In C++, the `printf` function from the C standard library allows for formatted output, utilizing format specifiers to control the layout of the printed data.
#include <cstdio>
int main() {
int age = 25;
printf("I am %d years old.\n", age);
return 0;
}
Understanding Output in C++
C++ uses the standard output stream, `std::cout`, for displaying information to the user. Properly formatting this output not only enhances readability but also ensures effective communication of the program's intent. Without formatting, output can appear cluttered or unclear, making it difficult for users to interpret.
To demonstrate basic output, consider the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program illustrates how to send text to the console. However, as programs grow more complex, the need for well-formatted output becomes critical.
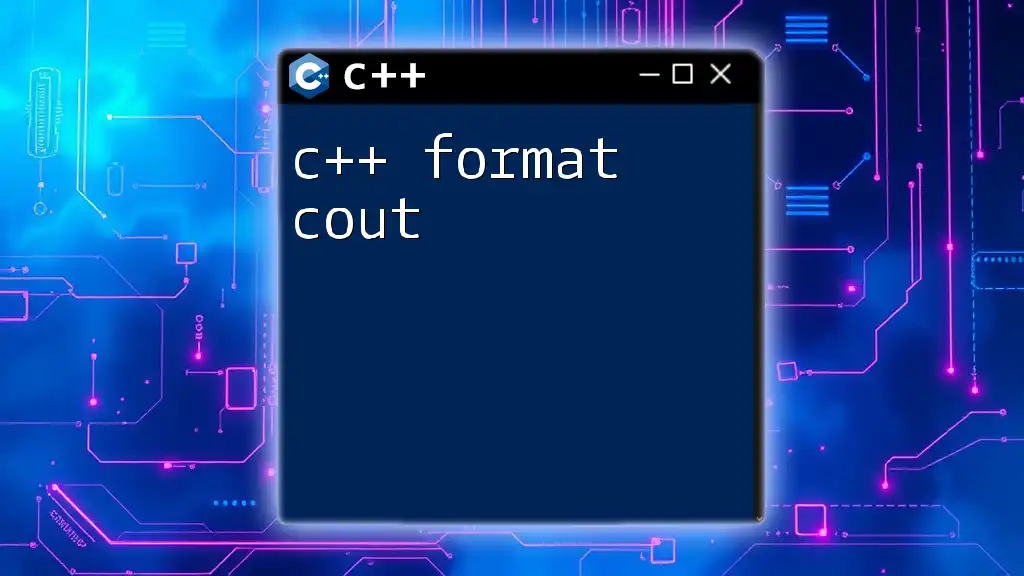
Introduction to Formatting in C++
What is Formatting?
Formatting in C++ refers to the methods and techniques used to control the appearance of output. Well-structured output improves user experience, making it easier to read and understand the information presented.
The `iomanip` Library
The `<iomanip>` header defines several useful manipulators to control the format of input/output. It is essential to include this library to access various formatting functions like setting precision, width, and fill characters. The importance of this library cannot be overstated, as it provides tools that enhance your control over output presentation.
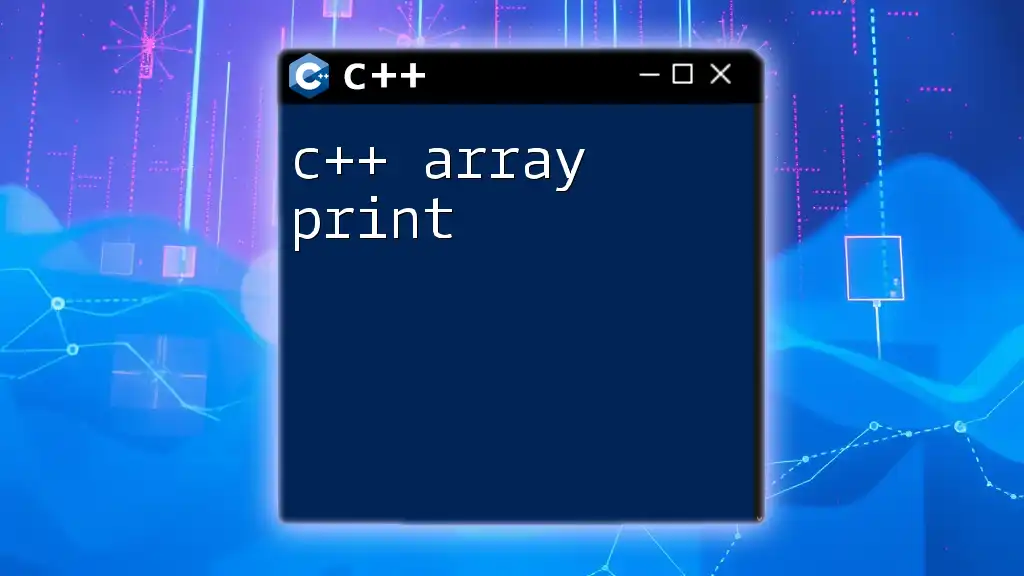
Formatting Methods in C++
Using `std::setw`
The `std::setw` manipulator is essential for controlling the width of the output. This can be particularly useful for aligning columns of data.
