In C++, a block comment is used to comment out multiple lines of code or text, beginning with `/` and ending with `/`, allowing programmers to describe or disable parts of their code without affecting execution.
Here’s a code snippet demonstrating a block comment:
/* This is a block comment.
It can span multiple lines. */
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Block Comment?
A block comment in C++ is denoted by the syntax `/* comment text here */`. This allows programmers to include longer sections of commentary in their code, spanning multiple lines. The primary purpose of comments is to enhance the readability of the source code by providing informative annotations.
Purpose and Benefits of Using Block Comments
Using block comments helps to clarify the intent of the code, making it easier for others (or even the original programmer) to understand what specific sections of the code are doing. They are particularly useful in larger files where providing context is essential.
Block comments can be beneficial in the following ways:
- They improve code readability and maintainability.
- They assist in explaining complex logic which might not be immediately clear.
- They facilitate debugging by allowing developers to temporarily disable sections of code while still retaining descriptive text about that code.
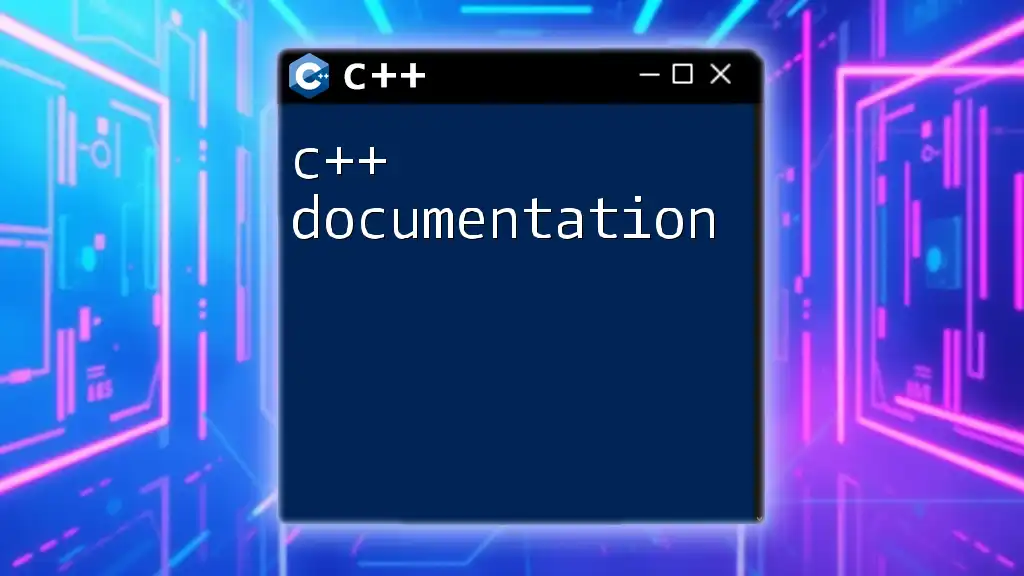
Syntax of Block Comments in C++
How to Write a Block Comment
Writing a block comment is straightforward. You start with `/` and finish with `/`, encompassing any text you want to include. Here’s a simple example:
/* This is a simple block comment in C++ */
int a = 5; // The variable 'a' is initialized to 5
Differences Between Block Comments and Line Comments
C++ supports two types of comments: block comments (`/* ... */`) and line comments (`//`). While both serve to document the code, they have different use cases.
- Block Comments can span multiple lines, making them ideal for detailed descriptions of functions or segments of code.
- Line Comments are typically used for small notes and annotations that fit on a single line.
Here’s a comparison of the two:
// This is a line comment
int b = 10;
/* This is a block comment
spanning multiple lines
*/
int c = 20;
Understanding when to use each type is critical. Line comments are often more convenient for brief notes and reminders, while block comments provide a clearer option for longer explanations.
