C++ rounding can be achieved using the `round()` function from the `<cmath>` library, which rounds a floating-point number to the nearest integer.
Here's a code snippet demonstrating this:
#include <iostream>
#include <cmath>
int main() {
double num = 4.7;
std::cout << "Rounded value: " << round(num) << std::endl; // Output: 5
return 0;
}
Understanding Rounding in C++
What is Rounding?
Rounding is the process of adjusting a number to its nearest specified value, which often leads to simpler figures that are easier to work with or interpret. In programming, rounding is crucial for avoiding errors in calculations, particularly when dealing with floating-point arithmetic. Miscalculations can arise from the inherent imprecision in how floating-point numbers are represented in computers. Therefore, effective rounding ensures that your data displays accurate representations of numbers.
Types of Rounding Methods
Rounding encompasses various techniques such as rounding up, rounding down, and more nuanced forms like rounding to the nearest even number or significant digits. Understanding these methods is essential when determining how to handle numerical data effectively in C++.
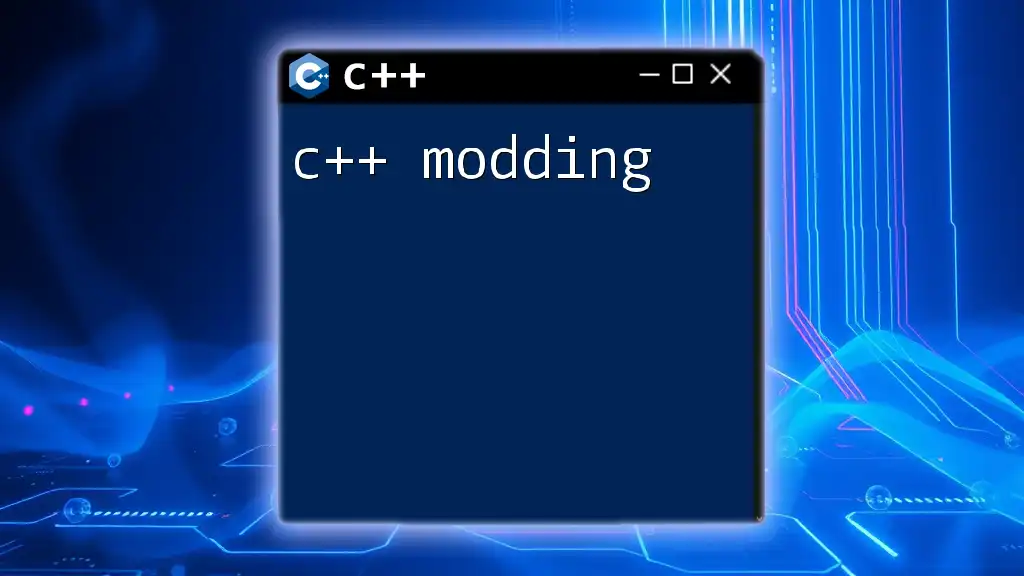
C++ Built-in Rounding Functions
Using `round()`
The `round()` function in C++ rounds a floating point number to the nearest integer. In cases where the fractional component is .5 or higher, it rounds up; otherwise, it rounds down. This behavior makes it particularly useful in many applications.
Prototype:
double round(double x);
Example:
#include <iostream>
#include <cmath>
int main() {
double num = 3.6;
std::cout << "Rounded value: " << round(num) << std::endl; // Outputs: 4
return 0;
}
Use cases: `round()` can be beneficial in scenarios such as financial calculations, where you want to ensure that numbers are represented without fractions.
Using `floor()`
The `floor()` function rounds a number down to the nearest integer, effectively discarding the fractional part. It is particularly useful when you need to ensure that values do not exceed certain thresholds.
Prototype:
double floor(double x);
Example:
#include <iostream>
#include <cmath>
int main() {
double num = 3.6;
std::cout << "Floor value: " << floor(num) << std::endl; // Outputs: 3
return 0;
}
Use cases: Use `floor()` when working with discrete quantities, such as the number of items to fit into a container.
Using `ceil()`
Conversely, the `ceil()` function rounds a number up to the nearest integer. This function guarantees that you do not underestimate a value — it's a common choice in cases requiring caution to avoid underestimating requirements.