Explanation and Purpose
When using `std::setw`, you specify the minimum width for the next output field. If the output exceeds this width, it will expand to accommodate the content. However, if the content is shorter, it will be padded with spaces (or another character if specified).
Code Example
Consider the following example, which demonstrates the use of `std::setw` for aligned output:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Item" << std::setw(5) << "Qty" << std::endl;
std::cout << std::setw(10) << "Apple" << std::setw(5) << 5 << std::endl;
std::cout << std::setw(10) << "Banana" << std::setw(5) << 10 << std::endl;
return 0;
}
This code sets the width for the columns, making it easy to read.
Using `std::setprecision`
Precision is crucial when dealing with floating-point numbers. The `std::setprecision` manipulator defines the number of digits displayed after the decimal point.
Explanation of Precision in Output
Precision settings become particularly important in scientific calculations or monetary values, where displaying an appropriate number of decimal places is essential.
Example of Setting Precision
Here's an example of using `std::setprecision`:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
This outputs `3.14`, showing only two decimal places, making the output cleaner and more structured.
Using `std::fixed` and `std::scientific`
Understanding the difference between fixed and scientific notation is vital for displaying numerical data correctly.
Fixed vs Scientific Notation
- Fixed: Displays floating-point numbers as regular decimal values.
- Scientific: Displays numbers in exponential form, which is useful for very large or very small values.
Code Snippet
Here is how to implement both formats:
#include <iostream>
#include <iomanip>
int main() {
double number = 123456.789;
std::cout << "Fixed: " << std::fixed << number << std::endl;
std::cout << "Scientific: " << std::scientific << number << std::endl;
return 0;
}
This program displays the number in both formats, allowing you to see how the representation changes based on the chosen formatting style.
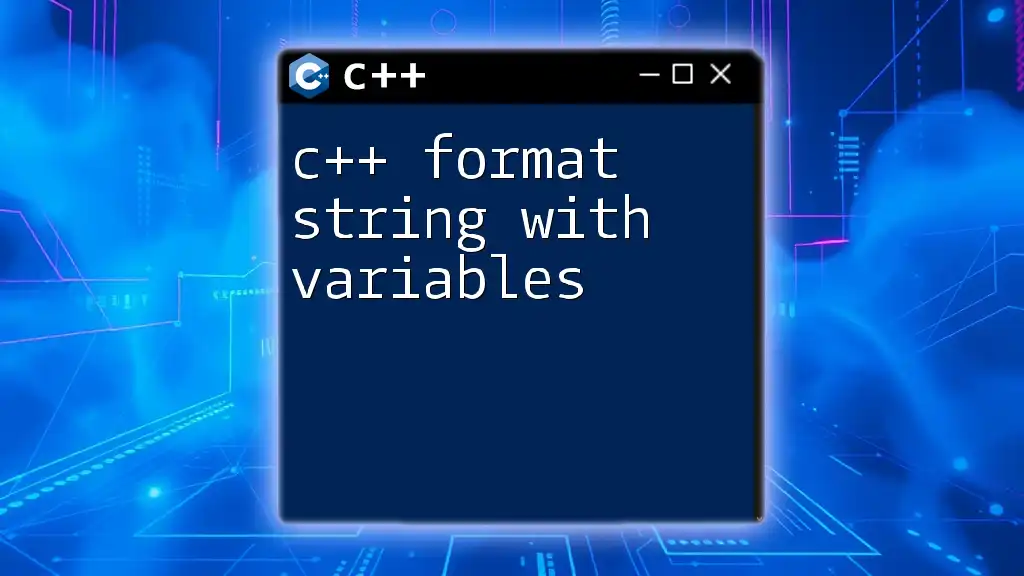
Advanced Formatting Techniques
Colored Output using ANSI Escape Codes
For programs with a command-line interface, adding color can significantly enhance user experience through better visual cues.
