In C++, a lock is used to ensure that only one thread accesses a shared resource at a time, preventing data races and ensuring thread safety.
Here's a simple code snippet demonstrating the use of `std::lock_guard` for locking:
#include <iostream>
#include <mutex>
#include <thread>
std::mutex mtx; // mutex for critical section
void printThreadId(int id) {
std::lock_guard<std::mutex> lock(mtx); // lock the mutex
std::cout << "Thread ID: " << id << std::endl;
}
int main() {
std::thread t1(printThreadId, 1);
std::thread t2(printThreadId, 2);
t1.join();
t2.join();
return 0;
}
Understanding Concurrency in C++
What is Concurrency?
Concurrency refers to the ability of a system to handle multiple tasks at once, which often leads to increased efficiency in program execution. It’s essential to differentiate concurrency from parallelism: while concurrency allows the overlapping of tasks, parallelism entails executing multiple tasks simultaneously. In a multi-threaded environment, multiple threads share resources, such as memory space. However, this leads to potential issues such as race conditions, where the output of a process can depend on the unpredictable timing of threads.
Why Use Locks?
Locks are critical in ensuring data consistency in multi-threaded applications. When multiple threads access shared resources without proper synchronization, data corruption or inconsistencies can occur. Using a lock mechanism allows one thread to access a resource while preventing others from doing so, thereby safeguarding the integrity of the data.
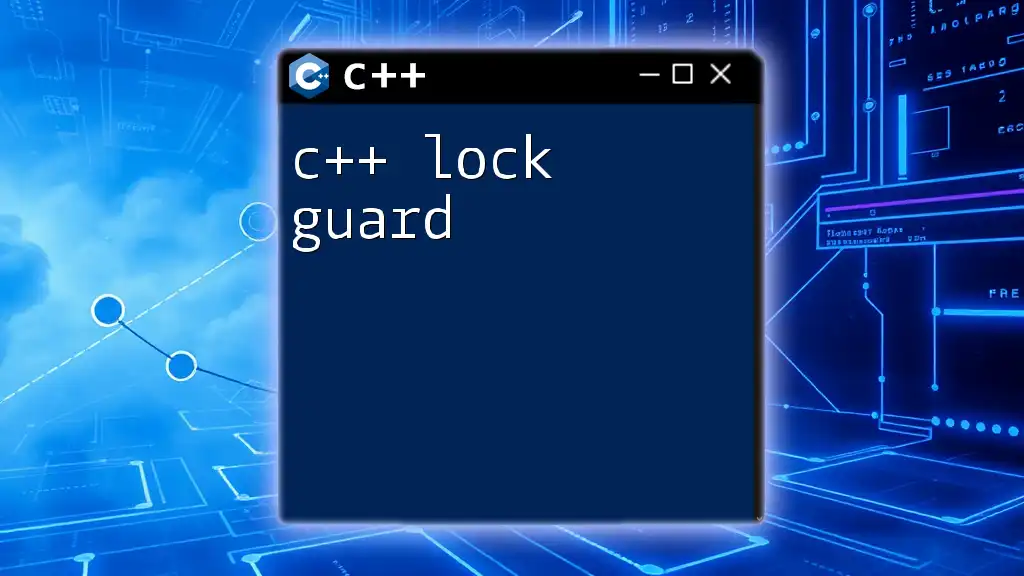
Types of Locks in C++
Mutex and Locking Mechanisms
A mutex, or mutual exclusion, is one of the fundamental locking objects in C++. It ensures that only one thread can access a resource at a time.
std::mutex
The basic locking mechanism provided by C++ is `std::mutex`. Here’s how to use it effectively:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // mutex for critical section
void print_block(int n, char c) {
mtx.lock(); // Locking the mutex
for (int i = 0; i < n; ++i) {
std::cout << c;
}
std::cout << "\n";
mtx.unlock(); // Unlocking the mutex
}
int main() {
std::thread t1(print_block, 50, '*');
std::thread t2(print_block, 50, '$');
t1.join();
t2.join();
return 0;
}
In this code snippet, `std::mutex` is used to control access to a console output section, ensuring that both threads do not interrupt each other, resulting in a clean output.
std::recursive_mutex
For cases where a thread needs to lock a mutex multiple times without blocking itself, `std::recursive_mutex` is the ideal choice. It allows re-entrant locking by the same thread.
std::timed_mutex
In scenarios where you want to attempt to lock without waiting indefinitely, `std::timed_mutex` can be used. This allows a thread to acquire the lock within a specified time frame.
std::shared_mutex
Introducing `std::shared_mutex` offers a more sophisticated approach by allowing multiple readers or a single writer. This mechanism is critical for applications that are read-heavy, as it lets concurrent read access while still preventing write contention.
