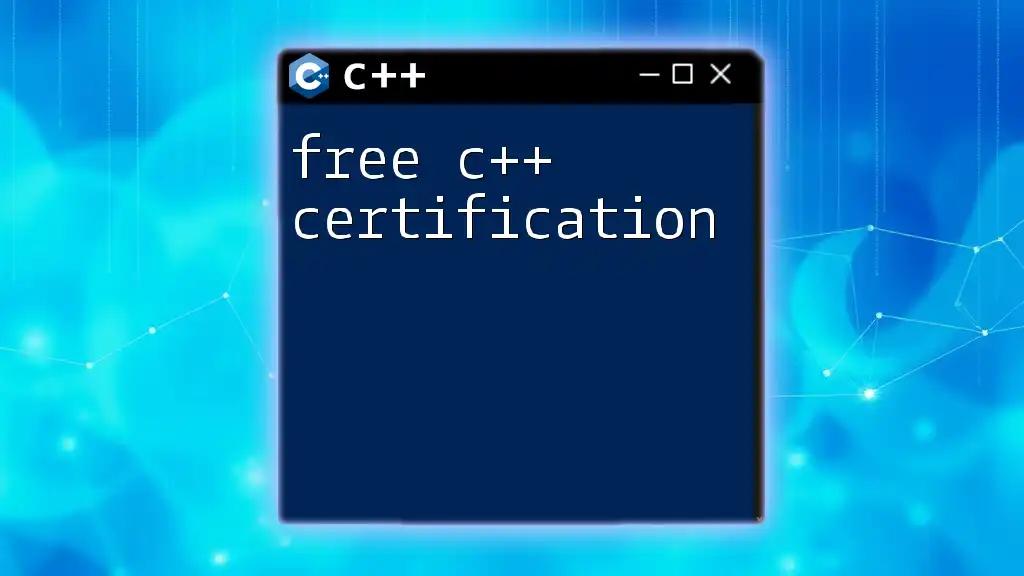
When to Use cerr
Error Handling
Effective error reporting is essential for robust application development. You should prefer using `cerr` anytime you need to inform the user about unexpected situations or errors encountered during program execution. This type of immediate feedback can be essential for debugging and problem resolution.
Consider this scenario where an input validation check fails:
if (inputValidationFailed) {
std::cerr << "Error: Invalid input provided." << std::endl;
}
In this example, when the input fails validation, a clear error message is sent directly to `cerr`, helping the user understand that they need to correct their input.
Best Practices
-
Timing of error output: Use `cerr` immediately after the error occurs. This ensures that users are promptly made aware of issues that may need their attention.
-
Clarity of error messages: It's not just about reporting an error; it's also about how clear and informative the message is. A well-structured error message can make all the difference in effective troubleshooting.
For example, a vague error message like:
std::cerr << "Err!" << std::endl;
is not helpful. Instead, use a more descriptive message that conveys what went wrong:
std::cerr << "Error: Invalid input provided." << std::endl;
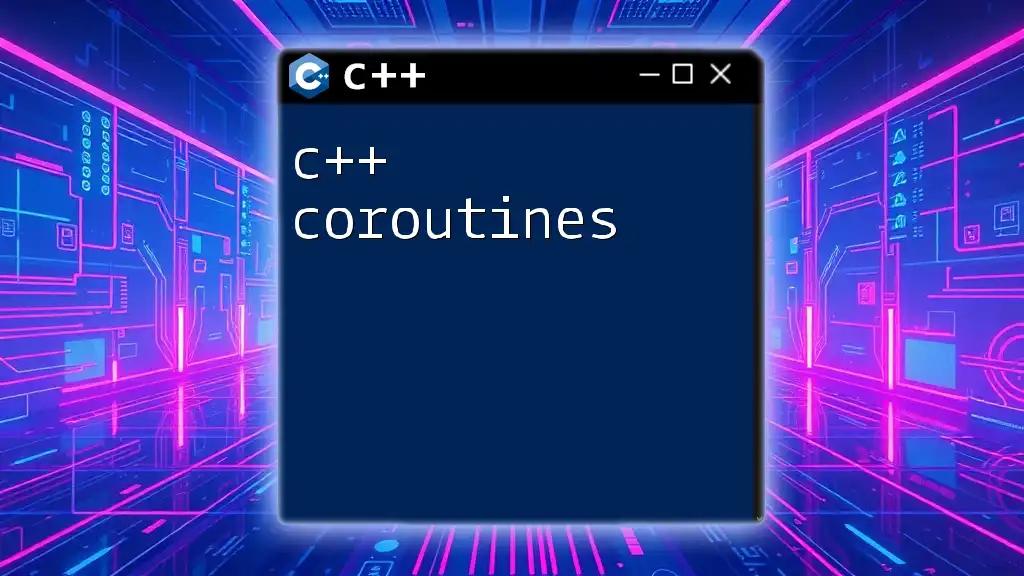
Redirecting cerr
Understanding Stream Redirection
Stream redirection in C++ allows you to direct output streams to different destinations, such as files or other streams. This could be particularly useful when you want to log errors into a file for later review instead of displaying them on the console.
