C++/CLI (Common Language Infrastructure) is a set of language extensions added to C++ to allow for managed code that enables interoperability with other .NET languages.
Here’s a simple code snippet demonstrating a basic C++/CLI program that outputs "Hello, World!" to the console:
#include <iostream>
using namespace System;
int main()
{
Console::WriteLine("Hello, World!");
return 0;
}
Understanding the Basics of CLR
What is the Common Language Runtime (CLR)?
Common Language Runtime (CLR) is a fundamental component of the .NET Framework, which provides a runtime environment for executing applications. It facilitates a number of critical functions that help developers create robust and secure software.
The functionality of the CLR includes:
-
Memory management: CLR automates memory allocation and deallocation, helping to prevent memory leaks.
-
Exception handling: It provides a consistent way to handle exceptions across different .NET languages.
-
Security: CLR incorporates various security measures, ensuring that applications run in a safe environment and adhere to security protocols.
How C++ Fits into CLR
C++ CLR is a variant of C++ designed to be managed by the CLR. Native C++ code is executed directly by the operating system, while managed C++ (C++ CLR) requires the CLR for execution, thus enabling additional features like garbage collection and exception handling.
Key features of C++ CLR include:
-
Integration with .NET: C++ CLR enables developers to leverage the full power of .NET libraries in their applications, allowing access to an extensive range of pre-built functions and classes.
-
Language interoperability: C++ CLR code can easily interoperate with other .NET languages like C# and VB.NET, providing flexibility in application development.
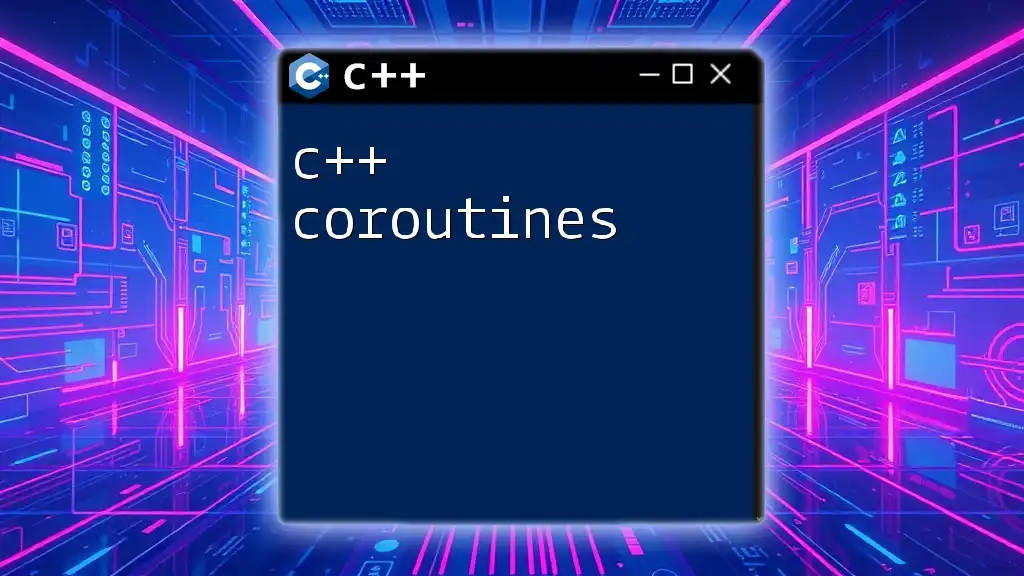
Setting Up Your Environment
Prerequisites for C++ CLR Development
Before starting with C++ CLR development, ensure you have the following:
-
Hardware requirements: A computer capable of running Visual Studio (typically any modern PC).
-
Software requirements: You need to install Visual Studio, preferably the latest version that supports C++ CLR, along with the relevant version of the .NET Framework.
Creating Your First C++ CLR Project
To embark on your C++ CLR journey, follow this quick step-by-step guide to create your first project:
-
Open Visual Studio.
-
Click on File -> New -> Project.
