The C++ `clock` function from the `<ctime>` library measures processor time consumed by the program, which can be useful for performance evaluation. Here is a simple example of how to use it:
#include <iostream>
#include <ctime>
int main() {
clock_t start = clock(); // Start the clock
// Your code here
clock_t end = clock(); // End the clock
double elapsed = double(end - start) / CLOCKS_PER_SEC; // Calculate elapsed time
std::cout << "Elapsed time: " << elapsed << " seconds." << std::endl;
return 0;
}
Understanding the C++ `<chrono>` Library
What is `<chrono>`?
The `<chrono>` library is a powerful component of the C++ Standard Library introduced in C++11. It provides tools to work with date and time, handle clock types, and measure durations with precision. Its extensibility makes it suitable for a variety of applications, from simple time measurements to complex timing mechanisms.
Key Components of `<chrono>`
Clock types
In the world of C++ clocks, the `<chrono>` library offers three main types of clocks:
-
System Clock: Represents the current wall-clock time. It can be used to retrieve the current date and time and is subject to changes by the operating system.
-
Steady Clock: This clock provides a monotonic time representation that does not change with system time adjustments. It is ideal for measuring elapsed time intervals without being affected by changes to the system clock.
-
High-resolution Clock: This clock offers the highest resolution provided by the system's hardware. It is often implemented using the underlying steady clock and is useful for profiling the performance of time-sensitive applications.
Duration
The duration in `<chrono>` represents a time span. It can describe how much time has passed between two time points, or it can define a specific duration directly. Durations can be specified in various time units, such as seconds, milliseconds, or microseconds.
Time Point
A time point captures a specific moment in time, often represented using a clock and a duration. By using time points, you can easily compute differences and intervals, which is essential for conducting time-related calculations.
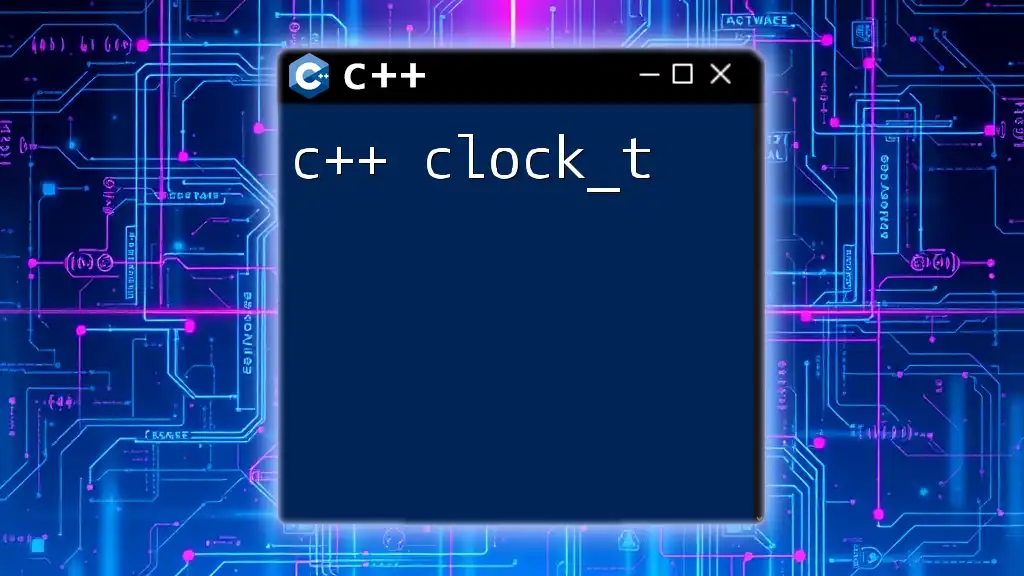
Using Clocks in C++
The System Clock
The system clock is a convenient way to access the current wall-clock time, suitable for most applications where you need to fetch the date and time.
Code Example:
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::cout << "Current time: " << std::ctime(&now_time);
return 0;
}
In this example, we fetch the current system time and convert it to a human-readable format using the `std::ctime()` method. This can be useful for logging, time-stamping events, or displaying the current time to users.
The Steady Clock
The steady clock is particularly valuable for measuring elapsed time since it is unaffected by changes to the system time (such as manual updates or NTP synchronization). This makes it ideal for performance measurements.
Code Example:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
auto start = std::chrono::steady_clock::now();
std::this_thread::sleep_for(std::chrono::seconds(1));
auto end = std::chrono::steady_clock::now();
std::chrono::duration<double> elapsed_seconds = end - start;
std::cout << "Elapsed time: " << elapsed_seconds.count() << " seconds" << std::endl;
return 0;
}
In this snippet, we simulate a delay of one second using `std::this_thread::sleep_for()`. By measuring the elapsed time before and after the sleep function, we find out how long our operation took, giving us insight into the operation's efficiency.