Introduction to ANSI Codes
ANSI escape codes provide a way to format text with colors and styles in the terminal. While platform-dependent, they are widely supported in various environments.
Code Example for Colored Output
You can use the following example to print colored text in the console:
#include <iostream>
int main() {
std::cout << "\033[31mThis is red text\033[0m" << std::endl; // Red text
std::cout << "\033[32mThis is green text\033[0m" << std::endl; // Green text
return 0;
}
This snippet sets the text color to red and green, respectively, showcasing how to draw attention to specific outputs.
Custom Format Specifiers using `std::format` (C++20)
With the arrival of C++20, the `std::format` feature provides a modern and powerful way to format output.
Overview of `std::format`
Unlike traditional formatting methods, `std::format` allows you to embed expressions directly into your format string, substantially improving code readability.
Example Code Snippet
Here’s how to use `std::format`:
#include <iostream>
#include <format>
int main() {
int age = 30;
std::cout << std::format("I am {} years old.", age) << std::endl;
return 0;
}
This code demonstrates the simplicity and clarity of using `std::format`, which neatly incorporates variable values into strings.
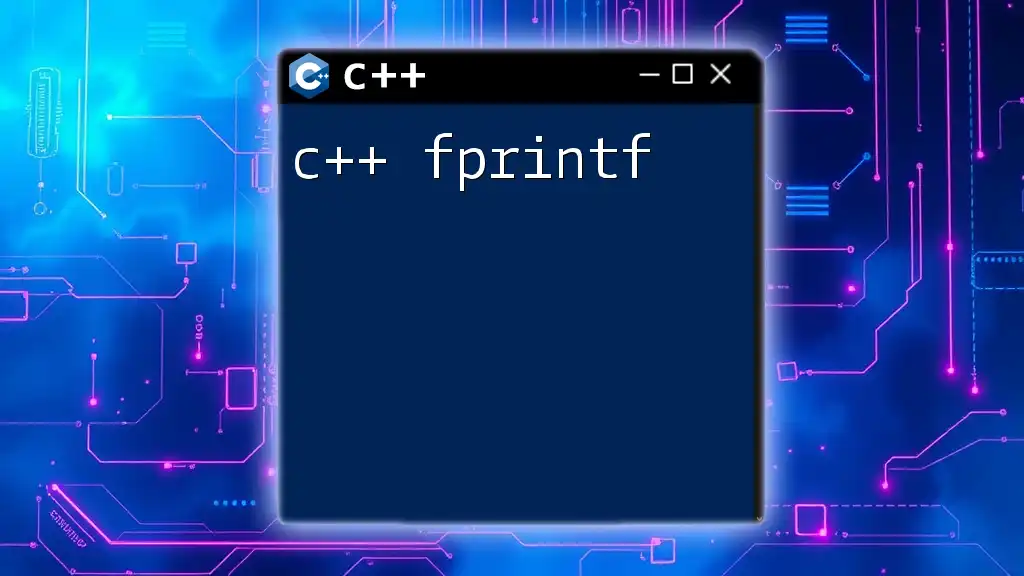
Common Formatting Errors and Troubleshooting
Miscalculations in Width and Precision
One common pitfall with formatting is miscalculating widths and precisions, leading to unexpected output. Always double-check values passed to `std::setw` and `std::setprecision` to ensure they align with your intentions.
Debugging Output Issues
When troubleshooting formatted output, it is useful to print variable contents alongside formatted strings. Consider using temporary variables to evaluate what is being outputted at each step.