#include <iostream>
#include <thread>
#include <shared_mutex>
std::shared_mutex rw_mutex;
void read_data(int id) {
rw_mutex.lock_shared();
std::cout << "Reader " << id << " is reading.\n";
rw_mutex.unlock_shared();
}
void write_data(int id) {
rw_mutex.lock();
std::cout << "Writer " << id << " is writing.\n";
rw_mutex.unlock();
}
int main() {
std::thread readers[5];
std::thread writer(write_data, 1);
for (int i = 0; i < 5; ++i) {
readers[i] = std::thread(read_data, i);
}
writer.join();
for (auto& r : readers) {
r.join();
}
return 0;
}
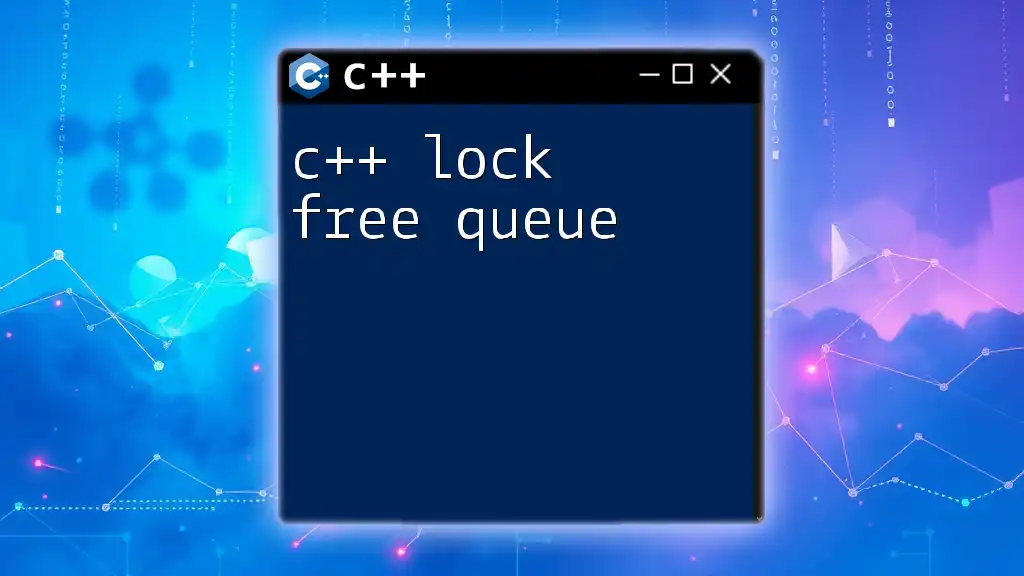
Advanced Locking Mechanisms
Condition Variables
Condition variables enable threads to wait until they are notified to proceed. They are particularly useful for producer-consumer problems where one thread produces data that another needs to consume.
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void print_id(int id) {
std::unique_lock<std::mutex> lck(mtx);
while (!ready) cv.wait(lck);
std::cout << "Thread " << id << " is ready\n";
}
void go() {
std::lock_guard<std::mutex> lck(mtx);
ready = true;
cv.notify_all();
}
int main() {
std::thread threads[10];
for (int i = 0; i < 10; ++i) threads[i] = std::thread(print_id, i);
std::cout << "10 threads ready to race...\n";
go(); // GO signal!
for (auto& th : threads) th.join();
return 0;
}
Lock Guard
Utilizing a lock guard, specifically `std::lock_guard`, simplifies your locking strategy by ensuring that the mutex is automatically released when the guard object goes out of scope. This minimizes the risk of forgetting to unlock a mutex and helps in maintaining cleaner code.
void safe_print(int n) {
std::lock_guard<std::mutex> lock(mtx);
std::cout << "Thread " << n << " is running safely.\n";
}
Unique and Shared Locks
std::unique_lock and std::shared_lock are valuable when you need more control over locks. They provide flexibility in lock ownership and the ability to defer locking. A `std::shared_lock` enables multiple threads to read data while exclusive writing is prevented.