-
In the project templates, select Visual C++ and choose CLR.
-
Name your project and specify the location.
-
Click OK to create the project.
Familiarize yourself with the created project structure, including source files, headers, and resource files.
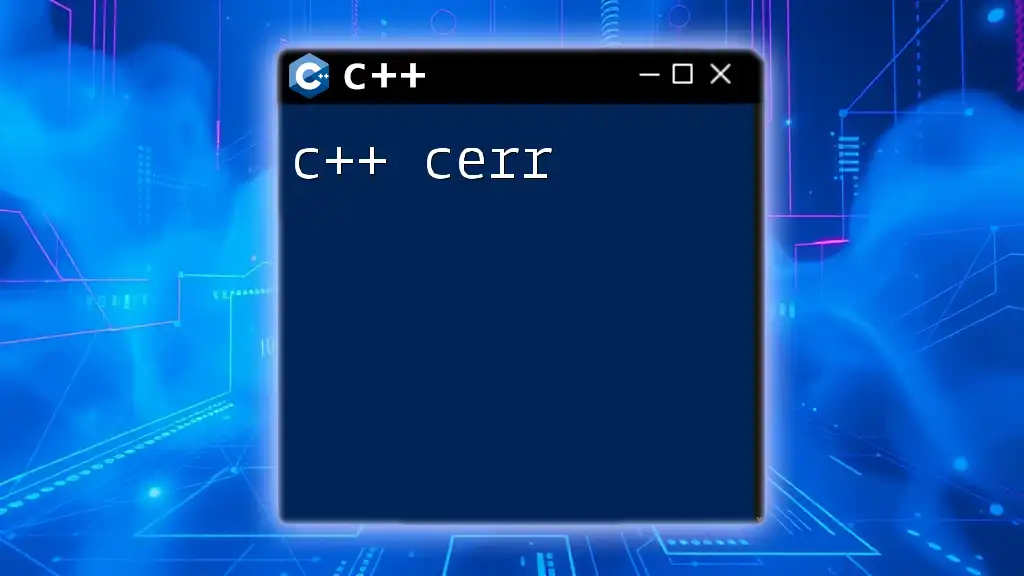
Core Concepts of C++ CLR Development
Syntax and Structure of C++ CLR
C++ CLR syntax shares similarities with standard C++, but there are important distinctions. For instance, in managed code, you utilize `^` to declare a managed pointer, as follows:
int^ managedPointer = gcnew int(42);
CLR utilizes namespaces to avoid naming conflicts. The `System` namespace is a common one used in C++ CLR development, enabling access to essential classes such as `String`, `Console`, and many more.
Data Types and Structures in C++ CLR
In C++ CLR, you work with both standard C++ data types and managed types. For instance, the `System::String` type is utilized in lieu of traditional C-style strings:
String^ myString = "Hello, C++ CLR!";
Exception Handling in C++ CLR
Exception handling in C++ CLR is more standardized than in traditional C++. Use `try`, `catch`, and `finally` keywords to handle exceptions effectively. For example:
try
{
// Some risky code
}
catch (System::Exception^ ex)
{
Console::WriteLine(ex->Message);
}
finally
{
// Cleanup code
}
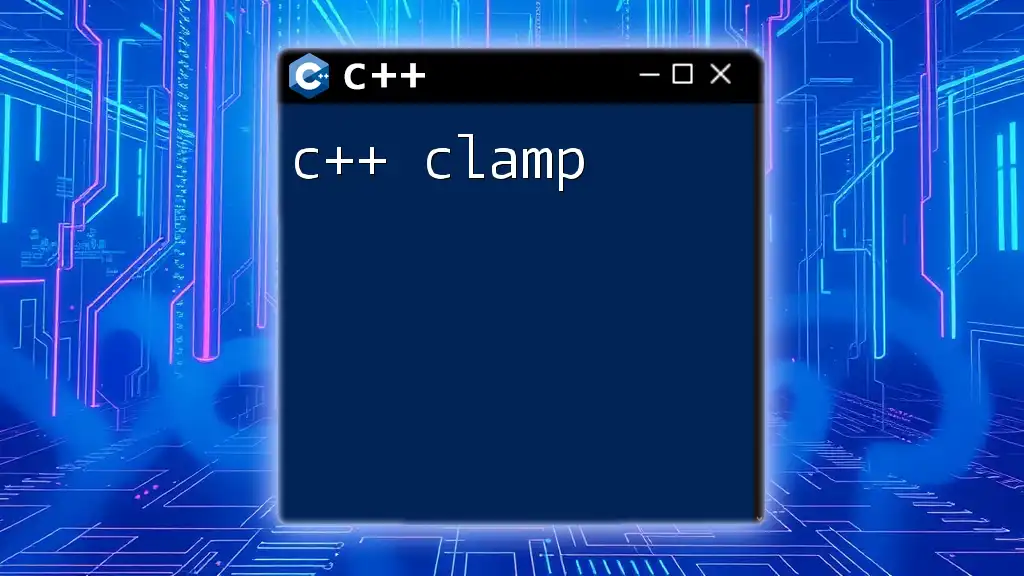
Advanced Features of C++ CLR
Working with .NET Libraries
One of the main advantages of C++ CLR is that it allows you to use existing .NET libraries with ease. You can import .NET libraries into your C++ project using the `#using` directive:
