A C++ lookup table is a data structure that allows for efficient retrieval of values indexed by keys, typically implemented using arrays or maps for quick access.
Here's a simple example of a C++ lookup table using a map:
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, int> lookupTable = {
{"apple", 1},
{"banana", 2},
{"orange", 3}
};
std::string key = "banana";
std::cout << "The value for '" << key << "' is: " << lookupTable[key] << std::endl;
return 0;
}
Understanding Lookup Tables
What is a Lookup Table?
A lookup table is a data structure that stores pre-calculated values, allowing for efficient retrieval. It typically consists of key-value pairs, where each key corresponds to a specific value. This concept is widely applicable in various programming scenarios, such as graphics rendering, algorithm optimization, and data processing.
Why Use Lookup Tables?
Performance benefits are one of the primary reasons for utilizing lookup tables. They accelerate data retrieval processes, reducing the need for repeated calculations. The speed gained from a lookup can be significant, especially in performance-sensitive applications like video games and simulations.
Practical examples of their use include:
- Game Development: In games, lookup tables can store predefined values, such as textures or sound parameters, which need quick access during runtime.
- Data Processing: When processing large datasets, lookup tables can help in mapping and transforming data more efficiently compared to iterative calculations.
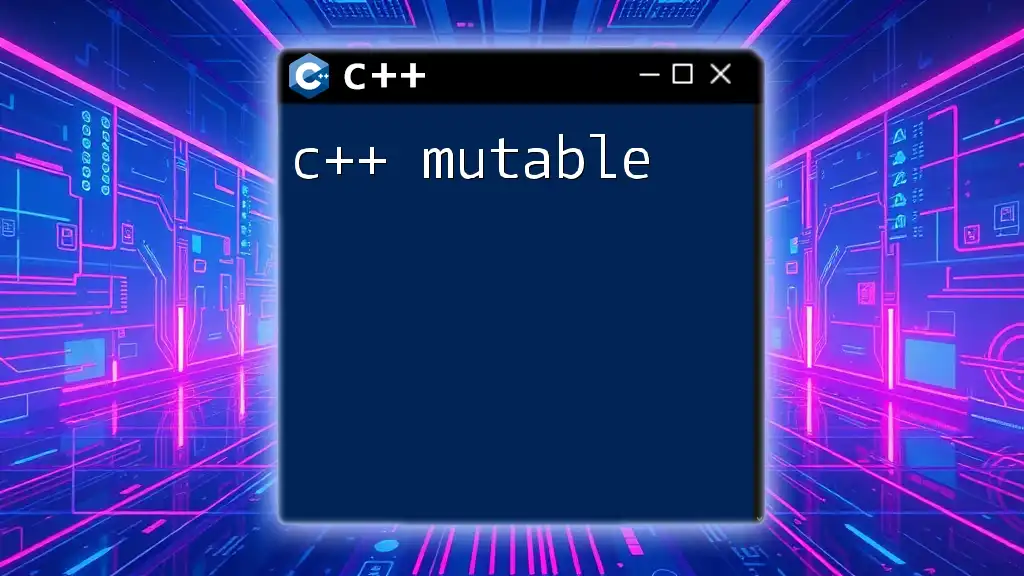
Creating a Lookup Table in C++
Basic Structure of a Lookup Table
To create a basic lookup table in C++, you can use associating key-value pairs. The most common C++ containers for this are `std::map` and `std::unordered_map`. Here is a simple example that maps integers to strings:
#include <iostream>
#include <map>
#include <string>
std::map<int, std::string> lookupTable = {
{1, "Apple"},
{2, "Banana"},
{3, "Cherry"}
};
std::cout << lookupTable[2]; // Output: Banana
In this example, if you want to access the fruit corresponding to `key 2`, you simply query the map: `lookupTable[2]`.
Using Arrays as Lookup Tables
For simpler lookups, you can also employ arrays. Arrays work best when dealing with a fixed range of keys. Here’s an example where we use an array to quickly convert numerical statuses into their string representations:
#include <iostream>
const char* statusLookup[] = {
"Inactive", // 0
"Active", // 1
"Suspended" // 2
};
std::cout << statusLookup[1]; // Output: Active
In this case, the lookup is direct and very efficient due to the array’s contiguous memory allocation.
