The `std::cout` is an object in C++ used for outputting data to the standard output stream (usually the console).
Here’s an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding std::cout
What is std::cout?
`std::cout` is the standard output stream in C++. It’s part of the C++ Standard Library, specifically included in the `<iostream>` header, and is primarily used to display output to the console. It helps programmers communicate results, debug outputs, and display information in real-time while the program is running.
The Basics of Output Streams
An output stream is a flow of data from a program to a destination, typically the console or a file. In C++, there are several output streams, but `std::cout` is the most commonly used for console output. It provides a simple interface for displaying various data types through the insertion operator (`<<`).
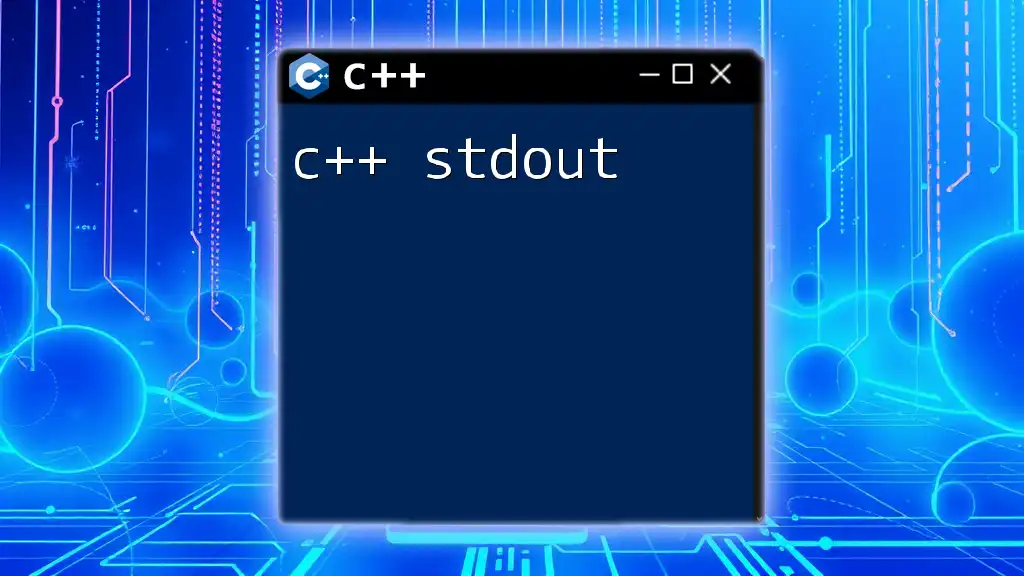
How to Use std::cout
Including the Necessary Header
To use `std::cout`, the `<iostream>` header file must be included at the beginning of your C++ program. This line is essential for accessing the features provided by the input and output stream library:
#include <iostream>
Basic Syntax of std::cout
The basic syntax of `std::cout` involves the insertion operator (`<<`), which takes the value you want to output and sends it to the standard output stream:
std::cout << "Your message here";
Each statement ends with a semicolon (`;`), which is standard in C++ syntax.
Outputting Basic Data Types
Integer Output
Displaying an integer can be done easily with `std::cout`. For example:
int number = 42;
std::cout << number << std::endl;
In this example, `number` is declared and initialized, and `std::cout` outputs the value, followed by an `std::endl`, which flushes the output buffer and adds a newline.
Floating-Point Output
Similarly, you can output floating-point numbers. For instance:
double pi = 3.14;
std::cout << pi << std::endl;
The `std::endl` allows for a cleaner output with automatic line breaks.
String Output
Outputting strings works seamlessly, whether using C-strings or `std::string`. Here’s an example with `std::string`:
std::string message = "Hello, World!";
std::cout << message << std::endl;
Here, `message` displays the text, followed by a newline.
Combining Multiple Outputs
Chaining Outputs
`std::cout` allows you to chain multiple outputs into a single statement:
std::cout << "The answer is: " << number << " and pi is approximately " << pi << std::endl;
This chaining feature can make the code more concise and easier to read. Remember that the insertion operator can handle concatenation of different types seamlessly.