#using <System.dll>
// Now you can use classes from System namespace
Example: Using a .NET Library:
#include <iostream>
using namespace System;
void useDateTime()
{
DateTime^ now = DateTime::Now;
Console::WriteLine("Current date and time: {0}", now);
}
Interoperability with Other Languages
C++ CLR supports language interoperability, allowing you to call functions from C# or other .NET languages. This is invaluable when leveraging existing components in an overarching application.
Practical Example:
If you have a C# method `Add` in your C# program, you can call it from your C++ CLR code after including the appropriate references.
Creating Windows Forms Applications
C++ CLR also allows you to develop Windows Forms applications effortlessly.
Setting Up a Windows Form is quite straightforward:
- Create a new C++ CLR Windows Forms project.
- Use the designer to add controls like buttons and text boxes.
Code Snippet Example: A Simple Form:
using namespace System;
using namespace System::Windows::Forms;
public ref class MyForm : public Form
{
public:
MyForm()
{
Button^ myButton = gcnew Button();
myButton->Text = "Click Me!";
myButton->Click += gcnew EventHandler(this, &MyForm::OnButtonClick);
Controls->Add(myButton);
}
private:
void OnButtonClick(Object^ sender, EventArgs^ e)
{
MessageBox::Show("Button Clicked!");
}
};
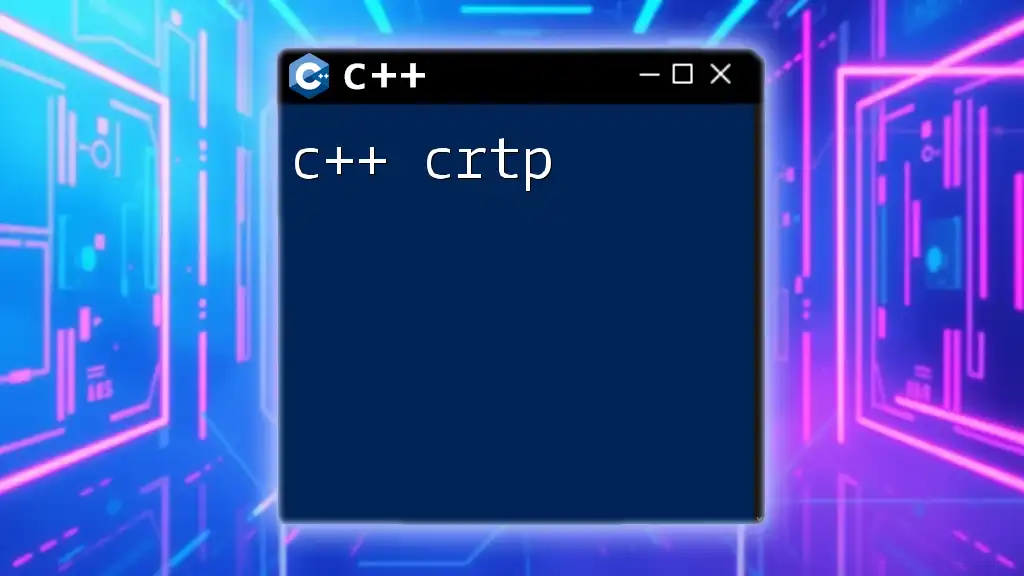
Debugging and Troubleshooting
Common Issues in C++ CLR
Debugging is an essential part of the development process. Some common issues might arise during C++ CLR development, ranging from linking errors to memory leaks.