Practical Examples
Here’s how you can redirect `cerr` to write error messages to a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream errorFile("errors.log");
std::streambuf* originalCerrBuffer = std::cerr.rdbuf(); // Save original buffer
std::cerr.rdbuf(errorFile.rdbuf()); // Redirect cerr to file
std::cerr << "This error will go to the file." << std::endl;
std::cerr.rdbuf(originalCerrBuffer); // Restore original cerr buffer
return 0;
}
In this example, we redirect `cerr` to an output file called `errors.log`. By saving the original buffer, we are also able to restore `cerr` back to its original state after we finish logging errors.

Comparison with cout
Performance Implications
Given that `cerr` is unbuffered while `cout` is buffered, it's essential to consider the performance implications in your application. If your application frequently generates error messages, using `cerr` ensures that users see those messages immediately without any delays. In contrast, if you’re merely informing about regular status updates, `cout` might be the better option due to its improved performance with larger volumes of data.
Use Cases
When deciding between `cerr` and `cout`, context is crucial. If an error occurs, such as a file failing to open, you should use `cerr`:
if (!file.open("data.txt")) {
std::cerr << "Error: Failed to open data.txt." << std::endl;
} else {
std::cout << "File opened successfully." << std::endl;
}
By using `cerr` for errors, you prioritize the user's immediate awareness of issues within the application.
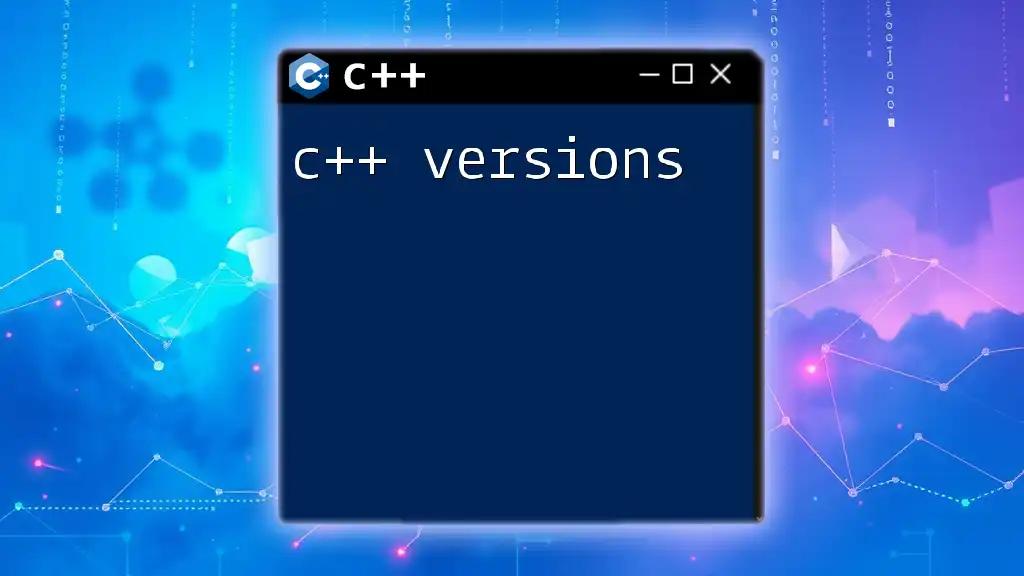
Common Mistakes When Using cerr
Misunderstanding Buffering
Many developers mistakenly assume that all output streams behave the same. Confusing `cerr` with `cout` can lead to issues, particularly in timing and visibility of error messages. Remember that `cerr` is unbuffered, while `cout` is not.
