C++ structured bindings allow you to unpack multiple values from a tuple, pair, or array into separate variables in a concise and readable way.
#include <tuple>
#include <iostream>
int main() {
std::tuple<int, double, std::string> myTuple(1, 3.14, "Hello");
auto [a, b, c] = myTuple; // structured binding
std::cout << a << ", " << b << ", " << c << std::endl;
return 0;
}
What is Structured Binding in C++?
C++ structured binding is a modern feature introduced in C++17 that allows for unpacking the elements of various types, including tuples, pairs, and user-defined types, into separate named variables. This feature significantly simplifies code by reducing the verbosity of declarations and making it more readable. Rather than creating multiple variables line by line, structured bindings empower programmers to extract multiple values from a single expression in a single declaration.
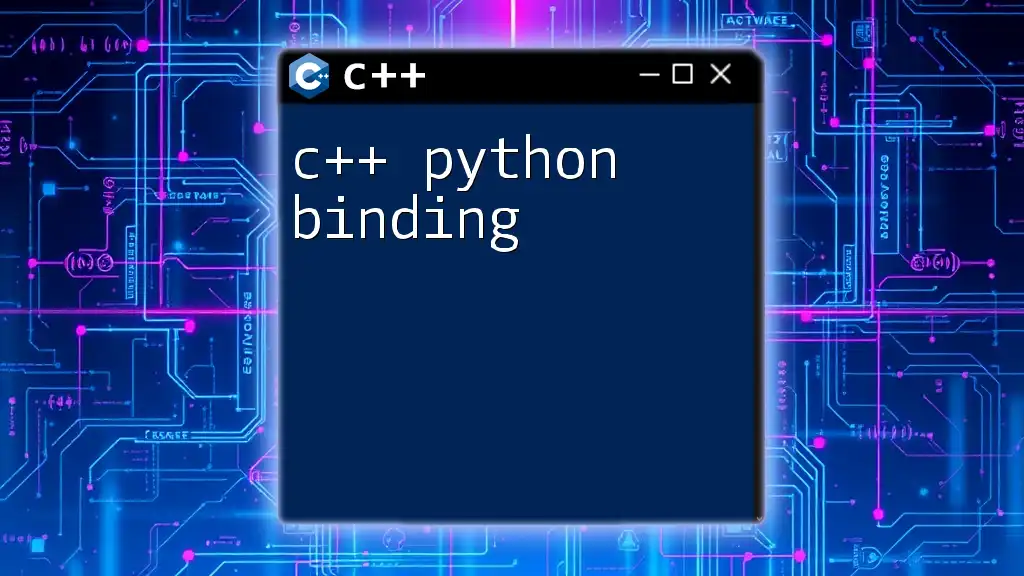
Historical Context
C++ has continually evolved, adding features that streamline programming practices. Prior to C++17, unpacking multiple values typically required the use of `std::tie`, `std::pair`, or `std::tuple`, often leading to verbose and less clear code. The introduction of structured binding represents a significant step towards simplifying this process, allowing developers to write cleaner and more maintainable code.
