C++ commands are the instructions and syntax used in C++ programming to perform specific actions, such as declaring variables or controlling program flow. Here's an example of a simple command to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Commands
What are C++ Commands?
C++ commands are the instructions that you issue within the C++ programming language to perform specific tasks. They allow you to control the flow of execution, manage data, and achieve desired outcomes within your programs. Understanding how these commands work is essential for writing efficient and maintainable code.
Unlike functions that are pre-defined blocks of code designed to perform specific tasks, C++ commands can be thought of as the building blocks that dictate how your program operates. Mastering these commands will give you a foundation upon which to build more complex programs and systems.
Types of C++ Commands
C++ commands can be categorized into several types, primarily focusing on their functionality. Some key categories include:
- Control Flow Commands: Manage the execution path of code.
- Input/Output Commands: Facilitate data exchange between the program and the user or files.
- Memory Management Commands: Handle dynamic memory allocation and deallocation.
- Data Structure Commands: Enable manipulation of collections of data.

Setting Up Your C++ Environment
Choosing a Compiler
To work with C++, you'll need a compiler to translate your code into executable programs. Popular compilers such as GNU Compiler Collection (GCC), Clang, and Microsoft Visual C++ (MSVC) offer varying features and performance levels. Your choice should depend on your system, personal preferences, and specific project requirements.
Writing Your First C++ Command
Once you have set up your environment and chosen a compiler, you can write your first C++ command. Here’s a step-by-step guide to compiling and running a simple program:
- Open a text editor and create a new file named `hello.cpp`.
- Enter the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Save the file and compile it using your chosen compiler, for example:
g++ hello.cpp -o hello
- Run the program:
./hello
Your terminal should display "Hello, World!" confirming that your first C++ command was successful!
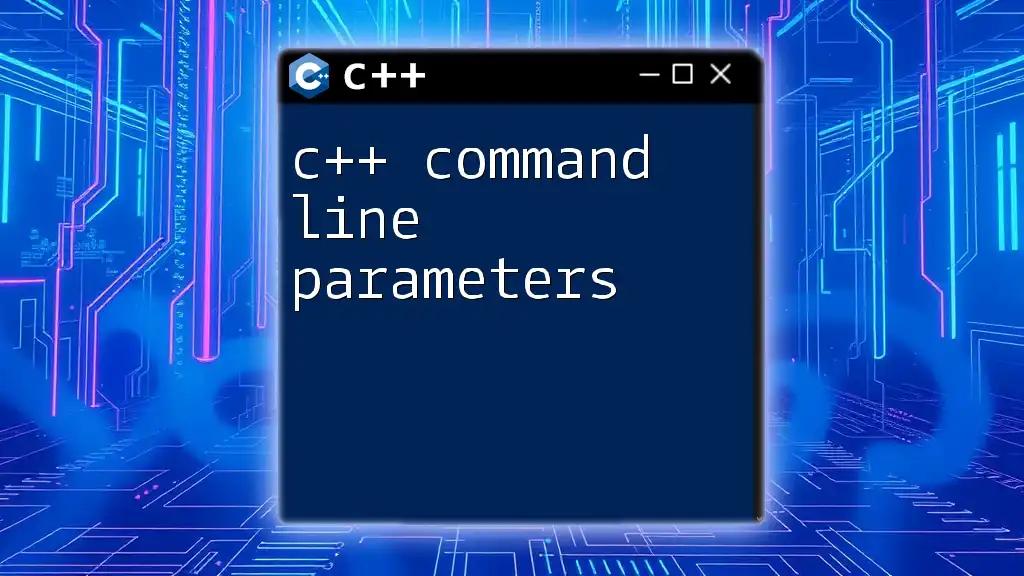
Key C++ Commands
Control Flow Commands
If-Else Statements
If-else statements allow you to execute different blocks of code based on certain conditions, providing a straightforward way to implement decision-making in your program.
Here’s an example illustrating the use of an if-else statement:
int number = 10;
if (number > 0) {
std::cout << "Number is positive" << std::endl;
} else {
std::cout << "Number is not positive" << std::endl;
}
This block of code checks if `number` is greater than zero. If true, it prints "Number is positive"; otherwise, it prints "Number is not positive". Understanding the logic flow is vital in creating responsive and interactive applications.
Switch Statements
A `switch` statement provides a more simplified syntax for evaluating multiple conditions based on variable values, making it easier to maintain and read.
