In C++, `const_cast` is used to add or remove the `const` qualifier from a variable, allowing you to modify a constant value or to remove the constness from a variable when it is safe to do so.
#include <iostream>
void modifyValue(const int* ptr) {
int* modifiablePtr = const_cast<int*>(ptr);
*modifiablePtr = 20; // Changes the value at the address
}
int main() {
int value = 10;
const int* ptr = &value;
std::cout << "Original value: " << value << std::endl;
modifyValue(ptr);
std::cout << "Modified value: " << value << std::endl; // Output will be 20
return 0;
}
Understanding const_cast
const_cast is a powerful feature in C++ that allows you to modify the const or volatile qualifiers on a variable. While C++ provides several type casting mechanisms, such as static_cast, dynamic_cast, and reinterpret_cast, const_cast specifically focuses on altering the constness of an object. This can be particularly useful when working with APIs and libraries that expect non-const arguments but you know that the original object doesn’t modify any constant data.
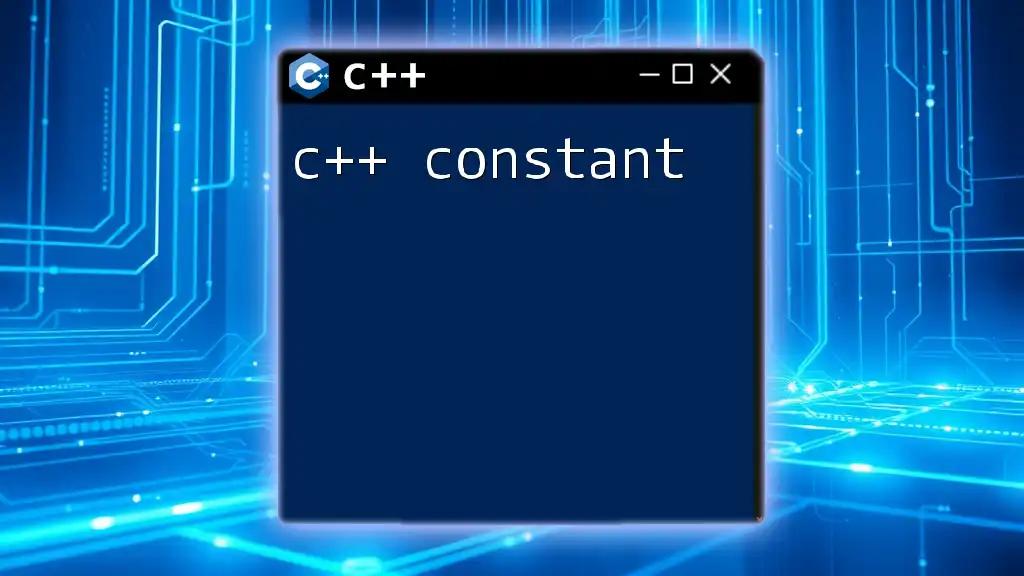
When to Use const_cast
There are several scenarios where using const_cast becomes essential:
-
Interfacing with APIs: Sometimes, libraries or functions require non-const arguments, even if the data doesn’t need modification. In such cases, const_cast allows you to pass const objects without losing the ability to access their data.
-
Legacy Code Management: Often in legacy applications, the code might have been written with const-correctness in mind, but as it evolves, new requirements may arise that necessitate temporary const removal.
-
Performance Considerations: In performance-critical applications, sometimes heaps of data can be passed as const objects to avoid unnecessary copies, yet the function called doesn't actually modify those objects.
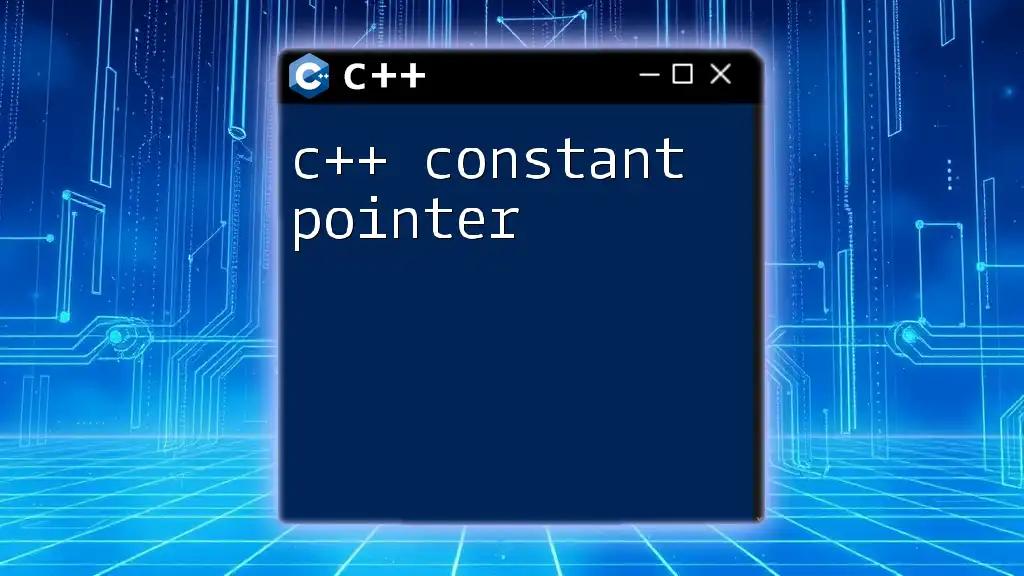
Syntax of const_cast
The general syntax for const_cast is:
const_cast<type>(expression)
- type: This specifies the target type of the expression after casting.