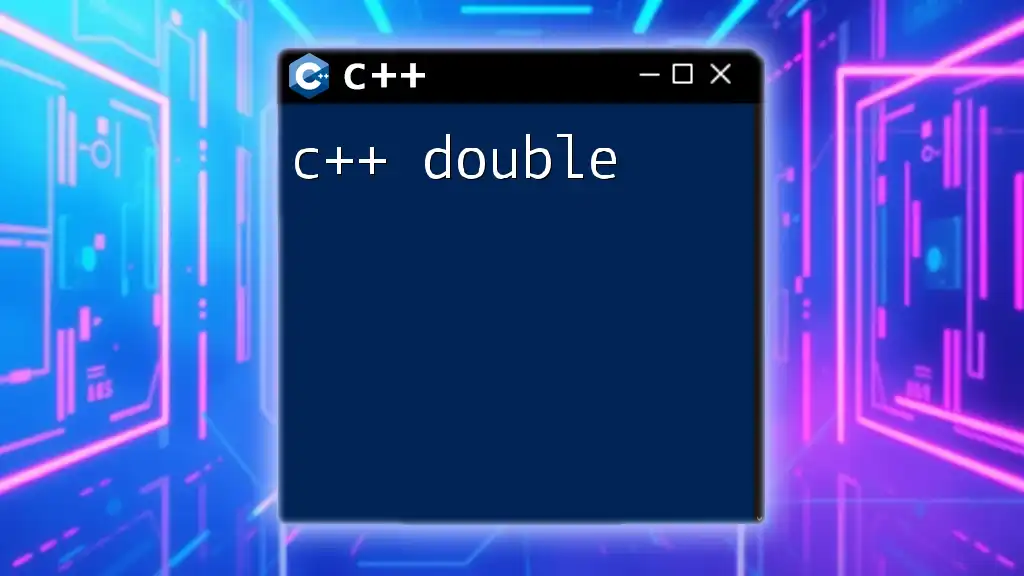
Advanced Techniques for Lookup Tables
Using std::unordered_map
For situations where speed is crucial and the order of elements doesn't matter, `std::unordered_map` provides a faster option. Unlike `std::map`, which is ordered, `std::unordered_map` uses hash tables under the hood. Here’s an example demonstrating its use:
#include <unordered_map>
#include <iostream>
std::unordered_map<int, std::string> unorderedLookupTable = {
{1, "Red"},
{2, "Blue"},
{3, "Green"}
};
std::cout << unorderedLookupTable[2]; // Output: Blue
This technique is particularly helpful when the volume of unique keys is high and lookup speed is paramount.
Hash Functions and Their Role
Hash functions are integral to the functioning of unordered maps. They convert keys into unique hash values, facilitating quicker data access. For instance, if you want to implement a custom hash function, you might do it like this:
struct CustomHash {
std::size_t operator()(const std::string& s) const {
return std::hash<std::string>()(s);
}
};
// Usage example
std::unordered_map<std::string, int, CustomHash> customHashTable;
This feature is particularly useful when you are dealing with strings or other complex data types.
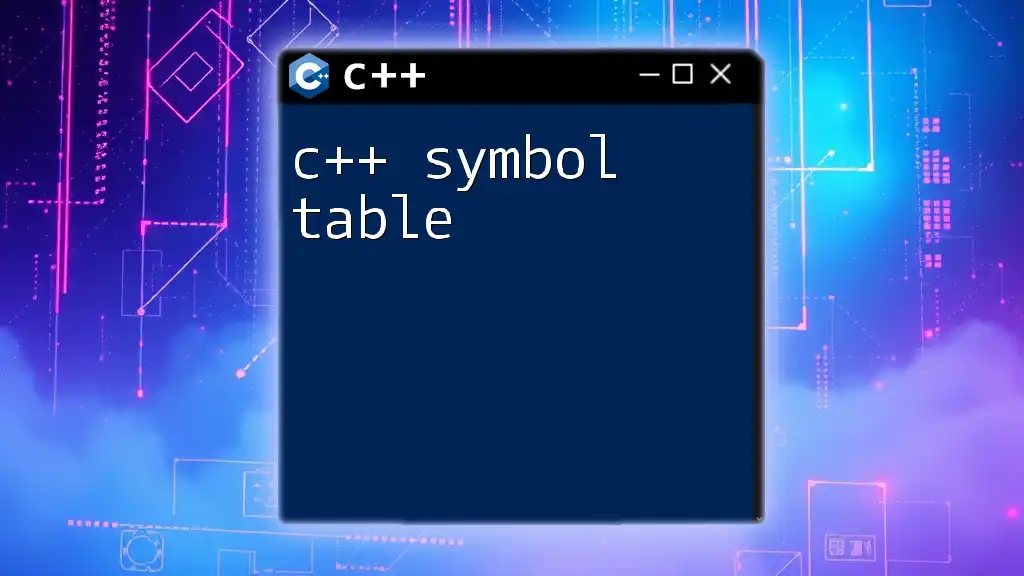
Optimizing Lookup Tables
Memory Efficiency
One critical aspect of working with lookup tables is optimizing their memory usage. Here are some tips:
- Choosing appropriate data types can significantly reduce the memory footprint. For example, if keys are limited to a small range, prefer smaller types like `uint8_t`.