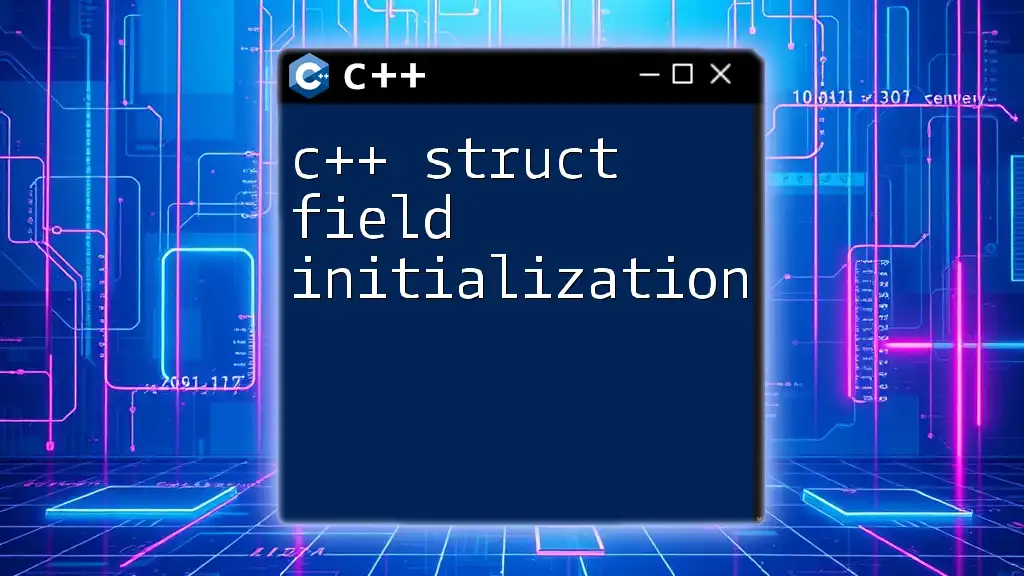
Understanding the Basics of C++ Structured Binding
How Structured Binding Works
The basic syntax of structured binding is simple yet powerful. It utilizes the `auto` keyword followed by an identifier list in square brackets, which captures the values being unpacked from an expression. For example:
auto [var1, var2] = expression;
In this syntax:
- `auto`: Automatically deduces the types of the variables being declared.
- `[var1, var2]`: Represents the individual components being unpacked from the expression.
Benefits of Using Structured Binding in C++
Structured binding provides several advantages that enhance the C++ programming experience:
- Increased Code Readability: By allowing multiple variables to be declared in a single statement, structured binding improves the visual clarity of code.
- Improved Ease of Maintenance: Code written with structured bindings is often easier to read and understand, which is essential for long-term maintenance.
- Reduced Boilerplate Code: Structured bindings eliminate repetitive code, making it less prone to errors.
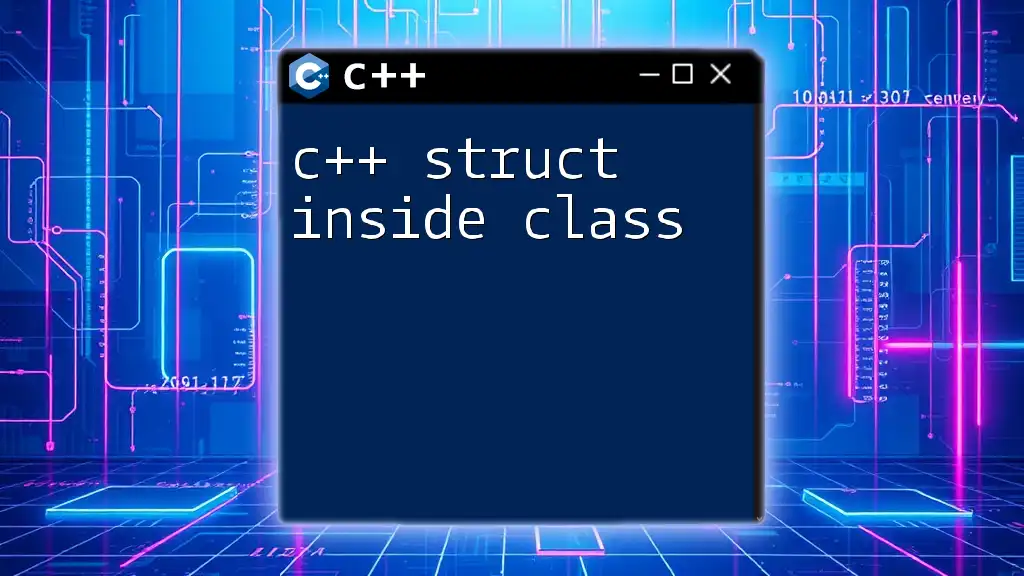
Syntax and Usage of C++ Structure Binding
Basic Syntax of Structured Binding
The core syntax of structured binding can be seen in its use with various data structures. Here’s how to unpack values from a tuple and pair:
Using `std::tuple`:
std::tuple<int, double> myTuple(10, 20.5);
auto [first, second] = myTuple;
In this example, `first` will hold the value `10`, and `second` will have the value `20.5`.