- expression: This is the original expression that you want to cast.
Using the correct syntax ensures that you can efficiently manage the constness of variables in your applications.
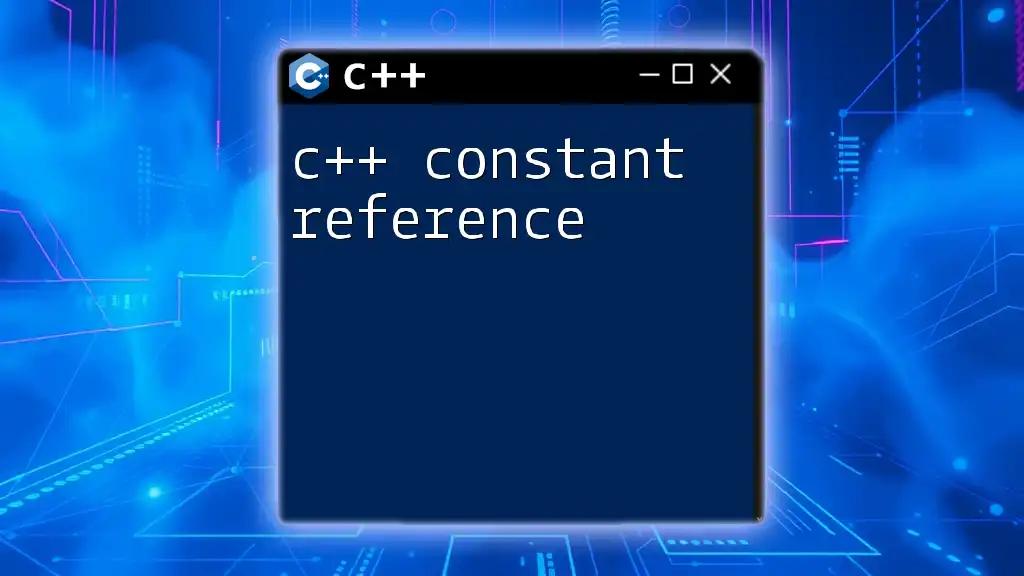
Example: Basic Usage of const_cast
Here’s a simple example demonstrating the fundamental use of const_cast:
void printString(const char* str) {
// const_cast to modify string
char* mutableStr = const_cast<char*>(str);
mutableStr[0] = 'H'; // Changing the first character
}
int main() {
const char* myString = "Hello, World!";
printString(myString);
// Output: Error - modifies a string literal
}
In this example, the intention is to change the initial character of a string literal. However, doing so results in undefined behavior since string literals are inherently stored in a read-only section of memory. This demonstrates that while const_cast can allow changes, care must be taken to avoid manipulating immutable data.

Safe Use of const_cast
To use const_cast safely, you should ensure that you are only modifying data that is indeed mutable. Here’s an example that showcases the safe application of const_cast:
void safePrint(const std::string& str) {
std::string& modifiableStr = const_cast<std::string&>(str);
modifiableStr.append(" with const_cast.");
std::cout << modifiableStr << std::endl;
}
// Usage
std::string greeting = "Hello";
safePrint(greeting);
In this scenario, we take a `const` reference to a string, convert it into a non-const reference using const_cast, and append additional text. Since `greeting` is a mutable object, this operation is safe and valid. It serves as an example of responsible usage of const_cast while avoiding undefined behavior.
