The `std::copy_n` function in C++ efficiently copies a specified number of elements from one range to another, and here is a simple example demonstrating its usage:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5);
std::copy_n(source.begin(), 3, destination.begin());
// Output copied elements
for (int num : destination)
std::cout << num << " ";
return 0;
}
Understanding the Basics of std::copy_n
What is std::copy_n?
`std::copy_n` is a powerful function in the C++ Standard Library that allows you to copy a specified number of elements from one range to another. This function is especially useful when you only want to copy a subset of elements, rather than an entire sequence, enabling more efficient code by reducing unnecessary operations.
Syntax Overview
The syntax of `std::copy_n` is straightforward:
std::copy_n(InputIterator first, Size count, OutputIterator result);
In this syntax:
- `InputIterator first` is the beginning of the source range.
- `Size count` is the number of elements you want to copy.
- `OutputIterator result` is the beginning of the destination range where the elements will be copied.
Parameters of std::copy_n
- InputIterator first: This parameter specifies the start of the source range you want to copy from. It must be a valid iterator pointing to the first element to copy.
- Size count: This defines how many elements will be copied. Ensure that this number does not exceed the number of available elements in the source range.
- OutputIterator result: This is the destination where the copied elements will be stored. This iterator must point to a location that can accommodate at least `count` elements.
Return Value
`std::copy_n` returns an iterator pointing to the element immediately following the last element copied to the destination. This allows for easy chaining of operations if needed.

How std::copy_n Works
Under the Hood: Iterators and Copy Mechanics
Understanding how iterators function is crucial for grasping `std::copy_n`. An iterator acts like a pointer, allowing you to navigate through containers (such as arrays or vectors) without needing to know the specifics of the underlying data structure. When `std::copy_n` is called, it reads from the input iterator `first`, advances that iterator to copy elements according to the `count`, and writes to the output iterator `result`.
Example of std::copy_n in Action
To illustrate how `std::copy_n` works, consider the following example:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5); // Prepare the destination with enough space
std::copy_n(source.begin(), 3, destination.begin()); // Copy 3 elements
std::cout << "Copied elements: ";
for (int i : destination) {
std::cout << i << " "; // Output will be: 1 2 3 0 0
}
return 0;
}
In this example, we define a source vector containing five integers and a destination vector of the same size. By calling `std::copy_n`, we specify that we only want to copy the first three elements from the `source` to the `destination`. The expected output, as shown, confirms that only the first three integers have been copied, leaving the remaining positions in the destination vector unchanged (initially zero).

Use Cases for std::copy_n
Common Scenarios for Using std::copy_n
`std::copy_n` is particularly advantageous when you need to copy a specific number of elements from a source range to a destination. You might use it when dealing with:
- Fixed-length sequences: For instance, copying a specific number of entries from sensor data readings.
- Partial processing: When only a subset of input data is necessary for further analysis or computation.
Performance Considerations
Using `std::copy_n` is generally efficient since it minimizes overhead by copying exactly the required number of elements without iterating through unnecessary data. This can lead to improved performance over alternatives that copy entire sequences.