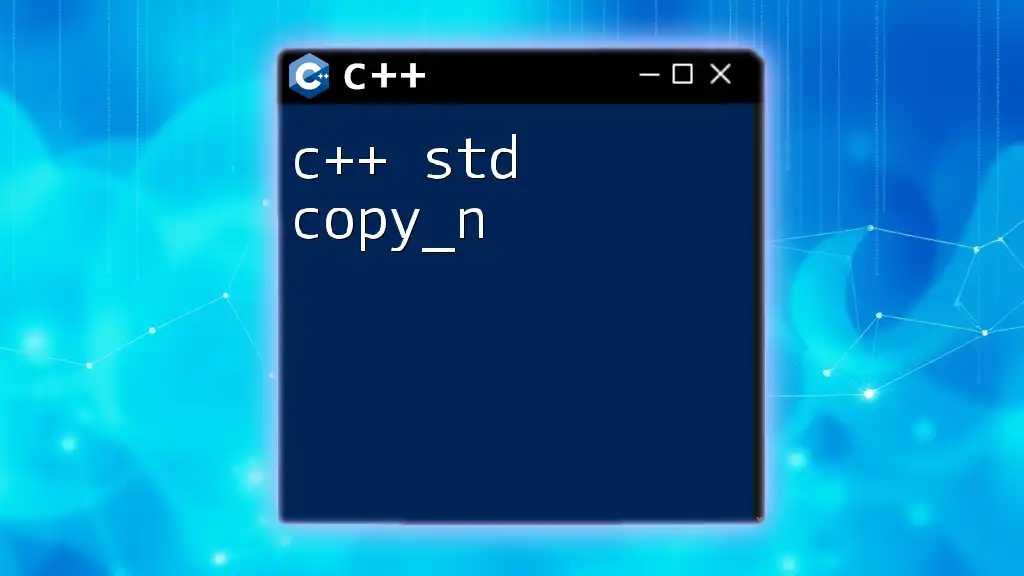
Formatting Output
Manipulating Output Precision
To control the precision of floating-point output, you can use `std::setprecision` from the `<iomanip>` header:
#include <iomanip>
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
In this code, `std::fixed` ensures that the number prints in fixed-point notation, while `std::setprecision(2)` limits it to two decimal places.
Using manipulators for text formatting
Setting Width
You can also manipulate the width of the output:
std::cout << std::setw(10) << number << std::endl;
In this case, `std::setw(10)` sets a width of 10 characters for the output. If the number is shorter, it will be right-aligned with spaces filling the extra space.
Filling Characters
To customize the filling character, you can combine `std::setfill` with `std::setw`:
std::cout << std::setfill('*') << std::setw(10) << number << std::endl;
Here, the output will be padded with asterisks instead of spaces, providing a visually distinctive format.
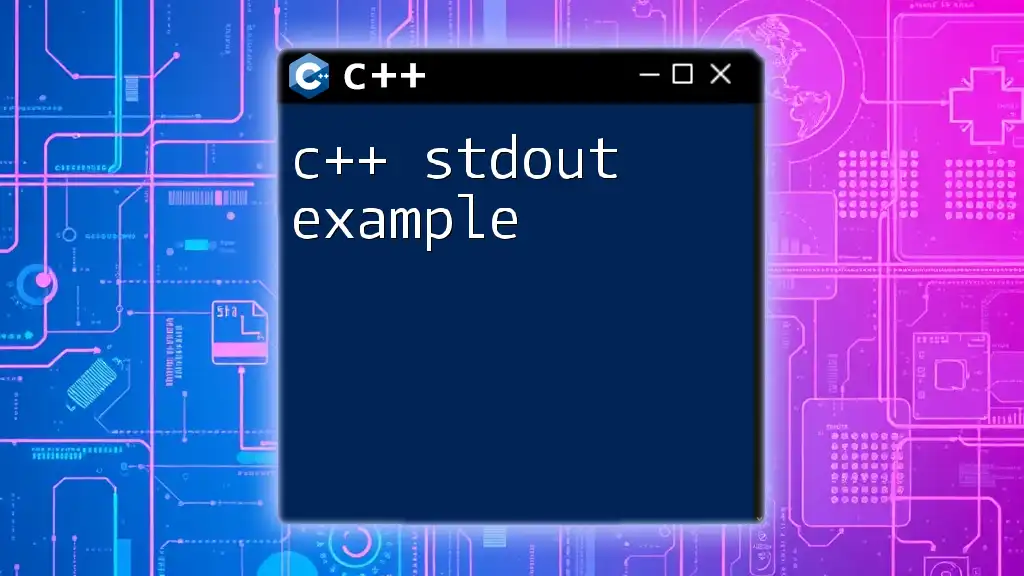
Error Handling with std::cout
Checking if Output is Successful
`std::cout` reliably outputs data, but errors can occur (e.g., if the output stream is not connected). It’s a good practice to check if your output has been successful by using the `std::cout.fail()` function:
if (std::cout.fail()) {
std::cerr << "Output failed!" << std::endl;
}
This ensures that your program is resilient and can handle unexpected circumstances.
