The `strchr` function in C++ is used to locate the first occurrence of a specified character in a string, returning a pointer to that character or `nullptr` if the character is not found.
#include <cstring>
#include <iostream>
int main() {
const char* str = "Hello, World!";
char ch = 'o';
const char* result = strchr(str, ch);
if (result) {
std::cout << "Character '" << ch << "' found at position: " << (result - str) << std::endl;
} else {
std::cout << "Character not found." << std::endl;
}
return 0;
}
Understanding the Syntax of `strchr`
The function `strchr` is part of the C++ standard library, specifically included in the `<cstring>` header file. It is crucial for manipulating C-style strings (character arrays). The syntax of `strchr` can be broken down as follows:
char* strchr(const char* str, int character);
Parameters:
- `const char* str`: This parameter is the input string where the search will take place. It is important to note that this string should be a null-terminated string.
- `int character`: This parameter represents the character you want to locate in the string. It is passed as an integer, which allows you to use character literals directly.
Return Value:
- The function returns a pointer to the first occurrence of the specified character within the string. If the character is not found, it returns `nullptr`.
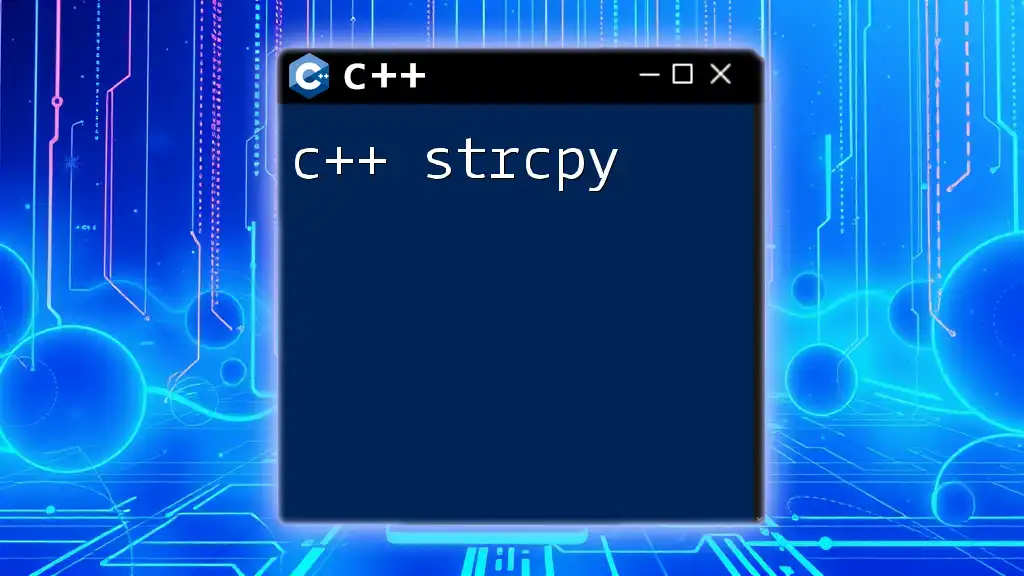
How `strchr` Works
`strchr` operates by scanning the string from the beginning to the end, character by character. When it encounters the specified character, it returns a pointer to that character's position within the string. If it reaches the end of the string and still hasn't found the character, it returns `nullptr`.
Memory Considerations:
Being a pointer-based manipulation function, `strchr` requires a good understanding of memory allocation in C++. Since it deals with the address of the characters in a character array, proper handling of pointers is essential to avoid memory-related issues, such as segmentation faults.