- Using enums in tables can also enhance clarity and reduce memory usage. By mapping enums to their corresponding values, you maintain type safety without wasting memory.
Performance Tuning
To ensure that lookup operations are swift, consider profiling and measuring the lookup times. An efficient way to do this is by utilizing high-resolution clocks available in the `<chrono>` library:
#include <chrono>
#include <iostream>
// Hypothetical lookup function
void performLookupOperation() {
// Simulated lookup action
}
auto start = std::chrono::high_resolution_clock::now();
performLookupOperation();
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Lookup time: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end - start).count()
<< "µs\n";
This snippet helps in identifying performance bottlenecks that need to be addressed.
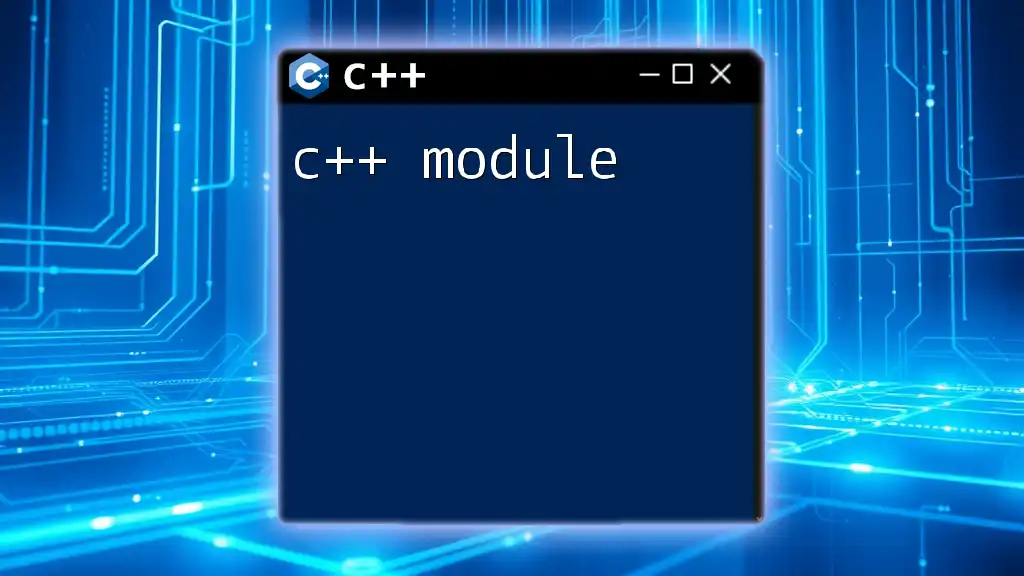
Common Mistakes and Troubleshooting
Misconfigurations
One common pitfall is failing to properly initialize a lookup table before accessing it. Ensure that all keys are defined and correctly mapped. If you access a key that doesn’t exist, the behavior can lead to unexpected results or even crashes.
Performance Issues
If you encounter performance issues, profiling is key. Ensure you are using the right container that meets your performance needs. For example, if lookups are slow, you might have transitioned from using `std::map` to `std::unordered_map` but neglecting to check key consistency can result in invalid performance gains.
