In C++, you can round down a floating-point number to the nearest integer using the `floor` function from the `<cmath>` library. Here's a quick example:
#include <iostream>
#include <cmath>
int main() {
double number = 5.7;
double roundedDown = floor(number);
std::cout << "Rounded down: " << roundedDown << std::endl; // Output: 5
return 0;
}
Understanding Rounding in C++
Rounding is a fundamental operation in programming, particularly when dealing with numerical values. When we talk about rounding, we refer to methods that help approximate numbers to a desired degree of precision. In C++, rounding down is a common requirement, especially when handling calculations that involve monetary values, statistical analysis, or any scenario where losing the decimal portion is necessary.
What is Rounding?
Rounding is the process of altering the digits of a number to make it easier to work with while maintaining a value close to the original number. The three primary types of rounding include:
- Round off: Adjusting the number to the nearest integer.
- Round up: Increasing the number to the nearest integer.
- Round down: Decreasing the number to the nearest integer.
These methods help in simplifying complex numerical calculations and facilitating more straightforward data manipulation.
The Difference Between Round Down and Round Up
When it comes to rounding, understanding the distinction between rounding down and rounding up is crucial:
-
Rounding down takes any given number and reduces it to the nearest integer less than or equal to that number. For example, rounding down 5.8 results in 5.
-
Rounding up, on the other hand, takes a number and increases it to the nearest integer greater than or equal to that number. So, rounding up 5.3 results in 6.
In scenarios such as financial transactions where fractions of a cent may not be processed, rounding down is often preferable.
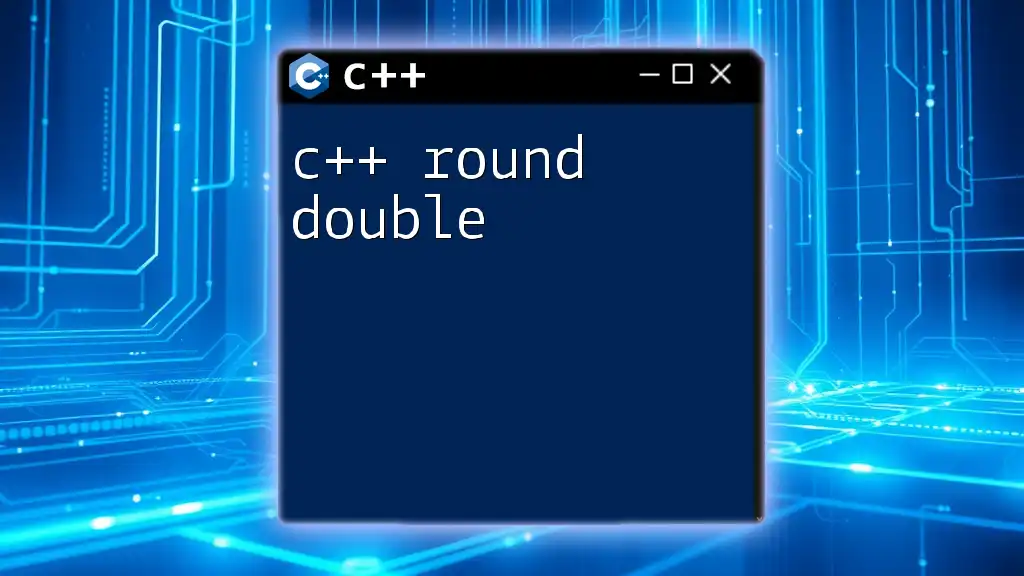
Rounding Down in C++
The `floor()` function is one of the primary means of rounding down in C++. This function belongs to the `<cmath>` library and is essential for returning the largest integer value that is less than or equal to the specified number.
The Floor Function
The `floor()` function can be used as follows:
#include <iostream>
#include <cmath>
int main() {
double value = 5.8;
double roundedDown = floor(value);
std::cout << "Floor of " << value << " is " << roundedDown << std::endl; // Output: 5
return 0;
}
Explanation
In this code snippet, we include the `<cmath>` header, which provides access to the `floor()` function. We define a double variable, `value`, and apply the `floor()` function, storing the result in `roundedDown`. This effectively truncates any decimal portion, giving us the largest integer less than or equal to `value`.