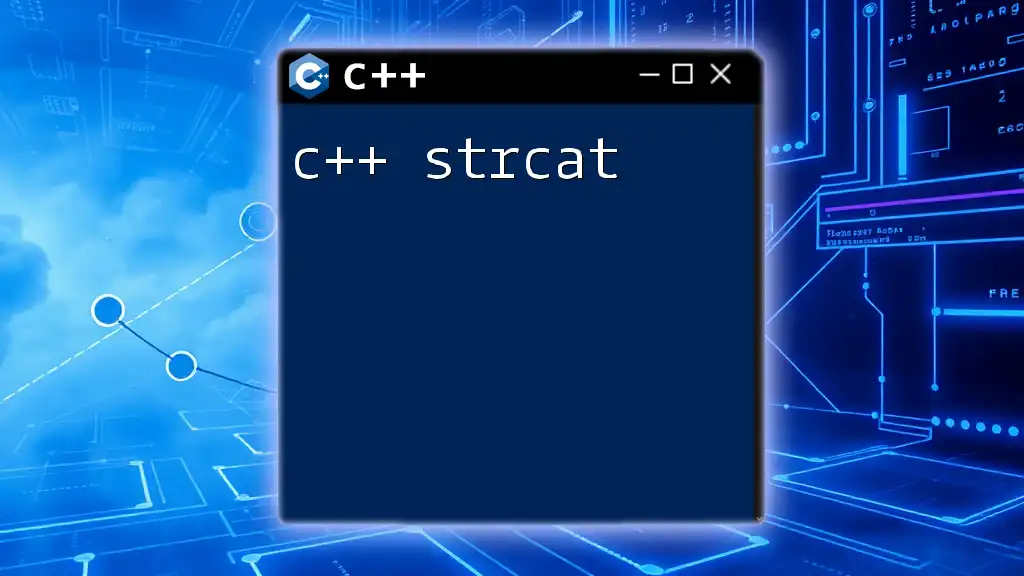
Practical Examples of `strchr`
Basic Example
Let’s look at a straightforward implementation of `strchr` to search for a character in a string.
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello, World!";
const char* result = strchr(str, 'W');
if (result) {
std::cout << "Found: " << result << std::endl; // Output: World!
} else {
std::cout << "Character not found." << std::endl;
}
return 0;
}
In this example, we define a string `"Hello, World!"` and then use `strchr` to look for the character `'W'`. If the character is found, it prints the substring starting from that character; otherwise, it informs the user that the character is not present.
Advanced Example
Sometimes, you may need to find multiple occurrences of a character. Below is a code snippet illustrating how to handle such cases:
#include <iostream>
#include <cstring>
int main() {
const char* str = "banana";
const char* current = str;
char character = 'a';
while ((current = strchr(current, character)) != nullptr) {
std::cout << "Found '" << character << "' at position: " << (current - str) << std::endl;
current++; // Move to the next character
}
return 0;
}
In this example, the loop continues searching for `'a'` until no more occurrences are found. By updating `current` to point one character beyond the last found position, it ensures that the search continues for subsequent occurrences.
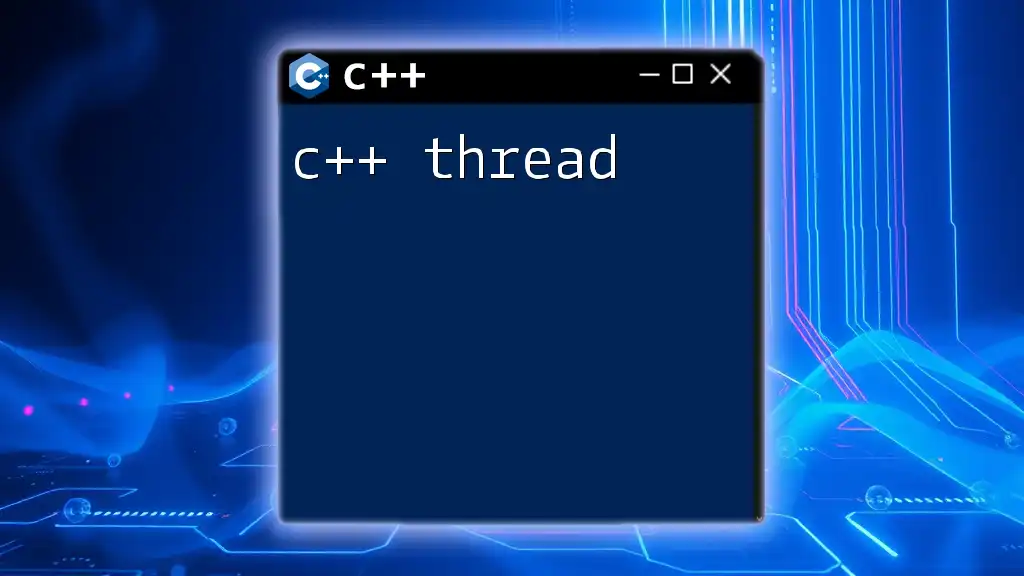
Handling Character Not Found Scenario
While using `strchr`, be mindful that if the character does not exist in the string, it returns `nullptr`. Thus, always check for this condition to prevent dereferencing a `nullptr`, which leads to runtime errors. For instance:
if (result == nullptr) {
// Handle the case where the character isn't found
}
This check allows your program to manage cases where characters are not found gracefully, avoiding crashes and bugs.