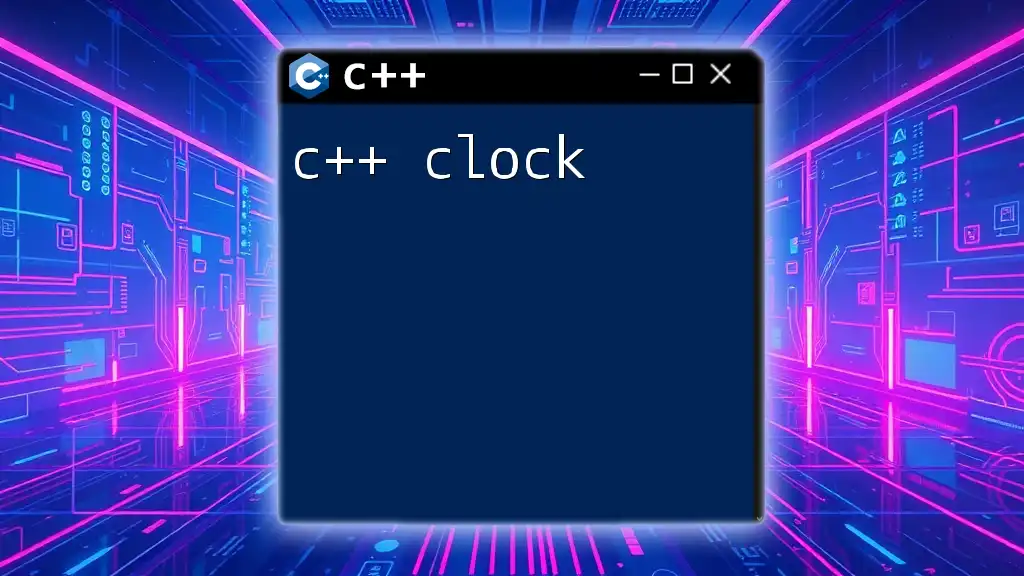
Best Practices for Using Locks
Choosing the Right Locking Mechanism
In deciding which locking mechanism to use, consider the nature of the operations performed. A mutex should suffice for most cases, but if your application has many read operations and few write operations, a shared_mutex could offer performance benefits.
Minimizing Lock Contention
Lock contention can severely degrade performance. Strategies such as minimizing the duration of locked sections, breaking tasks into smaller, less-locked segments, and using concurrent data structures can effectively reduce this issue.
Avoiding Deadlocks
Deadlocks occur when two or more threads are waiting for each other to release locks, leading them to wait indefinitely. To prevent deadlocks:
- Ensure that all threads acquire locks in a consistent order.
- Use try-lock mechanisms to attempt to lock without waiting.
- Implement timeout conditions to handle prolonged waits.
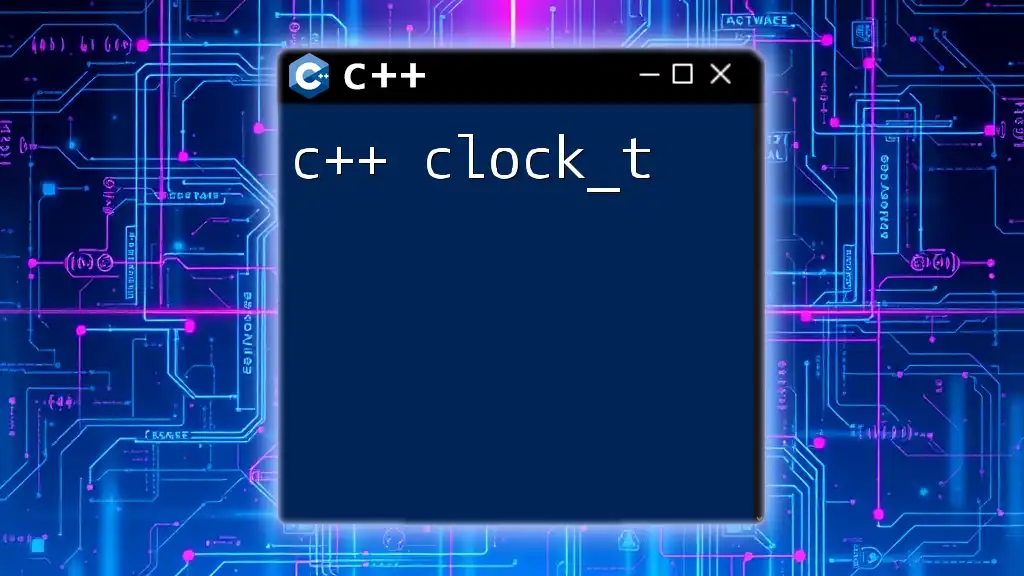
Performance Implications of Locks
Using locks inevitably comes with performance trade-offs. Locking introduces overhead, delays, and context switching, all of which can impact throughput. Profiling your multi-threaded application to find potential bottlenecks is crucial for improving performance.
