In C++, a `const` variable is a constant value that cannot be modified after its initial definition, providing a way to protect critical values within your program.
Here's a code snippet demonstrating the use of a `const` variable:
#include <iostream>
int main() {
const int maxScore = 100; // maxScore cannot be changed
std::cout << "The maximum score is: " << maxScore << std::endl;
return 0;
}
The Basics of C++ Const Keyword
What is the C++ Const Keyword?
The `const` keyword in C++ is a powerful and essential tool in the programming language that indicates an immutable variable. When a variable is declared with `const`, its value cannot be changed after initialization. This characteristic is crucial for protecting data and ensuring that the program behaves as expected.
Syntax of Const Variables
Declaring a const variable requires a specific syntax. The general format resembles a typical variable declaration, but it includes the `const` keyword:
const int maxScore = 100;
This line indicates that `maxScore` is a constant integer initialized to 100, and any attempts to modify `maxScore` will result in a compile-time error.
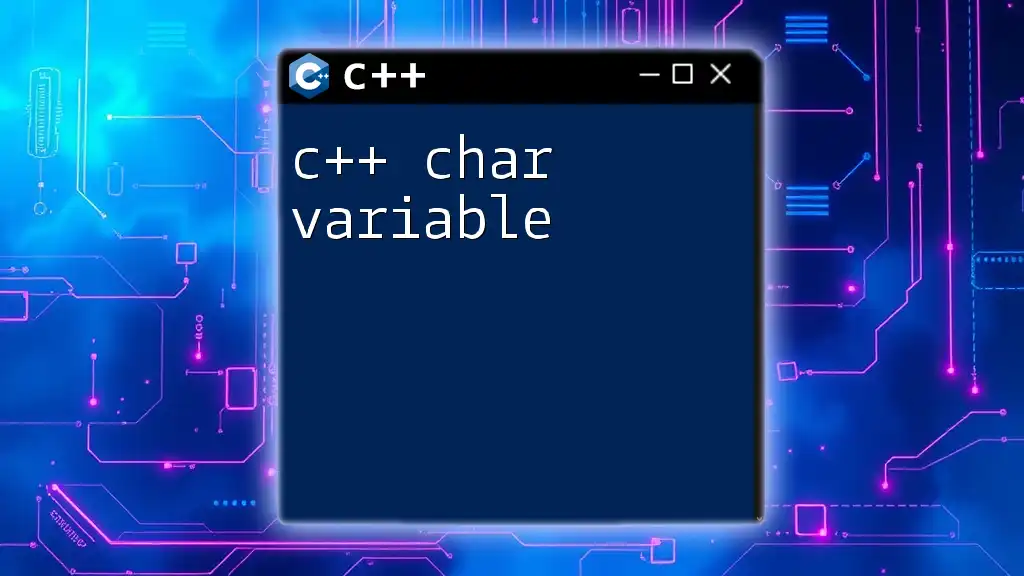
Declaring and Using Const Variables
Declaring Const Variables
To declare a const variable, simply precede the variable type with the `const` keyword. This declaration must be accompanied by an initialization since the value of a const variable cannot be altered later.
const double PI = 3.14159;
Here, `PI` is a constant double representing the mathematical constant pi.
Using Const Variables in Code
Utilizing const variables in your code can help prevent errors. For instance, consider this simple code snippet:
if (score > maxScore) {
// Error handling code
cout << "Score exceeds maximum allowable limit!";
}
In this example, `maxScore` acts as a defined limit, making your program more resilient to unintended changes while providing clarity about the variable's intent.