Not Handling Threads Properly
In multithreaded scenarios, special care must be taken when using `cerr`. Since multiple threads may simultaneously write to `cerr`, this can lead to interleaved output, making error messages difficult to read. It’s best to manage access to `cerr` through mutexes or other synchronization mechanisms to ensure thread safety.
Here’s a simple example that highlights potential interference in a multithreaded context:
#include <thread>
#include <iostream>
void logError() {
std::cerr << "Error logged from thread." << std::endl;
}
int main() {
std::thread t1(logError);
std::thread t2(logError);
t1.join();
t2.join();
return 0;
}
In this snippet, the error messages logged from each thread may not appear cleanly, making it hard to discern which message came from which thread. Proper synchronization techniques should be employed in such cases.
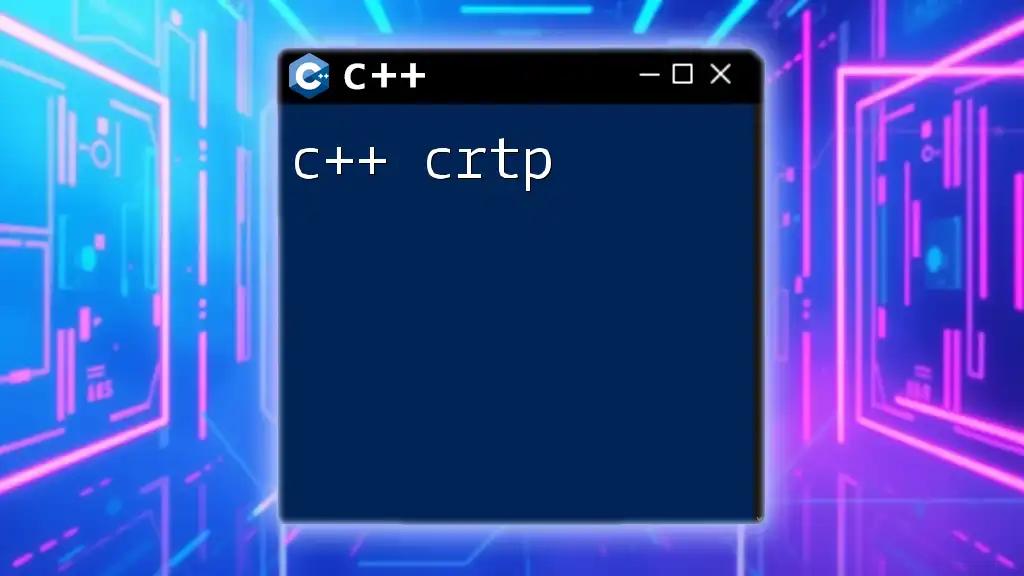
Conclusion
Understanding and effectively utilizing `c++ cerr` is essential for robust error reporting in your applications. By leveraging its properties, you can ensure that error messages are delivered promptly, enhancing user experience and simplifying the debugging process. Remember to practice clear messaging and consider the contexts where `cerr` shines the most.
Experiment with these examples and best practices, and soon you will be adept at managing error outputs efficiently in your C++ applications. For further exploration, consider diving into advanced topics surrounding C++ streams, file I/O, and multithreading techniques. These skills will help you develop applications that are not only functional but also user-friendly and robust.