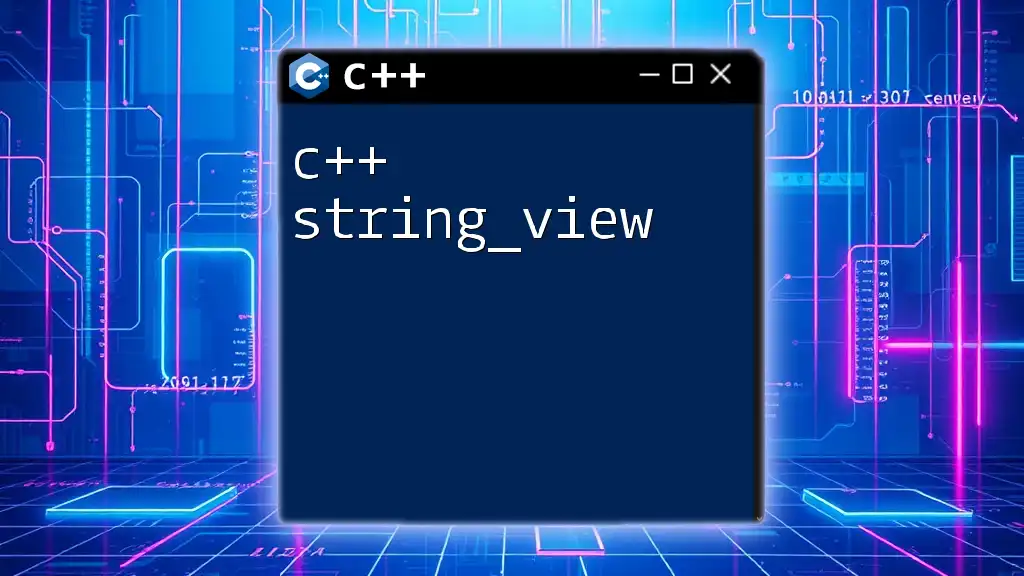
Common Use Cases for `strchr`
`strchr` is frequently utilized in various programming contexts, including:
- Finding Substrings: It can be used to extract parts of a string starting from a specific character.
- Input Validation: When aiming to confirm that a user input meets certain criteria, `strchr` can be employed to check if specific characters are present.
- Parsing and Tokenization: `strchr` aids in breaking down strings into tokens based on specific delimiters, simplifying complex string parsing tasks.
