C++ Python binding refers to the technique of connecting C++ code with Python, enabling seamless integration and allowing developers to use C++ libraries within Python applications.
Here's a simple example using the `pybind11` library to create bindings:
#include <pybind11/pybind11.h>
int add(int a, int b) {
return a + b;
}
PYBIND11_MODULE(example, m) {
m.def("add", &add, "A function that adds two numbers");
}
Make sure you have `pybind11` installed to compile this code, which will expose the `add` function to Python.
Understanding the Basics of Binding
What is Binding?
Binding in programming refers to the process of linking one programming language to another, allowing them to interact with each other. In the context of C++ and Python, binding enables the use of C++ code within a Python program. This is crucial for utilizing the high-performance capabilities of C++ while leveraging the simplicity and ease of Python.
Binding can be categorized into two main types: static binding (resolved at compile-time) and dynamic binding (resolved at runtime). This guide will primarily focus on dynamic binding, which is more prevalent in the C++ Python binding context.
Why Bind C++ and Python?
Choosing to bind C++ with Python is primarily driven by the strengths each language brings to the table. C++ is a compiled language, known for its exceptional performance and control over system resources. Python, on the other hand, is a high-level interpreted language that allows for rapid prototyping and ease of use. By combining these strengths, developers can achieve a blend of performance and ease-of-use that is hard to match.
For instance, performance-critical tasks (such as algorithms, graphics rendering, or processing large datasets) can be implemented in C++, while the high-level business logic, user interactions, and scripting can be managed through Python. This duality enhances overall productivity and optimizes runtime efficiency.
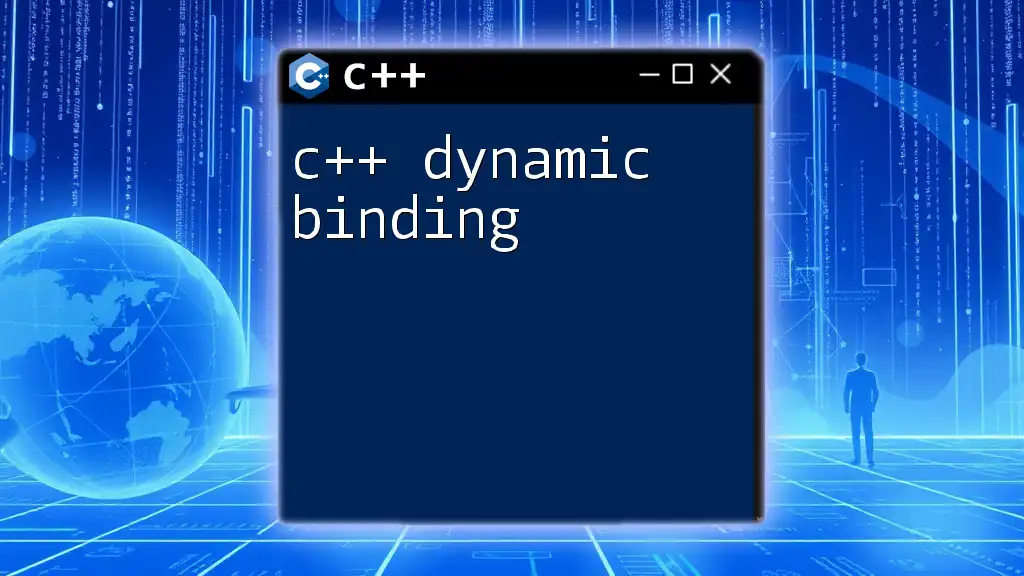
Setting Up Your Environment
Installing Required Tools
Before diving into C++ Python binding, you need to set up your development environment. Here’s a high-level overview of the tools you'll need:
- Python: Download and install the latest version of Python from the official website and ensure to add it to your system PATH.
- C++ Compiler: Depending on your operating system, you might opt for GCC on Linux, Visual Studio on Windows, or Clang on MacOS.
- Binding Libraries: Depending on your choice of binding methods (SWIG, Boost.Python, or Pybind11), you’ll need to install these libraries:
- For SWIG, follow the installation instructions on their official website.
- For Boost.Python, you can use a package manager or build it from source.
- For Pybind11, it can often be installed through pip: `pip install pybind11`.
Configuring Your Development Environment
Setting up your IDE is crucial for a smooth workflow. Popular IDEs like Visual Studio, CLion, or PyCharm can support both C++ and Python environments. Organize your project structure to keep C++ source files, Python wrappers, and interface definitions clearly separated.
