The `restrict` keyword in C++ is used to indicate that a pointer is the only reference to the object it points to, allowing for potential optimization by the compiler.
void exampleFunction(int* restrict ptr1, int* restrict ptr2) {
*ptr1 = 5; // Modifies the value pointed to by ptr1
*ptr2 = 10; // Modifies the value pointed to by ptr2
}
Understanding the C++ Restrict Keyword
What is the `restrict` Keyword?
The restrict keyword is primarily a C feature introduced to help the compiler optimize memory access. In C++, its use is not as common as in C, but it can still provide significant performance improvements when applied correctly. By declaring a pointer with the restrict qualifier, you assert to the compiler that, for the lifetime of the pointer, no other pointer will reference the same memory location. This information allows the compiler to make optimizations that can improve the performance of your program.
Why Use `restrict`?
One of the fundamental ways restrict enhances performance is through efficient memory access. When the compiler knows that pointers do not overlap, it can generate more optimized code that exploits the underlying hardware architecture better. This can reduce cache misses and improve memory throughput. Essentially, using restrict enables developers to offer hints to the compiler about the expected behavior of pointer access, allowing it to make better decisions during the optimization phase.
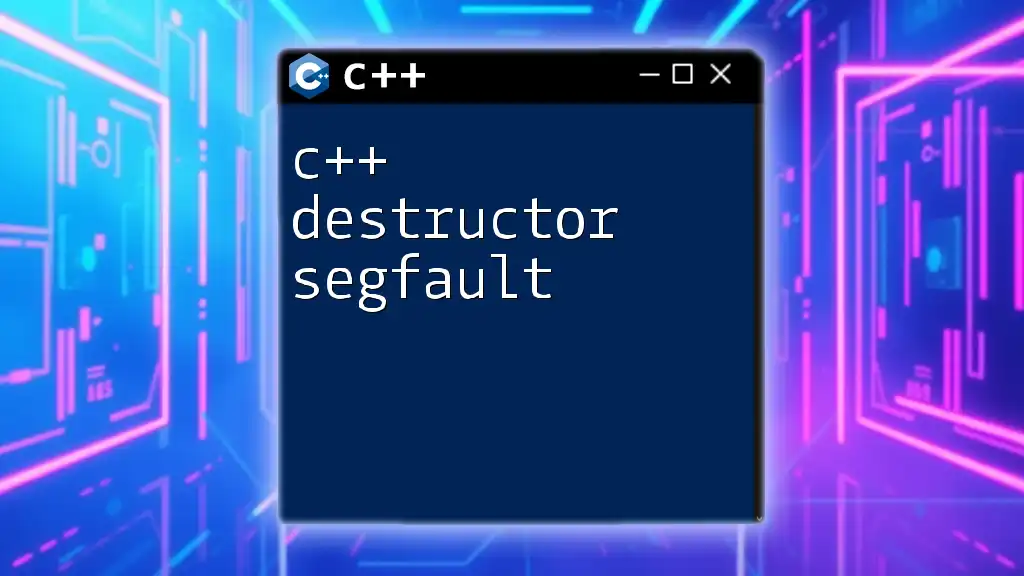
The Role of `restrict` in C++
Syntax of the Restrict Keyword
To use the restrict keyword in C++, you generally place it in the declaration of a pointer. Here’s a basic example:
void process(int* __restrict p, int* __restrict q) {
// Function implementation
}
In this code snippet, both pointers `p` and `q` are marked as restrict. This declaration implies that during the execution of the `process` function, the pointers will not point to overlapping memory regions. The compiler leverages this information to optimize memory accesses efficiently.
Function Pointer Usage with `restrict`
The restrict keyword can also be beneficial when using function pointers. For instance, consider this example where restrict is applied to enhance a core function's performance:
void updateData(int* __restrict dataA, int* __restrict dataB) {
*dataA = *dataB;
}
Here, the function updateData modifies the value of `dataA` based solely on the value pointed to by `dataB`, asserting that `dataA` and `dataB` do not overlap in memory.