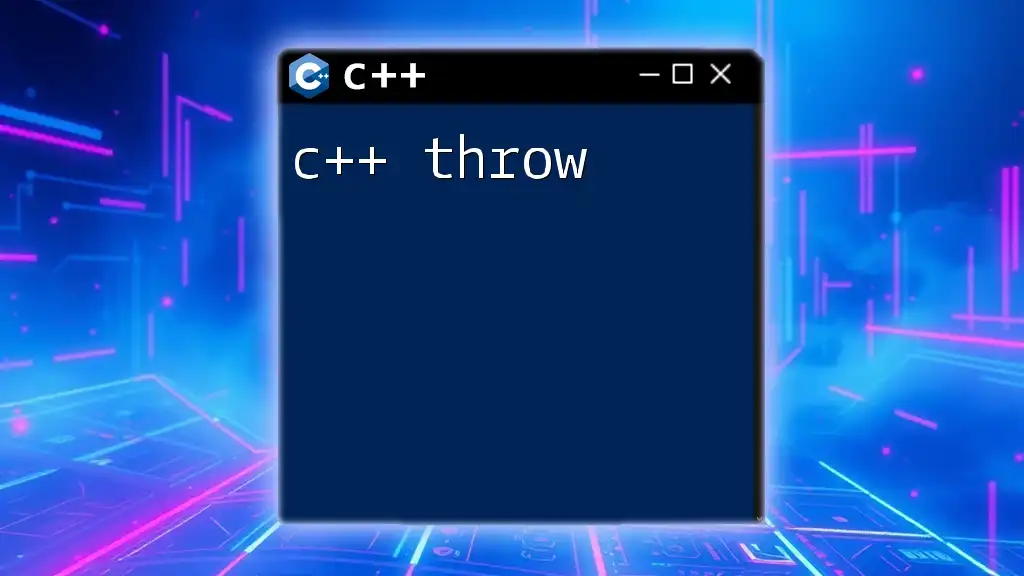
Performance Considerations
While `strchr` is efficient for most string searching needs, realize it scans the string character by character. In scenarios where performance is critical or when dealing with very large strings, consider alternatives or optimizations, such as using other string manipulation functions like `find`, which could provide better performance with modern C++ strings (`std::string`).
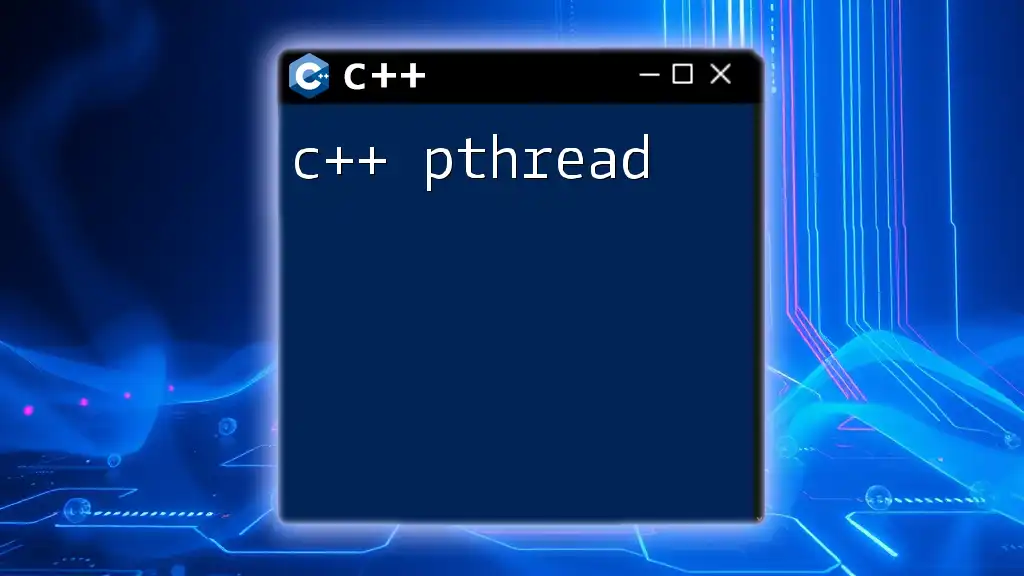
Best Practices When Using `strchr`
- Pointers and Memory Management: Always ensure that the string passed to `strchr` is valid and null-terminated to prevent undefined behavior.
- Error Handling Practices: Always implement checks for `nullptr` to handle cases where the character isn’t found.
- Choosing the Right Character Type: When using `strchr`, remember that the character is treated as an integer. You can use characters directly, but understand the implications of character encoding.
