The `strcat` function in C++ is used to concatenate two C-style strings by appending the source string to the destination string.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
int main() {
char destination[20] = "Hello, ";
char source[] = "world!";
strcat(destination, source);
std::cout << destination << std::endl; // Output: Hello, world!
return 0;
}
Understanding `strcat`
What is `strcat`?
`strcat` is a standard library function in C++ that allows developers to concatenate two C-style strings. This function is part of the `<cstring>` header file and is essential for string manipulation in applications that require direct handling of character arrays.
The main purpose of `strcat` is to append the `src` string to the end of the `dest` string, effectively combining the contents of both strings into the destination buffer. This functionality is crucial in scenarios where strings need to be constructed dynamically or where performance optimizations are essential, such as in embedded systems.
Overview of String Handling in C++
C++ offers two primary ways to manage strings: C-style strings, which are arrays of characters terminated by a null character (`'\0'`), and C++ strings, which are objects of the `std::string` class. While C-style strings allow for a low-level manipulation of memory, they can lead to complex problems like buffer overflows and memory leaks.
In this context, `strcat` is particularly useful when working with C-style strings. Understanding the differences between these two approaches is crucial for effectively using `strcat` in C++ programming.
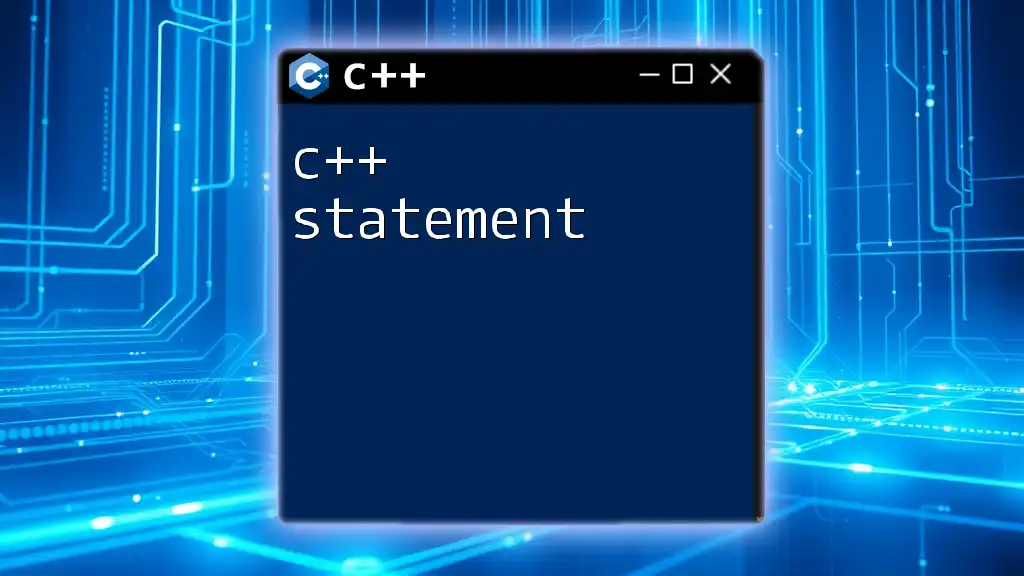
Syntax and Parameters of `strcat`
Syntax of `strcat`
The `strcat` function follows a simple syntax structure:
char* strcat(char* dest, const char* src);
In this prototype:
- The `dest` parameter is the destination string to which the source string (`src`) will be appended.
- It returns a pointer to the destination string, enabling developers to use it in a fluent manner.
Parameters Explained
- `dest`: This is the destination string that must have enough space allocated to hold the combined length of both strings. It must be initialized properly and should include enough buffer size to accommodate the resulting concatenated string.
- `src`: This is the source string that you want to add to the `dest`. It does not need to be pre-allocated like `dest`; however, it must be a valid null-terminated string.
Proper initialization of these strings is crucial; failure to do so can lead to undefined behavior and potential crashes.