Here is how you can use a switch statement:
int day = 5;
switch (day) {
case 1: std::cout << "Monday"; break;
case 2: std::cout << "Tuesday"; break;
default: std::cout << "Another day"; break;
}
In the example, if `day` equals 1, it prints "Monday". If it equals 2, it prints "Tuesday". For any other value, it defaults to "Another day". A switch statement is particularly useful when dealing with multiple explicit conditions.
Input/Output Commands
Using cin and cout
C++ has a robust system for handling input and output, primarily through `std::cin` and `std::cout`. These allow you to receive input from the user and display output effectively.
Here’s how to use `cout` and `cin` for simple user interaction:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
std::cout << "You are " << age << " years old." << std::endl;
In this snippet, the program prompts the user to enter their age and then outputs what the user entered. Using streams allows seamless user interaction and enhances the program's usability.
Memory Management Commands
New and Delete
Dynamic memory allocation in C++ can be achieved through the `new` and `delete` commands, allowing you to create and destroy objects at runtime.
For example:
int* ptr = new int;
*ptr = 25;
std::cout << *ptr << std::endl;
delete ptr;
In this code:
- `new` allocates memory for a variable of type `int`.
- `*ptr = 25;` assigns a value to the dynamically allocated memory.
- `delete ptr;` frees the allocated memory, preventing memory leaks.
Understanding how to manage memory effectively is crucial in creating efficient programs.
Data Structure Commands
Using Arrays
Arrays are a fundamental data structure in C++ that allow you to store multiple items of the same type under a single name. Here’s how to declare and use an array:
int numbers[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << " ";
}
In this example, we create an array `numbers` and use a loop to print its elements. Arrays are essential for managing collections of data efficiently.
Vectors from Standard Template Library (STL)
Vectors, a part of the STL, provide a dynamic array that can grow and shrink in size. They are more versatile than traditional arrays, making them a preferred choice in many situations.
Here’s how you can use a vector:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int num : vec) {
std::cout << num << " ";
}
The vector automatically manages memory for you, making it easier to work with collections of data while providing features like dynamic sizing and built-in methods for data manipulation.
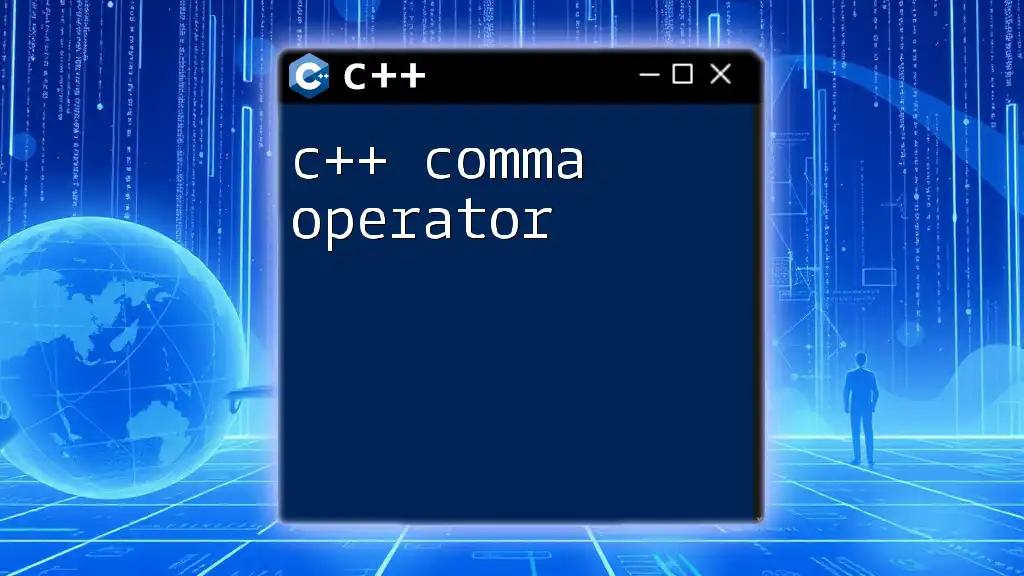
Advanced C++ Commands
Lambda Functions
Lambda functions are a powerful feature in C++ that allow you to create anonymous functions on the fly. They’re particularly useful for functions that are passed as parameters or used in algorithms.
Here’s a simple lambda example:
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Outputs 8
In this code, we've defined a lambda that takes two integers and returns their sum, showcasing the flexibility and expressiveness which lambda functions bring to the table.
Exception Handling
C++ allows for exception handling through `try`, `catch`, and `throw` commands, enabling you to create robust programs that can gracefully handle errors and unexpected behavior.