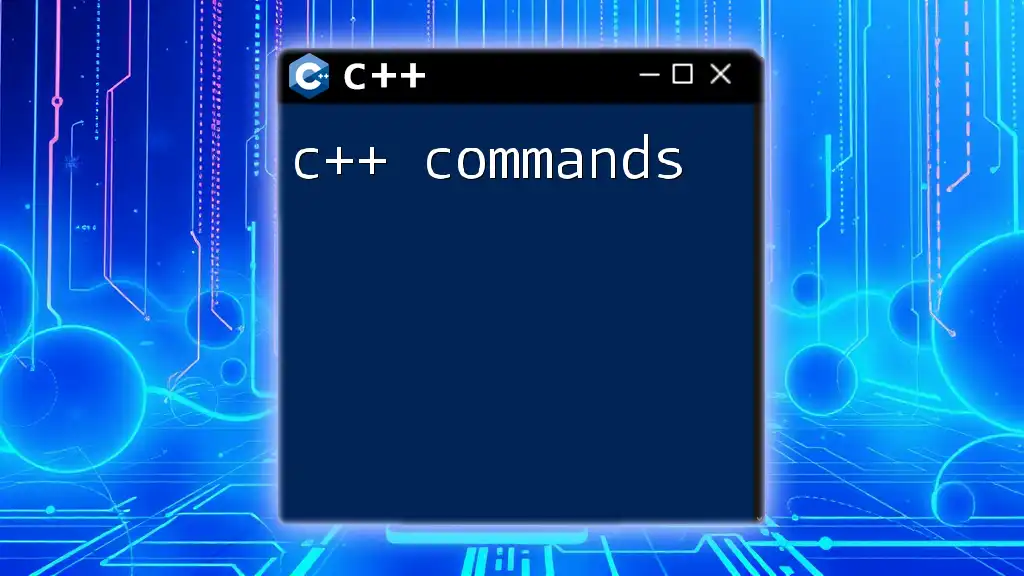
When to Use Block Comments in C++
Best Practices for Using Block Comments
Knowing when to utilize block comments is just as important as knowing how to write them. Here are situations where block comments excel:
- When elaborating on complex logic or algorithms that require detailed explanation.
- To summarize the purpose of a large section of code, such as a full function or a set of related functions.
- When providing context for settings or variables that have significant implications in the code’s execution.
Commenting Functions or Code Blocks
Block comments are particularly useful when documenting functions. A well-placed block comment can significantly aid anyone reading the code later. For instance:
/* This function calculates the factorial of a number
using recursion. It returns -1 for negative inputs. */
int factorial(int n) {
if (n < 0)
return -1; // Error case
return (n == 0) ? 1 : n * factorial(n - 1);
}
In this example, the block comment succinctly explains what the function does, making it easier to understand its purpose immediately.
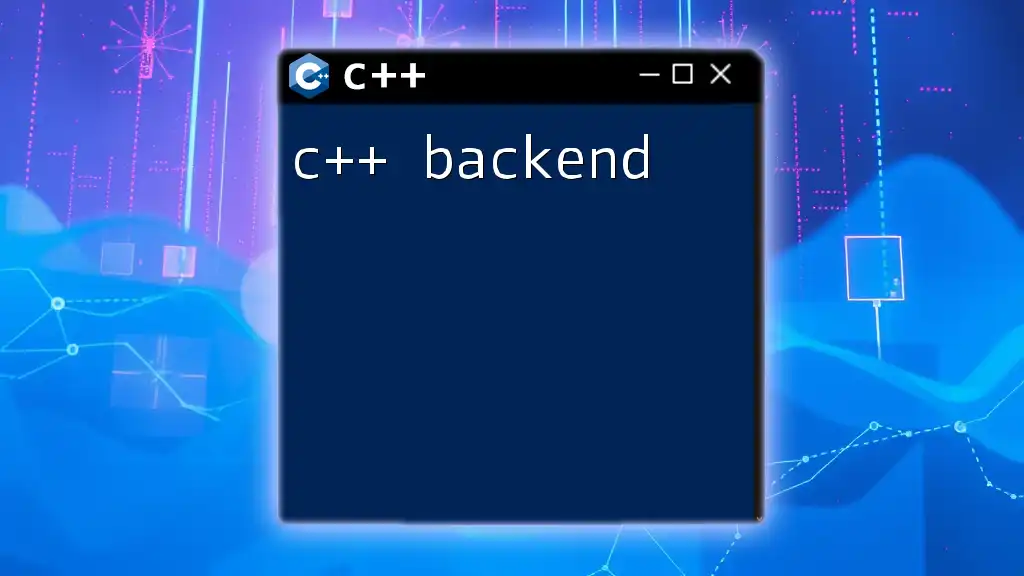
Common Mistakes with Block Comments
Forgetting to Close Block Comments
One of the prevalent issues when using block comments is forgetting to close them properly. This can lead to compiler errors since the code following an unclosed comment will be rendered inactive.