Using std::cerr for Error Messaging
While `std::cout` is used for standard output, `std::cerr` is designed for error messages. It does not buffer the output, meaning messages appear immediately, which is crucial for debugging:
std::cerr << "An error occurred!" << std::endl;
This immediate output contrasts with `std::cout`, where output may be buffered and appear later.
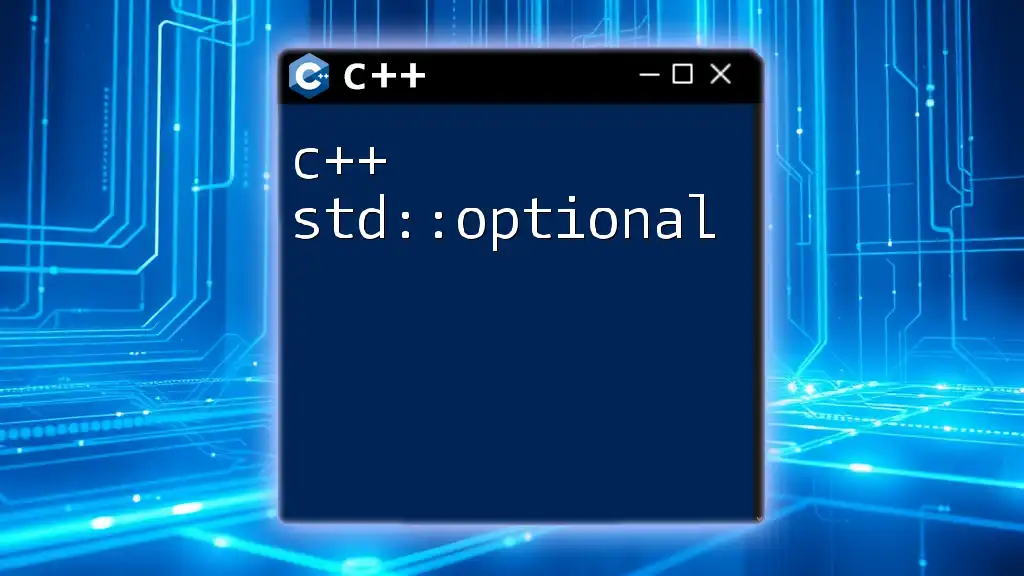
Best Practices for Using std::cout
Keeping Output Clear and Concise
When using `std::cout`, strive for clarity. Clear and concise messages enhance usability, especially in larger applications. Use descriptive messages and organize outputs logically.
Performance Considerations
Be mindful that excessive use of `std::cout` can impact program performance, particularly in loops or repetitive tasks. Aim to minimize console outputs in performance-critical sections of your code to avoid latency.
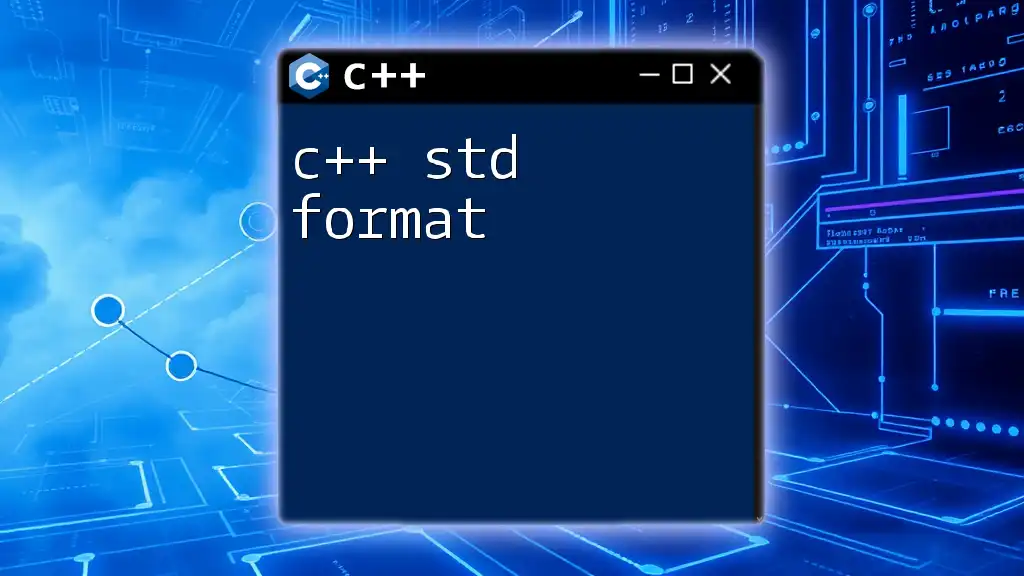
Conclusion
In this comprehensive guide on C++ `std::cout`, we explored its functionality, how to format output, errors handling, and best practices for effective usage. Mastering `std::cout` will significantly enhance your coding productivity and your capability to present data clearly.