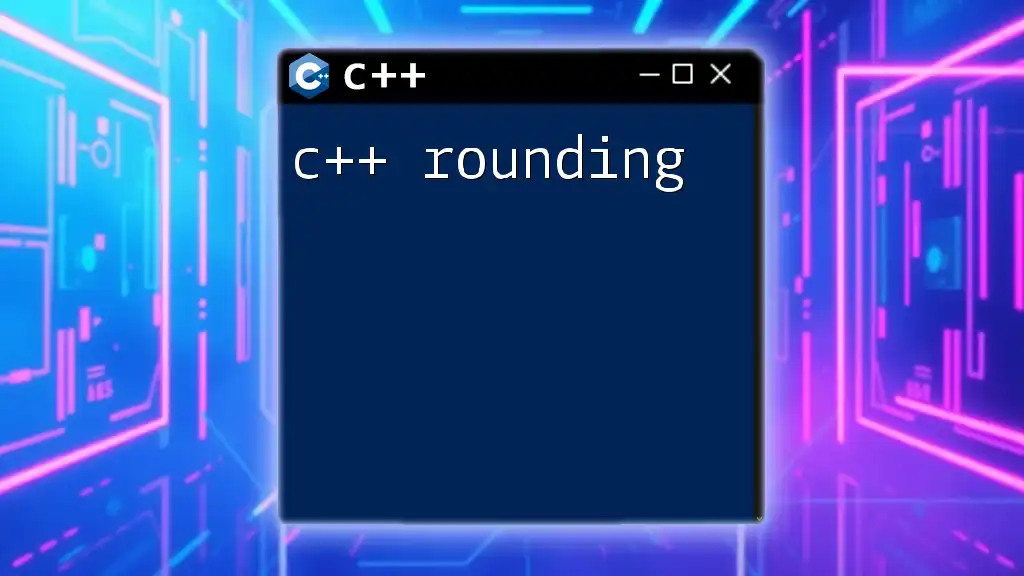
Alternative Methods for Rounding Down
While the `floor()` function is a straightforward approach, there are alternative methods to achieve rounding down in C++.
Type Casting
Type casting can be a simple and effective way to truncate decimal values in C++. When you cast a floating-point number to an integer, the decimal portion is discarded.
Code Snippet: Rounding Down via Type Casting
#include <iostream>
int main() {
double value = 7.9;
int roundedDown = static_cast<int>(value);
std::cout << "Rounded down using type casting: " << roundedDown << std::endl; // Output: 7
return 0;
}
Explanation
In this example, we define a double variable `value` and cast it to an integer using `static_cast<int>`. This operation effectively rounds down `7.9` to `7` by removing the decimal value. This method can be useful when you need an integer result and can tolerate the loss of precision.
Using Integer Division
Integer division is another straightforward method to achieve rounding down. When you divide two integers in C++, the result is also an integer, which automatically rounds down.
Code Snippet: Rounding Down with Integer Division
#include <iostream>
int main() {
double value = 9.5;
int roundedDown = value / 1; // integer division
std::cout << "Rounded down using integer division: " << roundedDown << std::endl; // Output: 9
return 0;
}
Explanation
In this example, `value` is treated as a double, but when you divide by `1`, it converts the resulting value to an integer through implicit integer division. This effectively rounds down to `9` by discarding the decimal.
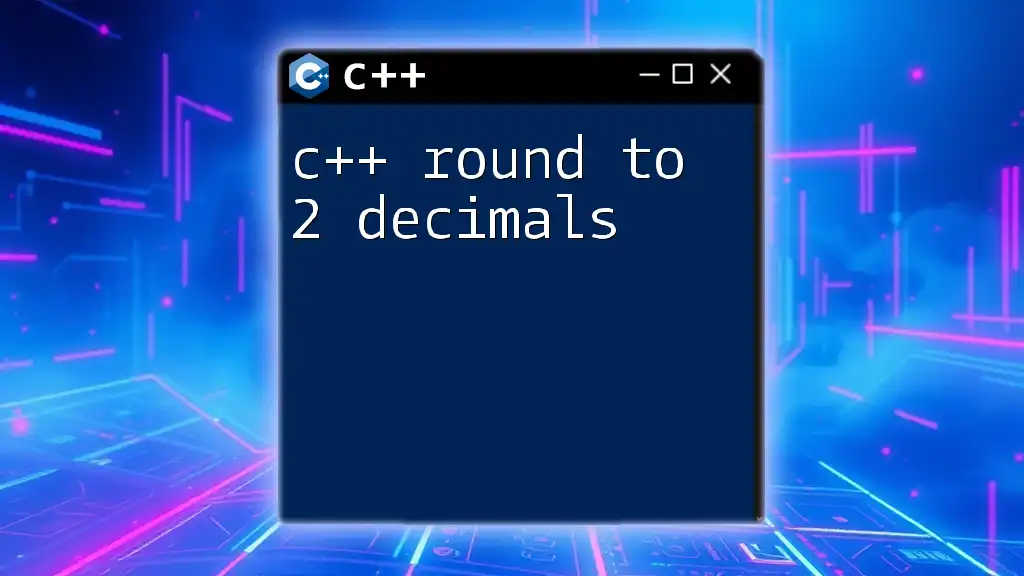
Common Use Cases for Rounding Down in C++
Data Analysis and Statistics
When conducting data analysis, you may often work with datasets containing decimal values. In many cases, particularly in finance, you may want to implement rules that only deal with whole numbers. For instance, if you are calculating discounts or taxes, you might want to round down to ensure that you are not overcharging customers.
Game Development
In game development, rounding down can be essential for mechanics such as score calculations, inventory management, or determining player levels. For example, if a player collects 9.9 experience points, rounding down ensures they do not gain an entire level until reaching 10.
Mathematical Calculations
Rounding down becomes particularly useful in complex mathematical computations where precision is less critical than ensuring results remain within expected ranges. For example, when capturing dimensions in construction, values are often rounded down to ensure fittings match actual measurements.
