A constant pointer in C++ is a pointer whose address cannot be changed after initialization, meaning it always points to the same memory location, while still allowing modification of the value stored at that location.
int value = 42;
int* const ptr = &value; // ptr is a constant pointer to an int
*ptr = 100; // This is allowed, value is now 100
// ptr = &anotherValue; // This line would cause a compilation error
What is a Constant Pointer?
A constant pointer is a pointer whose address cannot be changed once it is assigned. This means that while you can modify the value that the pointer points to, you cannot make the pointer point to a different address. This feature is crucial, especially in situations where maintaining the integrity of the pointer is necessary.
To clarify further, it’s important to distinguish between a constant pointer and a const pointer. A constant pointer maintains its address, while a const pointer prevents modification of the value it points to.
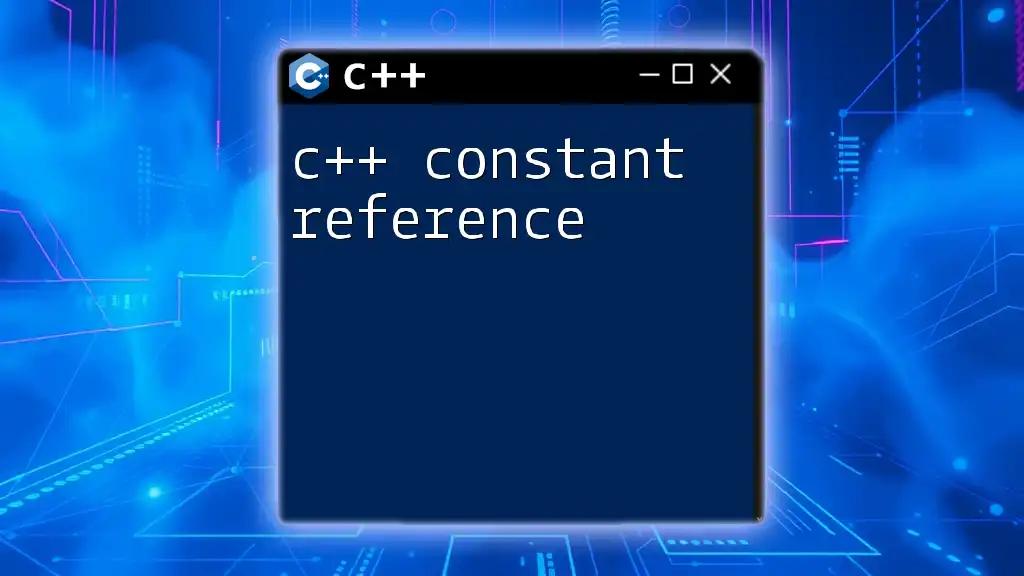
Understanding the Syntax
The syntax for declaring a constant pointer uses the `const` keyword after the asterisk that denotes a pointer. For example:
int* const ptr = new int(5);
In this declaration, `ptr` is a constant pointer to an integer. You can see that:
- `int*`: Indicates it points to an integer.
- `const ptr`: Shows that `ptr` itself cannot be reassigned to point elsewhere.
Understanding this syntax is crucial as it forms the basis of utilizing constant pointers properly in your C++ programs. The placement of `const` is key, affecting the behavior of your code significantly.

How to Declare and Initialize Constant Pointers
Declaring and initializing constant pointers is straightforward. Start by defining the type of data to be pointed to, followed by the `const` keyword, and finally assign a value to it.
Here’s how it looks in practice:
const int* p1 = new int(10); // Const pointer to an int
int* const p2 = new int(20); // Constant pointer to an int
In the above code:
- `p1` is a constant pointer; however, the integer it points to can be modified.
- `p2` is a constant pointer that cannot be reassigned to point to another integer, although the integer it points to can still be modified.
Use Cases: Constant pointers are particularly useful when you want to ensure that a pointer remains attached to a specific memory location while allowing changes to the data it references. They prevent accidental reassignment, which could lead to undefined behaviors or memory leaks.

Accessing Values via Constant Pointers
Accessing and modifying values through constant pointers is straightforward. Since you can still dereference constant pointers, you can retrieve and manipulate the value at the pointed-to address.
Here’s a simple example:
int value = *p2; // Accessing the value pointed by the constant pointer
In this line, we dereference `p2` to get the integer value it points to.
Additionally, you can modify the value pointed to by the constant pointer:
*p2 = 30; // Valid operation
However, keep in mind that you cannot reassign the constant pointer itself:
p2 = new int(25); // Error: Cannot reassign a constant pointer
Thus, understanding how to correctly access and manipulate values via constant pointers is vital for effective programming.