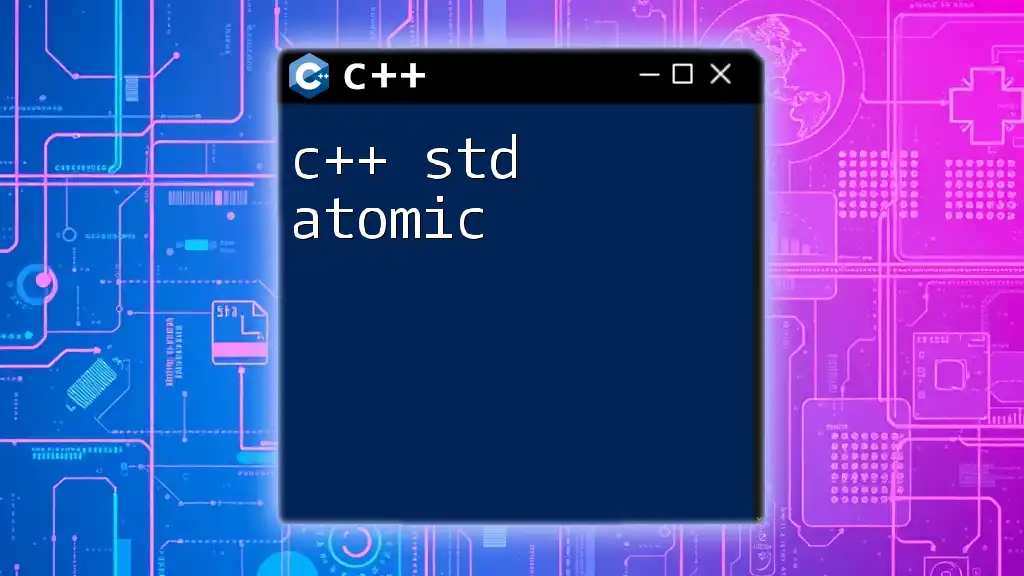
Additional Resources
To deepen your understanding of `std::cout` and other C++ features, consider exploring recommended books, online courses, and the official C++ documentation. Engaging with the community through forums can also provide valuable insights and shared experiences.
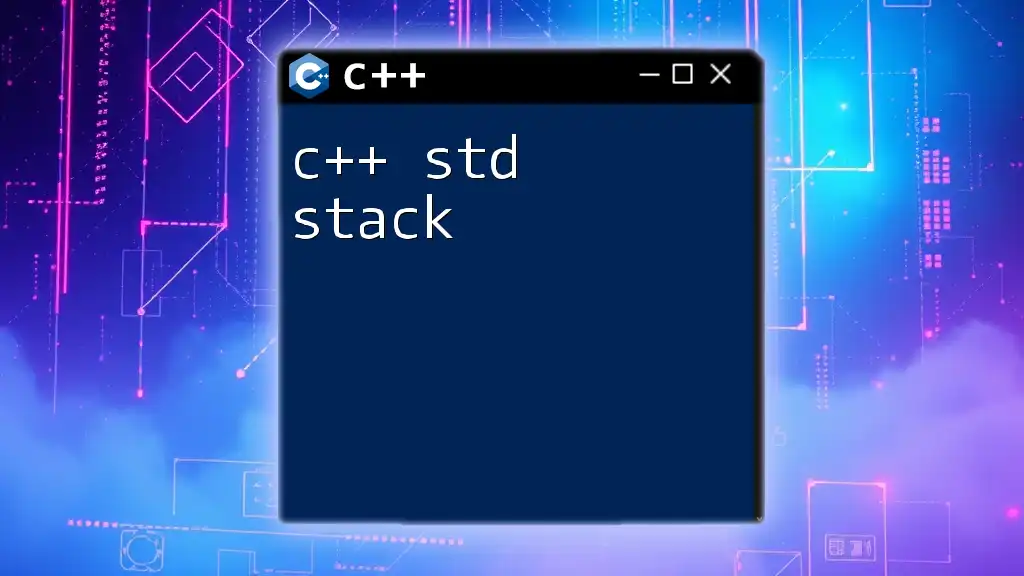
Call to Action
If you have questions or comments about `std::cout`, feel free to leave a message! Subscribe to our blog for more focused resources and tutorials on C++ programming. Happy coding!