C++ conditional variables are synchronization primitives that allow threads to wait for certain conditions to be met before proceeding, typically used in conjunction with a mutex to avoid busy-waiting.
Here's a simple code snippet demonstrating the use of conditional variables:
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void worker() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return ready; }); // Wait until 'ready' is true
std::cout << "Worker thread proceeding after condition is met." << std::endl;
}
int main() {
std::thread t(worker);
std::this_thread::sleep_for(std::chrono::seconds(1)); // Simulate work
{
std::lock_guard<std::mutex> lock(mtx);
ready = true; // Change the condition
}
cv.notify_one(); // Notify the waiting thread
t.join();
return 0;
}
What are C++ Conditional Variables?
C++ conditional variables are synchronization primitives that allow threads to pause execution and wait for certain conditions to be met before proceeding. In a multithreaded environment, threads frequently need to communicate with one another. Conditional variables facilitate this communication by making it easier to signal and wait for events.
Unlike other synchronization mechanisms such as mutexes, which merely provide mutual exclusion, conditional variables enable threads to synchronize based on specific conditions. They effectively allow one or more threads to wait for notifications from other threads, which can help in optimizing resource usage and improving efficiency.
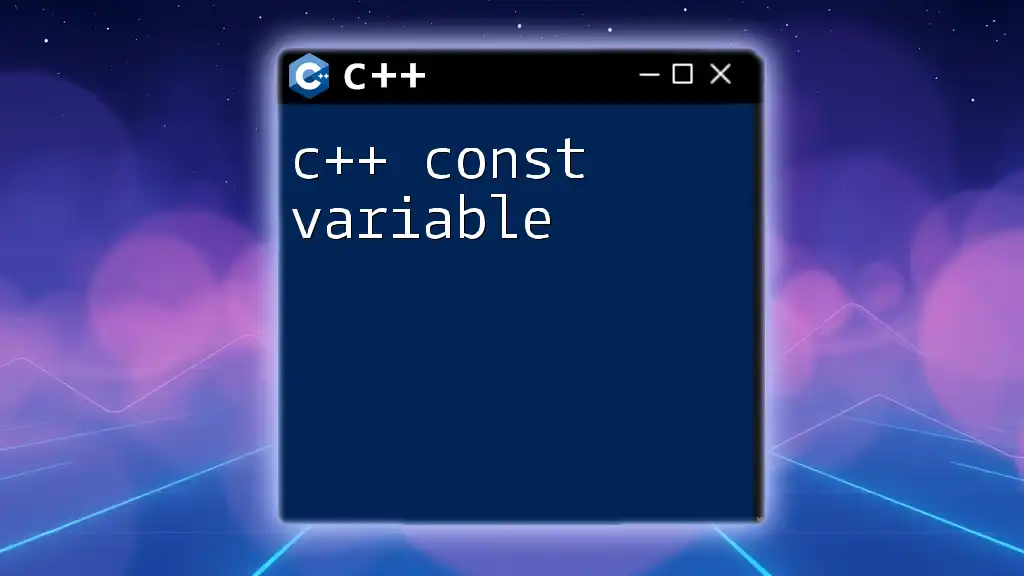
When to Use C++ Condition Variables
C++ conditional variables are particularly useful in scenarios where you need threads to collaborate or share resources. Common situations include:
- Producer-Consumer Patterns: Where one thread produces data and another consumes it, ensuring that the consumer waits until data is available.
- Task Coordination: When multiple threads need to wait for specific tasks to complete before proceeding.
- State Management: In cases where threads need to wait for state changes or specific events.
The advantages of using conditional variables include reduced CPU usage, allowing threads to sleep while waiting for conditions, and simplified code by abstracting complex synchronization logic.
