The `strcpy` function in C++ is used to copy a null-terminated string from a source to a destination buffer.
#include <cstring>
int main() {
char source[] = "Hello, World!";
char destination[50];
strcpy(destination, source);
return 0;
}
What is C++ strcpy?
The `strcpy` function is a fundamental part of string manipulation in C and C++. It is defined in the C Standard Library and allows you to copy one string to another, which is particularly useful for handling C-style strings.
The syntax of `strcpy` is as follows:
char* strcpy(char* destination, const char* source);
Explanation of Parameters:
- destination: This parameter specifies where you want the copied string to be stored. It's crucial that this memory location is large enough to hold the string being copied.
- source: This is the string to be copied. The function reads the string from this location until it encounters the null character (`'\0'`), which signifies the end of the string.
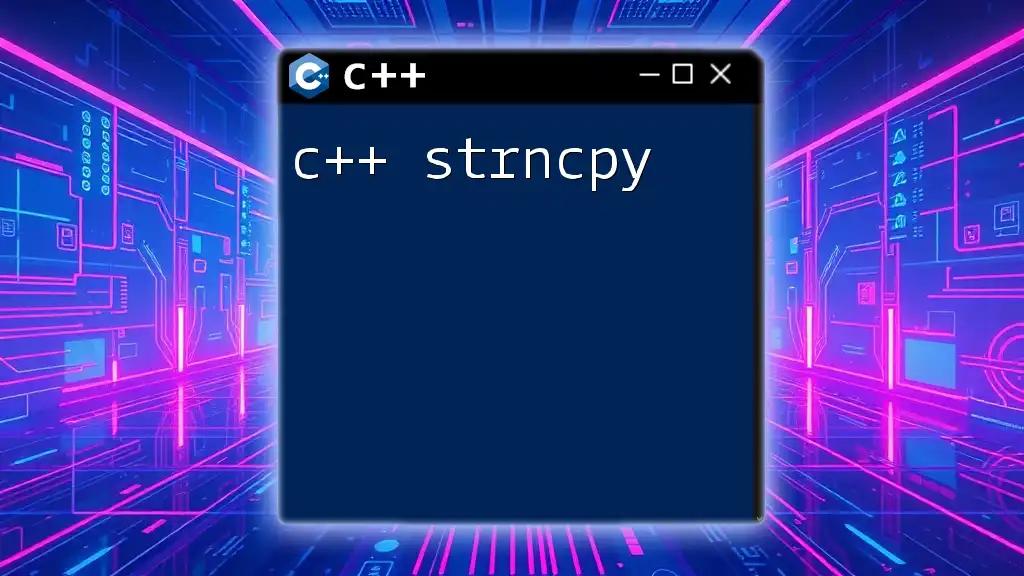
When to Use strcpy
Using `strcpy` is appropriate in scenarios where you are dealing with C-style strings (character arrays) instead of the more modern C++ string types. There are specific cases where `strcpy` shines:
- Performance: For low-level programming, especially in embedded systems or performance-critical applications, `strcpy` may offer a speed advantage over C++ `std::string`.
- Interfacing with Legacy Libraries: If your project interfaces with older C libraries, understanding and correctly using `strcpy` becomes essential.

How strcpy Works
Understanding the mechanics behind `strcpy` enhances your ability to use it effectively. When you call `strcpy`, it performs the following steps:
- It initializes a pointer to the beginning of the source string.
- It starts copying characters from the source to the destination one by one.
- The function continues copying until it reaches the null-terminator in the source string.
- After copying, it automatically appends a null character at the end of the destination string to signify its termination.
Importance of Null-Termination
This null-termination is critical because it indicates where the string ends. Without it, functions relying on string length may read beyond the intended memory allocation, potentially leading to undefined behavior or crashes.

Code Example of strcpy
Here’s a straightforward example demonstrating the use of `strcpy` in C++:
#include <iostream>
#include <cstring> // Required for strcpy
int main() {
const char* source = "Hello, World!";
char destination[50]; // Ensure destination has sufficient space
strcpy(destination, source);
std::cout << "Copied string: " << destination << std::endl;
return 0;
}
Explanation of the Code:
In this example:
- A constant character pointer `source` points to the string "Hello, World!".