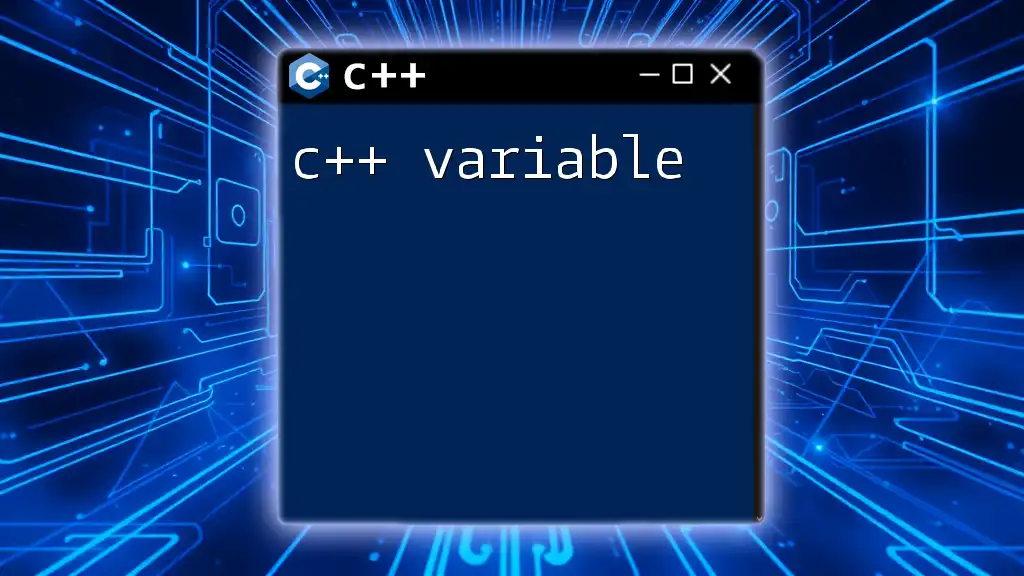
The Benefits of Using C++ Const Variables
Enhancing Code Safety and Reliability
One of the primary advantages of using const variables is improved safety. By making a variable constant, you can prevent accidental modification during the execution of your code. For example:
const int numDays = 30;
// numDays = 31; // This line will cause a compile-time error
This provides clarity in your code, preventing mistakes that could arise from changing significant values.
Improving Performance
Using const variables can also lead to performance enhancements. Compilers often optimize code based on the knowledge that certain variables won't change. This ability allows the compiler to make optimizations, potentially resulting in faster code execution.
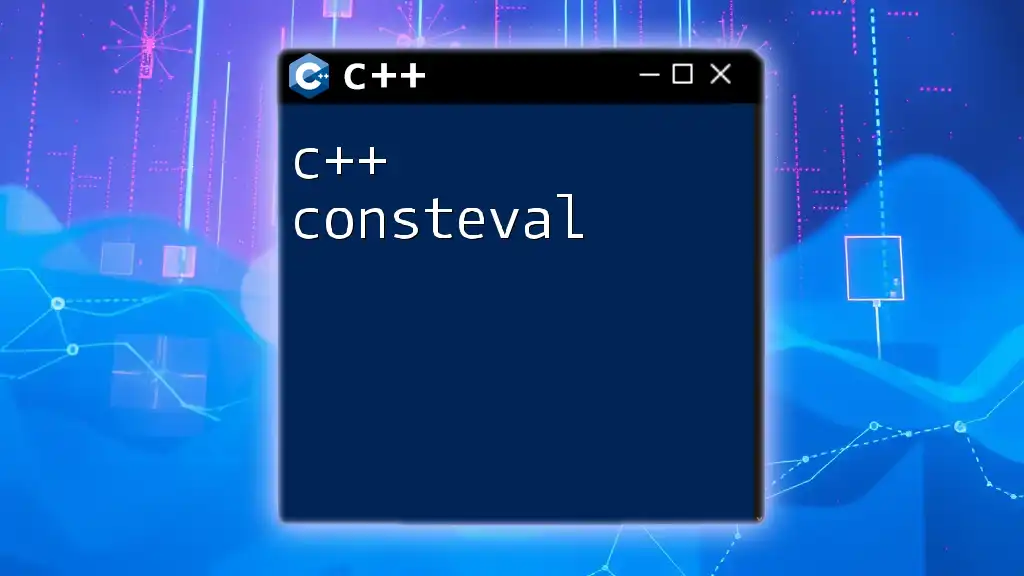
Const Variables vs Non-Const Variables
Comparing Const and Non-Const Variables
Understanding the distinction between const and non-const variables is essential. While regular variables can be modified at any point in the program, const variables serve as constants once declared. The inability to alter const variables ensures that critical data remains unchanged, promoting program stability.