Debugging Best Practices include:
- Utilize breakpoints to pause execution and inspect variables.
- Use watch windows to monitor state variables throughout execution.
Tools for Debugging
Visual Studio provides robust debugging tools. You can set breakpoints, examine variable states in real-time, and utilize the immediate window to test commands dynamically.
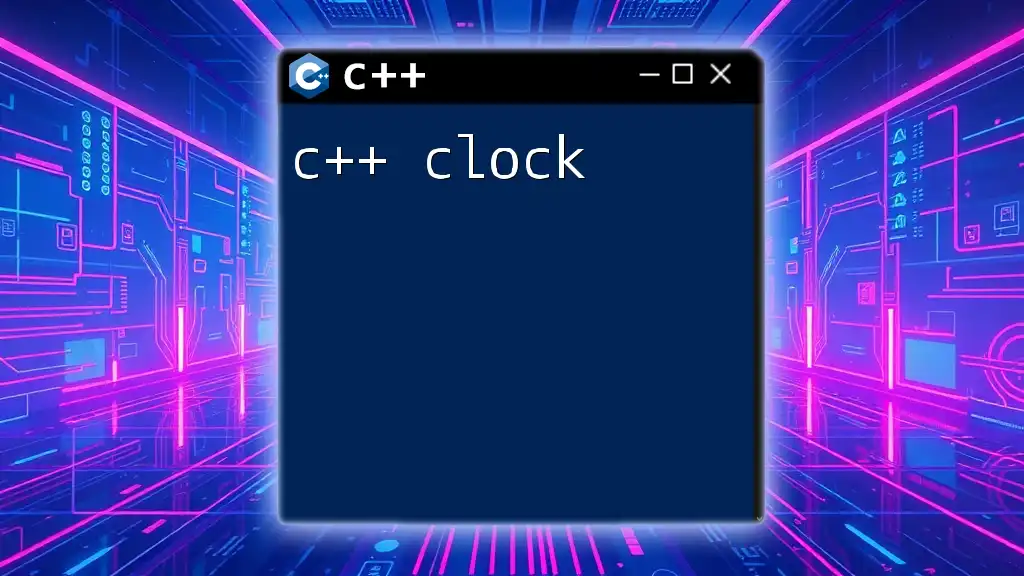
Best Practices in C++ CLR Development
Performance Optimization Techniques
When designing applications with C++ CLR, memory management can significantly impact performance. The CLR automates garbage collection, but developers should be mindful of object instances and their lifecycle to balance performance and resource efficiency.
Code Maintenance and Readability
Writing maintainable code is critical. Adhere to structured coding practices, such as consistent naming conventions, commenting code, and breaking complex functions into manageable pieces.