Using `std::pair`:
std::pair<int, int> myPair(1, 2);
auto [x, y] = myPair;
Similarly, `x` and `y` will unpack to `1` and `2` respectively.
Types of Variables in Structured Binding
Structured binding can also be utilized with arrays, as shown here:
int arr[2] = {4, 5};
auto [a, b] = arr;
In this case, `a` will be `4`, and `b` will be `5`.
Structured Bindings with User-defined Types
Structured binding is not limited to standard library types; it can also be applied to user-defined types like structs and classes. For instance:
struct Point { int x; int y; };
Point p{10, 20};
auto [xPos, yPos] = p;
In this example, `xPos` will hold `10`, and `yPos` will hold `20`, simplifying the unpacking process for data members of the struct.
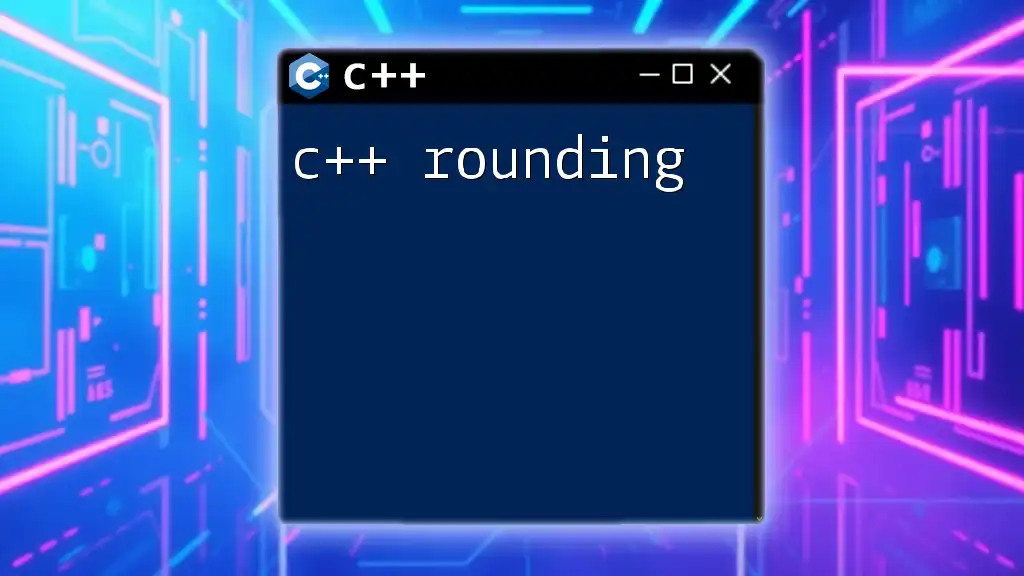
Advanced Concepts in Structured Binding C++
Lifetime Issues and Reference Binding
One of the nuanced features of structured binding is reference binding. When you want to capture references to the original values, you can use a `&` after the `auto`. Here’s an example:
std::vector<int> vec = {10, 20, 30};
for (auto& [index, value] : enumerate(vec)) {
// Modify vector elements
value += 5; // Modifies the vector directly
}
This example shows that when you use a reference in the structured binding, modifications to `value` directly impact the original vector, allowing for efficient manipulation. Care must be taken when working with references, particularly regarding the lifetime of the objects involved to avoid dangling references.
Structured Binding with Arrays and Pointers
Structured binding can also apply to pointers. Consider an example that involves unpacking pointers:
int* ptr = new int[2]{1, 2};
auto [firstElem, secondElem] = *ptr; // Unpacking dereferenced pointer
However, be mindful of memory management when dealing with dynamically allocated memory; always remember to `delete[]` the allocated array after use to avoid memory leaks.
