A C++ mutex lock is a synchronization primitive that prevents multiple threads from simultaneously accessing shared resources, ensuring thread safety.
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void printThreadSafe(int id) {
mtx.lock(); // Lock the mutex
std::cout << "Thread " << id << " is executing.\n";
mtx.unlock(); // Unlock the mutex
}
int main() {
std::thread t1(printThreadSafe, 1);
std::thread t2(printThreadSafe, 2);
t1.join();
t2.join();
return 0;
}
Understanding the Need for Mutexes
What is a Race Condition?
In multithreaded programming, a race condition occurs when two or more threads attempt to modify shared data simultaneously, leading to unpredictable results. For example, consider a banking application where multiple threads are trying to update an account balance simultaneously. If these threads do not synchronize their operations, the final balance may reflect incorrect values, as incremental updates can overlap.
How Mutex Addresses Race Conditions
A mutex (short for mutual exclusion) acts as a locking mechanism that ensures only one thread can access a particular section of code or data at a time. By implementing a mutex, you can prevent race conditions by ensuring that one thread completes its work with the shared resource before another thread proceeds. This means that while one thread holds the mutex lock, other threads attempting to acquire the lock will be blocked until it is released.
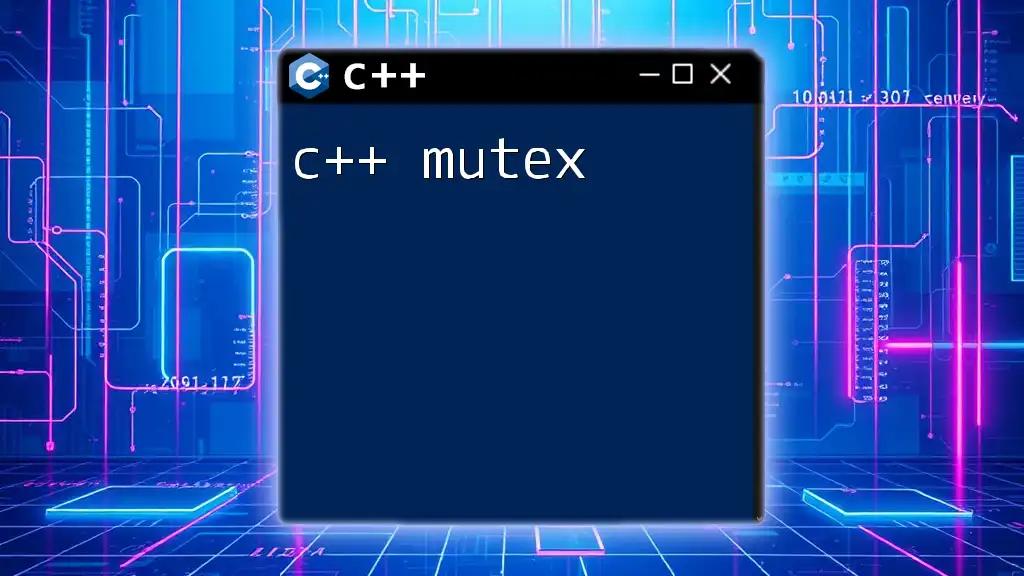
C++ Mutex Lock: Key Concepts
Types of Mutexes
C++ provides several types of mutexes that cater to different threading needs:
- Basic Mutex: `std::mutex` is the simplest form, providing exclusive access to a thread.
- Recursive Mutex: `std::recursive_mutex` allows the same thread to acquire the lock multiple times without causing a deadlock. This is useful for functions that may call themselves recursively.
- Timed Mutex: `std::timed_mutex` allows threads to attempt to acquire a lock for a specified amount of time before giving up, preventing indefinite blocking.
- Shared Mutex: `std::shared_mutex` permits multiple threads to read resources concurrently while allowing exclusive access for writing, facilitating read-heavy operations.
Basic Syntax and Operations
The basic operations associated with mutexes are straightforward. Below are the primary components used in C++ for working with mutexes, encapsulated in the `<mutex>` header.
