The `mutable` keyword in C++ allows a member of a `const` object to be modified, enabling flexible design while still maintaining const-correctness.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
class Example {
public:
mutable int counter; // mutable member
Example() : counter(0) {}
void increment() const {
counter++; // modifying a mutable member in a const function
}
};
int main() {
const Example ex;
ex.increment();
std::cout << ex.counter << std::endl; // Output: 1
return 0;
}
What is `mutable`?
The `mutable` keyword in C++ serves a crucial role in allowing specific member variables of a class to be modified even when they are part of a `const` object. This means that if an instance of a class is declared as `const`, its non-`mutable` members cannot be altered. However, if a member is marked as `mutable`, its value can still be changed, even within a `const` member function. This functionality can be particularly beneficial in scenarios where internal state changes are necessary, but the interface needs to remain constant.
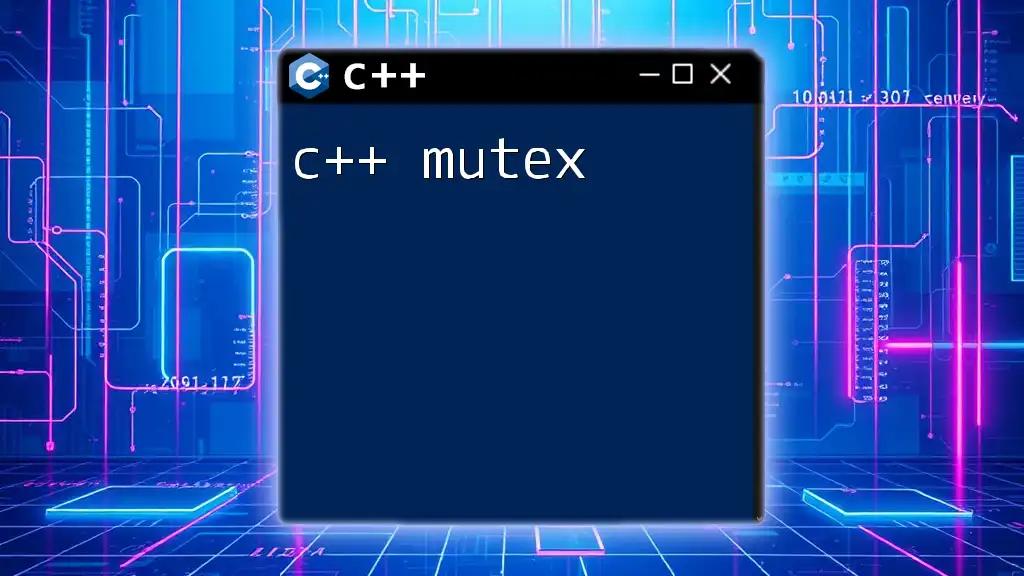
Why Use `mutable`?
Using `mutable` provides several significant advantages:
- Flexibility in Constants: It allows certain states to be changed without compromising the constness of the entire object.
- Caching Mechanisms: This can be highly useful when implementing caching mechanisms in constant functions, where intermediate computations may need to be stored.
- Thread Safety: Mutable can help manage mutable states in a multi-threaded context, allowing for safe modifications without requiring the entire function to be non-const.
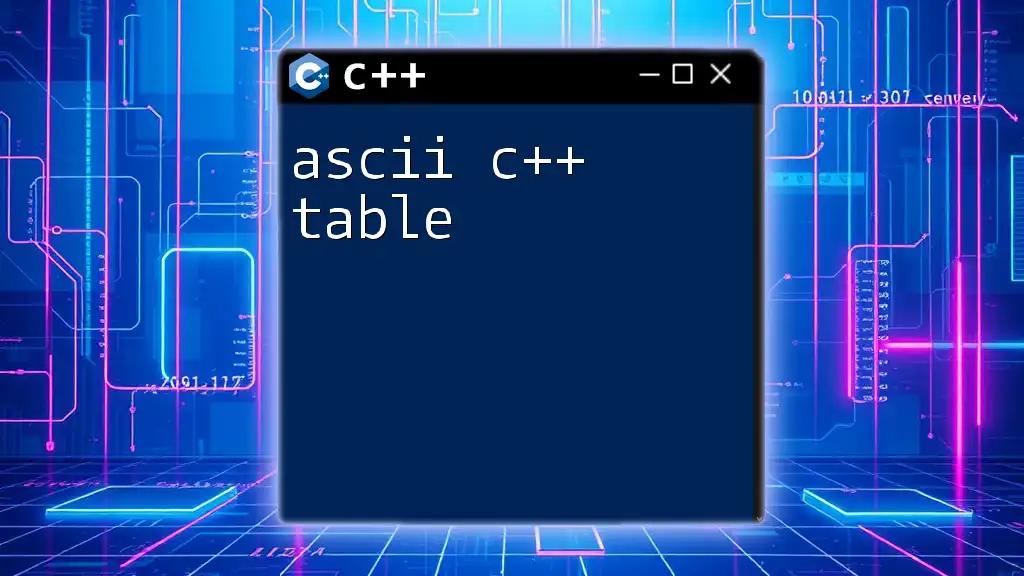
Understanding the Syntax of `mutable`
Basic Syntax
The syntax for using `mutable` within a class is straightforward. It is simply placed before the type declarator in a class definition.
class Example {
public:
mutable int counter;
void increment() const {
counter++;
}
};
In this example, while the `increment` function is marked as `const`, it can still modify the `counter` member as it is declared with `mutable`.
Applying `mutable` in Class Members
The real power of `mutable` shines when applied to class member variables, especially within `const` member functions. When you declare a member variable as `mutable`, you indicate that it holds a state that can change independently of the object's logical constness.
To illustrate:
class Logger {
public:
mutable bool is_dirty; // Tracks if the logger needs to write to disk
Logger() : is_dirty(false) {}
void log(const std::string &message) const {
// Log the message...
is_dirty = true; // Still able to modify `is_dirty` in a const method
}
};
In this Logger class, the `is_dirty` variable can mark whether the logger needs to write to the disk, and it can change even in a method declared as `const`.