- A character array `destination` is declared with a size of 50 to hold the copied string.
- The `strcpy` function is invoked to copy the string from `source` to `destination`.
- Finally, the copied string is printed to the console.
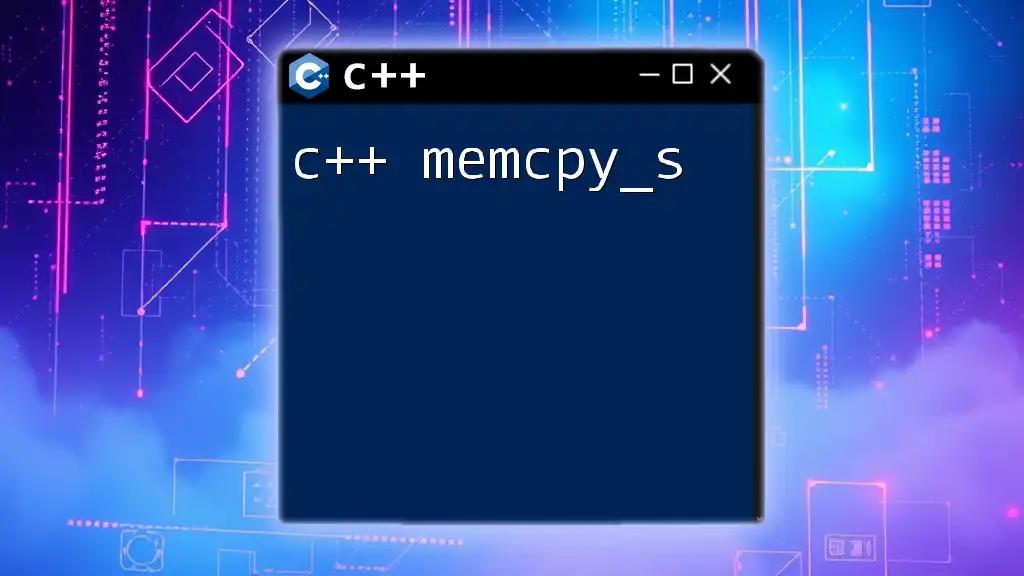
Potential Issues with strcpy
While `strcpy` is useful, it carries inherent risks, primarily related to buffer overflow. Buffer overflow occurs when the data being written to a buffer exceeds the size of that buffer, overwriting adjacent memory and potentially leading to security vulnerabilities.
Solutions and Best Practices
To guard against buffer overflow, consider the following approaches:
- Use strncpy: This safer version of `strcpy` allows you to specify the maximum number of characters to copy, thus protecting against overflow. Its syntax is:
char* strncpy(char* destination, const char* source, size_t num);
An example using `strncpy` would look like this:
#include <cstring>
char destination[50];
strncpy(destination, source, sizeof(destination) - 1);
destination[sizeof(destination) - 1] = '\0'; // Manually null-terminate
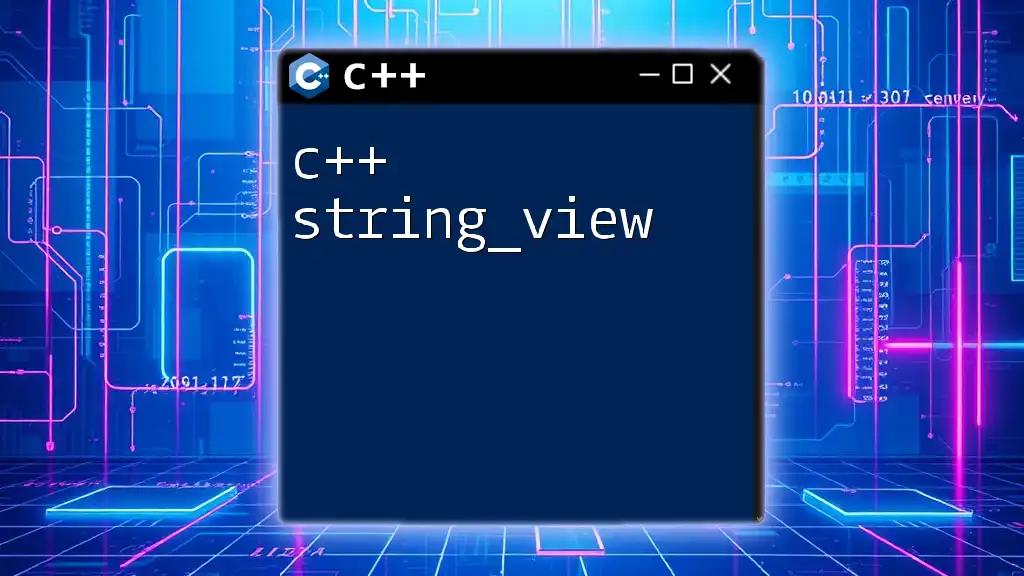
Best Practices When Using strcpy
When using `strcpy`, adhere to these best practices to ensure that your program remains robust and secure:
- Ensure Adequate Memory Allocation: Always allocate enough memory for the destination string to accommodate the source string, including the null terminator.