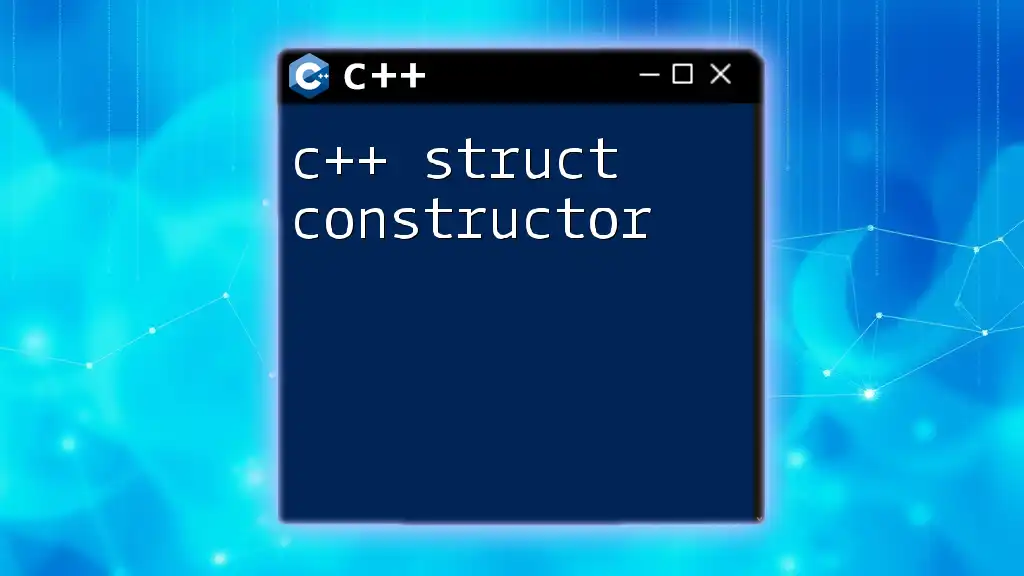
Common Use Cases for C++ Structured Binding
Utilizing Structured Binding in Functions
Structured binding shines when returning multiple values from functions. This can be done conveniently with `std::pair` or `std::tuple`:
std::pair<int, double> func() { return {1, 2.5}; }
auto [i, d] = func();
This code effectively unpacks the returned pair into two separate variables, `i` and `d`, without needing verbose syntax.
Structured Binding in Range-based Loops
Structured binding enhances clarity within loops, especially when iterating over associative containers like maps:
std::map<std::string, int> myMap = {{"apple", 5}, {"banana", 10}};
for (auto [key, value] : myMap) {
std::cout << key << ": " << value << std::endl;
}
In this loop, structured binding allows for the clear and concise extraction of key-value pairs, improving the overall readability of the code.
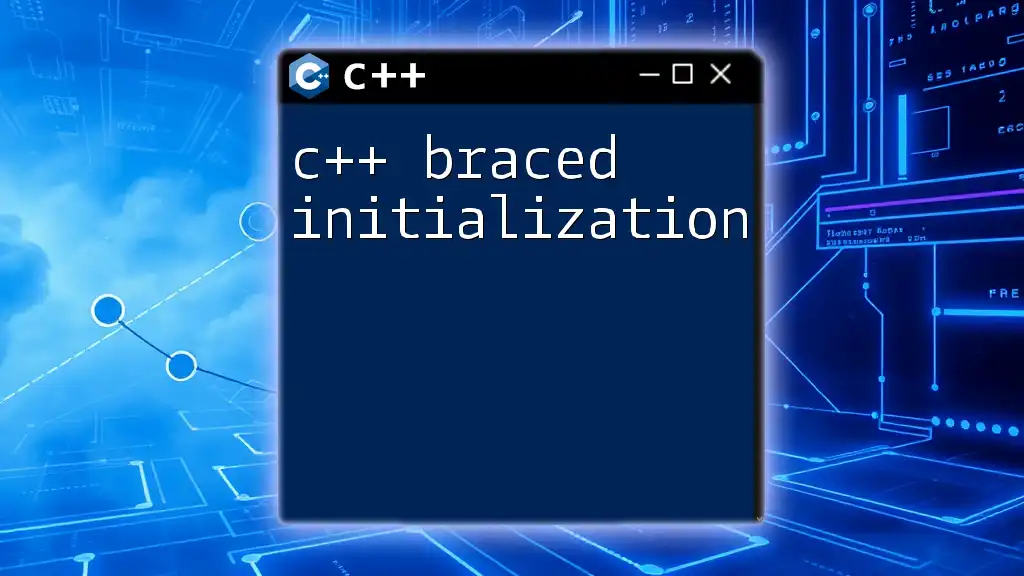
Best Practices and Tips for Using Structured Binding
When to Use Structured Binding
Consider using structured binding when your code requires extracting multiple values from a composite type. It’s particularly useful when the context is clear, and readability can be enhanced. However, traditional unpacking methods might still be appropriate in scenarios requiring clarity over compactness, such as with complex data structures.