For example:
/* This is an unclosed block comment
int d = 30; // This line would cause a compilation error
This situation will result in a compilation error, as the compiler cannot determine where the block comment ends.
Overusing Block Comments
Another mistake is the tendency to overuse block comments, leading to confusion rather than clarity. Striking a balance is key—too many comments can clutter the code, making it harder to read.
Aim for concise and relevant comments. For instance, instead of writing:
/* This modifies the value */
/* This changes the maximum value allowed for the user input */
Consider something clearer yet brief:
/* Adjusting max user input to prevent overflow */
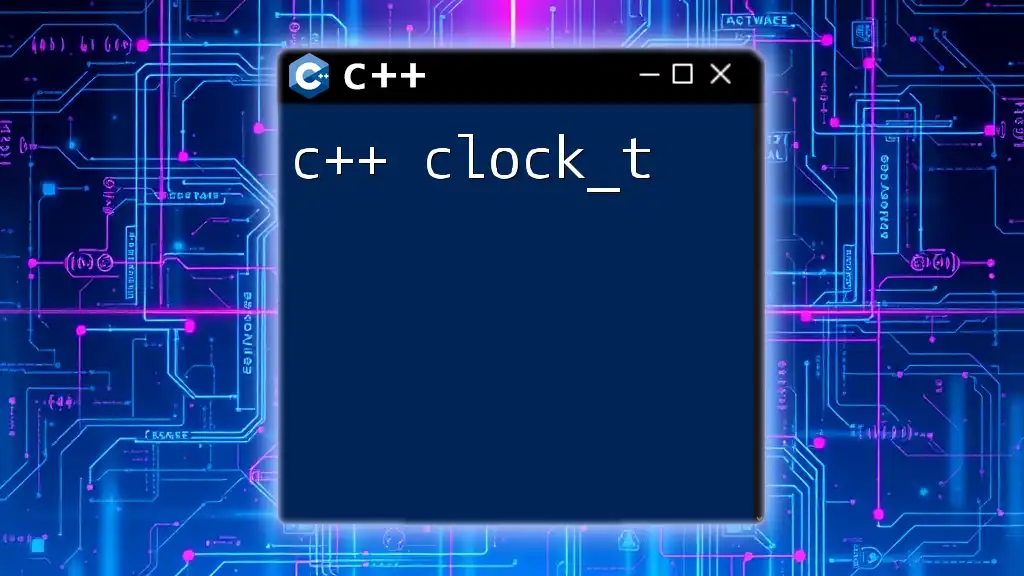
Best Practices for Writing Clear and Effective Block Comments
Clarity and Brevity
When writing block comments, strive for clarity and brevity. The goal is to enrich the code with context without overwhelming it with jargon. Lengthy and confusing comments can be just as problematic as unclear code. Here’s a comparison:
/* This modifies the value */ // Vague comment
/* Adjust the maximum allowed user input to prevent overflow */ // Clear comment
The second comment gives precise information while expressing clear intent.