The Role of Constant Pointers in Function Parameters
Using constant pointers as function parameters provides additional safety and performance benefits. It helps ensure that the function cannot change which object the pointer is pointing to, reducing the risk of bugs.
Consider this simple function which demonstrates the usage of a constant pointer:
void modifyValue(int* const ptr) {
*ptr = 100; // Modifying the value pointed to
}
In this example, `modifyValue` takes a constant pointer as an argument. Inside the function, you can modify the integer value but not reassign `ptr` to point somewhere else.
Benefits of Using Constant Pointers in Functions:
- Performance Improvements: Pass by pointer rather than value, which is more efficient for large data structures or objects.
- Preventing Accidental Changes: It safeguards against accidental pointer reassignment, maintaining the stability of your data during function execution.
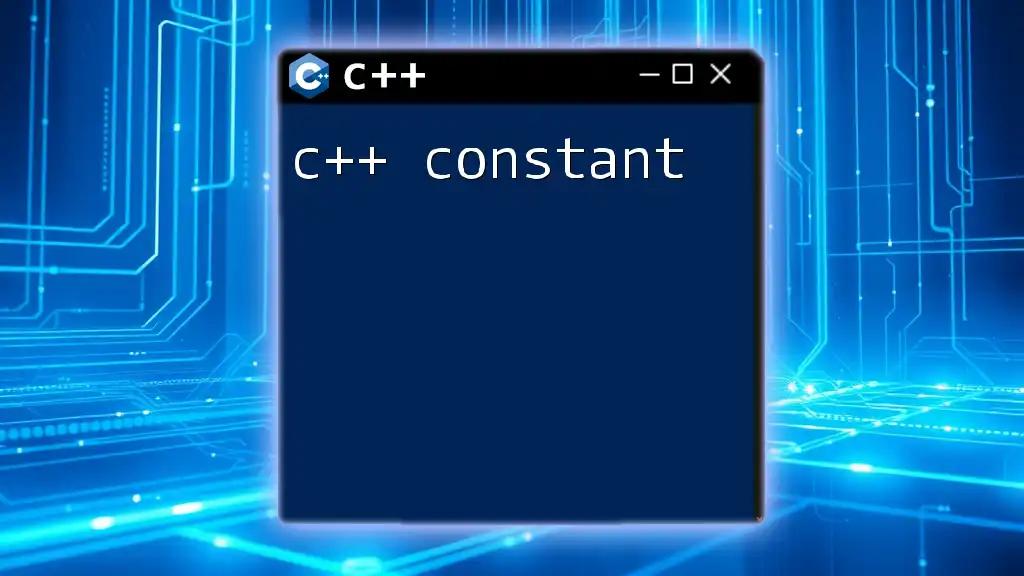
Common Mistakes When Working With Constant Pointers
Even experienced developers can encounter pitfalls when using constant pointers. Some of the most common mistakes include:
- Forgetting Const Correctness: Failing to declare a pointer or variable as `const` leading to unintentional changes in code logic.
- Reassigning a Constant Pointer: Attempting to reassign a pointer that has been declared as constant, which results in a compilation error. For instance:
p2 = new int(15); // Error: Cannot reassign a constant pointer

Best Practices for Using Constant Pointers
To maintain clean and effective code when working with constant pointers, consider the following best practices:
- Always Initialize Constant Pointers: Make it a point to initialize constant pointers upon declaration to avoid undefined behaviors.
- Use Constant Pointers in Complex Structures: Leverage constant pointers when designing complex data structures like linked lists, where you want to ensure that pointers remain fixed while allowing modifications to the nodes.
For example, a simple struct with a constant pointer may look like this:
struct Node {
int value;
Node* const next; // Constant pointer to the next node
};
This structure ensures that the pointer to the next node remains unchanged throughout the node's lifecycle, thus preserving the integrity of the linked list.
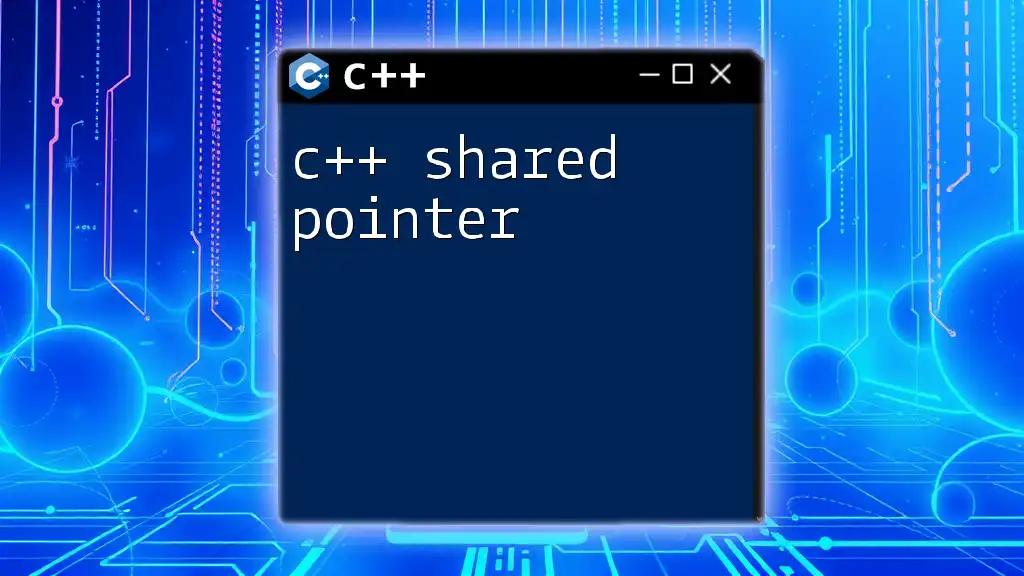
Conclusion
Understanding C++ constant pointers is vital for writing robust and maintainable code. By keeping your pointers constant where necessary, you avoid common pitfalls and improve the clarity and reliability of your programs. Constant pointers allow for flexibility with memory management while ensuring strict adherence to specific addresses, preventing inadvertent errors.
Encourage yourself to explore and practice with constant pointers further, as their effective usage can lead to considerable improvements in your coding efficiency and code quality. Through careful application, you can master this important aspect of C++ programming.

Additional Resources
- Use online compilers to experiment with code snippets.
- Check out recommended books and online courses to deepen your understanding of pointers and other C++ features. Investigate FAQs to see how others have navigated common questions surrounding constant pointers in C++.