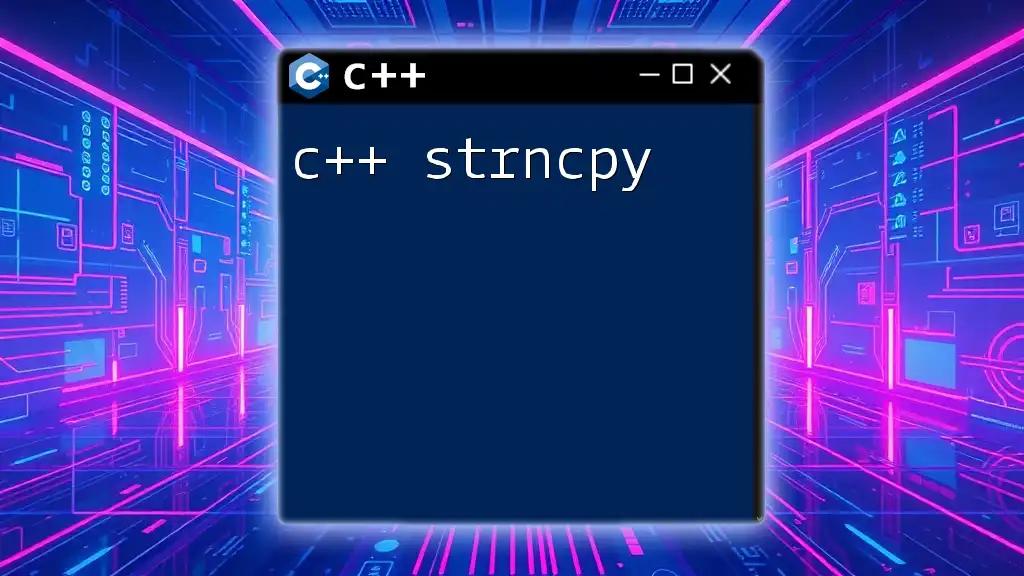
Alternatives to std::copy_n
Overview of Other Copy Functions
While `std::copy_n` is a versatile tool, there are other functions in the Standard Library that might be better suited for different scenarios:
- `std::copy`: Use this when you need to copy all elements from a source sequence. Unlike `std::copy_n`, which allows you to specify a count, `std::copy` will copy until the end of the range.
- `std::memcpy`: Ideal for efficient copying of raw memory. It is generally faster than STL algorithms but should be used with caution as it does not handle type safety.
- `std::copy_if`: When you need to copy elements based on certain criteria. This function allows conditional copying, providing more flexibility at the potential cost of performance.
Comparison Table
Here's a quick reference that demonstrates how `std::copy_n` compares to its alternatives:
Function | Use Case | Complexity |
---|---|---|
`std::copy` | Copying entire sequence | O(n) |
`std::copy_n` | Copying a specified number | O(n) |
`std::memcpy` | Copying raw memory efficiently | O(n) |
`std::copy_if` | Conditional copying | O(n) |

Best Practices for Using std::copy_n
Handling Edge Cases
When using `std::copy_n`, you must be vigilant about edge cases. For instance, if the count exceeds the total number of elements in the source range, it may lead to undefined behavior. Therefore, always validate your counts relative to the container sizes before calling this function.
Using std::copy_n with Different Data Types
One of the key strengths of `std::copy_n` is its ability to work with various data types, whether basic types like `int` or complex user-defined types. For example, consider copying objects of a custom struct:
#include <iostream>
#include <algorithm>
#include <vector>
struct Point {
int x, y;
};
int main() {
std::vector<Point> source = {{1, 2}, {3, 4}, {5, 6}};
std::vector<Point> destination(3); // Prepare for 3 copies
std::copy_n(source.begin(), 2, destination.begin()); // Copy 2 elements
std::cout << "Copied Points: ";
for (const auto& p : destination) {
std::cout << "(" << p.x << ", " << p.y << ") "; // Output: (1, 2) (3, 4) (0, 0)
}
return 0;
}
This code snippet demonstrates the copying of a custom type `Point`. The first two `Point` objects are successfully copied, leaving the rest of the destination initialized to default values.