The High-Resolution Clock
The high-resolution clock is essential when you need the best possible time resolution. It is beneficial for performance testing and applications requiring precise timing, like animations and real-time systems.
Code Example:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Some high-cost operation
for (int i = 0; i < 1000000; ++i) {}
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start);
std::cout << "Operation took: " << duration.count() << " microseconds" << std::endl;
return 0;
}
In this example, we perform a loop that simulates some intensive computation. By noting the time before and after the operation, we can effectively measure how much time it took, providing essential information about performance in microseconds.
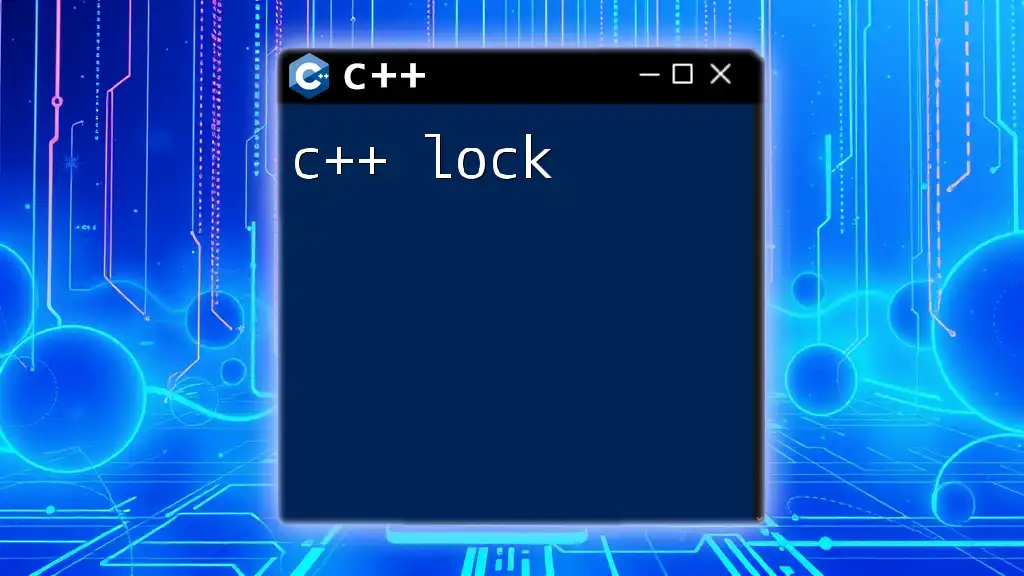
Working with `duration`
Creating and Manipulating Durations
Durations help quantify time spans. The `<chrono>` library allows you to create and manipulate both individual and relative durations using its various duration types.
Code Example:
#include <iostream>
#include <chrono>
int main() {
using namespace std::chrono;
duration<int> one_minute(60);
duration<double> one_and_a_half_minutes(90.0);
std::cout << "One minute in seconds: " << one_minute.count() << std::endl;
std::cout << "One and a half minutes in seconds: " << one_and_a_half_minutes.count() << std::endl;
return 0;
}
Here, we create two durations, one for 60 seconds (1 minute) and another for 90 seconds (1.5 minutes). Utilizing the `count()` method allows us to easily retrieve the duration in seconds.
Conversions Between Durations
Converting from one duration type to another can be essential, especially in performance-critical applications or wherever consistent time unit representation is necessary.
Code Example:
#include <iostream>
#include <chrono>
int main() {
std::chrono::seconds s(5);
std::chrono::milliseconds ms = std::chrono::duration_cast<std::chrono::milliseconds>(s);
std::cout << "5 seconds in milliseconds: " << ms.count() << std::endl;
return 0;
}
In this code snippet, we convert 5 seconds into milliseconds using `duration_cast`. The flexibility offered by `std::chrono` provides an easy way to work seamlessly with different time units according to application needs.