Example Project Structure
project/
├── src/ # C++ source code
│ └── math_library.cpp
├── include/ # Header files
│ └── math_library.h
├── wrappers/ # Python binding files
│ └── wrapper.cpp
└── main.py # Python entry point
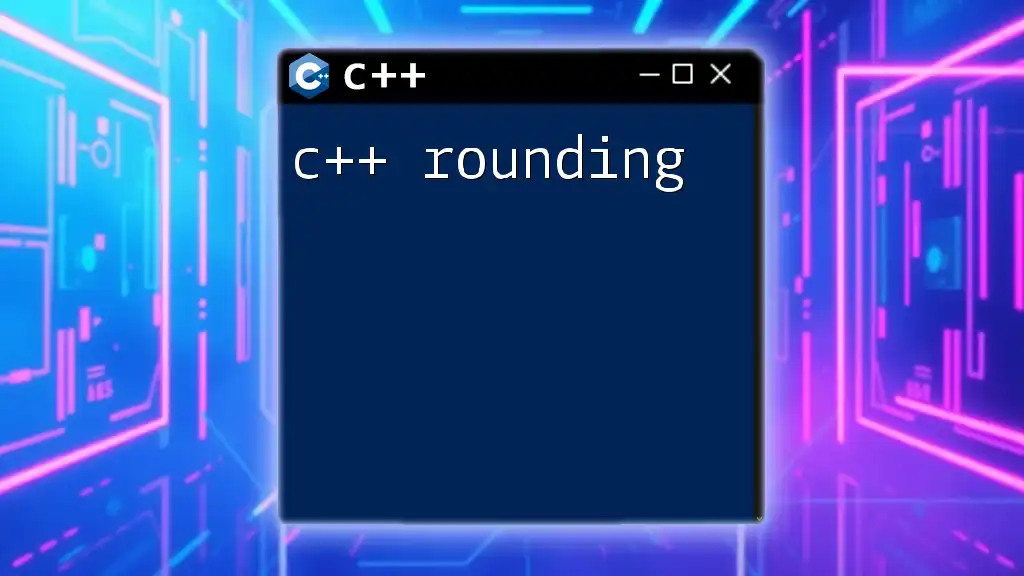
Methods of C++ Python Binding
Using SWIG (Simplified Wrapper and Interface Generator)
What is SWIG?
SWIG is an effective tool for creating binding code that allows C or C++ code to be called from various programming languages, including Python. It automates the generation of wrapper code, allowing seamless integration.
How to Create C++ Bindings with SWIG
To illustrate how to use SWIG, consider the following C++ code snippet that implements a simple math library:
// math_library.h
class MathLibrary {
public:
int add(int a, int b);
int subtract(int a, int b);
};
// math_library.cpp
#include "math_library.h"
int MathLibrary::add(int a, int b) {
return a + b;
}
int MathLibrary::subtract(int a, int b) {
return a - b;
}
Next, create a SWIG interface file called `math_library.i`:
%module math_library
%{
#include "math_library.h"
%}
%include "math_library.h"
Now, execute the SWIG command to generate the wrapper code:
swig -python -c++ math_library.i
Finally, compile the generated C++ code alongside your library:
g++ -shared -fPIC -I/usr/include/python3.x -o _math_library.so math_library_wrap.cxx math_library.cpp
You can now use the generated `_math_library.so` in Python:
import math_library
math_lib = math_library.MathLibrary()
print(math_lib.add(5, 10)) # Output: 15
Using Boost.Python
Introduction to Boost.Python
Boost.Python is a part of the larger Boost C++ Libraries and facilitates seamless interoperability between C++ and Python. It provides an easy-to-use API for creating bindings.
Creating Python Bindings Using Boost.Python
Here's an example of how to bind the same math library using Boost.Python.
Create a similar C++ file:
// math_library.cpp
#include <boost/python.hpp>
class MathLibrary {
public:
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
};
BOOST_PYTHON_MODULE(math_library) {
using namespace boost::python;
class_<MathLibrary>("MathLibrary")
.def("add", &MathLibrary::add)
.def("subtract", &MathLibrary::subtract);
}
You'll need to compile it with Boost.Python linked:
g++ -shared -fPIC -o math_library.so math_library.cpp -I/usr/include/python3.x -lboost_python3.x
You can now import it in Python:
import math_library
math_lib = math_library.MathLibrary()
print(math_lib.subtract(10, 3)) # Output: 7
Using Pybind11
What is Pybind11?
Pybind11 is a lightweight header-only library that provides a simple and efficient way to create bindings between C++ and Python.