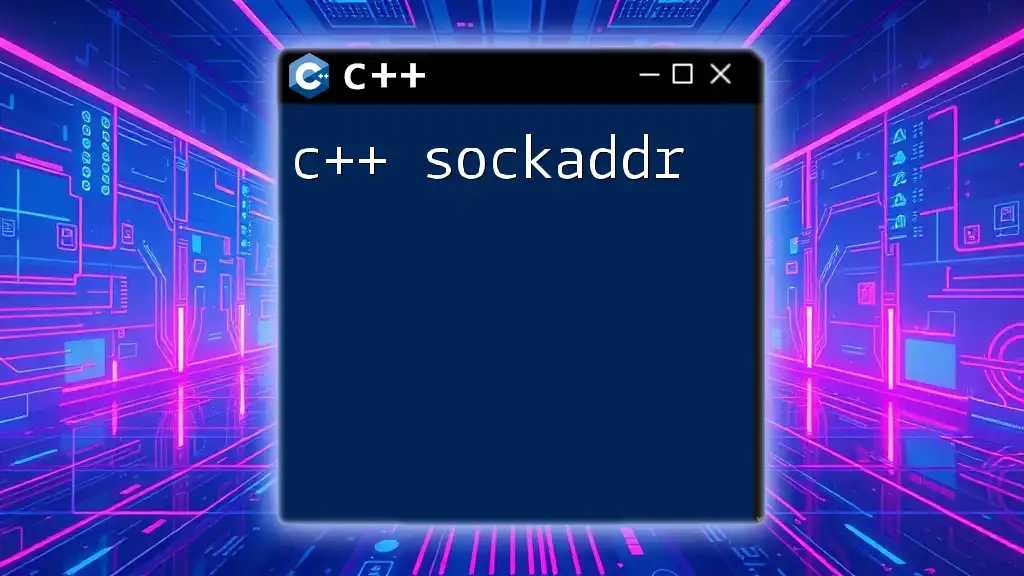
Conclusion
To summarize, a C++ lock is a fundamental aspect of multi-threaded programming that ensures safe access to shared resources. Understanding the various locking mechanisms, their appropriate use cases, and best practices enhances the ability to build robust and efficient C++ applications. Continuous learning and exploration of advanced synchronization primitives will only serve to strengthen your programming toolset.
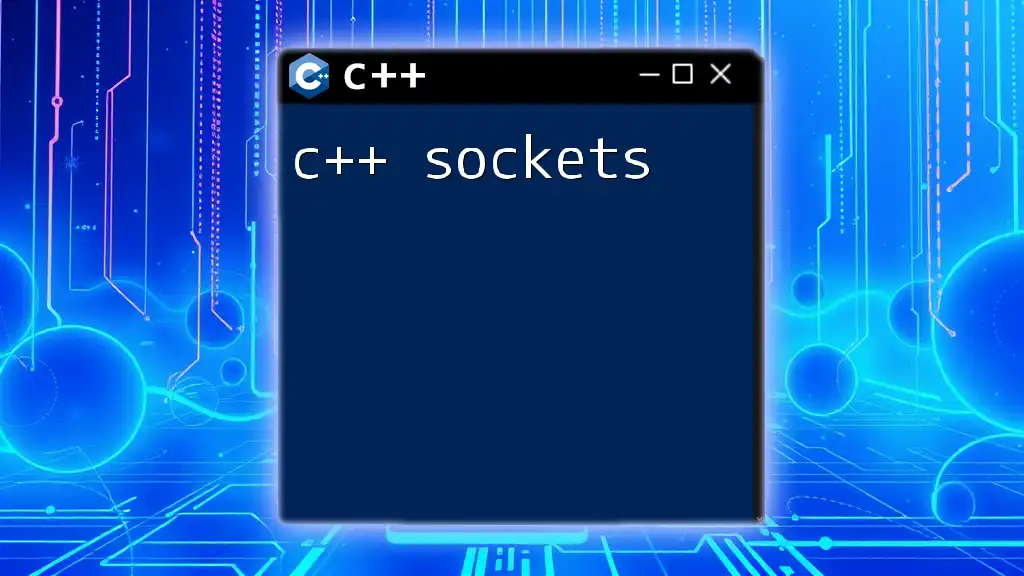
FAQs About C++ Locks
Common questions often revolve around the differences in lock types, the necessity of locking mechanisms, and their performance impacts. It's essential for developers to clarify these points to proceed with confidence in their multi-threaded programming endeavors. Exploring community forums and documentation can provide further insights into advanced use cases and solutions.