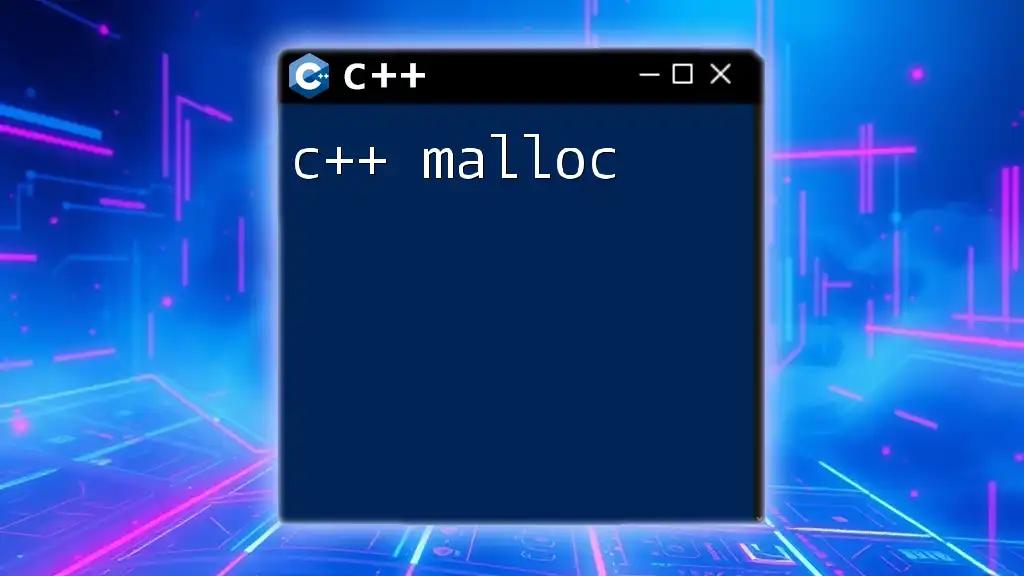
Implementing Mutex in C++
How to Create a Mutex Object
To utilize a mutex, you first need to create a mutex object. This can be done as follows:
#include <mutex>
std::mutex mtx; // Creating a mutex object
Creating a mutex is an essential first step that lets you manage concurrent access to shared resources throughout your application.
Basic Locking Mechanism
Locking a Mutex
Once you have created a mutex, the next step is to lock it. This operation is performed using the `lock()` method:
mtx.lock(); // Locking the mutex
When you call `lock()`, the calling thread gains exclusive access over the critical section of code. If another thread attempts to lock the same mutex, it will be blocked until the mutex is unlocked.
Unlocking a Mutex
After you're finished with the critical section, it is crucial to unlock the mutex:
mtx.unlock(); // Unlocking the mutex
Properly unlocking the mutex is vital, as it ensures that other threads can acquire the lock and prevents potential deadlocks.
Scoped Locking with std::lock_guard
One of the best practices for managing mutexes involves using `std::lock_guard`, which provides a way to automate the locking and unlocking process. It employs the RAII (Resource Acquisition Is Initialization) principle, where the mutex is automatically released when the lock guard goes out of scope.
Here’s an example:
#include <iostream>
#include <mutex>
std::mutex mtx;
void printThreadSafe() {
std::lock_guard<std::mutex> lock(mtx); // Automatically locks and unlocks
std::cout << "Thread Safe Output" << std::endl;
}
In the above example, `printThreadSafe()` ensures the mutex is automatically released once the function scope ends, dramatically reducing the risk of leaving a mutex locked inadvertently.
Advanced Locking with std::unique_lock
`std::unique_lock` provides more flexibility than `std::lock_guard`, as it allows you to unlock the mutex at any point during its lifetime without going out of scope. This is particularly useful when you may want to perform some operations without holding the lock.
Here is how you can employ `std::unique_lock`:
#include <iostream>
#include <mutex>
#include <thread>
std::mutex mtx;
void example() {
std::unique_lock<std::mutex> lock(mtx);
// Perform thread-safe operations
// lock can be released before going out of scope if needed
lock.unlock(); // Manually unlock if necessary
}
With `std::unique_lock`, you gain the ability to control the locking more granularly, improving overall flexibility in your multithreaded applications.

Best Practices for Using Mutex in C++
Avoiding Deadlocks
Deadlocks occur when two or more threads are waiting indefinitely for resources held by each other. One effective strategy to prevent deadlocks is using a lock hierarchy, where all threads acquire locks in a predefined order. This way, if multiple locks are required, all threads obtain them in the same sequence, minimizing the risk of circular waiting.
Using `std::lock` to acquire multiple mutexes simultaneously can also help prevent deadlocks by locking all required mutexes at once, ensuring that the threads do not get caught waiting for each other.
Minimizing Lock Duration
It’s crucial to keep the critical sections of your code as short and efficient as possible. By minimizing the duration of mutex locks, you reduce the chances of blocking other threads and improve the overall performance of your application. Only include the code that absolutely requires serialization within the locked scope.
Avoiding Unnecessary Locking
You should carefully evaluate when it is essential to use mutexes. Over-locking can lead to decreased performance and complicated designs. If a piece of code does not access shared resources, there's no need for a lock. Understanding the workload and context of your application can help you identify which operations truly warrant mutex protection.
