The `strncpy` function in C++ is used to copy a specified number of characters from one string to another, ensuring that the destination string is properly null-terminated if the source string is longer than the specified number.
Here's a code snippet example:
#include <iostream>
#include <cstring>
int main() {
const char* source = "Hello, World!";
char destination[20];
strncpy(destination, source, 5);
destination[5] = '\0'; // Ensure null-termination
std::cout << destination << std::endl; // Output: Hello
return 0;
}
Understanding `strncpy` in C++
What is `strncpy`?
`strncpy` is a standard library function in C++ that is used to copy a specified number of characters from one string (the source) to another string (the destination). The main purpose of `strncpy` is to prevent buffer overflow by allowing programmers to define the maximum number of characters to be copied. Unlike `strcpy`, which does not keep track of the number of characters copied and may result in overflow if the source string is larger than the destination buffer, `strncpy` imposes a size limit.
When to Use `strncpy`
You should consider using `strncpy` in scenarios where you are dealing with fixed-size buffers and need to ensure that you do not exceed those limits. This is particularly important in systems where overflows can lead to security vulnerabilities or unexpected behavior. While `strcpy` blindly copies until a null character is encountered, `strncpy` ensures that you only copy what's safe, making it a better choice for safety and security.

Syntax of `strncpy`
Breakdown of the Syntax
The syntax for `strncpy` is as follows:
char *strncpy(char *dest, const char *src, size_t n);
- `dest`: This is the pointer to the destination buffer where the string will be copied.
- `src`: This is the pointer to the source string that you want to copy.
- `n`: This is the maximum number of characters to copy from `src` to `dest`.

How to Use `strncpy` Effectively
Basic Example of `strncpy`
Here’s a simple example to illustrate how `strncpy` works:
#include <iostream>
#include <cstring>
int main() {
char src[] = "Hello, World!";
char dest[20];
strncpy(dest, src, sizeof(dest) - 1);
dest[sizeof(dest) - 1] = '\0'; // Null-terminate the string
std::cout << dest << std::endl; // Output: Hello, World!
return 0;
}
In this example, we define a source string `src` and a destination buffer `dest`. By using `strncpy`, we safely copy the content of `src` to `dest`. The expression `sizeof(dest) - 1` ensures that we leave space for the null terminator, which we manually add afterward to ensure the string is properly terminated.
Important Considerations When Using `strncpy`
Null-Termination
A key point to remember is that if the length of `src` is greater than or equal to `n`, the destination string will not be null-terminated. This can lead to unexpected behavior when using the destination string later on. Therefore, it is your responsibility as the programmer to ensure null termination after using `strncpy`.
Buffer Size Management
It’s crucial to manage buffer sizes correctly to prevent buffer overflow and ensure that the destination can comfortably hold the content being copied. Always allocate your destination buffer adequately, and check the size of the source string before copying.

Common Mistakes with `strncpy`
Forgetting to Null-Terminate
One common mistake developers make is forgetting to add a null terminator after the call to `strncpy`. For example:
#include <iostream>
#include <cstring>
int main() {
char src[] = "Hi!";
char dest[3];
strncpy(dest, src, sizeof(dest)); // No null termination
std::cout << dest << std::endl; // Undefined behavior!
return 0;
}
In this case, the output could be unpredictable since the destination does not have a null-terminated string, leading to potential junk values being printed or incomplete output.
Incorrect Buffer Sizes
Another prevalent issue is miscalculating the buffer size. For instance:
#include <iostream>
#include <cstring>
int main() {
char src[] = "A long string that won't fit.";
char dest[10];
strncpy(dest, src, sizeof(dest)); // Potential truncation
std::cout << dest << std::endl; // Output: A long str
return 0;
}
This code leads to truncation of the source string, and the programmer may inadvertently lose important data. Always verify that the destination buffer is large enough before copying.