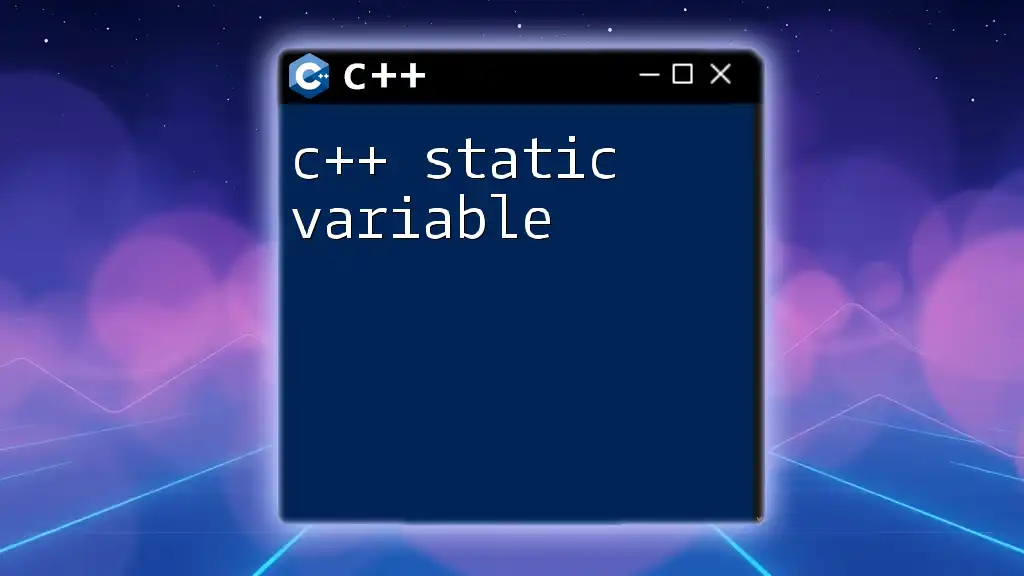
Setting Up C++ Conditional Variables
Required Headers
To use C++ conditional variables, you must include several standard headers in your source code:
#include <condition_variable>
#include <mutex>
#include <thread>
These headers provide the necessary definitions and functionalities for implementing conditional variables.
Basic Components of C++ Conditional Variables
- std::condition_variable: This is the actual conditional variable that threads will use to wait and notify other threads.
- std::unique_lock<std::mutex>: This lock is used to manage access to shared data and ensures that the condition variable is protected from race conditions.
The following code snippet shows how to declare these components:
std::condition_variable cv;
std::mutex mtx;
Here, `cv` is the condition variable used to manage the waiting threads, and `mtx` is a mutex that will protect shared data accessed by those threads.
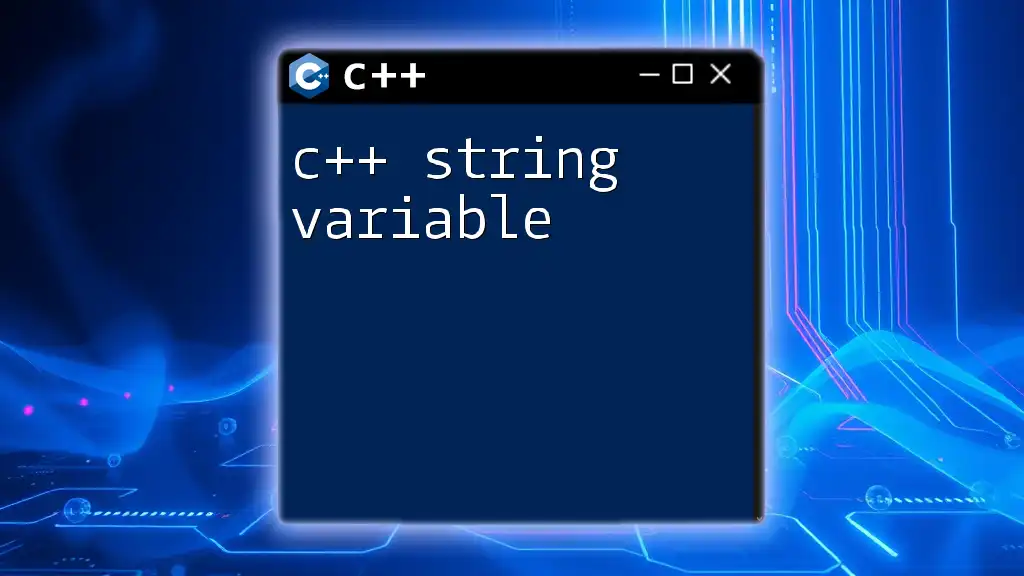
Using C++ Conditional Variable: Step-by-Step Guide
Step 1: Initializing Condition Variables
After declaring your conditional variable and mutex, the next step is to prepare for their usage within threads.
std::condition_variable cv;
std::mutex mtx;
bool ready = false; // Condition flag
In this example, we introduce a boolean variable `ready` that will be used as a condition for the threads to wait on.