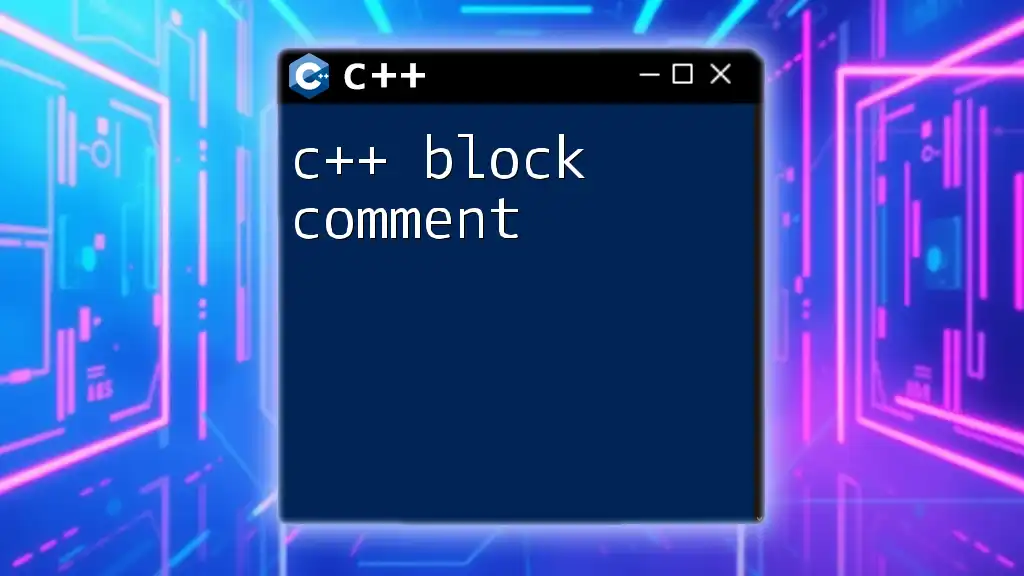
Practical Applications of C++ Clock
Benchmarking Code Performance
Measuring performance is crucial when developing software. Understanding how long certain operations take helps identify bottlenecks and optimize resource use.
Code Example:
#include <iostream>
#include <chrono>
void exampleFunction() {
// Simulate some work
for (volatile int i = 0; i < 1000000; ++i);
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
exampleFunction();
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start);
std::cout << "Function execution time: " << duration.count() << " microseconds" << std::endl;
return 0;
}
In this example, we call a dummy function to simulate a workload and then measure how long that function takes to execute in microseconds. Benchmarking like this can guide performance tuning and development priorities.
Creating Timers
Timers are major components in many applications, especially in gaming and interactive interfaces. C++ clocks provide a straightforward way to implement timers.
Basic Timer Code Example:
#include <iostream>
#include <chrono>
#include <thread>
void countdown(int seconds) {
for (int i = seconds; i > 0; --i) {
std::cout << "Timer: " << i << " seconds remaining." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
std::cout << "Time's up!" << std::endl;
}
int main() {
countdown(10);
return 0;
}
This simple countdown timer uses a loop to print the remaining seconds until the timer reaches zero, effectively demonstrating how to build interactive clocks to enhance user experience.
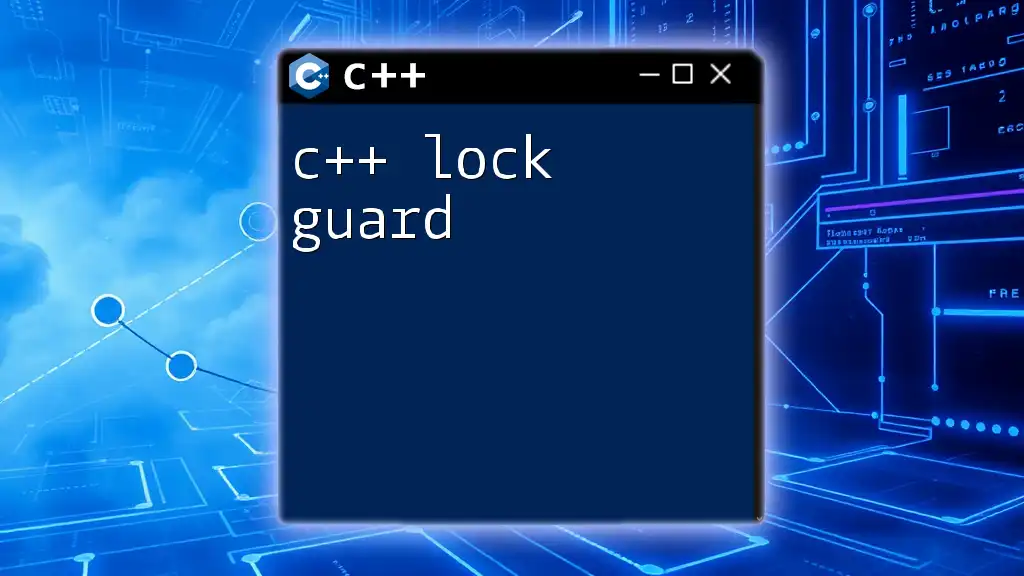
Best Practices for Using Clocks in C++
Avoiding Pitfalls
Understanding the operational characteristics of each clock type is crucial. For instance, relying on the system clock for elapsed time measurements can lead to inaccuracies due to adjustments in system time. It’s best to use the steady clock when accurate elapsed time is required.