Binding C++ Code with Pybind11
Similar to the previous examples, you can implement the bindings as follows:
// math_library.cpp
#include <pybind11/pybind11.h>
class MathLibrary {
public:
int multiply(int a, int b) { return a * b; }
};
namespace py = pybind11;
PYBIND11_MODULE(math_library, m) {
py::class_<MathLibrary>(m, "MathLibrary")
.def("multiply", &MathLibrary::multiply);
}
Compile with Pybind11 linked:
g++ -shared -fPIC -o math_library.so math_library.cpp -I/usr/include/python3.x -I/path/to/pybind11/include
Now you can use this in Python:
import math_library
math_lib = math_library.MathLibrary()
print(math_lib.multiply(6, 7)) # Output: 42
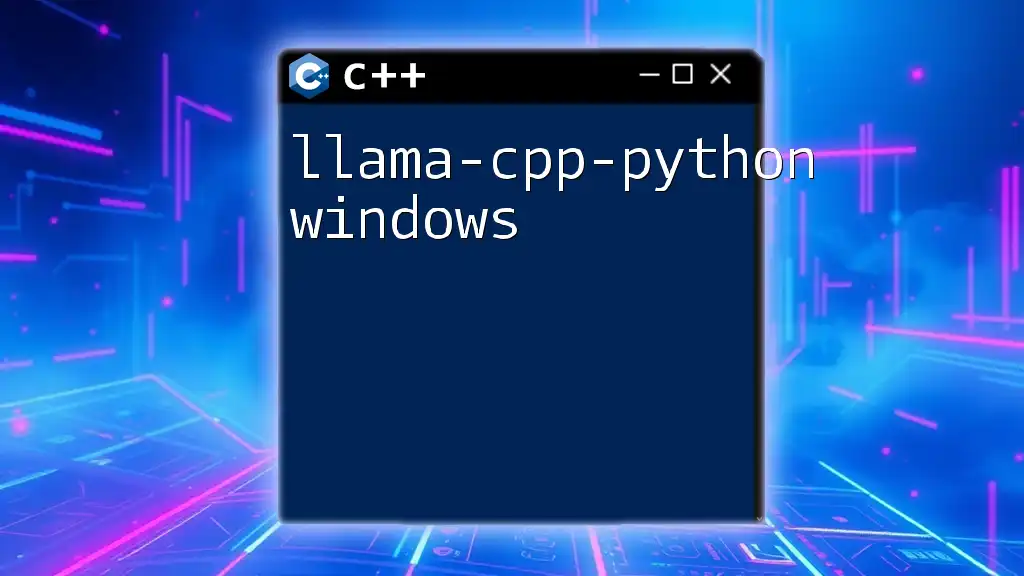
Practical Examples and Use Cases
Example Project: Simple MathLibrary
To create a practical example, let’s build a simple math library, which combines addition and multiplication functionalities.
Define the functionalities in C++:
// math_library.cpp
class MathLibrary {
public:
int add(int a, int b);
int multiply(int a, int b);
};
int MathLibrary::add(int a, int b) {
return a + b;
}
int MathLibrary::multiply(int a, int b) {
return a * b;
}
Next, bind it using Pybind11:
PYBIND11_MODULE(math_lib, m) {
py::class_<MathLibrary>(m, "MathLibrary")
.def("add", &MathLibrary::add)
.def("multiply", &MathLibrary::multiply);
}
This illustrative project showcases how easy it is to mix C++ performance and Python simplicity. Compile and run the Python code to witness the functional outcomes.
Use Case: Enhancing a Python Application with C++
Imagine you are working on an application that processes large datasets for machine learning. While Python excels in managing data and model training, operations like data normalization or matrix manipulation can significantly benefit from C++'s speed.
Suppose you develop a C++ library to perform these operations more efficiently, and you then create bindings to utilize this power directly within your Python scripts, thus achieving enhanced performance.