Step 2: Waiting for Conditions
Threads can wait for a condition to be met by calling the `wait()` method on the condition variable. The `wait()` call will automatically release the associated lock until the condition is signaled.
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, []{ return ready; });
In this snippet:
- A unique lock is created, locking the mutex `mtx`.
- `cv.wait(lock, []{ return ready; });` blocks execution until `ready` becomes `true`.
This usage ensures mutual exclusion while waiting for the condition to change.
Step 3: Notifying Threads
Once the condition changes (for example, when `ready` is set to `true`), one or more threads can be notified to continue execution. This can be done using `notify_one()` or `notify_all()`:
ready = true; // Set the condition
cv.notify_one(); // Notify one waiting thread
In this case, only one waiting thread will be released from its waiting state, while others will remain blocked until they are notified separately.
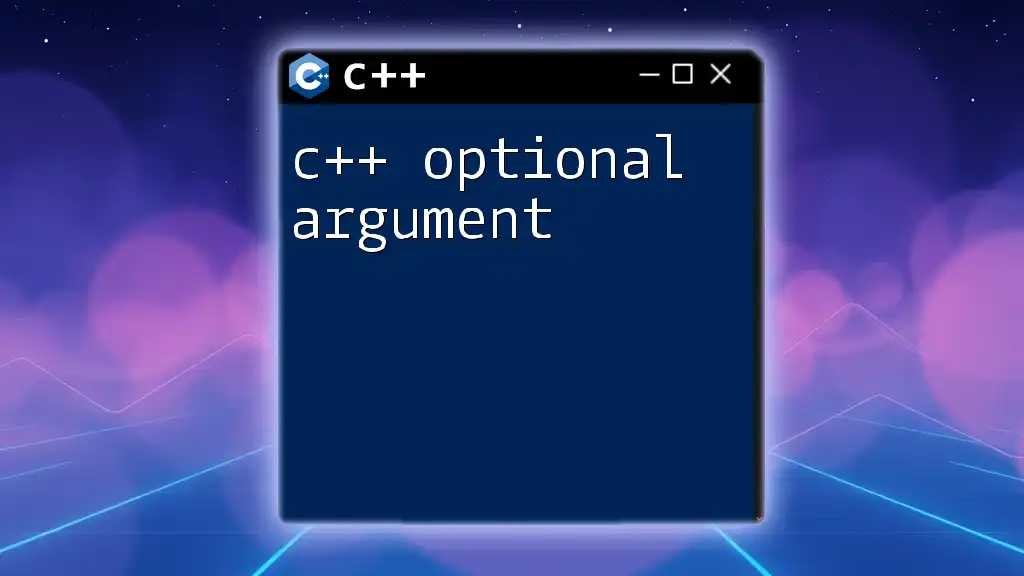
Common Patterns Using Condition Variables in C++
Producer-Consumer Problem
A classic example of using C++ conditional variables is in the producer-consumer problem, where a producer thread generates data and the consumer thread processes it.
Here's how you can implement such a pattern:
std::queue<int> dataQueue;
std::condition_variable cv;
std::mutex mtx;
bool data_available = false;
void producer() {
for (int i = 0; i < 10; ++i) {
std::unique_lock<std::mutex> lock(mtx);
dataQueue.push(i); // Produce data
data_available = true;
cv.notify_one(); // Notify the consumer
}
}
void consumer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, []{ return data_available; });
while (!dataQueue.empty()) {
int data = dataQueue.front(); // Consume data
dataQueue.pop();
// Process data
}
data_available = false; // Reset condition
}
}
In this code:
- The `producer()` function generates data and adds it to a queue.
- After producing data, it notifies the consumer that there is data available.
- The `consumer()` function waits for the notification, consumes the data, and processes it accordingly.
Other Usage Examples
Additional scenarios for using condition variables include:
- Task Execution: Threads waiting on certain tasks to complete before proceeding.