Practical Applications of `mutable`
Use Cases for `mutable`
`mutable` finds utility in various real-world applications:
- Caching Results in a Function: In scenarios where a function computes a result that may be reused, marking a class member as `mutable` allows that member to store the cached value without violating the `const` contract.
- Managing Internal States: In classes where the state can be ‘dirty’ or ‘clean’ (e.g., a graphical object that requires updates), `mutable` provides a mechanism to manage these states flexibly.
Example: Caching Results
To further illustrate, consider a caching mechanism implemented with `mutable`:
class Cache {
public:
mutable std::map<int, std::string> data;
std::string getData(int key) const {
if (data.find(key) == data.end()) {
// Imagine a complex calculation here
data[key] = "Computed Data";
}
return data[key];
}
};
In the `Cache` class, the `data` map can be populated in a function declared as `const`. This means once the data is computed for a specific key, it can be cached for later retrieval, improving performance without needing to modify the original object interface.

Common Misunderstandings about `mutable`
Misconceptions Surrounding `mutable`
It’s critical to clarify some common misconceptions surrounding `mutable`.
- Not All Members Can Be Mutable: Only non-static, non-const data members can be marked as `mutable`. This means you cannot apply `mutable` to regular variables within the class's functions or static members.
- Does Not Override Constness: The presence of `mutable` does not bypass the constness guarantee provided by C++. If an object is declared as `const`, the class member functions are not allowed to change any non-mutable member variables, and trying to do so will result in compilation errors.
When Not to Use `mutable`
There are prime scenarios where using `mutable` may lead to confusion or bugs:
- It should not be overused. Relying heavily on mutable states can complicate the design and make code harder to reason about.
- Avoid using `mutable` in cases where state changes do not logically belong to a constant operation. Keeping the class design clear and the interface consistent should always be a priority.
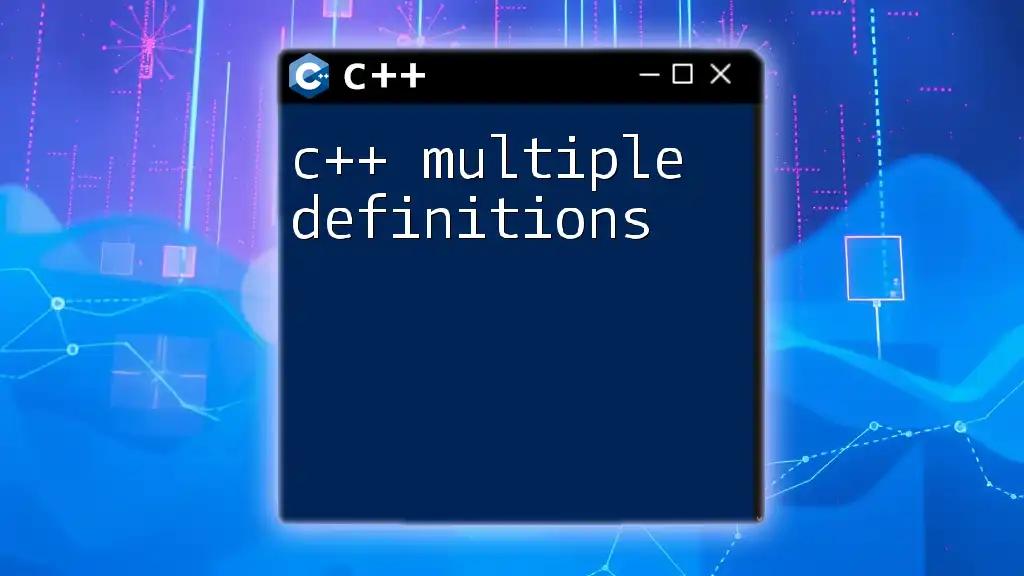
Performance Considerations
Impact on Performance
When considering performance, using `mutable` can lead to both positive and negative impacts. On the positive side, it can reduce overhead by avoiding unnecessary copies of data.
However, there can also be costs associated with readability and maintainability if the use of `mutable` leads to complicated code. Thus, it is essential to weigh the trade-offs of readability versus performance.
Benchmarking `mutable` Usage
To evaluate the performance implications effectively, one can set up benchmarks for performance metrics involving `mutable` usage. Here's a sample snippet of how you may approach this:
class Benchmark {
public:
mutable long operations;
void performOperation() const {
++operations; // Counter incremented in const context
}
};
In this `Benchmark` class, you can track how often a certain operation occurs, all while keeping the operation count modifiable even in a `const` context.

Conclusion
To recap, the `mutable` keyword in C++ provides an important mechanism for modifying class member variables in `const` contexts, enabling flexibility for specific use cases like caching and state management. Understanding when and how to use `mutable` effectively can enhance your C++ programming skills, allowing for more powerful and efficient class designs.
Further Reading and Resources
For those looking to delve deeper, consider exploring the official C++ documentation and resources about best practices with `mutable`. Additionally, practicing by implementing caching or state management mechanisms in your projects can solidify your understanding and proficiency with this feature.