Prototype:
double ceil(double x);
Example:
#include <iostream>
#include <cmath>
int main() {
double num = 3.4;
std::cout << "Ceil value: " << ceil(num) << std::endl; // Outputs: 4
return 0;
}
Use cases: `ceil()` is often applied in financial projections, such as determining the maximum number of items that can be bought within a budget.
Using `trunc()`
The `trunc()` function simply removes the fraction of a floating-point number, effectively rounding towards zero. It doesn't consider whether to round up or down; it merely truncates the value.
Prototype:
double trunc(double x);
Example:
#include <iostream>
#include <cmath>
int main() {
double num = 3.8;
std::cout << "Truncated value: " << trunc(num) << std::endl; // Outputs: 3
return 0;
}
Use cases: The `trunc()` function can be ideal when you are only interested in the integer part of a number and need to prepare data for external systems that do not handle fractional values well.
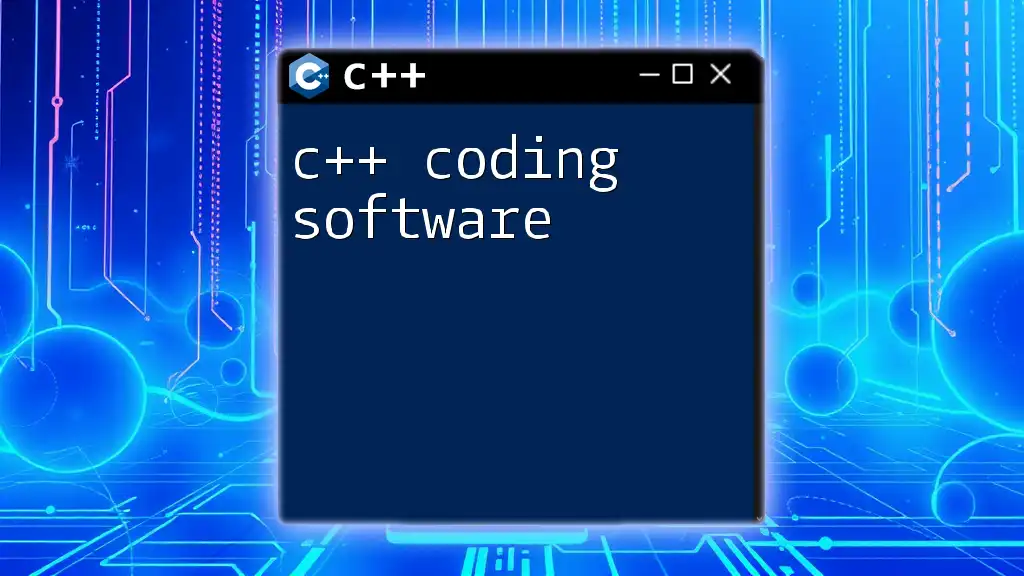
Custom Rounding Functions
Implementing Custom Rounding Logic
While the built-in functions serve general rounding purposes, scenarios may arise that require more specialized rounding logic. For instance, you might need to round to a specified number of decimal places rather than to the nearest whole number. Custom rounding functions can address needs specific to your application.
Example of Custom Rounding Function
Here’s a simple example of a custom rounding function that rounds a number to a specific number of decimal places.
#include <iostream>
#include <cmath>
double customRound(double num, int decimals) {
double factor = pow(10, decimals);
return round(num * factor) / factor;
}
int main() {
double num = 3.14159;
std::cout << "Custom rounded value: " << customRound(num, 2) << std::endl; // Outputs: 3.14
return 0;
}
Explanation: This function takes a number and the desired number of decimal places as arguments. It calculates a factor based on the number of decimals, multiplies the number by that factor, applies the standard `round()`, and then divides back to get the properly rounded number.