Example Scenario
Consider a simple scenario involving a counting loop:
int main() {
const int limit = 10;
int count = 0;
for (int i = 0; i < limit; ++i) {
count++;
}
// limit = 20; // This will cause a compile-time error
}
Here, the `limit` variable cannot be changed, ensuring that the loop iterates a consistent number of times without unintended alterations.
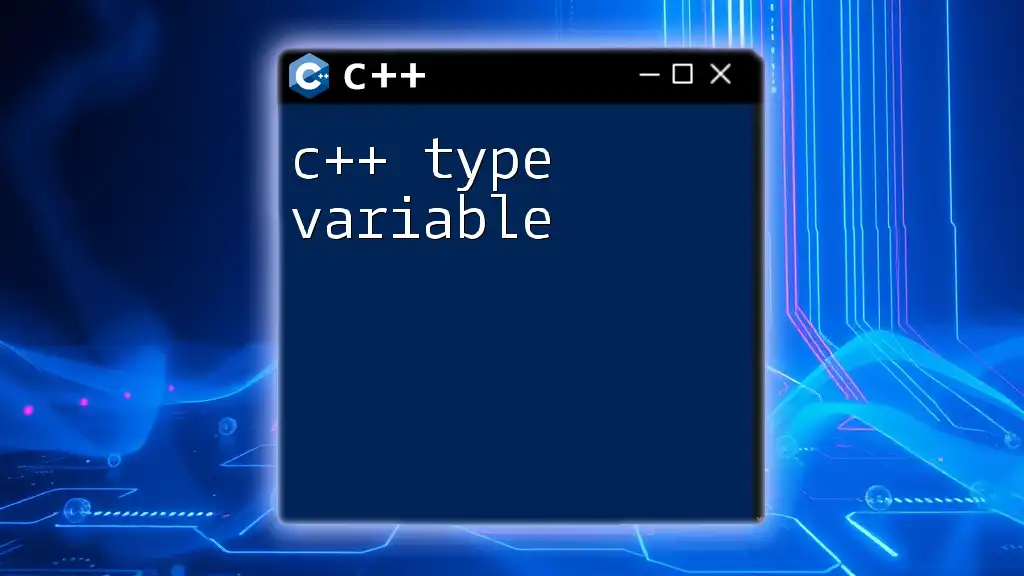
Scope and Lifetime of Const Variables
Local and Global Const Variables
The scope of a `const` variable can greatly impact its functionality in your program. Local const variables are limited to the block in which they were defined, while global const variables are accessible throughout your program.
For instance:
const int globalVar = 42; // Global const variable
void someFunction() {
const int localVar = 20; // Local const variable
cout << globalVar; // Accessing global var
cout << localVar; // Accessing local var
}
Lifetime and Storage Duration
The lifetime of const variables is related to their scope. Local const variables will exist only as long as the block is executed, whereas global const variables persist throughout the program's runtime. This understanding assists in memory management and resource allocation.
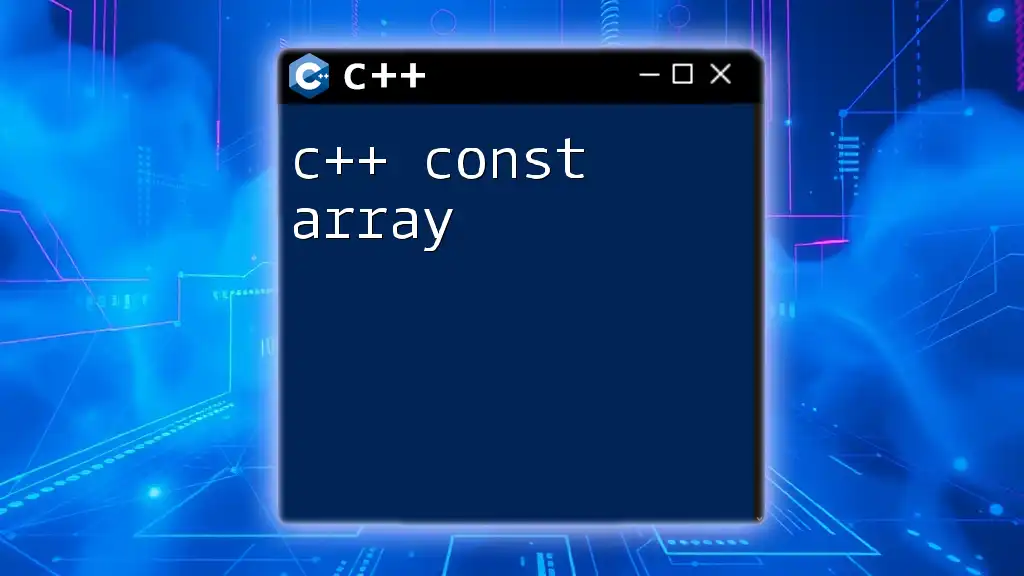
Const Pointers and References
Understanding Const Pointers
In C++, you can also have const pointers, which are pointers that cannot be changed to point to different addresses after their initialization. The syntax for declaring a const pointer is as follows:
int value = 5;
const int *ptr = &value; // ptr points to a const int
In this example, the pointer `ptr` cannot change the value it points to but can be reassigned to point to another integer type variable.