Performance Considerations
While structured binding improves code readability, it’s important to assess its impact on performance, especially when used in high-frequency loops or performance-critical sections of code. Generally, the overhead is negligible, but awareness is key when aiming for optimal performance.
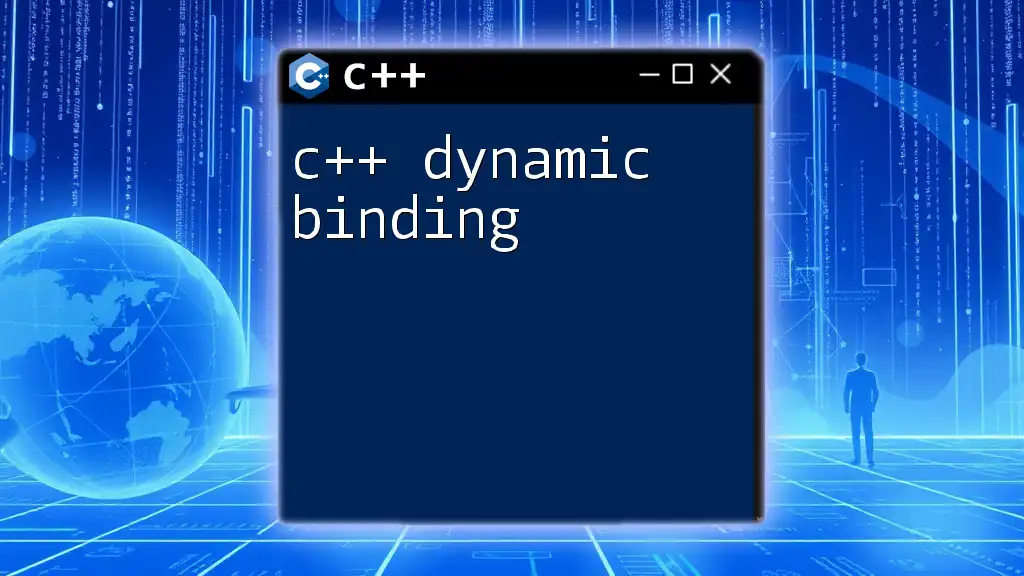
Conclusion
C++ structured binding is a powerful feature that enhances the language's capability to manage complex data types with ease and clarity. By employing structured binding, developers can significantly reduce syntax verbosity and improve code readability, leading to better maintainability and fewer bugs. As C++ continues to evolve, the importance and usefulness of structured binding are set to grow, encouraging developers to continually refine their practices and leverage new features effectively.
