C++ downcasting is the process of converting a base class pointer or reference to a derived class type, typically using `dynamic_cast`, to safely access derived class members.
#include <iostream>
class Base {
public:
virtual void show() { std::cout << "Base class" << std::endl; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived class" << std::endl; }
};
int main() {
Base* basePtr = new Derived(); // Base class pointer pointing to Derived class
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Downcasting
if (derivedPtr) {
derivedPtr->show(); // Accessing Derived class method
}
delete basePtr; // Clean up
return 0;
}
Understanding Downcasting
What is Downcasting?
Downcasting refers to the process of casting a pointer or reference of a base class to a derived class type. This mechanism is particularly vital in the context of polymorphism, allowing for dynamic type checks at runtime. In C++, downcasting is frequently used when you have a base class pointer that actually points to a derived class object.
When is Downcasting Used?
Downcasting becomes essential in scenarios where a base class pointer needs to access derived class members or methods that are not defined in the base class. For example, if you have a function that accepts a base class pointer, but you need to call specific functions of the derived class, downcasting becomes necessary.

Understanding Class Hierarchy
Overview of Class Hierarchy in C++
C++ utilizes a class hierarchy entrenched in OOP (Object-Oriented Programming) principles. A base class can provide common functionality, while derived classes can extend or override that functionality. The use of virtual functions allows for dynamic polymorphism, enabling the execution of the appropriate method based on the object's actual type rather than the type of the pointer.
Static vs. Dynamic Types
Understanding the difference between static and dynamic types is crucial. The static type is the type given in the declaration (the type of the pointer), while the dynamic type is the object that the pointer points to at runtime. When downcasting, it is essential to know both to ensure safe and correct type usage.
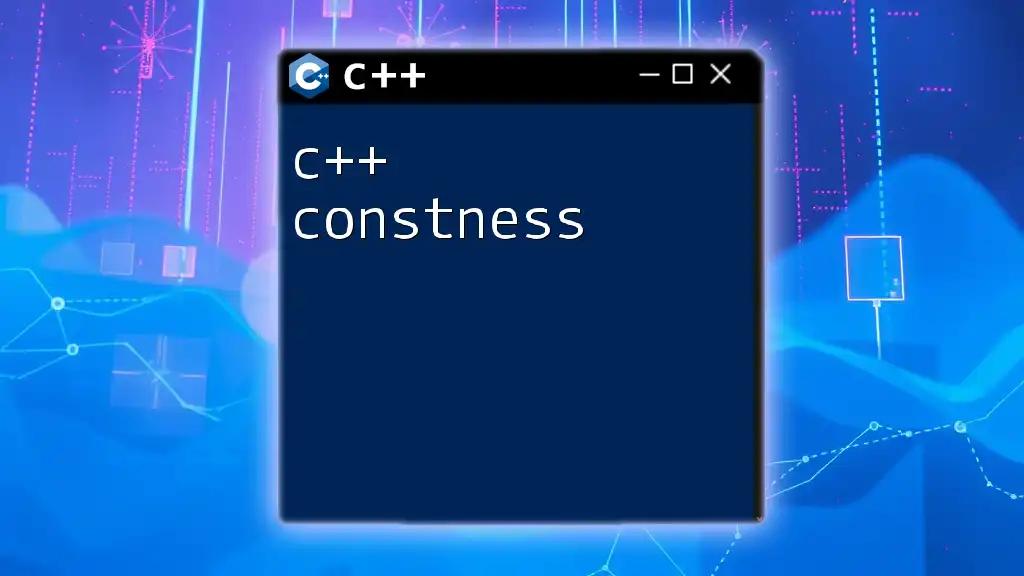
Types of Casting in C++
C++ Casting Operators
C++ provides four primary casting operators:
- `static_cast`: performs compile-time checks; does not check for valid types at runtime.
- `dynamic_cast`: performs runtime checks to ensure safe downcasting.
- `const_cast`: used to cast away the const-ness of an object.
- `reinterpret_cast`: for low-level reinterpreting of bit patterns and potentially unsafe casts.
Dynamic Cast
What is `dynamic_cast`?
`dynamic_cast` is a casting operator designed specifically for downcasting in polymorphic class hierarchies. It checks at runtime whether the object pointed to by the base class pointer is indeed of the derived class type. If the downcast is valid, it returns a pointer to the derived class; if not, it returns `nullptr`.
When to Use `dynamic_cast`
Utilize `dynamic_cast` when you need to ensure type safety in your downcasting. It's particularly useful when you are working with large class hierarchies or when base class pointers may point to different derived objects.
Example of `dynamic_cast`
class Base {
virtual void foo() {}
};
class Derived : public Base {
void foo() override {}
};
Base* b = new Derived(); // Upcasting
Derived* d = dynamic_cast<Derived*>(b); // Downcasting
if (d) {
// Downcast was successful
d->foo();
} else {
// Downcast failed
}
In this example, `dynamic_cast` confirms that the pointer `b` is indeed pointing to a `Derived` instance before it attempts to use it.
Static Cast
What is `static_cast`?
`static_cast` is less safe than `dynamic_cast`, as it performs no runtime checks. It simply compiles the code, assuming the programmer knows the object types involved. Consequently, if the cast is invalid, it may lead to undefined behavior.
When to Use `static_cast` for Downcasting
Use `static_cast` only when you're sure about the type of the objects involved. It’s suitable in scenarios where you control and understand the class hierarchy, thus reducing the risks associated with incorrect downcasting.