Const References
Const references are references that cannot be used to modify the variable they reference. This is particularly useful in functions where you want to prevent any modifications to the input parameter.
void printValue(const int &val) {
// Cannot modify val
cout << val << endl;
}
Utilizing const references increases code readability and performance by avoiding unnecessary copies.
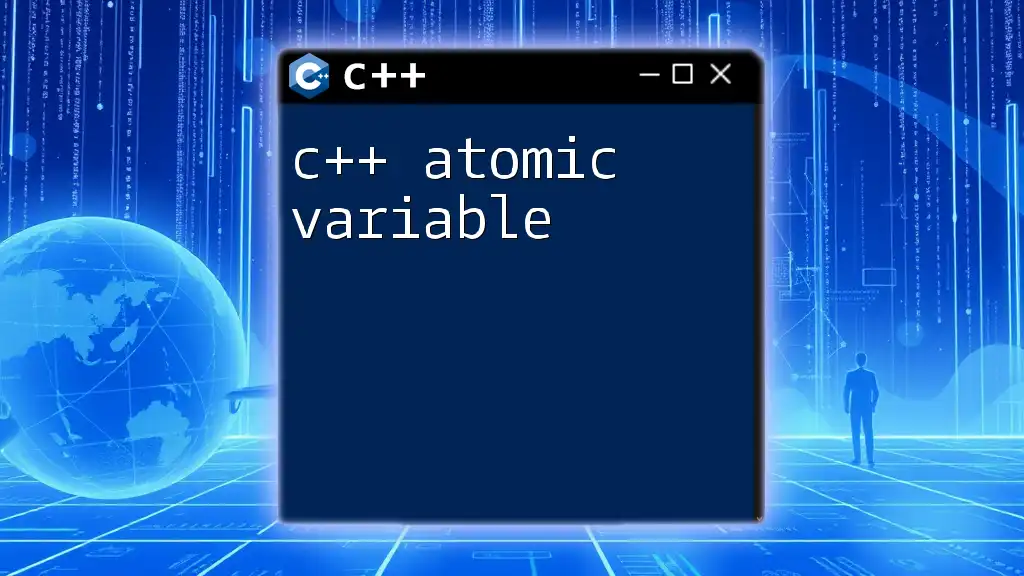
Constexpr Variables in C++
Introduction to Constexpr
C++ introduces `constexpr`, which allows certain variables to be evaluated at compile time. The primary distinction between `const` and `constexpr` is that `constexpr` applies to variables with guaranteed compile-time constants.
Using Constexpr for Compile-Time Constants
With `constexpr`, you can declare constants like this:
constexpr int getValue() {
return 42;
}
Using `constexpr` is particularly beneficial in defining constants used in array sizes and template parameters.