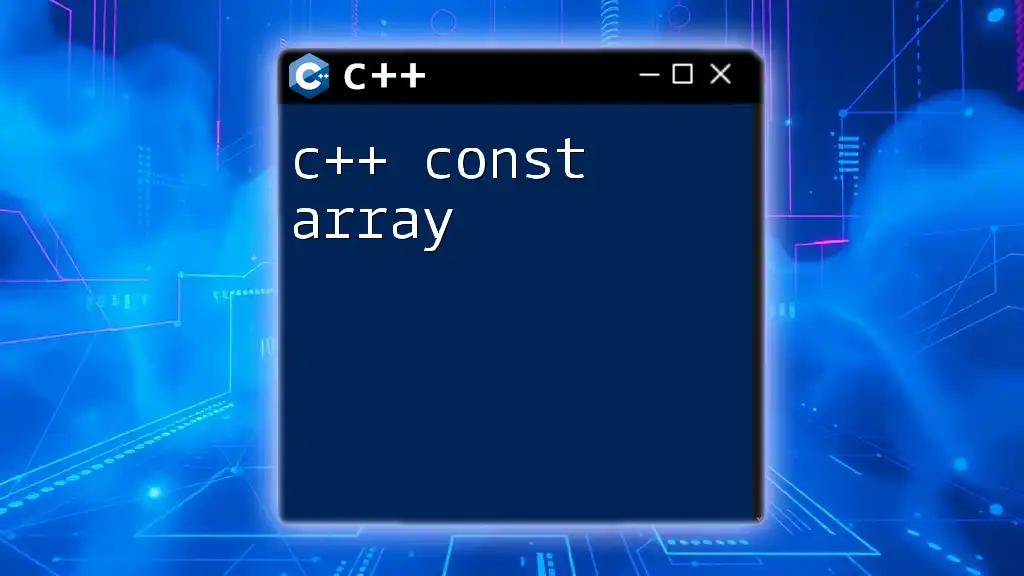
Common Mistakes with const_cast
Despite its utility, const_cast can lead to significant errors if misused. Key pitfalls include:
-
Modifying const Objects: Attempting to change the value of a true const object using const_cast can lead to unpredictable behavior, often manifesting as program crashes.
-
Misunderstandings of Behavior: Some developers mistakenly believe that const_cast can be used in any context. This misunderstanding often results in easy-to-miss bugs that arise from modifying something that shouldn’t be altered.
Here’s an example that illustrates common mistakes:
const int num = 10;
int* pNum = const_cast<int*>(&num);
// Undefined behavior: modifying a const variable
*pNum = 20;
In this case, num is declared as a constant, and modifying it through a pointer is an obvious misuse of const_cast, leading to undefined behavior.

Best Practices for Using const_cast
When using const_cast, it’s crucial to implement best practices to maintain code safety:
-
When to Avoid const_cast: If you can achieve your goal without using const_cast, it’s often better to do so. Working with const-correct code from the start is ideal.
-
Sparing Usage: Use const_cast sparingly, primarily for interfacing with APIs or libraries rather than as a crutch to bypass type safety.
-
Good Coding Practices:
- Thorough Documentation: Always explain why const_cast is being used to avoid confusion among other developers.
- Regular Code Reviews: Implement code reviews to identify potential misuse and enhance code quality.
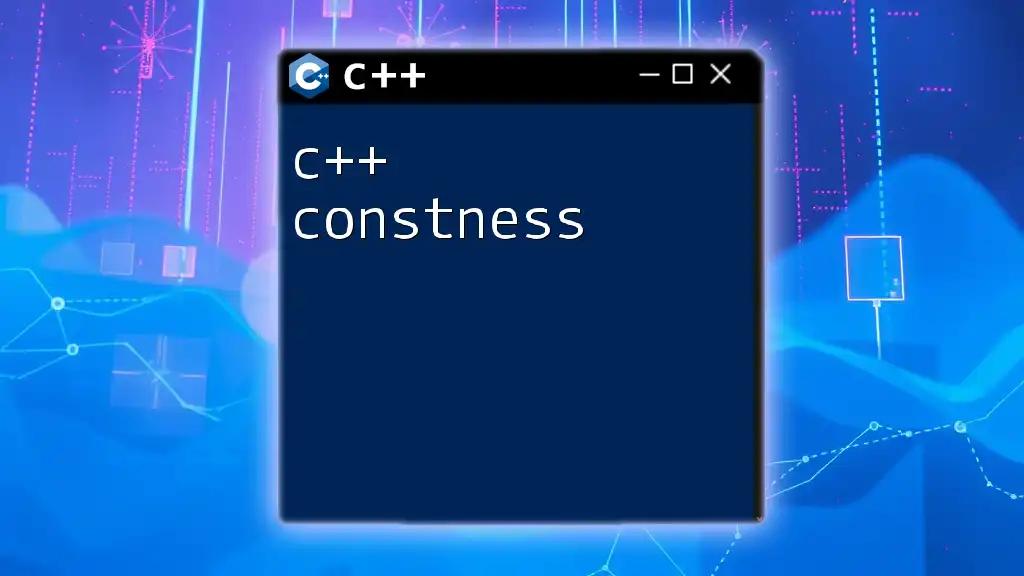
Alternatives to const_cast
While const_cast is invaluable in certain situations, it's essential to know other casting options available in C++:
-
static_cast: Used for conversions between compatible types, this cast enables safe conversions without const-casting.