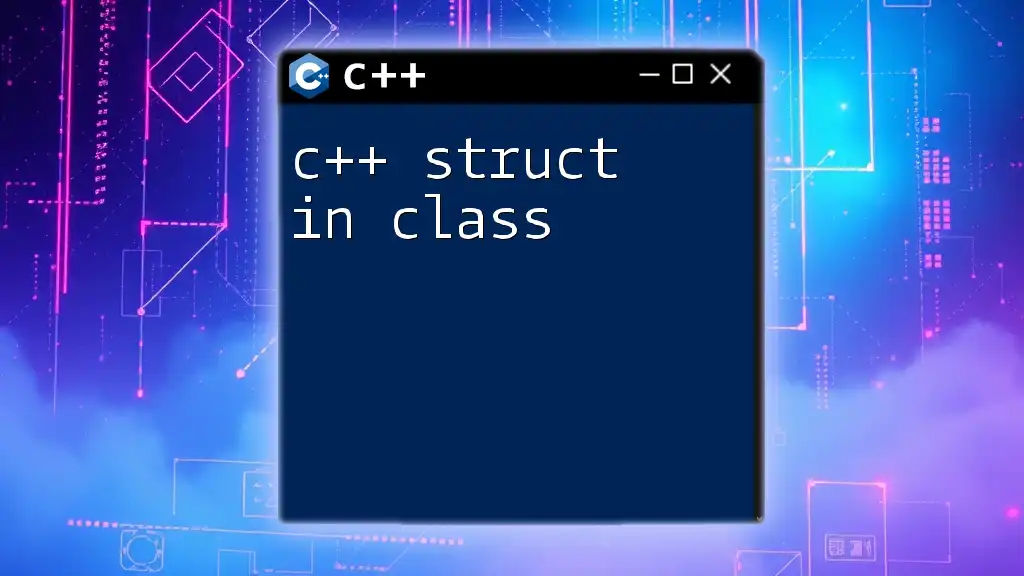
Additional Resources
To further your understanding of C++ structured binding, consider exploring additional readings, video tutorials, and community forums that discuss modern C++ features and facilitate collaborative learning amongst programmers.
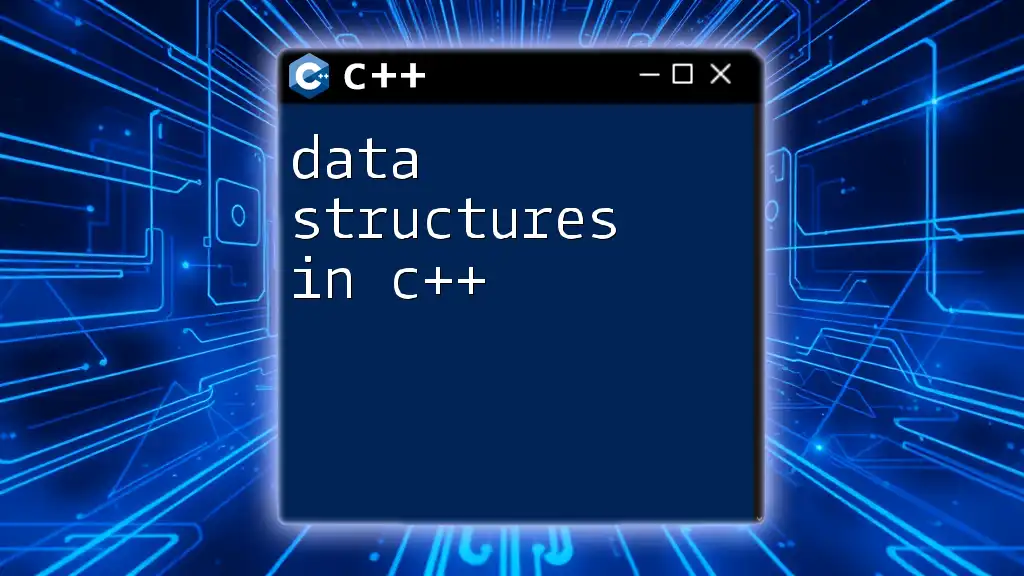
Call to Action
Implement structured binding in your projects today, and take note of how it improves your codebase's clarity. We encourage you to share your experiences and learnings in the coding community as you continue your journey to mastering C++.