Performance Considerations
When working with clocks, consider the underlying hardware and platform, especially when choosing between steady and high-resolution clocks. High-resolution clocks may introduce overhead that may not justify their use in low-precision environments, while steady clocks are optimal for long-term time measurements.
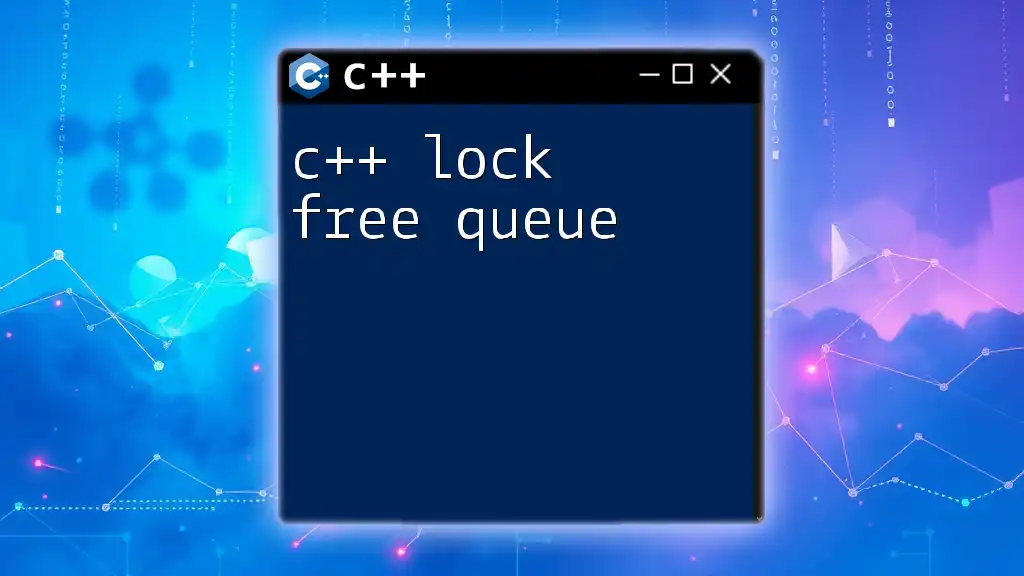
Conclusion
A C++ clock is an invaluable tool in a programmer's toolkit, offering precision and functionality that aid in various scenarios, from simple time displays to complex timing mechanisms. Familiarity with the `<chrono>` library and its components can enhance your ability to manage time effectively in your applications. Users are encouraged to experiment with the various clock types and their features to gain practical knowledge and skills in timing.
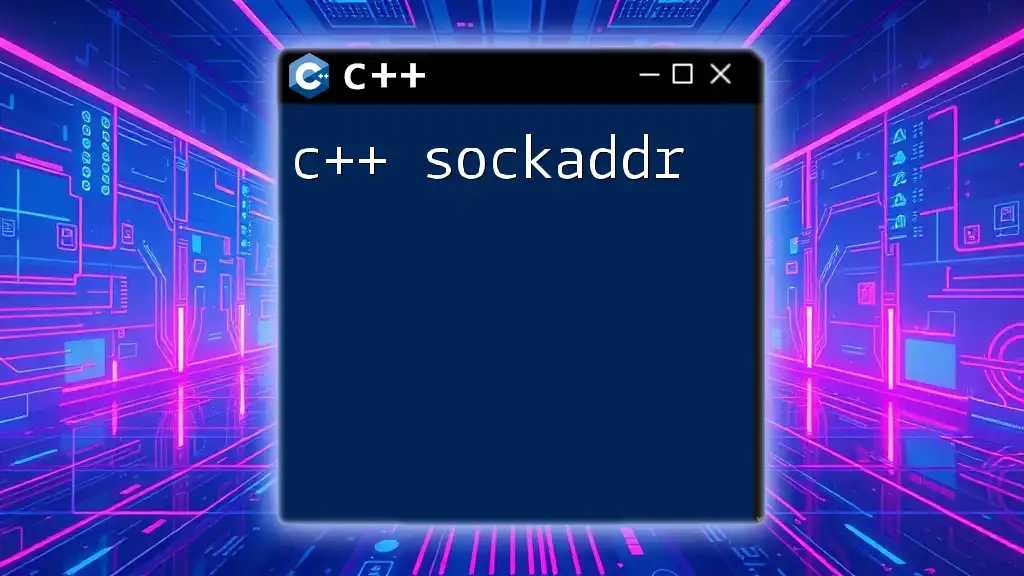
Additional Resources
For further exploration, it is recommended to consult the official C++ documentation on the `<chrono>` library and seek additional learning materials and exercises to solidify your understanding of the concepts introduced in this guide.