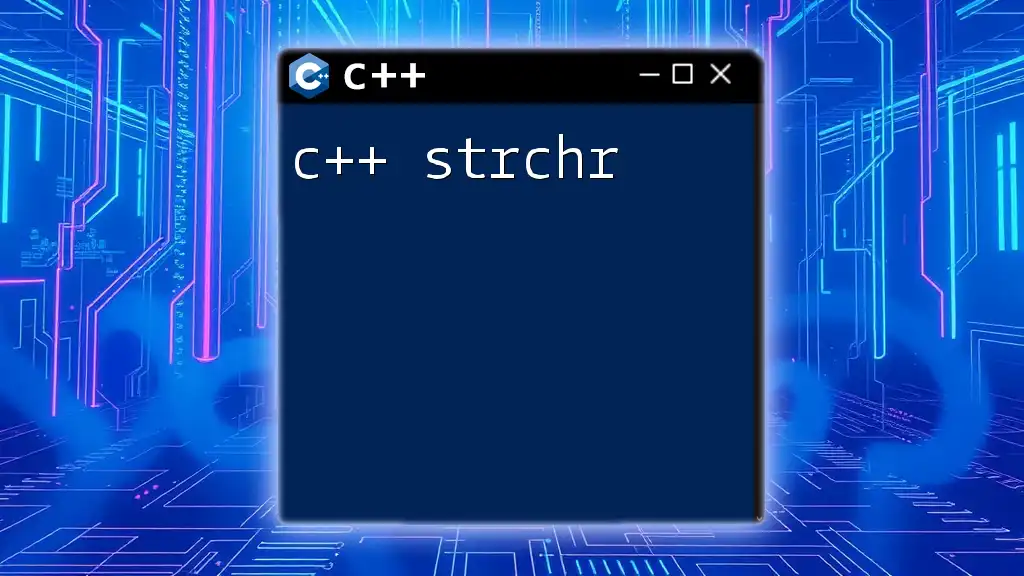
How `strcat` Works
Memory Management with `strcat`
Memory management is a key consideration when using `strcat`. When concatenating two strings, it’s essential to ensure that the destination string has enough memory to hold both the original and the new content. Mismanagement can lead to buffer overflows, leading to security vulnerabilities and application crashes.
For example, if you attempt to append a string to a buffer that isn’t large enough, data may overflow into adjacent memory, causing unpredictable behavior. Always calculate the required size for `dest` as:
size of dest >= size of original dest + size of src + 1 (for null terminator)
Example of Concatenation
Here’s a basic example demonstrating how to use `strcat`:
#include <iostream>
#include <cstring>
int main() {
char dest[20] = "Hello, ";
char src[] = "World!";
strcat(dest, src);
std::cout << dest << std::endl; // Output: Hello, World!
return 0;
}
In this example:
- We declare a `dest` character array with enough space.
- The `src` string is appended using `strcat`.
- The result, `Hello, World!`, is printed to the console.