- Always Null-Terminate Strings: Consider implementing checks or using safer alternatives to ensure strings are properly null-terminated. This prevents rogue memory accesses.
Avoiding strcpy Entirely
In many modern C++ applications, it's advisable to favor the C++ Standard Library's string handling features, such as `std::string`. Using `std::string` eliminates many of the risks associated with manual memory management, providing higher-level constructs that automatically handle memory allocation and avoid common pitfalls.
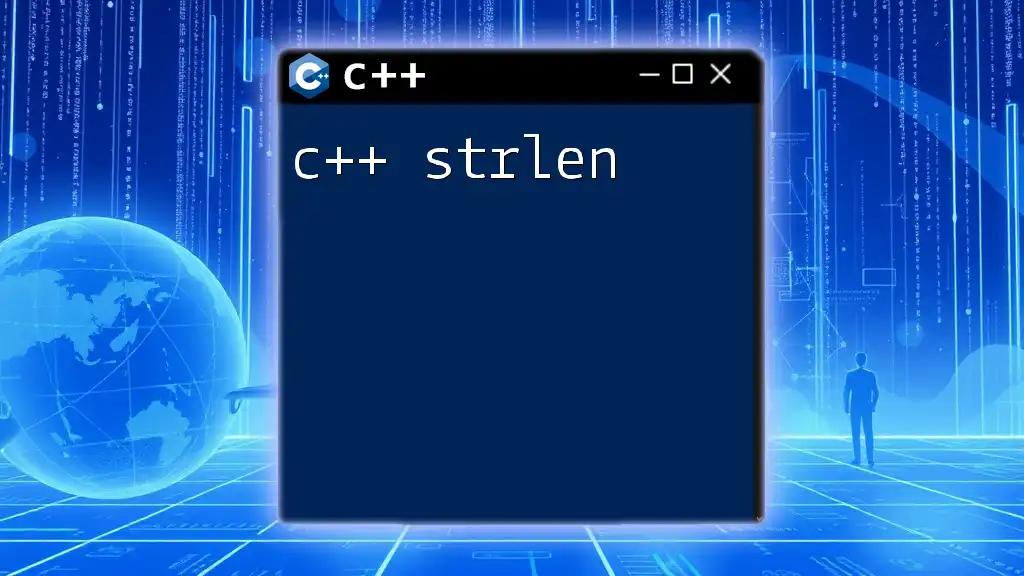
Conclusion
In summary, the `C++ strcpy` function is a powerful tool for string manipulation when working with C-style strings. Understanding its mechanics, potential risks, and safe usage guidelines is crucial for effective programming. As you delve deeper into C++, consider embracing safer alternatives, but never underestimate the importance of foundational knowledge in string handling.