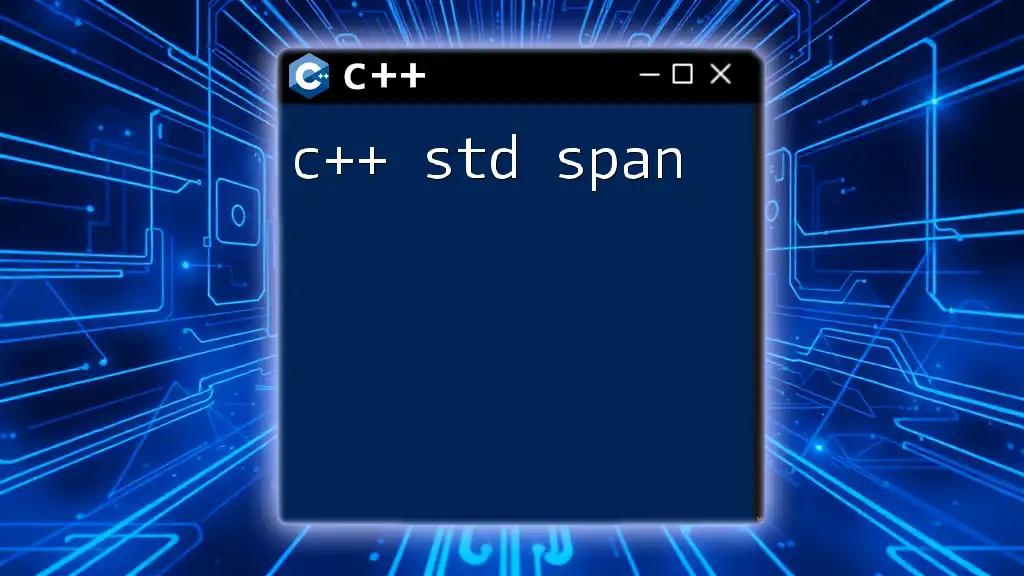
Conclusion
In summary, `std::copy_n` stands as a robust tool in the C++ programmer's toolkit, particularly for situations demanding precision and efficiency in copying specific numbers of elements. Understanding how to leverage this function allows for cleaner, more maintainable code and helps you utilize the full power of the C++ Standard Library.
As you continue your journey with C++, practicing the implementation of `std::copy_n` and experimenting with its alternatives will enhance your coding capabilities. Remember to consider the context of your operations to select the best function for your needs.
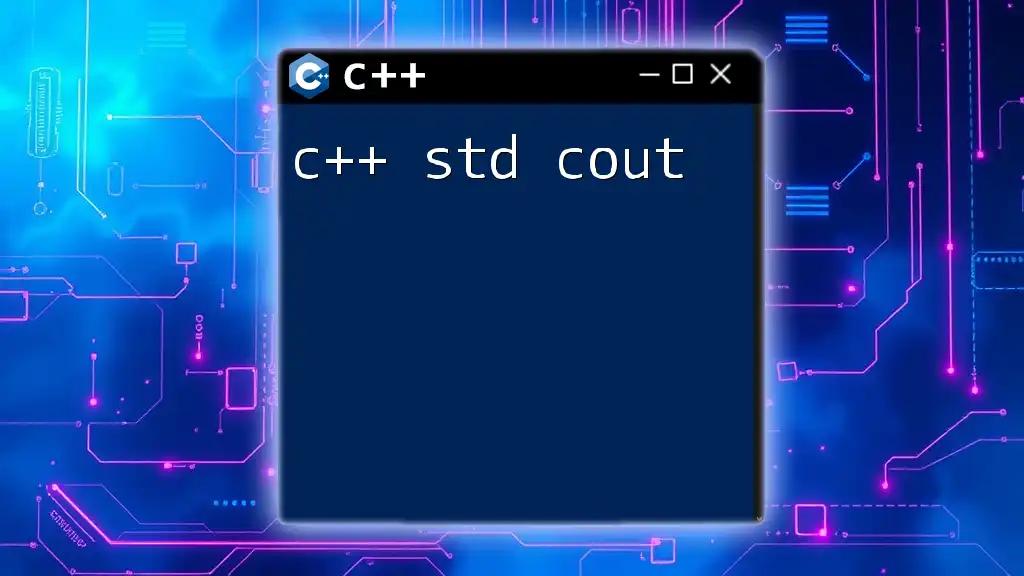
Further Resources
For more in-depth understanding and additional examples, consider exploring the official C++ documentation or engaging with community tutorials that delve into the nuances of C++ STL algorithms. Happy coding!