Best Practices for Using `strncpy`
Always Null-Terminate
As reiterated, always null-terminate your strings when using `strncpy`. Whether you do it manually or wrap it in a custom function, you must ensure your destination string is safe to use afterward.
Use the Right Buffer Size
Take the time to calculate the appropriate buffer sizes for your destination strings. This will help you avoid truncation and overflow. A good rule of thumb is to make the destination buffer slightly larger than the source string as a precaution.
Use `strncpy` in Secure Coding
Using `strncpy` contributes to secure coding practices, especially in environments where memory safety is critical. While it’s not foolproof, it helps mitigate some of the risks associated with string manipulation. Still, consider modern C++ alternatives like `std::array` or `std::string`, which offer dynamic memory management and built-in safety features.
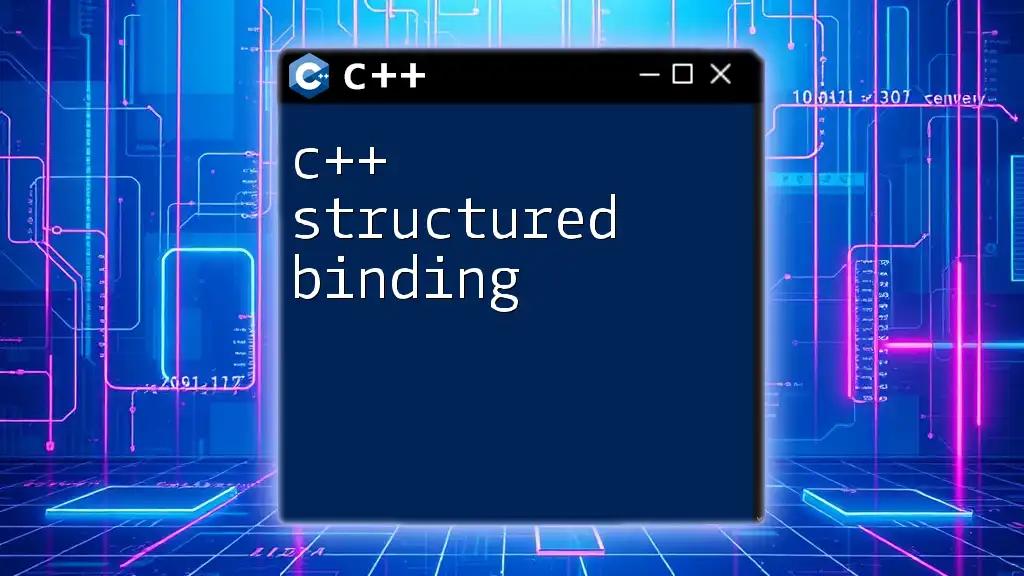
Advanced Use Cases for `strncpy`
Safely Handling User Input
Handling user input securely is a vital application for `strncpy`. Below is an example:
#include <iostream>
#include <cstring>
int main() {
char userInput[50];
char safeBuffer[20];
std::cout << "Enter your name: ";
std::cin.getline(userInput, 50);
strncpy(safeBuffer, userInput, sizeof(safeBuffer) - 1);
safeBuffer[sizeof(safeBuffer) - 1] = '\0'; // Null-terminate
std::cout << "Hello, " << safeBuffer << "!" << std::endl;
return 0;
}
In this case, `strncpy` is used to safely copy user input into a fixed-size buffer while ensuring that the input does not exceed the buffer size and is null-terminated.
Combining with Other String Functions
`strncpy` can be combined with other string manipulation functions to enhance usability and maintain control over memory management. For example, consider using `strcat` to append strings after ensuring safety with `strncpy` beforehand.

Conclusion
In summary, `strncpy` is a powerful function that plays an essential role in safe string manipulation in C++. However, developers must be diligent about managing null termination and buffer sizes. By following best practices and recognizing common pitfalls, you can leverage `strncpy` effectively in your C++ programming endeavors. Always stay informed about modern alternatives to ensure that your string handling remains secure and efficient.
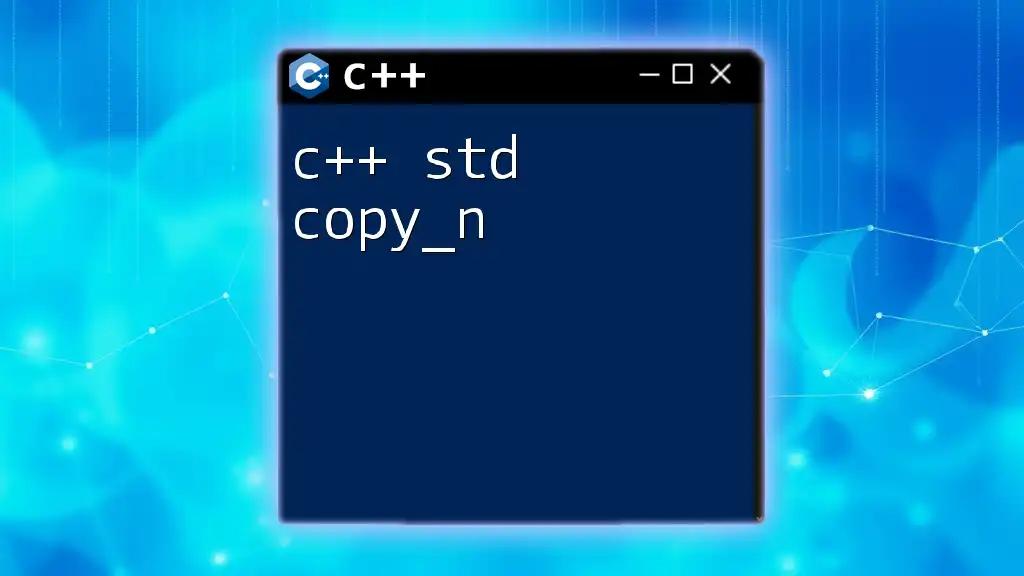
Additional Resources
For further reading, explore resources on string manipulation functions in C++. Look into recommended books or courses that provide deeper insights into effective string handling and memory management in C++.