In C++, `realloc` is used to resize a previously allocated memory block while preserving its contents, but it is important to note that it is not standard in C++ and is primarily a C function.
#include <cstdlib>
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int));
for (int i = 0; i < 5; ++i) arr[i] = i;
// Resize the array to hold 10 integers
arr = (int*)realloc(arr, 10 * sizeof(int));
if (arr == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1;
}
for (int i = 0; i < 5; ++i) std::cout << arr[i] << " "; // Existing contents preserved
free(arr); // Always remember to free allocated memory
return 0;
}
Understanding Memory Management in C++
Importance of Dynamic Memory Allocation
In C++, memory management is a crucial aspect of efficient programming. When working with large data sets or structures whose size isn't known at compile time, dynamic memory allocation comes into play.
Dynamic memory allocation allows the allocation of memory during runtime using the heap, which is different from stack memory that is fixed in size and scope. Using dynamic allocation, you can create flexible programs that can gracefully handle varying input sizes.
What is `realloc`?
The `realloc` function is a powerful tool in the C++ memory management arsenal. It allows the programmer to resize an existing memory block that was previously allocated with `malloc`, `calloc`, or `realloc` itself.
Unlike simple allocation functions such as `malloc` and `calloc`, which only allocate a block of memory, `realloc` can both expand and reduce the size of the allocated memory. This feature ensures minimal memory waste and can significantly enhance program efficiency.
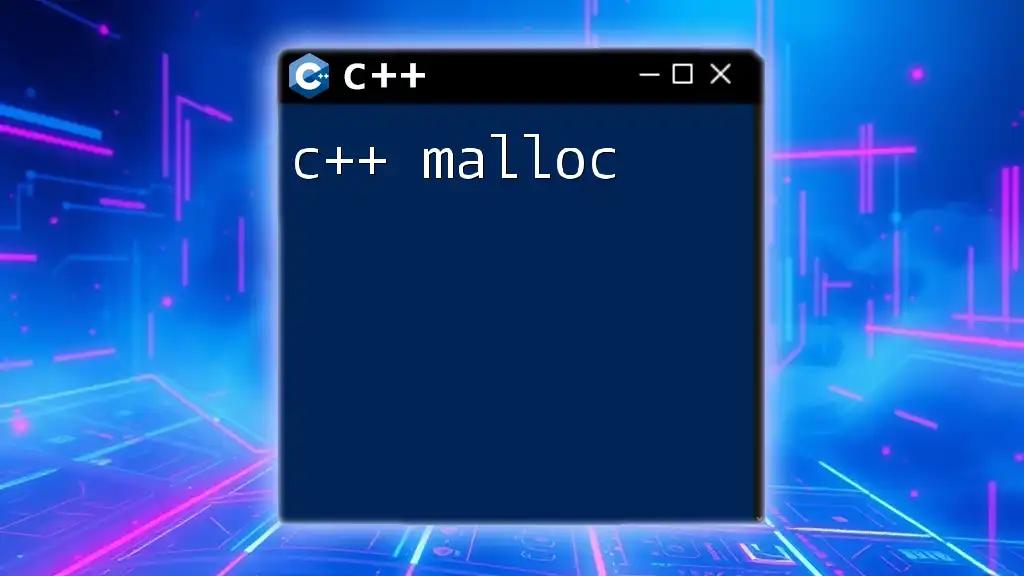
Syntax of `realloc`
Basic Syntax
The syntax for using `realloc` is as follows:
void* realloc(void* ptr, size_t newSize);
Parameters
-
`ptr`: This is a pointer to the memory block that was previously allocated. If this pointer is `NULL`, `realloc` behaves like `malloc`, allocating a new block of memory.
-
`newSize`: This indicates the desired size for the new memory block. If `newSize` is zero, the previously allocated memory block is freed.
Understanding how these parameters interact is essential for effective memory management.