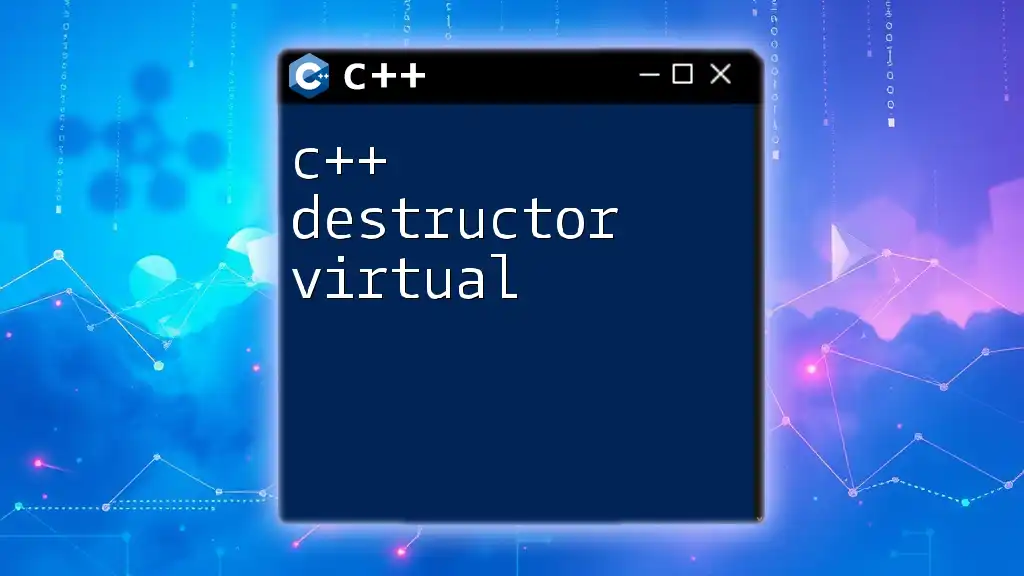
Practical Applications of the `restrict` Keyword
Performance Enhancements
Memory Access Patterns
Memory access patterns significantly impact the performance of an application, particularly in performance-critical scenarios. The restrict keyword allows the compiler to implement caching strategies more effectively. Consider this implementation:
void compute(int* __restrict arr1, int* __restrict arr2, int* __restrict out, int length) {
for (int i = 0; i < length; ++i) {
out[i] = arr1[i] + arr2[i];
}
}
In this code, the compiler can assume that `arr1`, `arr2`, and `out` point to different locations in memory. This knowledge lets it optimize the loop, possibly by reducing cache loading times and vectorizing the operations based on the given constraints.
Real-world Use Cases
The tangible benefits of restrict appear in environments such as high-performance computing (HPC), digital signal processing (DSP), and embedded systems. Algorithms that process large datasets can see marked improvement in performance. For instance, matrix multiplication or image processing tasks often benefit significantly from clearer memory access patterns enforced through restrict.
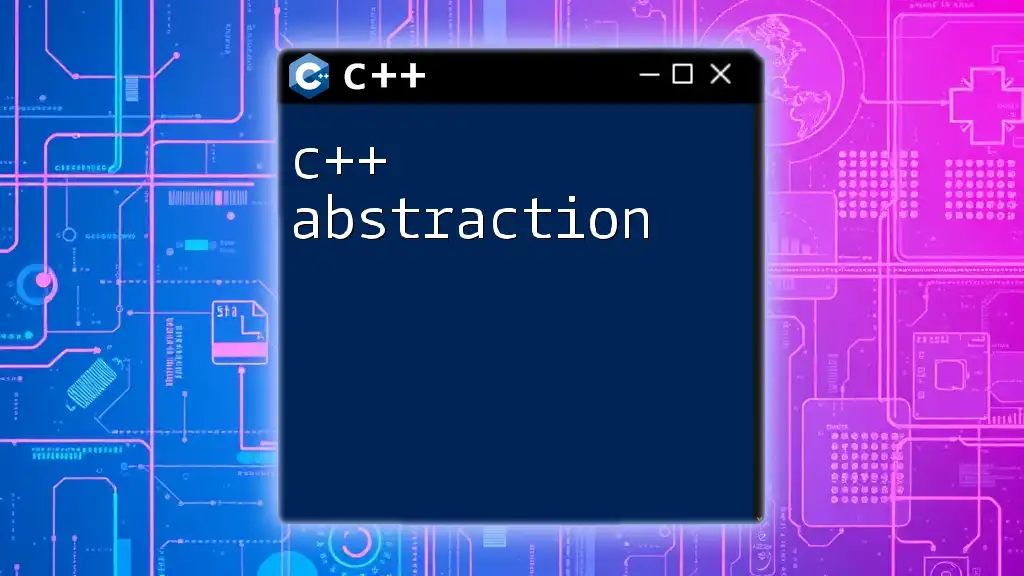
Limitations of the `restrict` Keyword
When Not to Use `restrict`
While the restrict keyword offers performance improvements, it's not suitable for every situation. In scenarios where pointers could overlap due to complex data manipulations, using restrict can lead to undefined behavior in your application. Therefore, it’s crucial to have a thorough understanding of the code to prevent erroneous assumptions about memory access.