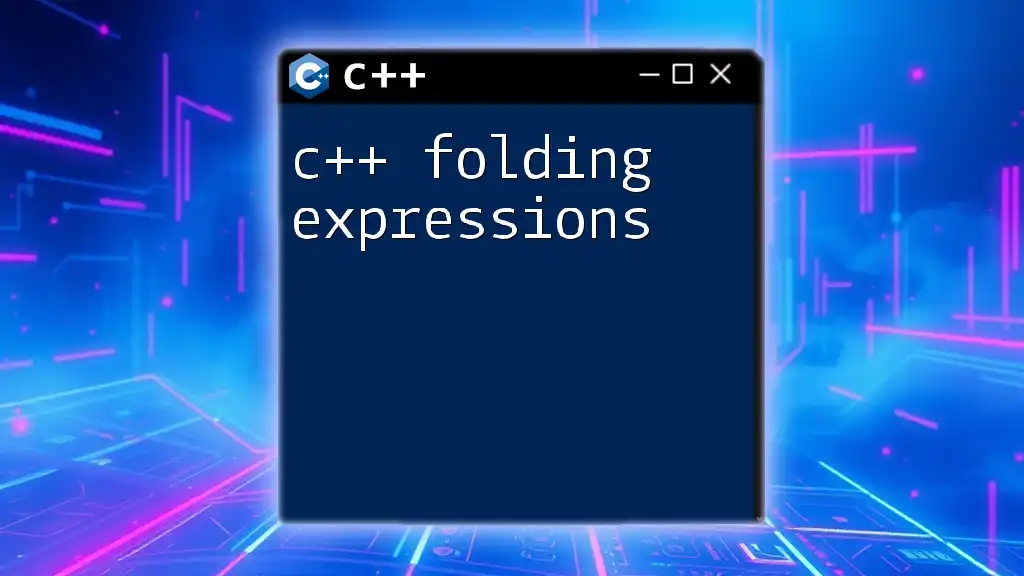
Best Practices for Rounding in C++
Avoiding Common Mistakes
One of the most prevalent mistakes arises from misunderstanding how rounding functions behave with negative numbers. For example, while `round(-2.5)` rounds to -3, `floor(-2.5)` results in -3 as well, while `ceil(-2.5)` returns -2. These subtleties can lead to unexpected results if not accounted for.
Performance Considerations
In performance-sensitive applications, consider the number of rounding operations performed. Built-in functions are usually optimized; however, custom implementations may require careful management to avoid unnecessary computations, especially in loops or large datasets. Choose the appropriate method based on the specific context and performance needs of your application.
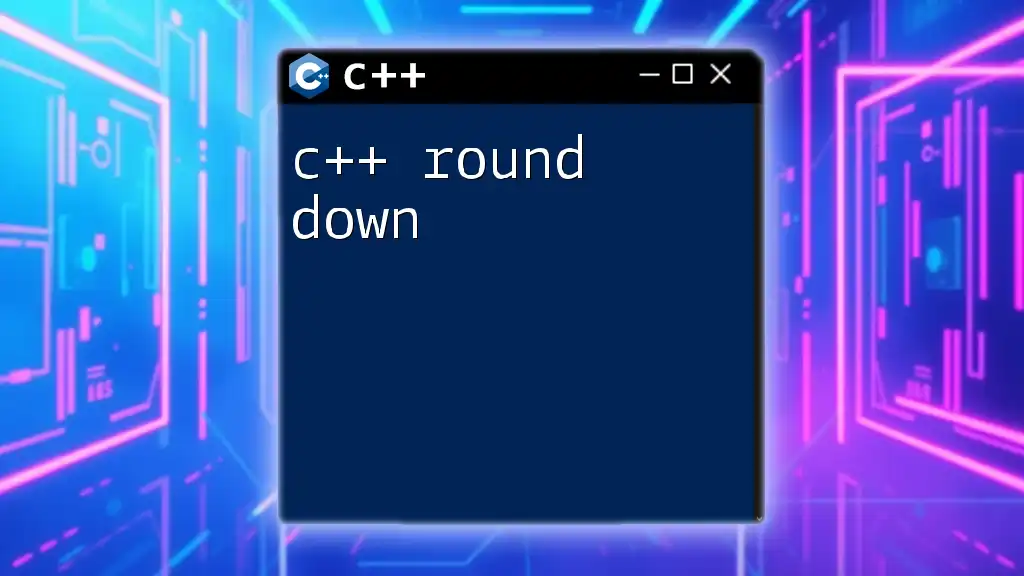
Conclusion
Understanding C++ rounding is not merely about applying built-in functions; it’s about knowing the nuances of each method, recognizing potential pitfalls, and crafting tailored solutions to meet your application’s requirements.
By developing an awareness of how and when to utilize rounding effectively, you can prevent errors in data handling and produce more reliable, user-friendly outputs.