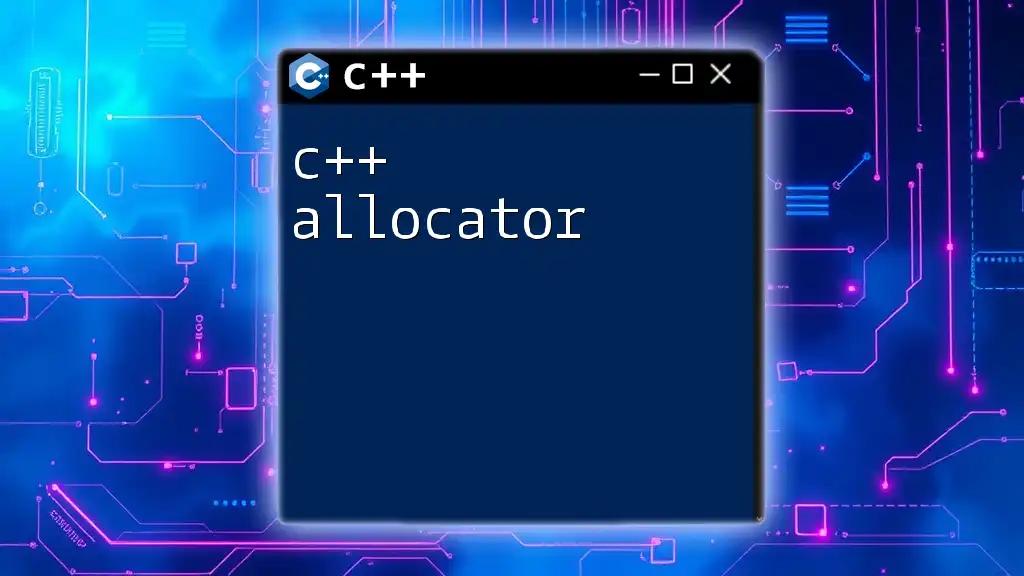
How `realloc` Works
Reallocation Process
When you call `realloc`, it attempts to resize the existing memory block pointed to by `ptr`. If the memory can be expanded in place, `realloc` returns a pointer to the same block. However, if the requested size is larger and cannot be accommodated, `realloc` may allocate a new memory block, copy the existing data to the new block, and free the old block.
The behavior of `realloc` can be summarized as follows:
- If reallocating to a larger size is possible within the current memory block, it will do so.
- If reallocation fails, the original memory block remains unchanged, which means data integrity is preserved.
- When the new size is smaller than the old size, only the beginning portion of the data is kept.
Memory Alignment
Memory alignment refers to how data types are aligned in memory, often dictated by the system architecture. `realloc` ensures that the new memory block maintains proper alignment, which can be crucial for performance optimization in C++.
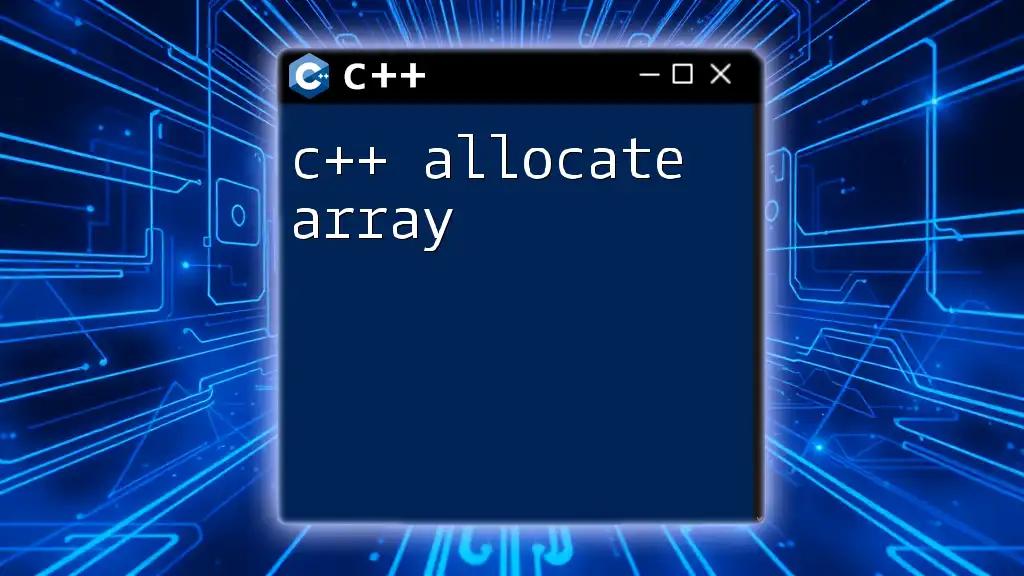
Using `realloc` in C++
Example 1: Basic Usage of `realloc`
Here’s a simple example that illustrates the basic usage of `realloc`:
#include <cstdlib>
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int));
// Populate the array
for (int i = 0; i < 5; i++) {
arr[i] = i + 1;
}
// Reallocating memory
arr = (int*)realloc(arr, 10 * sizeof(int));
// Populate the new array
for (int i = 5; i < 10; i++) {
arr[i] = i + 1;
}
// Displaying the array
for (int i = 0; i < 10; i++) {
std::cout << arr[i] << " ";