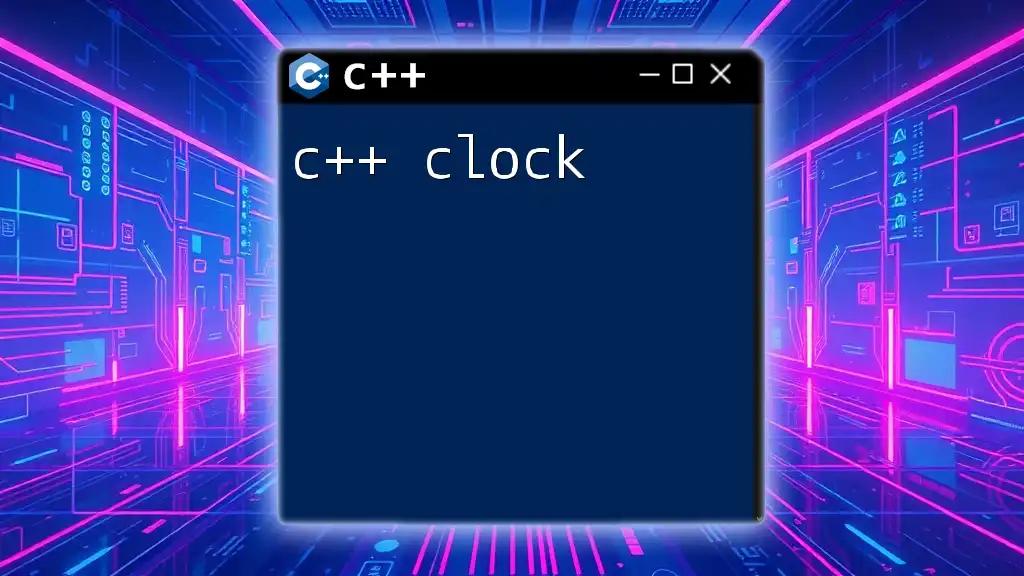
Debugging C++ Mutex Lock Issues
Common Issues with Mutex Locks
As you implement `c++ mutex lock` strategies, you may encounter certain issues such as race conditions and deadlocks. Identifying these problems early can save a lot of time down the line.
Race Conditions
Race conditions can often be discovered through thorough testing and debugging, by systematically testing numerous edge cases. Using tools like thread sanitizers helps identify conflicting access patterns while the program runs.
Deadlock Detection
Deadlocks may be more insidious and tougher to diagnose. Employing logging to trace which threads are waiting for locks can be a useful strategy. You can also use debugging tools that specialize in thread analysis, such as ThreadSanitizer.

Conclusion
Mutexes are a critical component of effectively managing concurrency in C++. Understanding and properly implementing `c++ mutex lock` techniques can save developers from potential pitfalls associated with multithreading, such as race conditions and deadlocks. By following the best practices outlined in this article, you can ensure that your multithreaded applications run smoothly and efficiently.
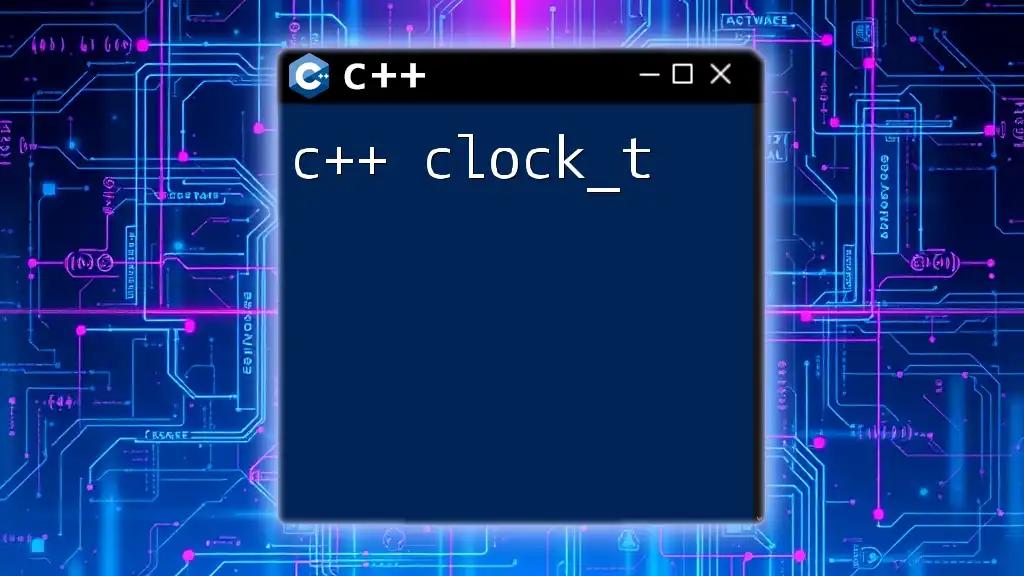
Call to Action
Now that you’re equipped with the knowledge of using mutexes in your C++ applications, try implementing these strategies in your own projects. Explore and experiment with the examples provided, and keep learning about more advanced topics in multithreading to further enhance your programming skills!