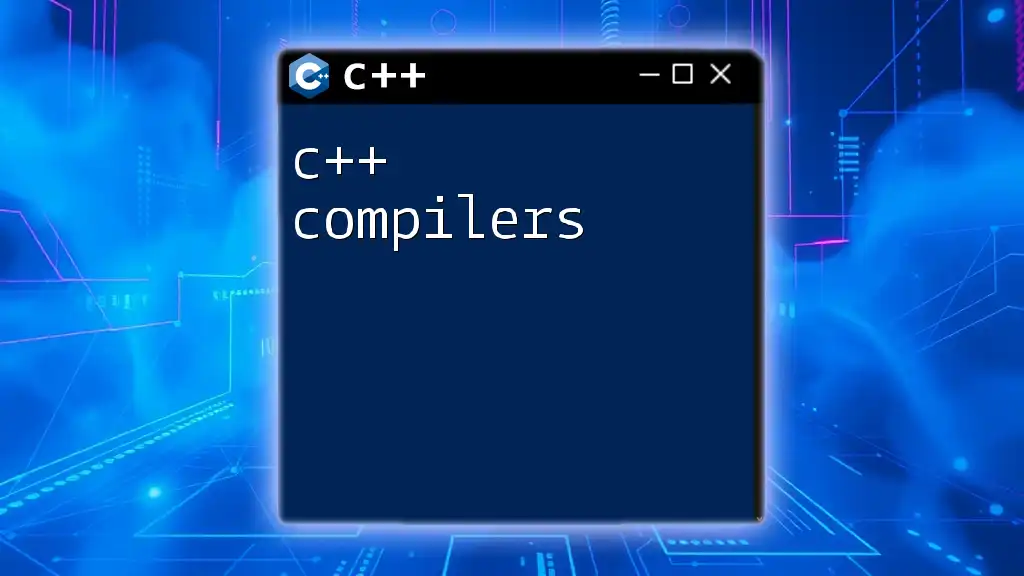
Conclusion
C++ lookup tables are powerful tools that can vastly improve performance and efficiency in your applications. They allow for fast data retrieval, reduced computations, and more organized code. By exploring the various aspects of creating and managing lookup tables—whether through basic structures like arrays or advanced hash maps—you can significantly optimize your C++ programs.
Make sure to experiment with the examples provided, and continue learning to become adept at implementing C++ lookup tables effectively. Happy coding!
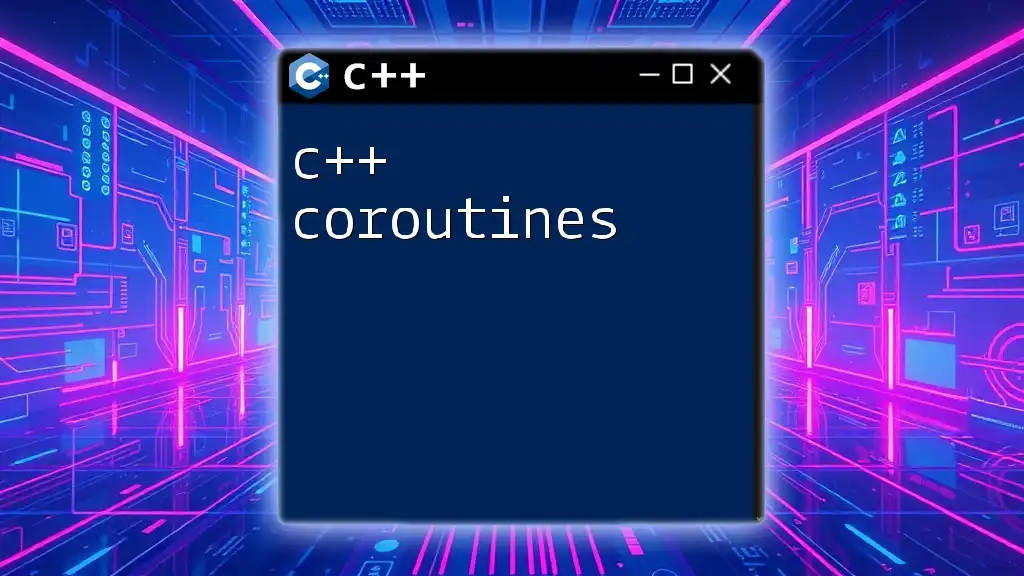
Additional Resources
For further reading and in-depth learning, consider exploring:
- Books such as "Effective Modern C++" by Scott Meyers
- Online resources including C++ reference documentation and community tutorials for standard library implementations.