Compatibility and Portability Concerns
Though most modern compilers support the restrict keyword, there are variations in implementation and full compliance. For maximum portability, it’s essential to check compiler documentation and adopt conditional compilation when necessary, ensuring that your code remains functional across different environments and systems.
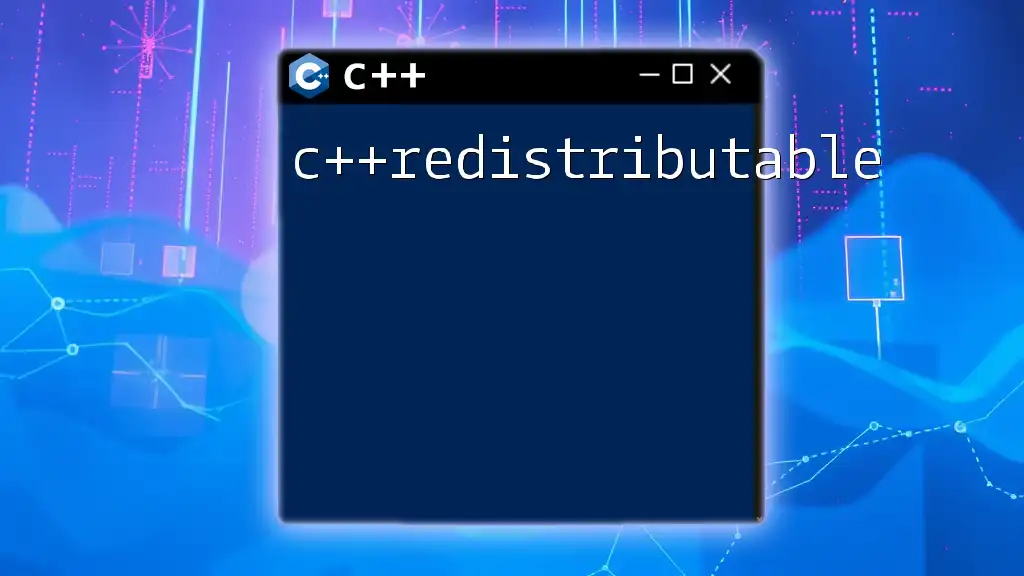
Best Practices for Using `restrict`
Guidelines for Effective Use
To leverage the restrict keyword effectively, consider the following practices:
-
Clear Code Comments: Always annotate your usage of restrict with comments explaining the reasoning, as this can greatly enhance code readability.
-
Isolate Use Cases: Aim to use restrict in performance-sensitive code sections where the benefits can be measured.
-
Benchmark Performance: Implement performance tests to evaluate the impact of adding the restrict qualifier. Sometimes, the gains might be insignificant compared to the added complexity.
Debugging Techniques for Code Using `restrict`
When debugging code that utilizes restrict, pay close attention to pointer assignments. Any inadvertent overlap caused by pointer reassignment or array manipulations could lead to unexpected results. Tools that analyze memory access patterns can assist in identifying potential clashes, and consider using assertions to check the validity of pointer states at various stages.