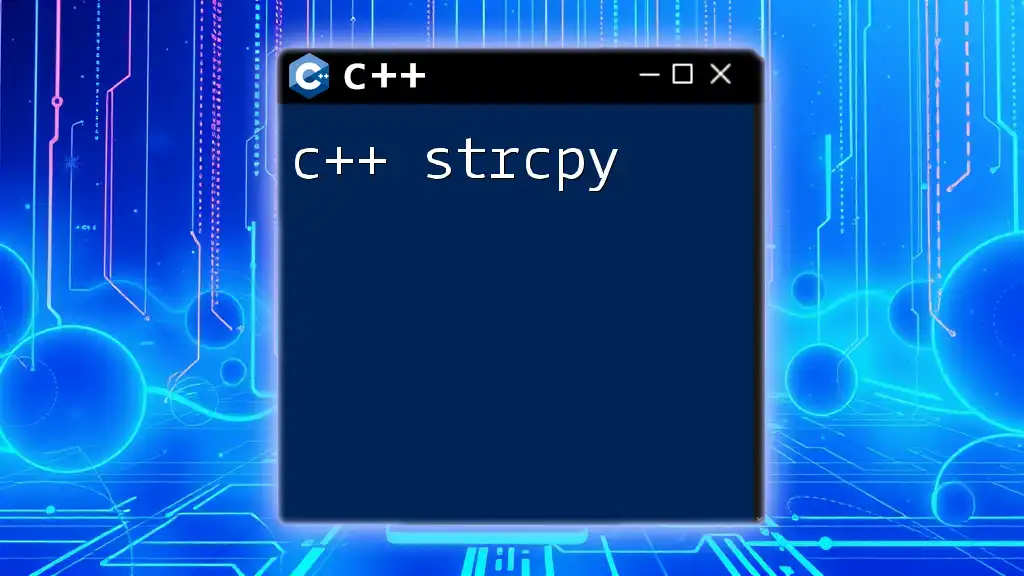
Best Practices for Using `strcat`
Safety Tips When Using `strcat`
To avoid common pitfalls associated with `strcat`, consider the following best practices:
- Always ensure that the `dest` array has sufficient space to hold the combined strings.
- Consider using `strncat`, which allows you to specify the maximum number of characters to append, providing an extra layer of safety against buffer overflow.
When to Use `strcat`
`strcat` is ideal for legacy C code, scenarios requiring precise memory management, and performance-critical applications where every byte counts. However, it is less suitable for modern C++ applications, which benefit from the automatic memory management provided by `std::string`.
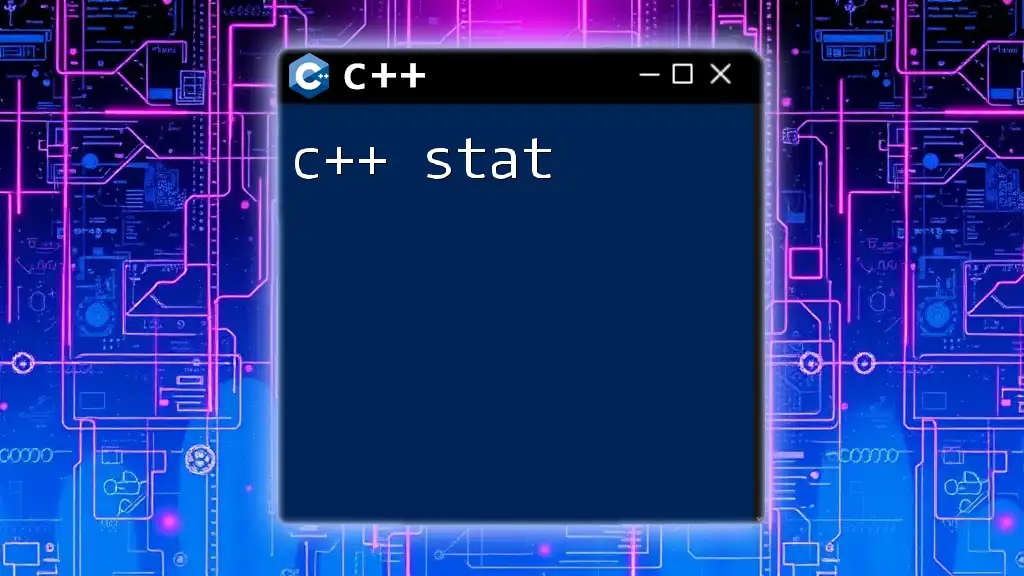
Common Mistakes with `strcat`
Initializing Strings Incorrectly
Improper initialization of strings can lead to catastrophic failures. For example:
char dest[10]; // Not initialized
char src[] = "TooLongString";
strcat(dest, src); // Undefined behavior
In this scenario, attempting to concatenate without initializing `dest` leads to undefined behavior, as `dest` might contain garbage values or lead to memory access violations.