}
free(arr);
return 0;
}
In this example, we first allocate memory for an array of five integers and populate it. When we call `realloc`, we expand the array to hold ten integers. After population, we print the contents of the array.
The expected output would show the integers from 1 to 10, confirming the successful resizing of the memory block.
Example 2: Handling Reallocation Failure
Proper error-handling when using `realloc` is vital to avoiding memory leaks and ensuring stability. Here is how you can effectively handle potential failures:
#include <cstdlib>
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int));
// Check if memory allocation was successful
if (!arr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1;
}
// Reallocating
int* newArr = (int*)realloc(arr, 100 * sizeof(int));
if (!newArr) {
std::cerr << "Memory reallocation failed!" << std::endl;
free(arr); // Free the original array
return 1;
}
arr = newArr; // Update pointer only if realloc was successful
free(arr);
return 0;
}
In this example, we first check if the allocation was successful. When reallocation takes place, we further confirm with another check. If `realloc` returns `NULL`, we are careful to free the original memory to prevent leaks.
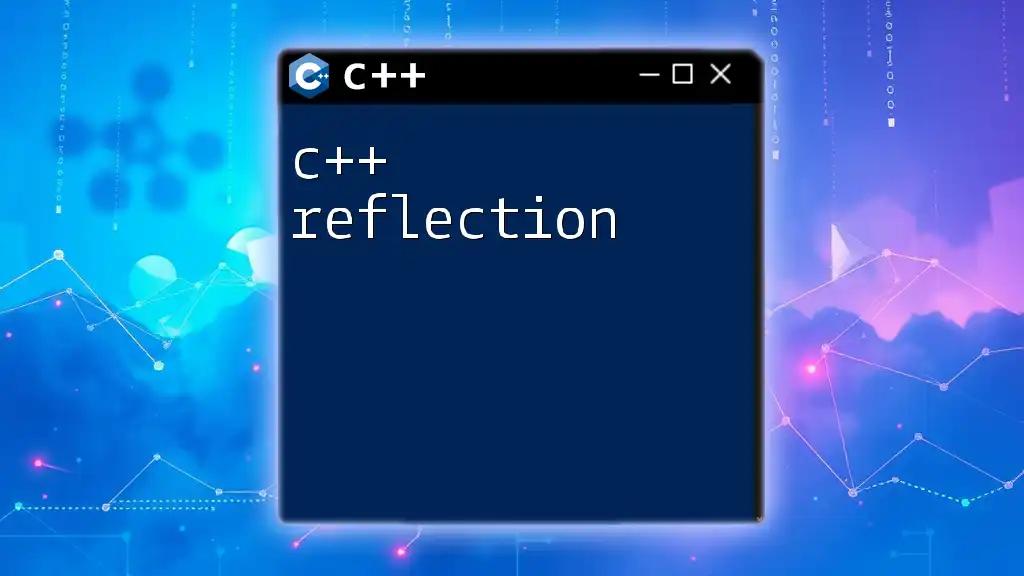
Best Practices for Using `realloc`
When to Use `realloc`
Consider using `realloc` when you need to dynamically resize an array or data structure that can grow or shrink based on runtime conditions. It allows you to manage memory efficiently without manually allocating and deallocating memory repeatedly.