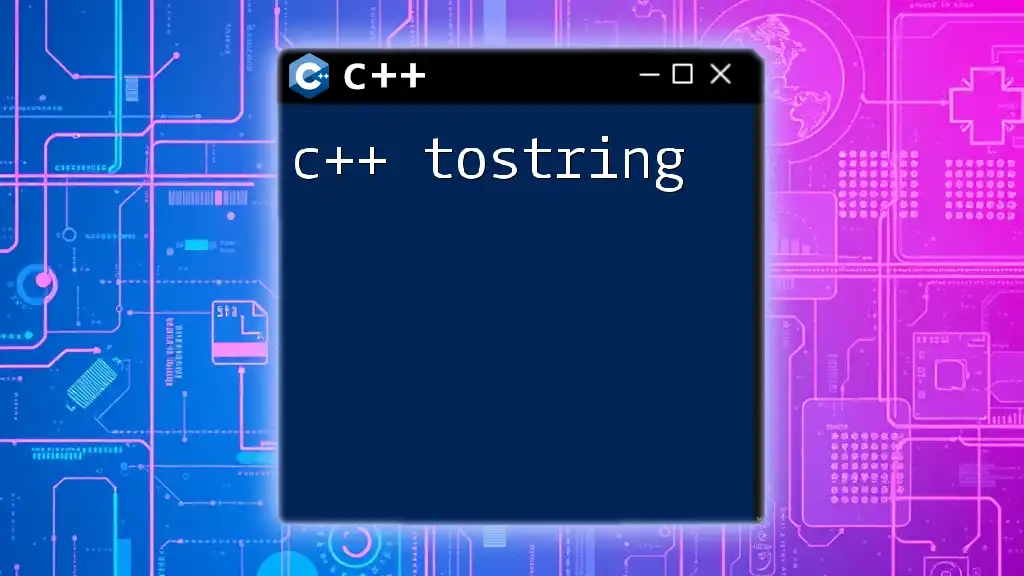
Error Handling and Debugging in C++ Python Binding
Common Issues and Solutions
While binding C++ code to Python is powerful, developers often encounter common issues:
Linking Errors: Often, these issues arise due to mismatched function signatures or forgetting to include certain libraries during compilation. Always ensure that the parameters in Python and C++ match precisely.
Type Conversion Errors: C++ and Python maintain different type systems, which may lead to runtime errors when data types are incompatible. Utilizing appropriate bindings and ensuring type safety can mitigate these problems.
For instance, if you pass a Python list to a C++ function expecting an array, you will encounter a type conversion error. Always validate input types.
Tips for Effective Debugging
Effective debugging requires leveraging the right tools. Here are some strategies to consider:
- gdb: Use the GNU Debugger to step through your C++ code as it is invoked by Python.
- valgrind: Tools like Valgrind can help detect memory leaks, which may arise when C++ is used alongside Python.
- Logging: Adding robust logging to both your Python and C++ code will provide insight into where errors occur.
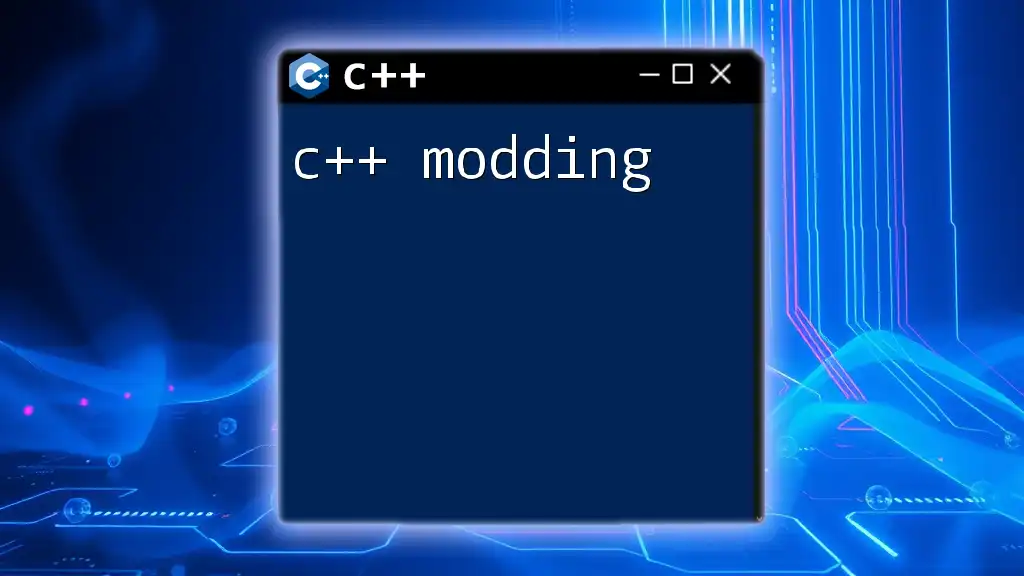
Conclusion and Future Directions
In conclusion, C++ Python binding offers a powerful combination of speed and flexibility, allowing developers to optimize performance-sensitive components while enjoying Python's simplicity. As the demand for efficient software solutions continues to grow, the integration between C++ and Python is likely to evolve, with tools becoming more user-friendly and automatic.