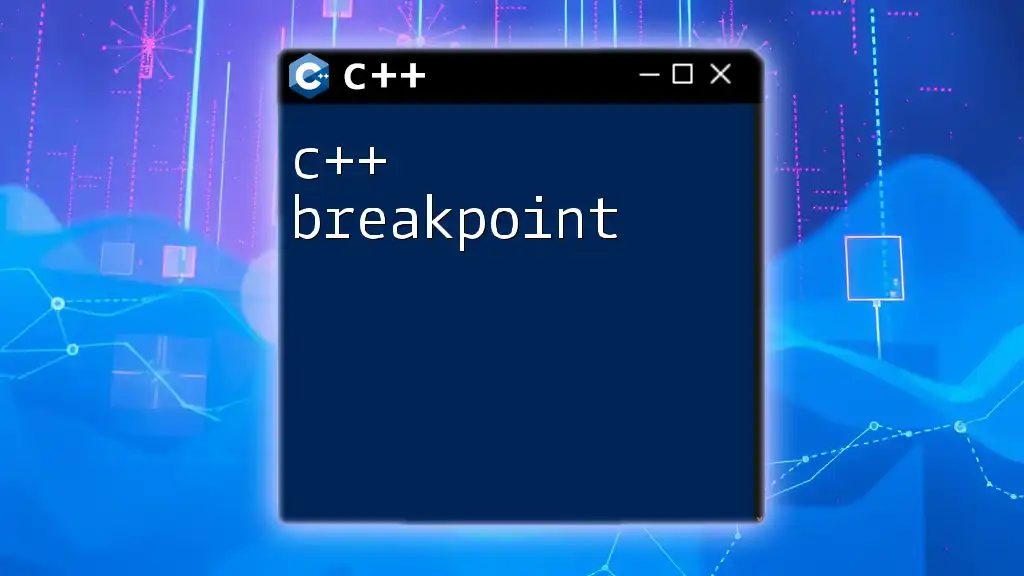
Best Practices for Formatting Output
Clarity and Readability
Always prioritize clear, readable output. Avoid overly complex formatting that may confuse users. Simple and logical structures go a long way in user satisfaction.
Consistency in Formatting
Maintaining a consistent format across your application strengthens professionalism and reliability. Consider using a central output utility function to standardize your formatting style.
Using Comments for Clarity
Commenting your code, especially where formatting commands appear, can aid in future maintenance and understanding. It will also help others working on your code or even your future self.
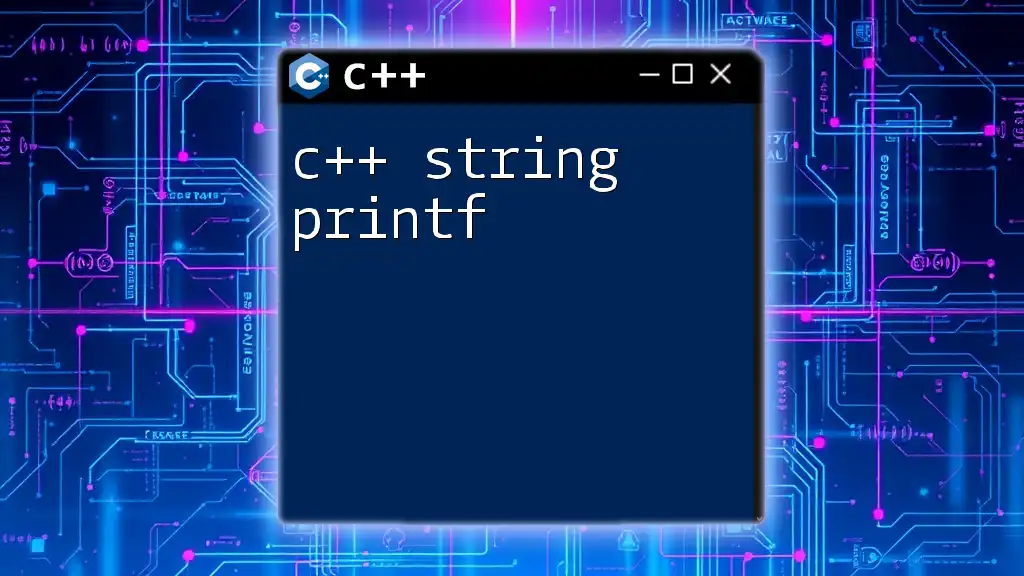
Conclusion
Effective formatting in C++ output is more than just a cosmetic choice; it serves to enhance clarity and user experience. By mastering manipulative functions like `std::setw`, `std::setprecision`, and the newer `std::format`, you empower your programs to communicate information clearly and effectively.
For a deeper dive into specific C++ features, keep exploring and practice implementing these techniques in your coding projects!