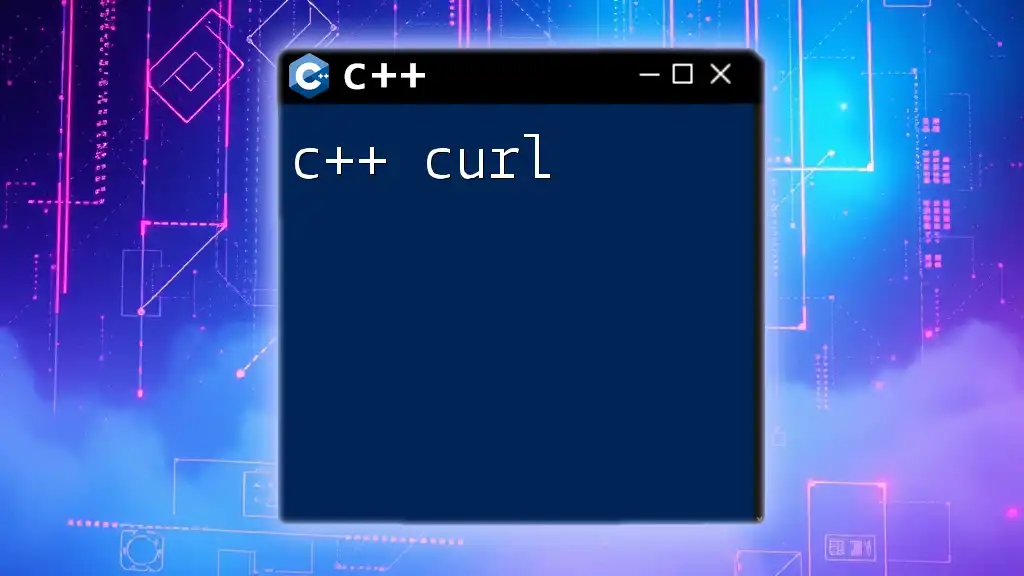
Conclusion
In summary, C++ CLR offers a powerful bridge between C++ and the .NET Framework, combining the robustness of C++ with the capabilities of managed code. Understanding its components and best practices enables developers to create efficient, safe, and scalable applications. Engage with deeper resources to further your mastery of C++ CLR and uncover its vast potential.
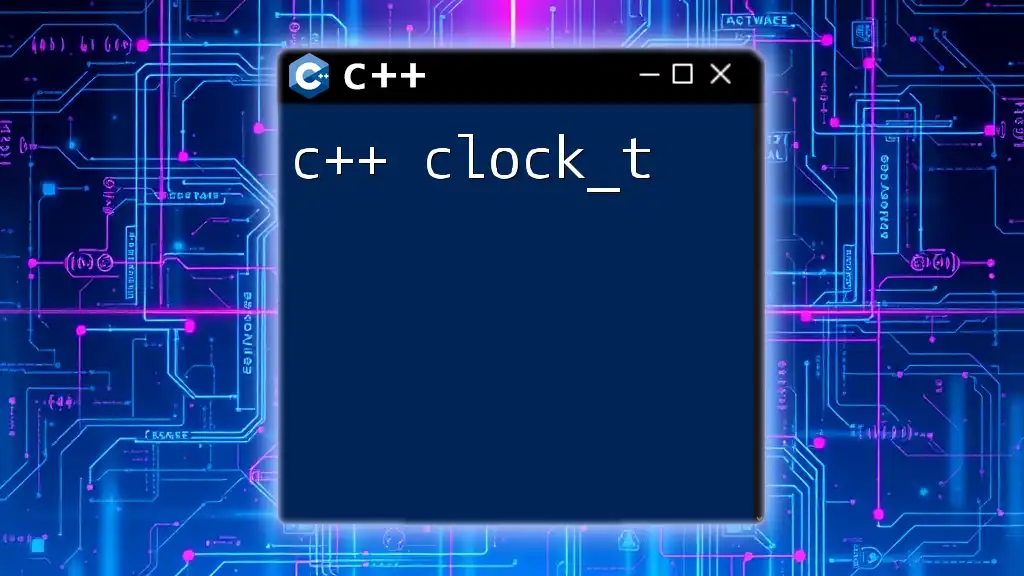
Additional Resources
For further understanding and expertise, explore the official C++ CLR documentation, recommended books, and relevant online courses that dive deeper into development with C++ CLR. Engage with community forums for collaborative learning and troubleshooting advice from peers.