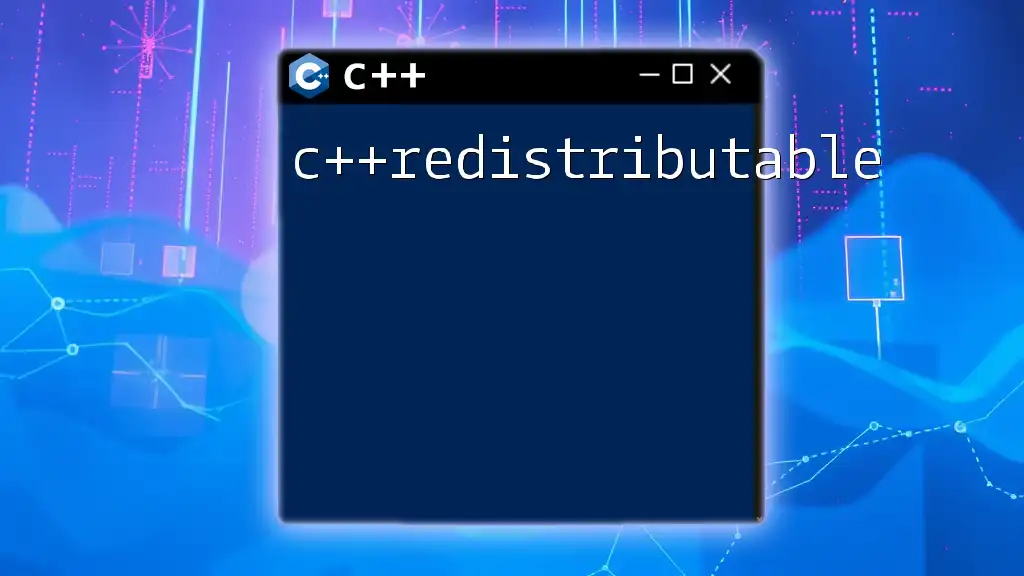
Common Mistakes with Const Variables
Avoiding Mistakes
Misunderstandings of the const modifier can lead to errors in code development. For example, trying to modify a const variable will result in a compile-time error, leading to unfinished functionality and frustrating debugging sessions.
Best Practices to Follow
To avoid common pitfalls, consider these best practices:
- Always initialize const variables at the time of declaration.
- Utilize const variables for defining constants rather than hardcoding values, which can be error-prone.
- Whenever possible, prefer const references for parameters in function signatures to enhance performance.
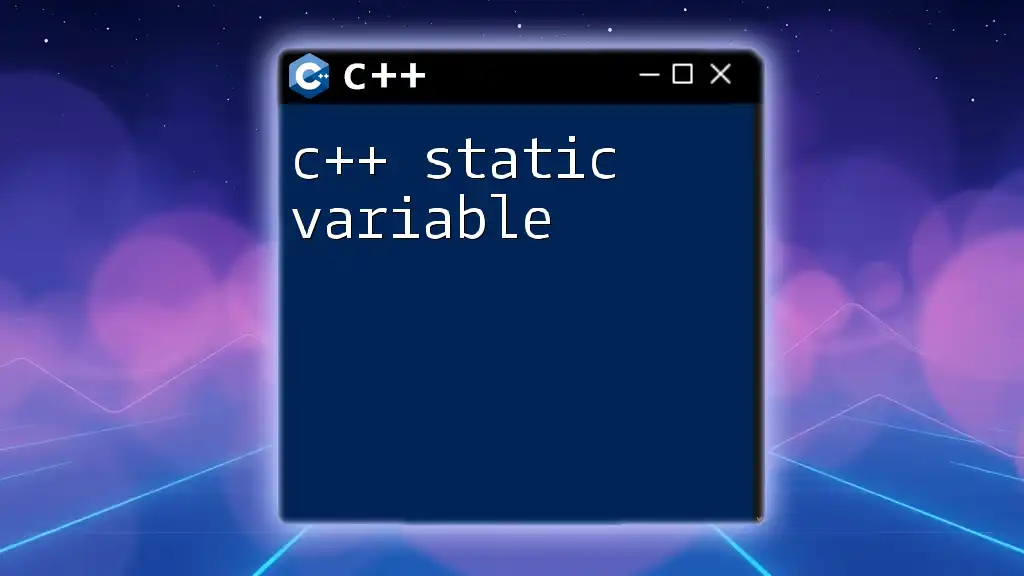
Conclusion
Using c++ const variables effectively enhances code safety, reliability, and performance. Understanding their usage alongside key distinctions between const and non-const entities is essential for any C++ developer. Implementing these practices in your coding routine will lead to more disciplined, readable, and maintainable code.