Forgetting Null Terminators
One critical aspect of C-style strings is the null terminator (`'\0'`). Omitting this can cause various issues:
char dest[20] = "Hi";
char src[] = "There";
strcat(dest, src); // Works because of the null terminator
Here, the `dest` string is initialized correctly. However, if you forget to ensure `dest` is null-terminated before calling `strcat`, the result could be unpredictable.
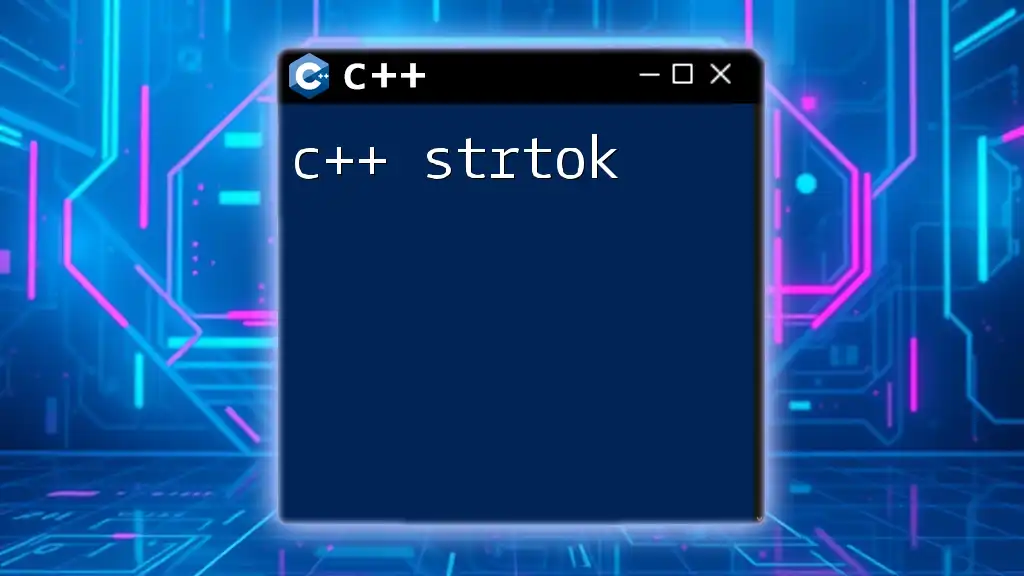
Alternatives to `strcat` in C++
Using `std::string`
In modern C++, employing `std::string` is often preferable to using `strcat`. The `std::string` class provides a more robust and safer method for string manipulation:
#include <string>
#include <iostream>
int main() {
std::string s1 = "Hello, ";
std::string s2 = "World!";
std::string result = s1 + s2;
std::cout << result << std::endl; // Output: Hello, World!
return 0;
}
This approach automatically manages memory and eliminates concerns about buffer overflows and null terminators.
Other C-style Alternatives
Another option is `strncat`, which offers a safer alternative to `strcat`. It allows you to control how many bytes are appended:
#include <iostream>
#include <cstring>
int main() {
char dest[20] = "Hello, ";
char src[] = "World!";
strncat(dest, src, 3); // Appending only 'Wor'
std::cout << dest << std::endl; // Output: Hello, Wor
return 0;
}
In this code segment, only the first three characters of `src` are appended to `dest`, preventing overflow while still merging strings.