Avoiding Memory Leaks
Memory leaks can be detrimental to performance, leading to inefficient use of resources. Always ensure that any memory you allocate is eventually freed. Use tools like Valgrind to detect memory leaks during testing.
Debugging `realloc` Issues
Common pitfalls with `realloc` can include improper checking of returned pointers and failing to release memory upon errors. To debug realloc issues:
- Use assertions to validate memory pointers.
- Log the sizes being requested and any failures.
- Always set pointers to `NULL` after freeing them to avoid dangling references.
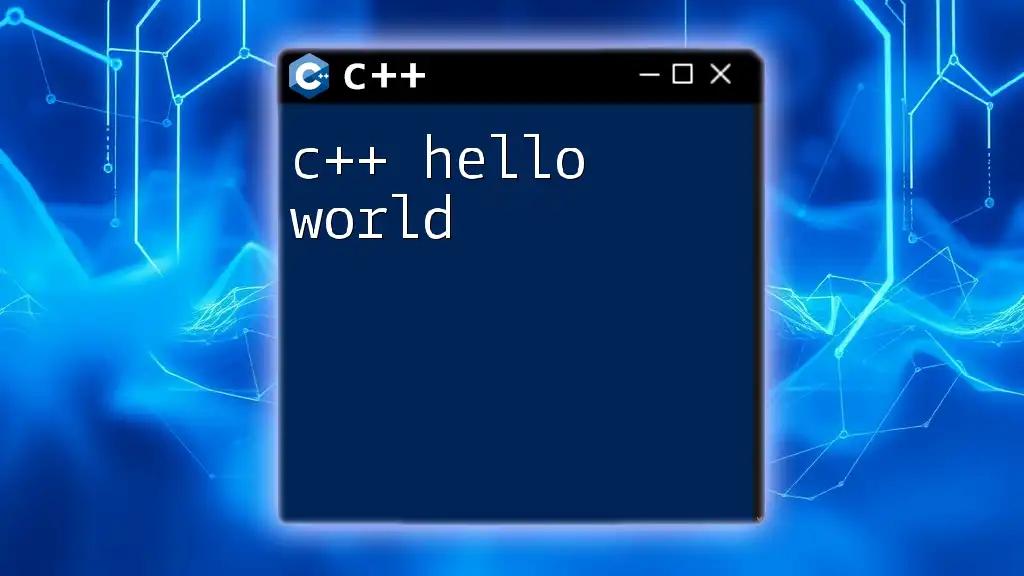
Conclusion
In summary, the `realloc` function is a vital component of memory management in C++. Understanding its syntax, functionality, and best practices can significantly enhance your programming capabilities and ensure efficient memory use. As dynamic memory allocation becomes an integral part of effective C++ programming, it’s essential to practice these concepts and integrate them into your projects.