Consistency in Commenting Style
Consistency in style helps enhance code maintainability. Following predefined conventions for comments can avoid ambiguity. When possible, keep a style guide and ensure all team members adhere to it. This is especially important in larger projects where multiple developers may contribute.
Use of Fundamentals: Formatting and Style
Effective use of formatting in block comments can significantly improve readability. A common practice is to use leading asterisks for multi-line comments, which visually separates them from the code:
/*
* Calculate the square of a number
* - Input: an integer value
* - Output: the square of the input
*/
int square(int input) {
return input * input;
}
By formatting in this manner, you can ensure that comments are easily distinguishable, thereby enhancing the overall readability of the source code.
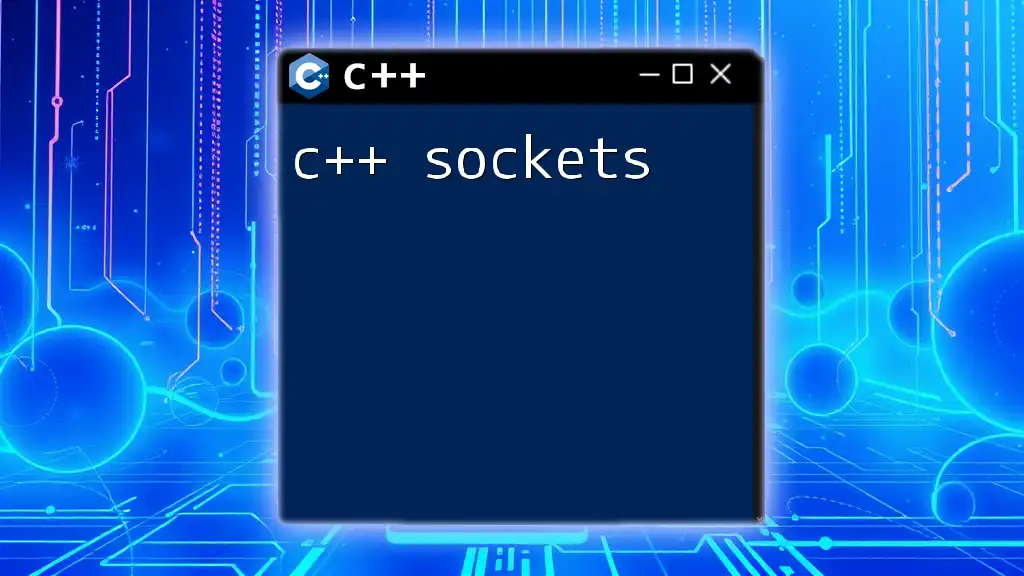
Tools and Resources to Enhance Commenting Practices
Code Linters and Style Checkers
To promote best practices in comment writing and code overall, consider using code linters and style checkers. Tools such as `cpplint` and `ClangFormat` help identify improper commenting styles and enforce consistency throughout the codebase.
Learning Resources
For those looking to improve their commenting skills in C++, various resources are available:
- Recommended books and guides focused on C++ best practices.
- Online courses that cover programming principles, emphasizing code documentation.