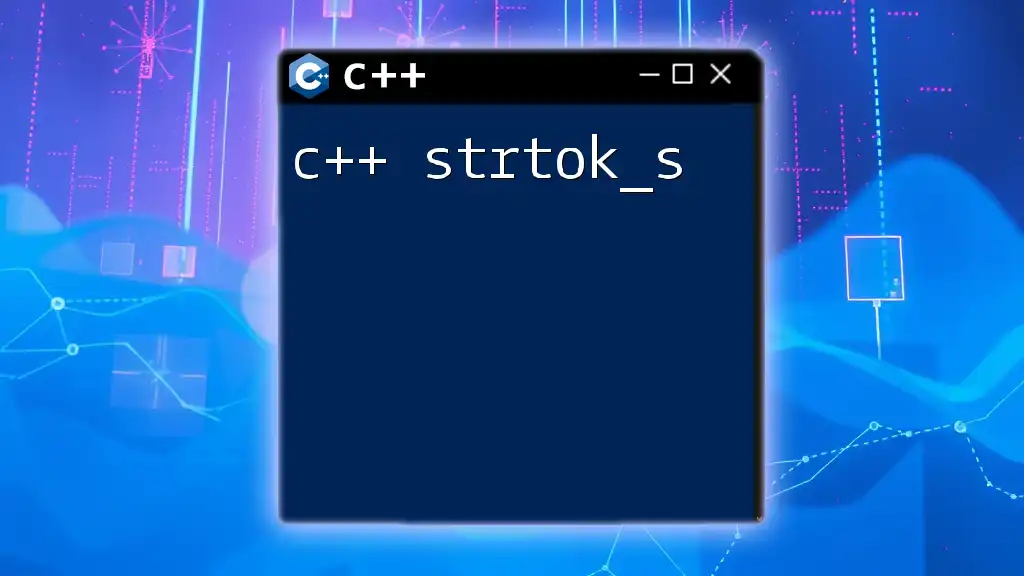
Conclusion
Summary of Key Points
The `strcat` function is a powerful tool in C++ for concatenating C-style strings. However, its usage requires a clear understanding of memory management and safety practices. By following best practices, such as ensuring proper buffer sizes and considering alternatives, developers can effectively use `strcat` in their applications.
Final Thoughts
When working with string manipulation in C++, prioritizing safe practices ensures robust and reliable software development. There are many resources available for further learning about string handling in both C and C++. Embracing modern alternatives like `std::string` will lead to cleaner, safer, and more maintainable code.
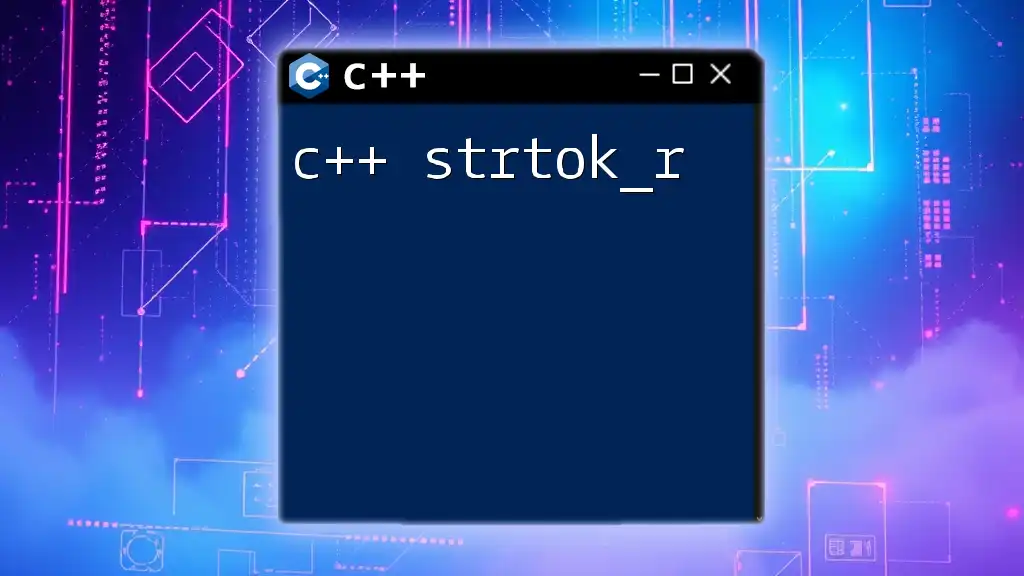
Additional Resources
Further Reading
For deeper insights into string manipulation in C++, consider reviewing the official documentation and exploring resources tailored to both beginners and experienced developers alike.
Community and Support
Engaging with online forums and communities dedicated to C++ can provide invaluable support and information for resolving string-related issues or enhancing your understanding of string manipulation in the language.