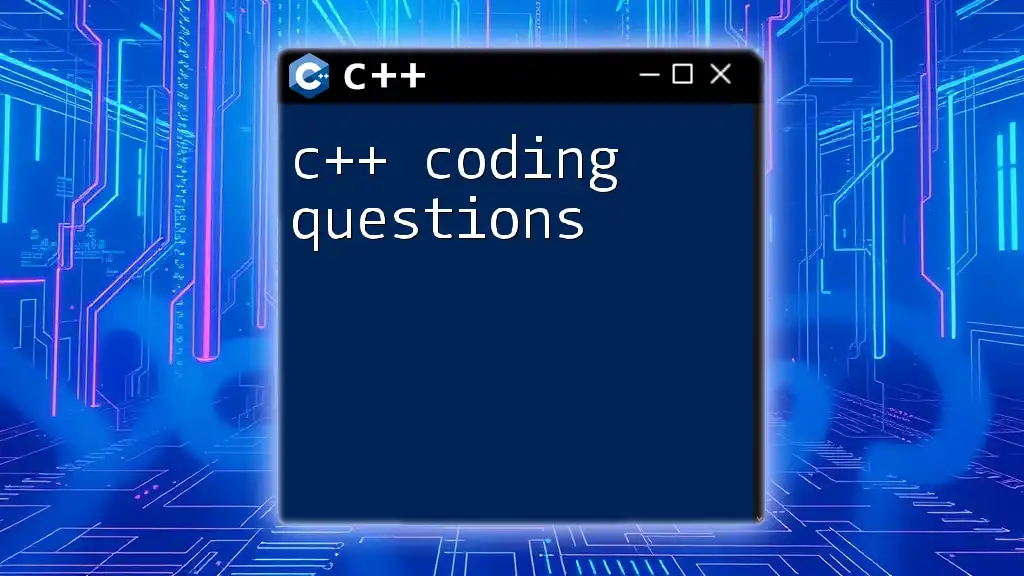
Additional Resources
For further insight into C++ rounding and mathematical functions, refer to the C++ documentation available on the official websites and consider exploring textbooks and courses that delve into numerical methods and mathematical modeling.
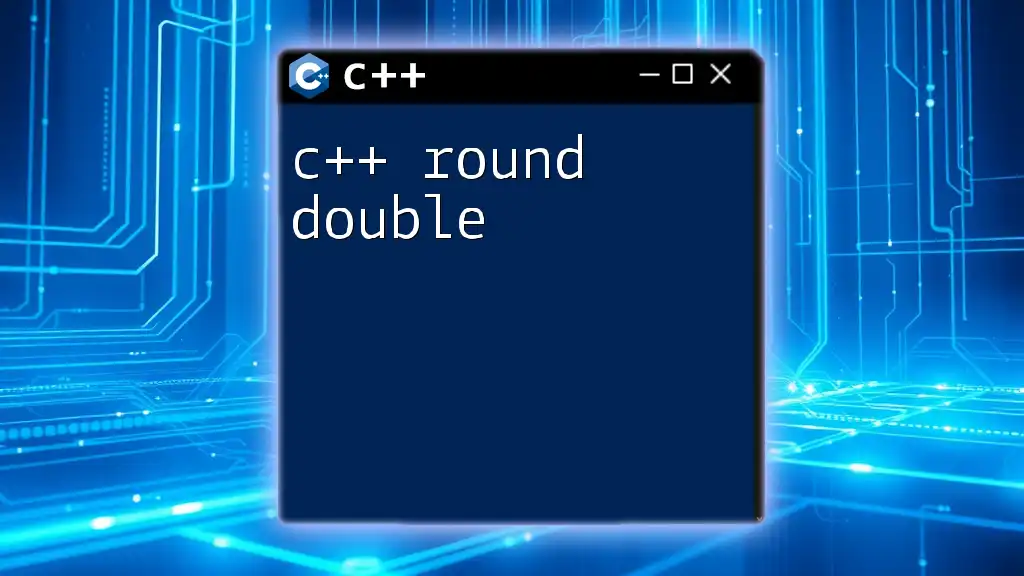
Call to Action
Practice using rounding functions in your projects to solidify your understanding. Stay tuned for more posts on essential C++ programming techniques that can enhance your coding skills and efficiency.