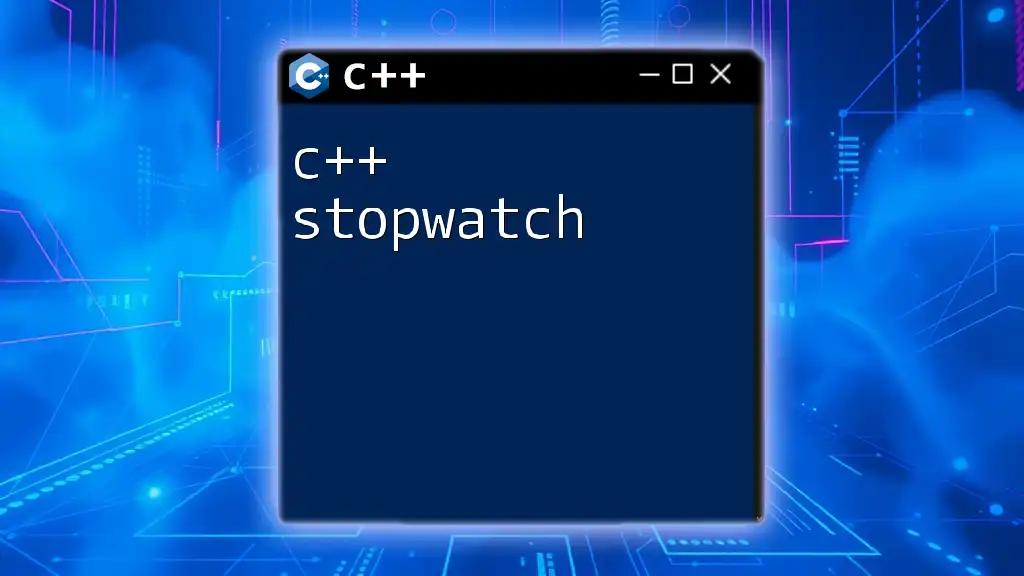
Additional Resources
To further enrich your understanding of string manipulation in C++, consider exploring the following:
- Links to official C++ documentation
- Recommended books and tutorials for more in-depth knowledge
- Online communities for C++ learners and professionals
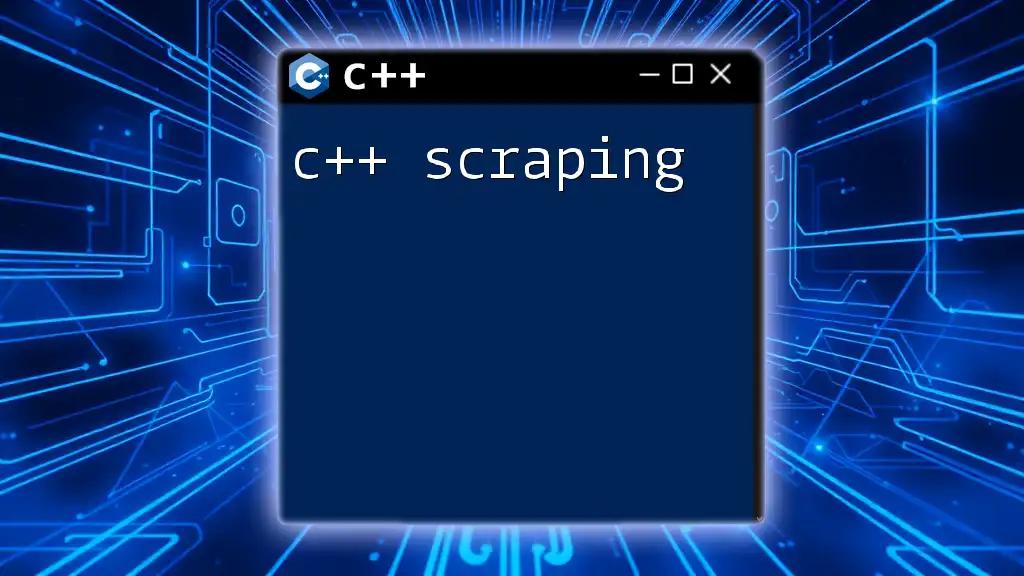
Frequently Asked Questions (FAQs)
What is the difference between strcpy and strncpy?
`strcpy` copies a string without controlling the length, whereas `strncpy` specifies the maximum number of characters to copy, adding a layer of safety.
Can I use strcpy with std::string?
While possible, it's generally not recommended. C++ strings automatically handle memory management, making `strcpy` unnecessary and potentially risky.
Are there any alternatives to strcpy?
Yes, consider using safer functions like `strncpy`, or leverage C++ `std::string` and its methods which eliminate concerns about manual memory management.