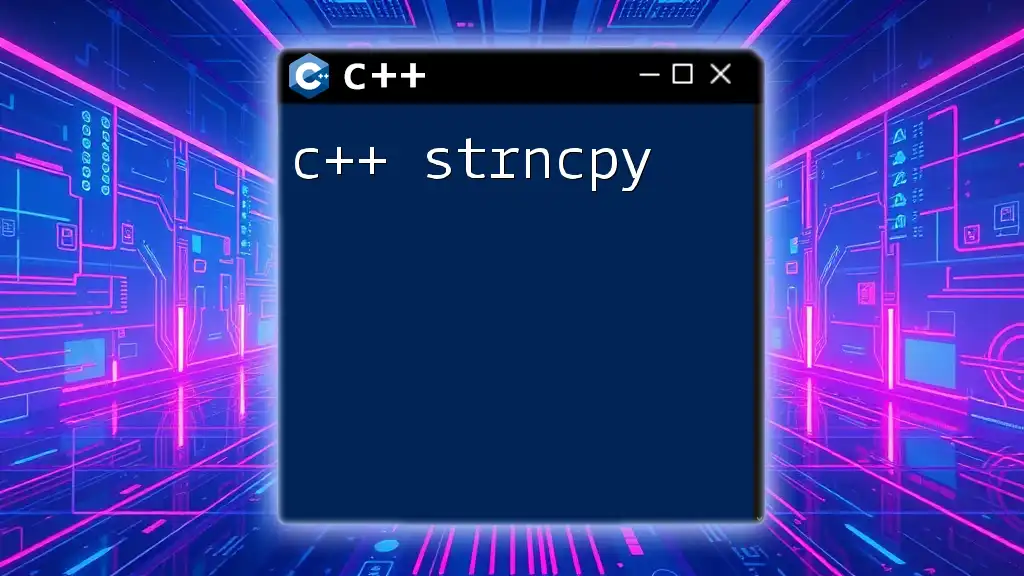
Conclusion
In this guide, we've explored the C++ `strchr` function in depth, from its syntax and functionality to practical applications and best practices. Mastery of this function can greatly enhance your ability to manipulate strings effectively. Practicing with various examples will help solidify your understanding and make you a more proficient C++ programmer.
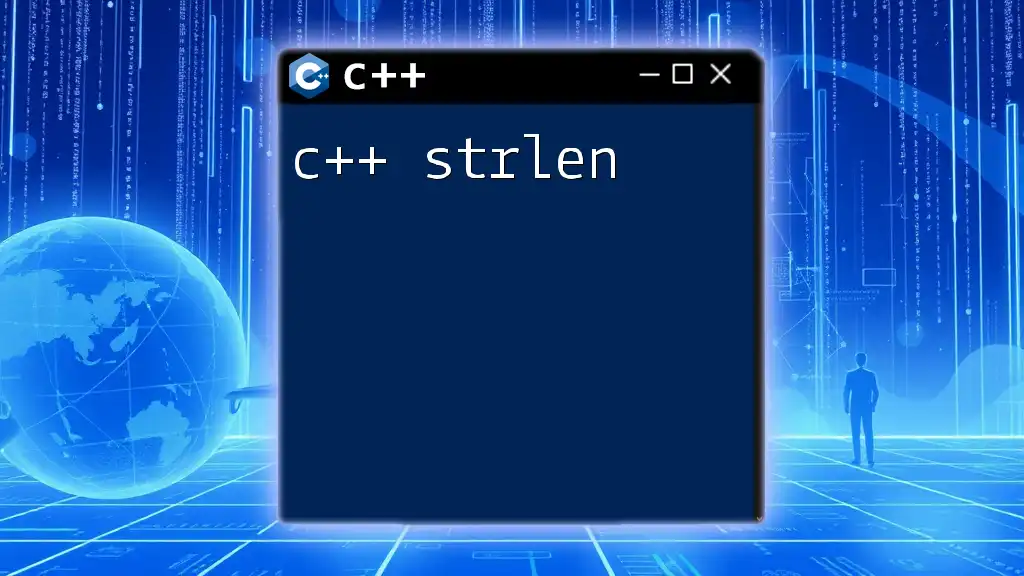
Additional Resources
For further reading, explore the C++ Standard Library documentation, online tutorials on string manipulation, and recommended books focused on C++. As you dive deeper into string handling in C++, you will find that functions like `strchr` are invaluable tools in your programming toolkit.