- Resource Management: Threads waiting for resources to become available, such as network connections or file handles.
std::condition_variable resource_cv;
std::mutex resource_mtx;
bool resource_available = false;
void request_resource() {
std::unique_lock<std::mutex> lock(resource_mtx);
resource_cv.wait(lock, []{ return resource_available; });
// Take resource and process it
}
void release_resource() {
std::unique_lock<std::mutex> lock(resource_mtx);
resource_available = true;
resource_cv.notify_all(); // Notify all waiting threads
}
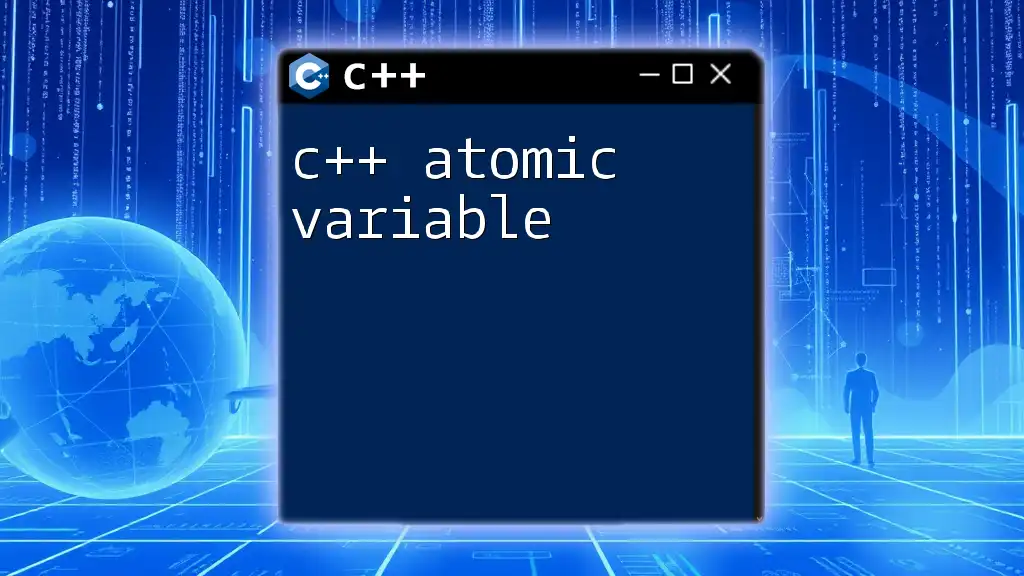
Best Practices for Using C++ Conditional Variables
To effectively utilize C++ conditional variables, consider the following best practices:
-
Avoid Spurious Wakeups: Be aware that the `wait()` function might wake up without a `notify` call. Always use conditions in a looping structure.
-
Protect Shared State: Always lock the associated mutex when reading or modifying shared state variables to avoid race conditions.
-
Limit Notifications: Use `notify_one()` if only one thread requires activation. Use `notify_all()` sparingly to avoid unnecessary wake-ups.
By adhering to these guidelines, you can ensure robust, efficient, and clear multithreaded code.
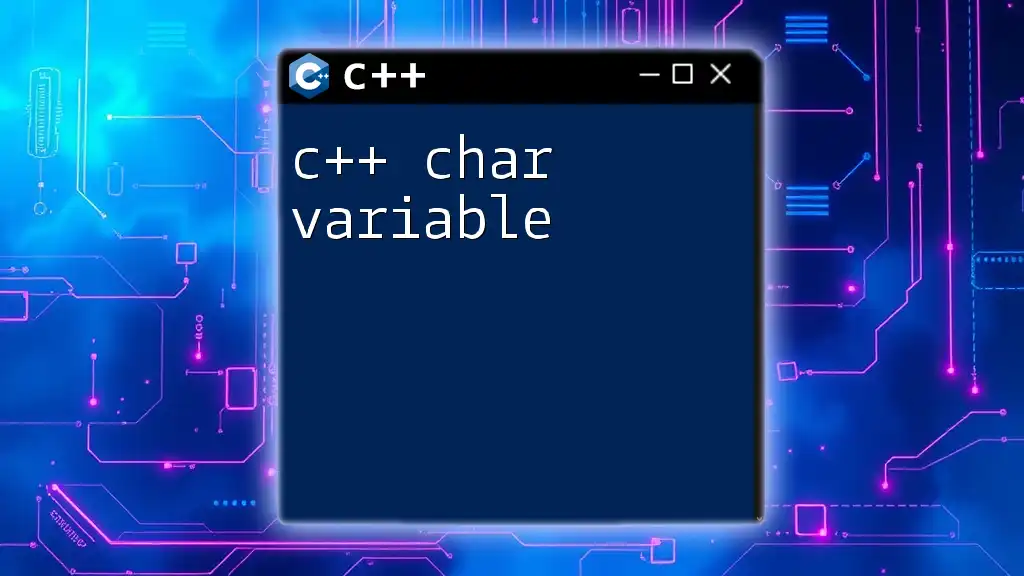
Debugging Issues with C++ Condition Variables
Common Problems
When working with conditional variables, it’s crucial to be cautious of common pitfalls like deadlocks and race conditions:
-
Deadlocks: Two or more threads waiting indefinitely for conditions that cannot be satisfied can cause a deadlock. Properly review your lock acquisitions and ensure that locks are always released.