Here’s an example of exception handling:
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
In this instance, a runtime error is thrown and subsequently caught, allowing the program to continue running rather than crashing. Exception handling is crucial for writing resilient software.
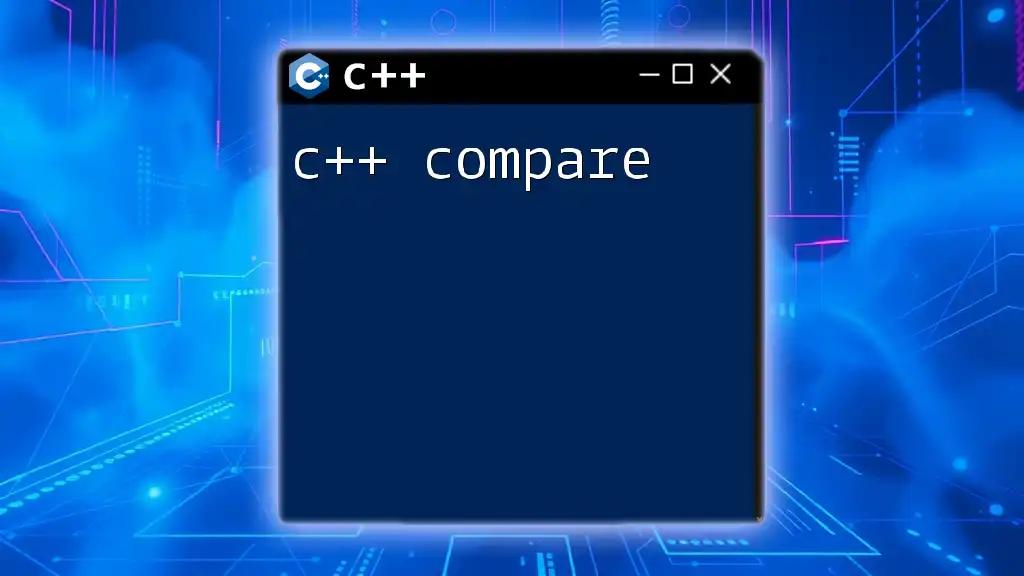
Best Practices in Using C++ Commands
Writing Clean Code
Writing clean, maintainable code involves using meaningful variable names, proper indentation, and sufficient comments. By documenting your code effectively, you make it easier for others (and yourself) to understand your logic and purpose behind commands.
Performance Optimization
Efficiently using C++ commands impacts the performance of your applications. Analyze your code for redundancy, favor algorithms with better time and space complexity, and consider using efficient data structures. Strive to write code that runs efficiently but remains accessible and easy to read.
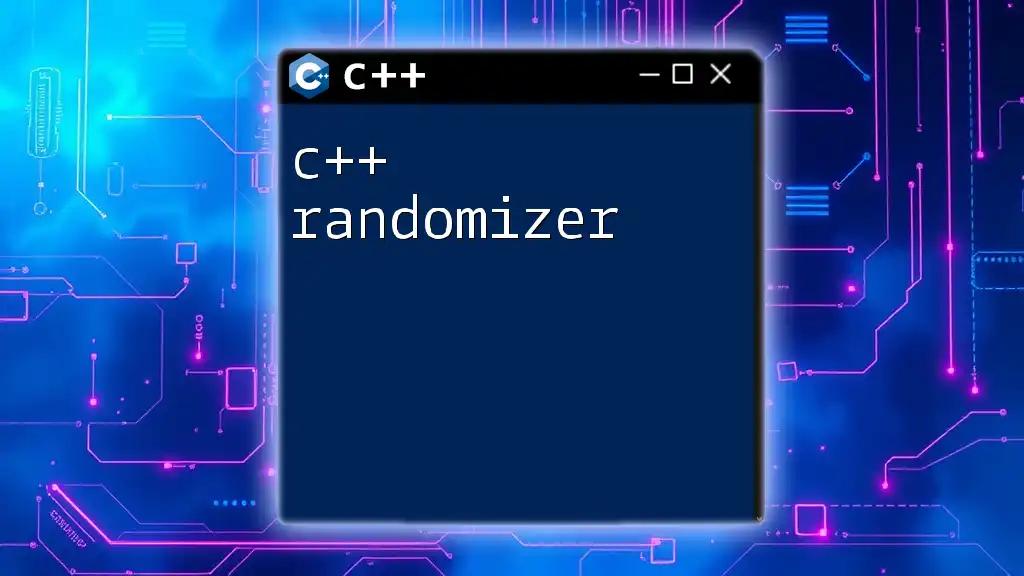
Conclusion
Mastering C++ commands is vital for effective programming in C++. With these foundational skills, you can take your programming abilities to the next level, facilitating the creation of robust, efficient, and interactive applications. The journey in C++ programming continues to evolve—embracing these commands opens the door to continued learning and discovery in the vast world of programming.