Future trends in this space could include deeper integration with machine learning frameworks, improved IDE support for mixed-language projects, and enhanced community support for addressing common challenges.
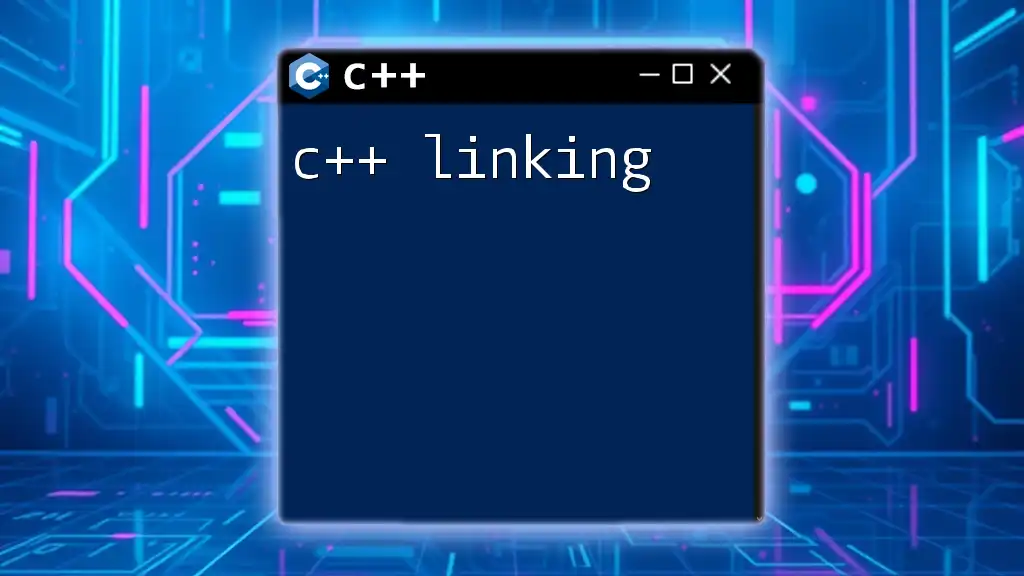
Additional Resources
For those who wish to further explore C++ Python binding, there are many resources available, including:
- Official documentation for SWIG, Boost.Python, and Pybind11.
- Online courses focusing on C++ and Python integration.
- Blogs and community forums where you can ask questions and share experiences.
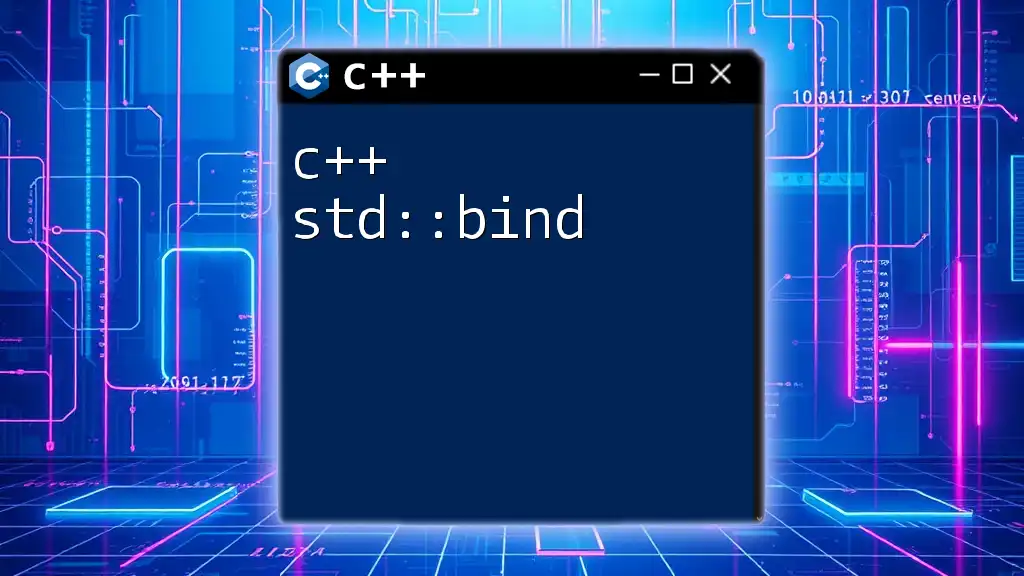
Call to Action
As you familiarize yourself with the methods of C++ Python binding, consider experimenting with the code examples provided here. Share your experiences, challenges, and successes. If you’re looking to deepen your understanding, consider signing up for our specialized courses or workshops designed to streamline your learning process and empower you to excel in this integrated programming world.