-
Race Conditions: Shared state changes must be protected. Accessing shared variables outside of a lock can lead to inconsistent states.
Tools and Techniques
Utilizing debugging tools can help uncover synchronization issues. Recommended tools include:
- Valgrind: For detecting memory leaks and checking thread synchronization.
- ThreadSanitizer: A fast data race detector that can identify threading issues during development.
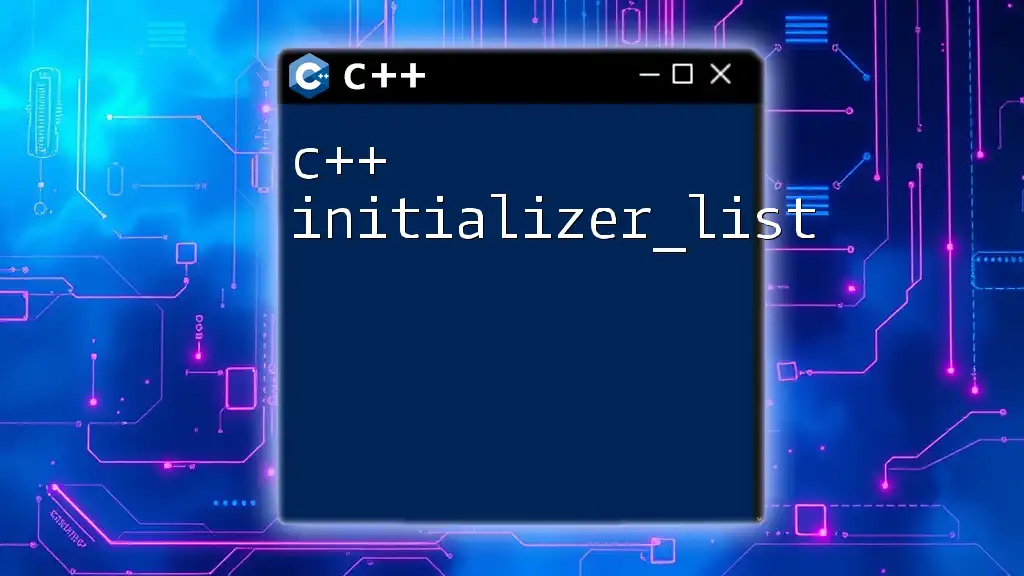
Conclusion
C++ conditional variables are an essential tool for thread synchronization, allowing you to devise sophisticated multithreaded applications. Understanding their usage will enhance your ability to manage complex interactions between threads while ensuring both performance and clarity in your code.