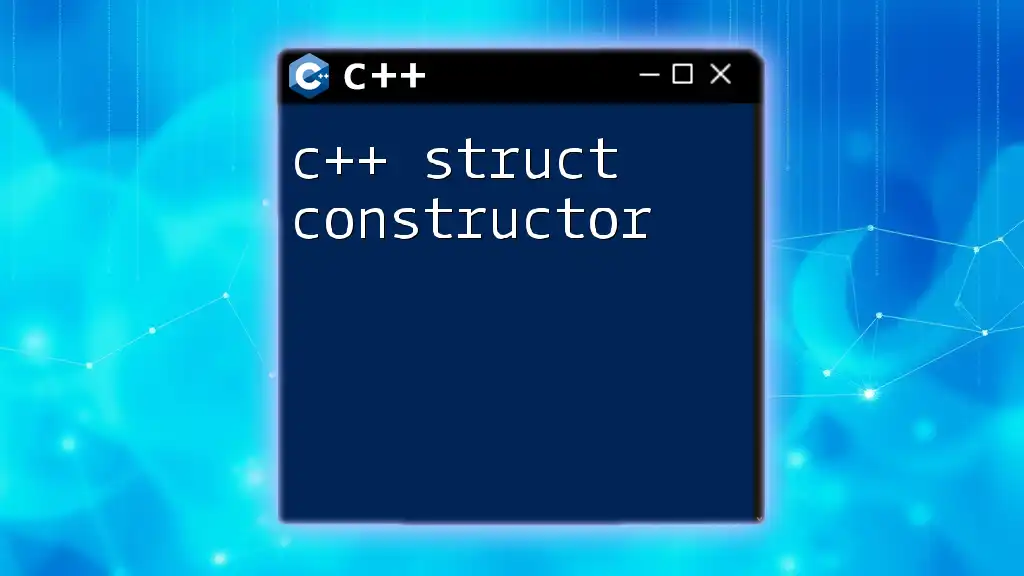
Conclusion
The C++ restrict keyword is a powerful tool for developers aiming to optimize their applications. By providing the compiler with information on memory access patterns, the restrict keyword enables enhanced performance, particularly in computationally intensive scenarios. Understanding when and how to apply restrict effectively can lead to significant benefits in your codebase. So, dive into your projects, explore the capabilities of restrict, and watch the performance of your applications soar.
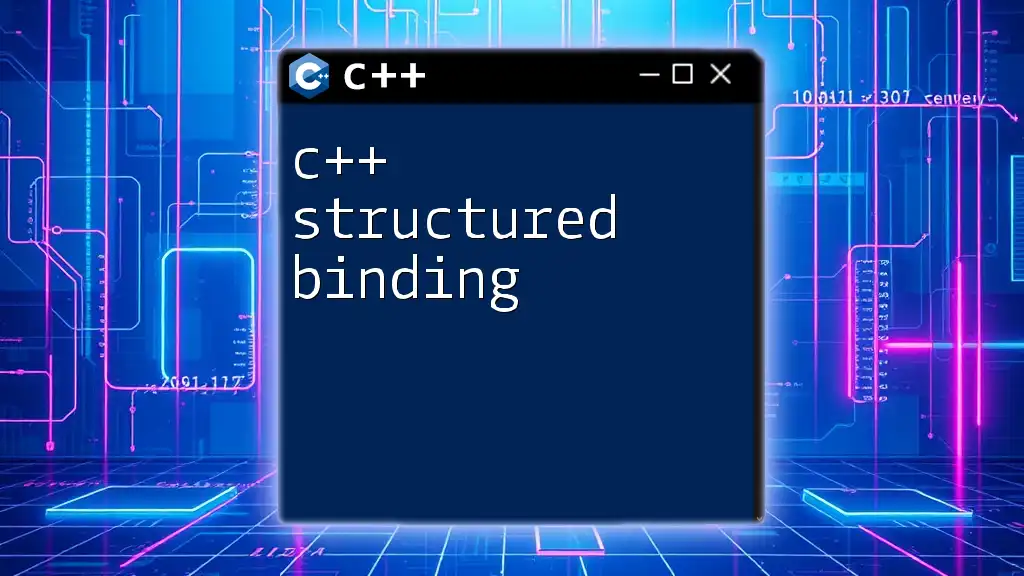
Additional Resources
Books and Online References
- For further exploration of the restrict keyword and performance optimizations in C++, refer to specialized programming literature and compiler optimization guides.
Example Projects
- Consider contributing to or studying open-source projects that utilize restrict effectively to see practical implementations and gain insights into the benefits it can bring to a codebase.