Example of `static_cast`
Base* b = new Derived(); // Upcasting
Derived* d = static_cast<Derived*>(b); // Downcasting without check
d->foo(); // Assumes b is actually a Derived
Here, `static_cast` performs the downcast without any viability checks, which can be risky if the base class pointer `b` does not point to a `Derived` object.
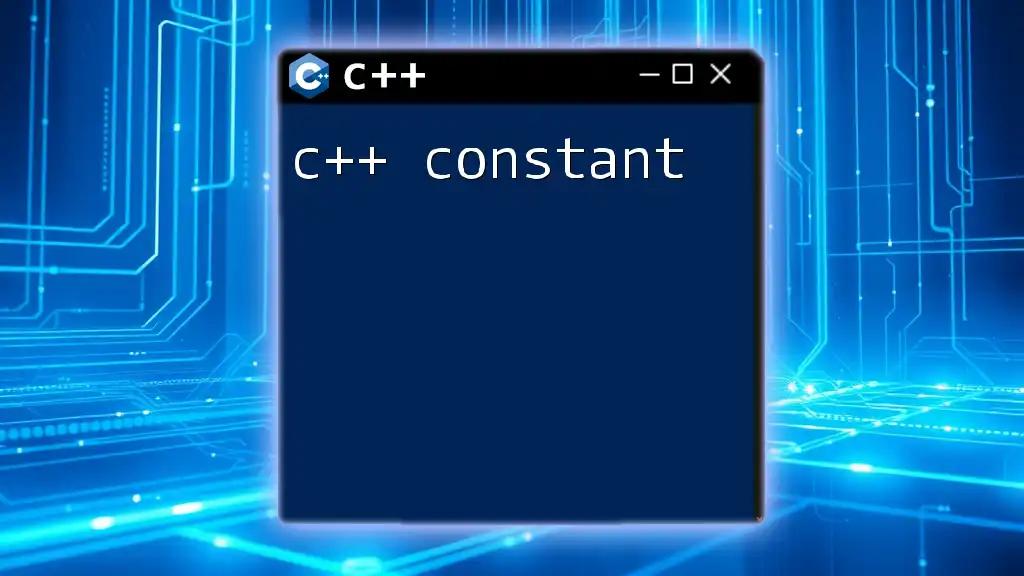
Safety Considerations with Downcasting
Why Downcasting Can Be Dangerous
Downcasting introduces risks because if a base class pointer points to an object of an incompatible derived class, attempting to access members specific to the derived class can result in undefined behavior, crashes, or program errors.
Using `typeid` for Type Safety
To mitigate the risks associated with downcasting, you can use the `typeid` operator to ensure the object is indeed of the correct type before attempting to cast.
Example of Type Check with `typeid`
if (typeid(*b) == typeid(Derived)) {
Derived* d = static_cast<Derived*>(b);
d->foo();
} else {
// Handle the error
}
In this example, `typeid` checks the actual type of the object pointed to by `b`, safeguarding against unsafe downcasting.

Best Practices for Downcasting
Minimizing the Need for Downcasting
One of the best approaches is to minimize the scenarios where downcasting is necessary. This can be achieved by careful design of your class hierarchy and utilizing virtual functions effectively.
Alternatives to Downcasting
Consider employing interfaces or abstract classes that expose common functionalities without requiring explicit downcasting. Additionally, adopting design patterns like the Visitor pattern can help manage different object types without relying on downcasting, leading to cleaner and more maintainable code.
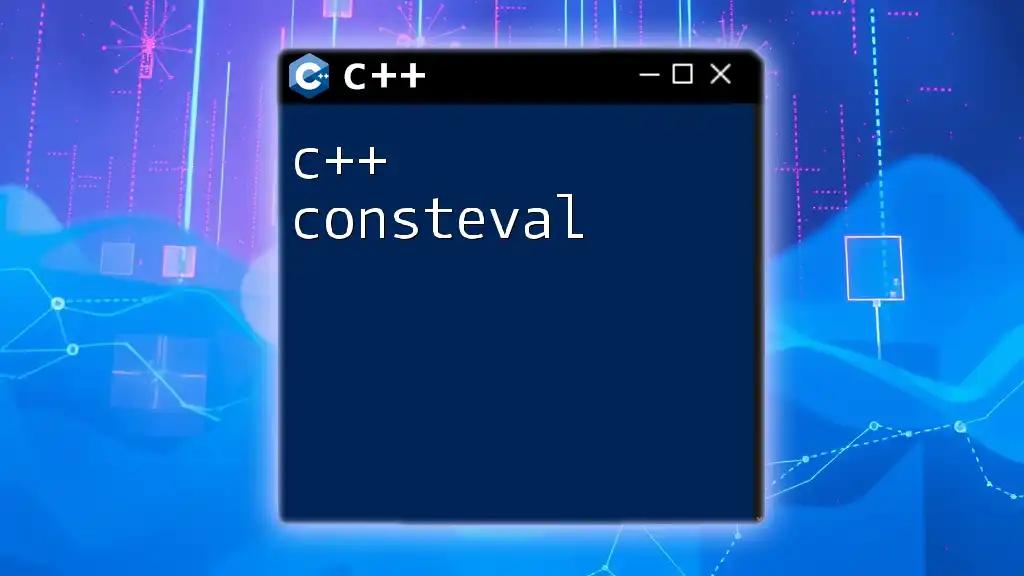
Conclusion
In summary, understanding C++ downcasting is vital for utilizing polymorphism effectively within class hierarchies. The choice between `dynamic_cast` and `static_cast`, along with adhering to best practices and implementing safety checks, can greatly enhance type safety and reduce the risks of runtime errors. Mastery of these concepts allows developers to write robust, object-oriented code in C++. For further learning, consider exploring more C++ resources and practical coding.