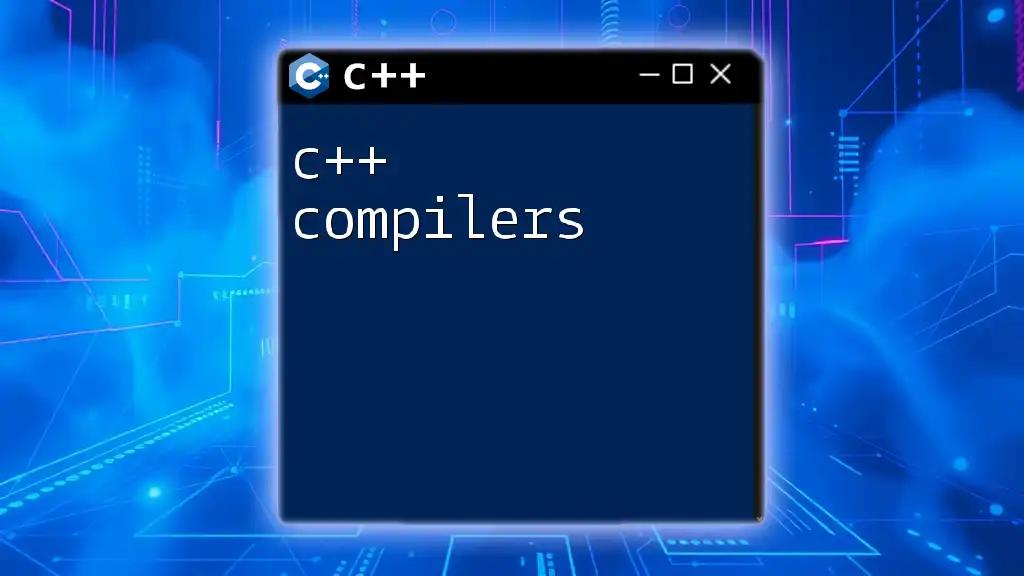
Additional Resources
For further exploration:
- Refer to the official C++ documentation.
- Consider reading foundational books like "The C++ Programming Language" by Bjarne Stroustrup.
- Engage with community forums such as Stack Overflow and Reddit for support and discussion.
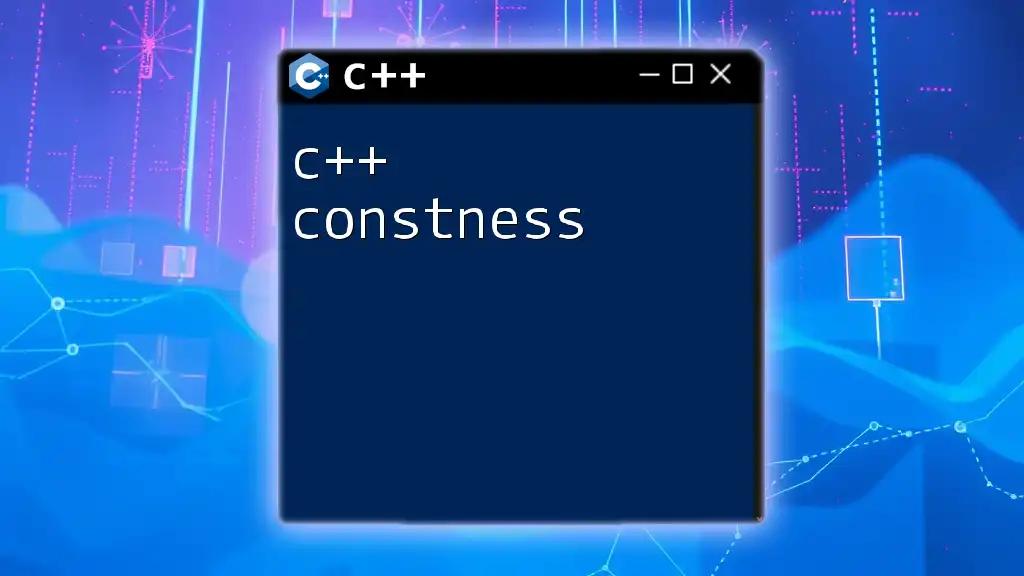
Call to Action
We encourage you to dive deeper into C++ programming, experiment with the commands discussed, and share your thoughts and questions to enhance your learning journey!