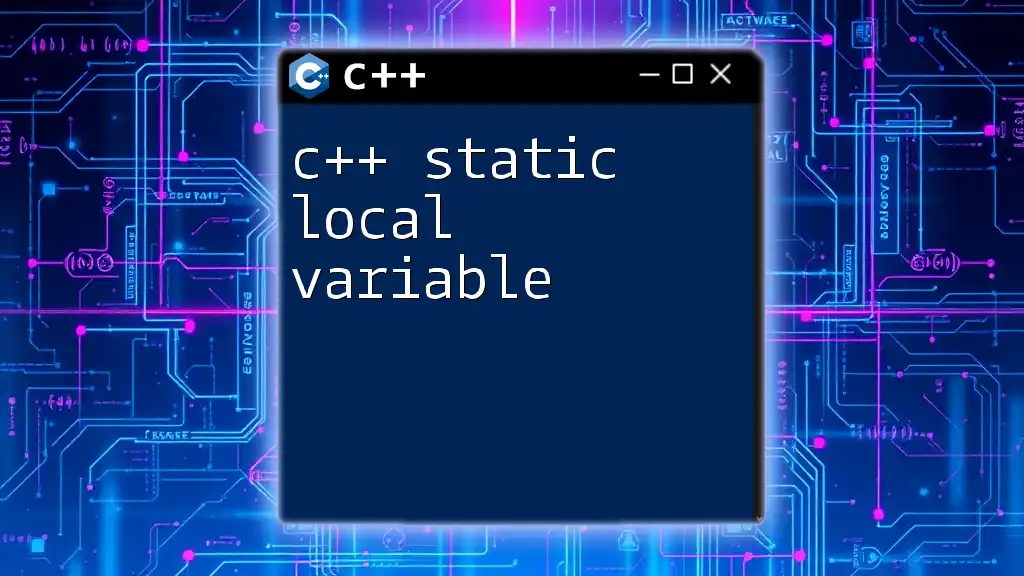
Further Reading & Resources
For those looking to delve deeper into C++ conditional variables, consider the following resources:
- [C++ Reference Documentation](https://en.cppreference.com/)
- Advanced multithreading tutorials on platforms like Coursera or Udemy.
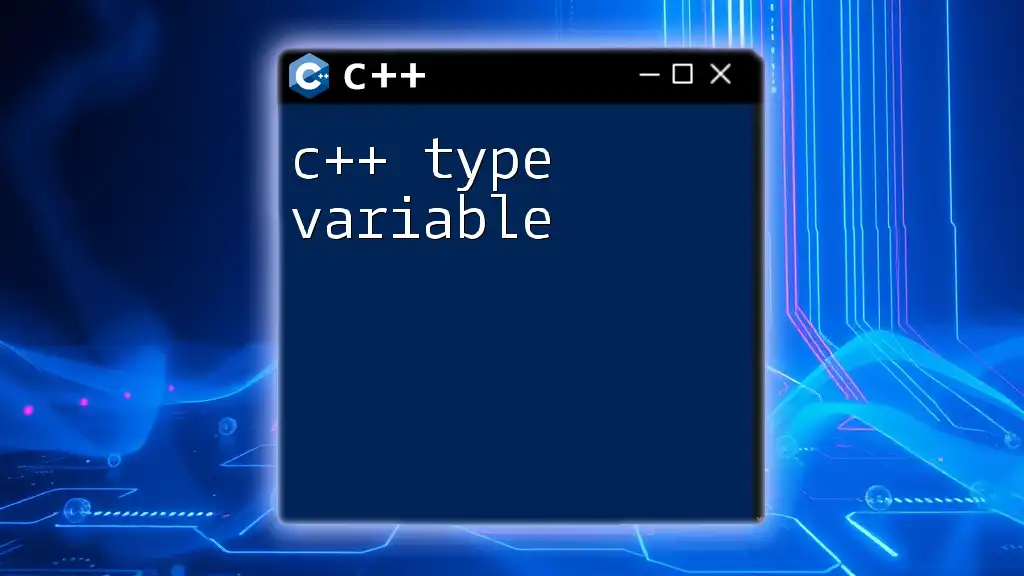
FAQs about C++ Conditional Variables
-
What is a conditional variable? A conditional variable is a synchronization primitive in C++ that allows threads to wait for certain conditions to be met.
-
How do I avoid issues with condition variables? Use unique locks, check conditions in a loop, and ensure proper mutex management to avoid deadlocks and race conditions.
-
Can one thread notify multiple threads? Yes, using `notify_all()` will wake all waiting threads, but it’s generally better to use `notify_one()` if only one thread needs to proceed.
By grasping these concepts and implementing best practices, you will be well-equipped to handle multithreading in C++ with confidence.