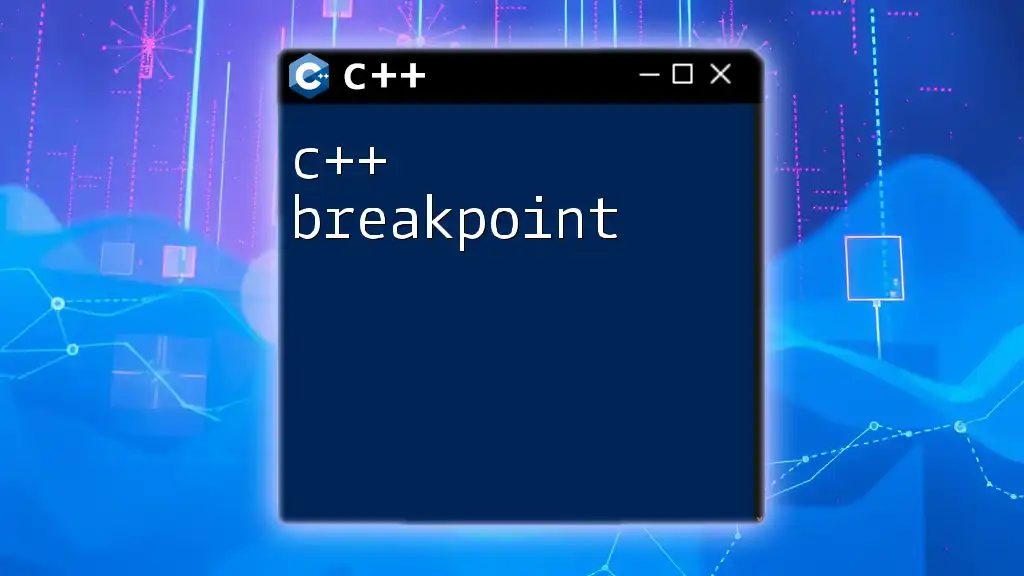
Conclusion
In summary, understanding and effectively using C++ block comments is crucial in fostering code clarity and maintainability. By employing these comments judiciously, you ensure that your code remains readable and its intent clear, benefitting both current and future developers who may work on the same codebase.
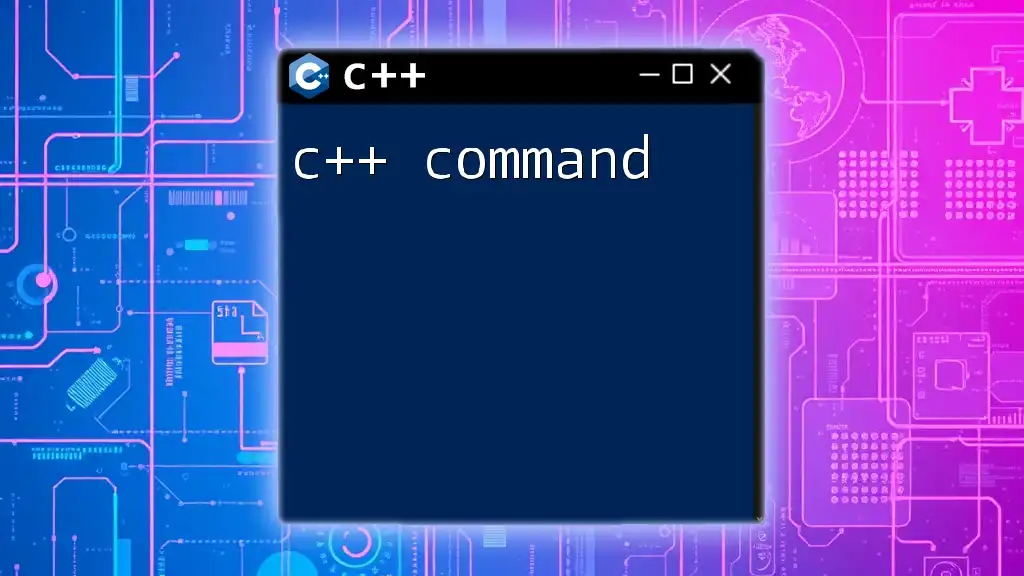
FAQs About C++ Block Comments
What is a block comment in C++?
A block comment is a multi-line comment that can span several lines, indicated by the syntax `/* ... */`.
Can block comments be nested?
No, block comments cannot be nested in C++. The inner comment will not be recognized if it is placed within an existing block comment.
Are there performance implications of excessive comments?
While comments themselves don’t directly affect performance, having too many comments may make code harder to read and maintain, eventually leading to errors or oversights in logic. Thus, clarity and judicious use of comments are key.