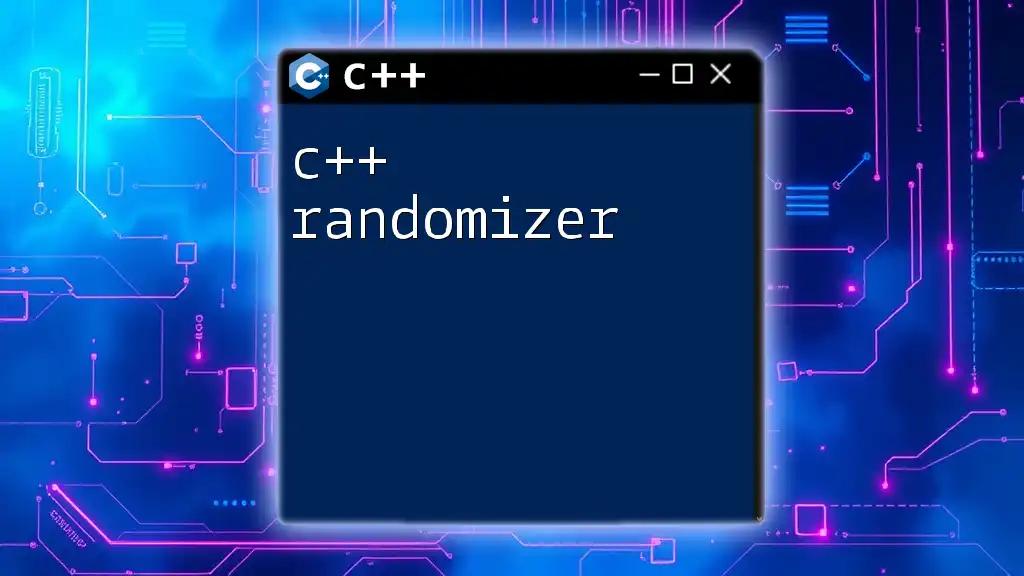
Further Reading
To master dynamic memory management in C++, consider delving into authoritative books, comprehensive tutorials, and hands-on online courses that explore advanced topics. Engaging with practical exercises will solidify your mastery over memory management techniques in C++.
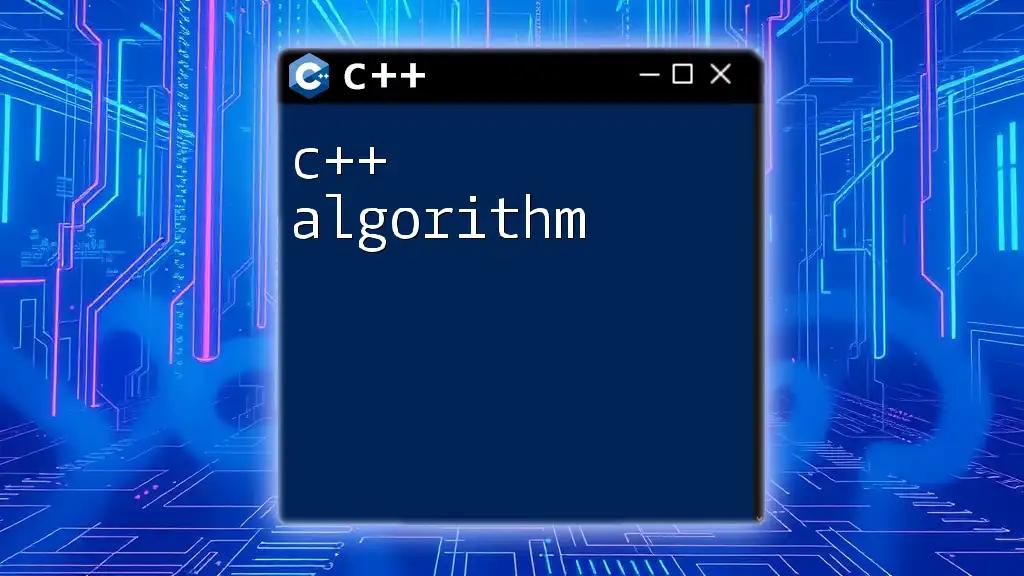
FAQs about `realloc`
What should I do if I forget to check if `realloc` returns NULL?
If you neglect to check if `realloc` returns `NULL`, you might attempt to use a null pointer, leading to undefined behavior. Always implement error checking after any memory allocation or reallocation.
Can `realloc` resize to a smaller size?
Yes, `realloc` can resize to a smaller size. This can free up memory that is no longer needed while keeping the initial data intact up to the new limit.
Is `realloc` thread-safe?
`realloc` itself is not inherently thread-safe. The behavior can depend on the underlying implementation and system. When using `realloc` in a multi-threaded program, ensure that proper synchronization techniques are utilized to prevent concurrent access issues.