-
dynamic_cast: Primarily used for safely downcasting in class hierarchies (with polymorphism), ensuring accurate type-safe checks.
-
reinterpret_cast: Allows low-level casts between types, including pointer types and fundamental types, suited for system-level programming.
Choosing the right casting mechanism will lead to safer and more maintainable code.
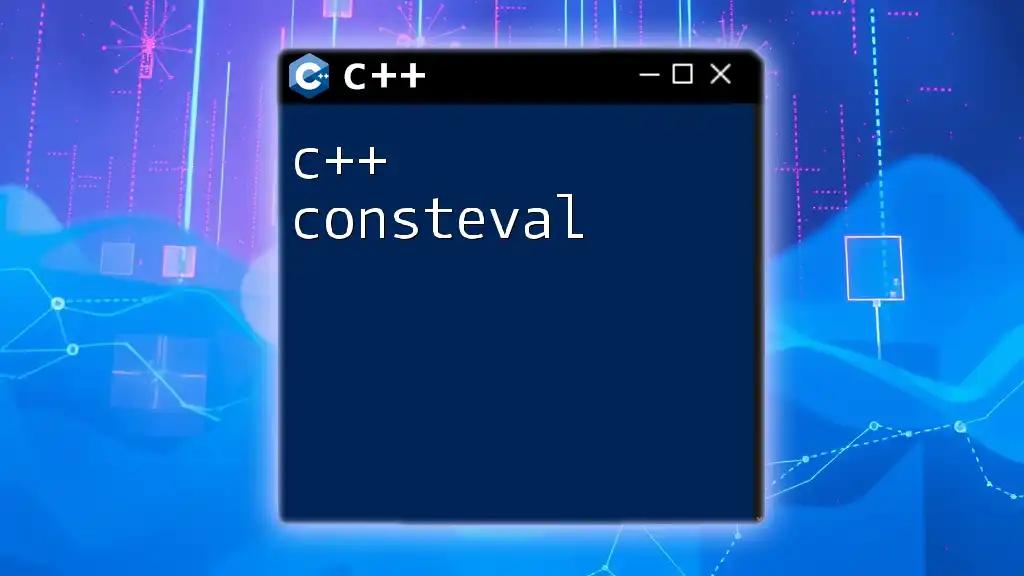
Conclusion
In summary, const_cast is a useful tool in C++ for managing constness, but it should be employed judiciously and appropriately. By understanding when and how to use it safely, recognizing common mistakes, and adhering to best practices, you can meaningfully enhance the quality of your C++ code. Always remember, good software development principles promote const-correctness, so use const_cast wisely!
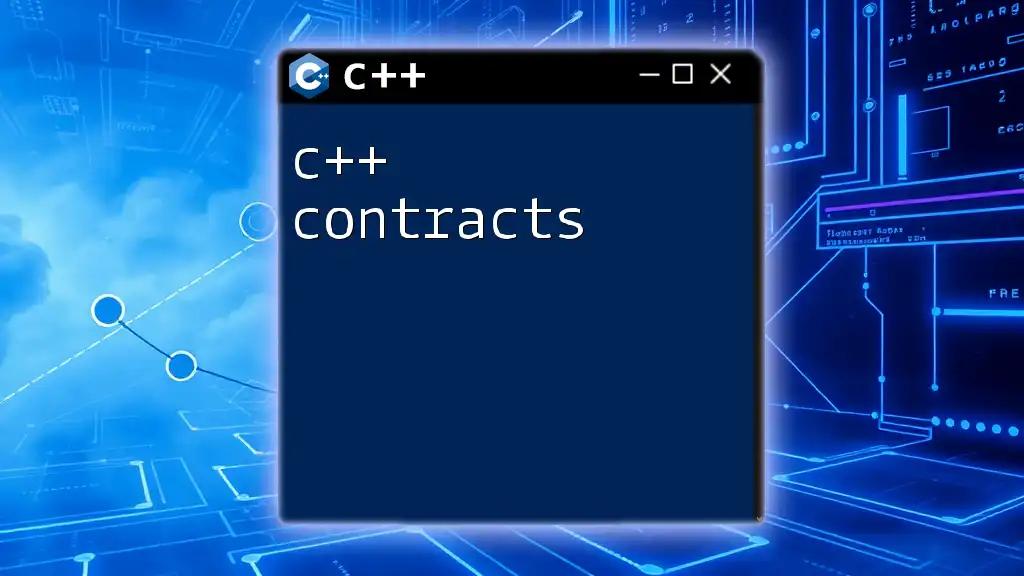
Additional Resources
For further reading and understanding of type casting in C++, refer to the official C++ documentation and explore additional literature focused on best practices in modern C++ development.