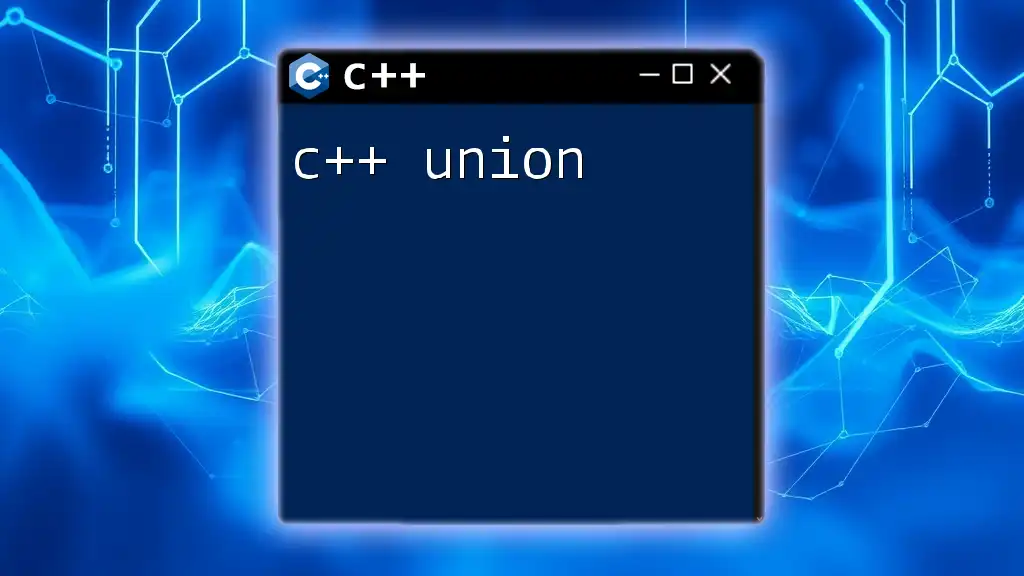
C++ Round Up: Contrast and Comparison
The Ceil Function
For completeness, it’s essential to understand the counterpart of rounding down: rounding up. The `ceil()` function, also found in the `<cmath>` header, serves this purpose by returning the smallest integer greater than or equal to the specified number.
Code Snippet: Using the Ceil Function
#include <iostream>
#include <cmath>
int main() {
double value = 5.3;
double roundedUp = ceil(value);
std::cout << "Ceil of " << value << " is " << roundedUp << std::endl; // Output: 6
return 0;
}
Choosing Between Round Down and Round Up
When deciding whether to round down or round up in your applications, it's vital to consider the context. Factors that may influence your choice include:
- The desired outcome: Is precision or approximation more critical?
- The type of data: Are you working with financial, statistical, or other numerical data?
- Business logic: What are the requirements for the outputs in your specific domain?
By understanding these nuances, one can effectively choose which rounding method to apply in different scenarios.
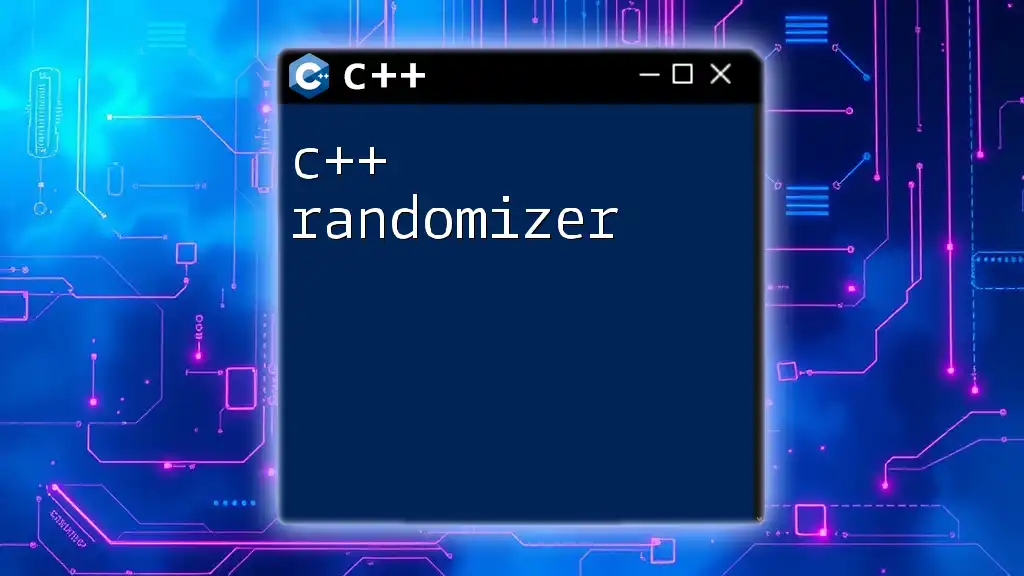
Conclusion
In C++, understanding how to effectively round down is essential for performing accurate calculations, especially in areas such as finance, data analysis, and game development. Whether you choose to use the `floor()` function, type casting, or integer division, each method has its own merits and use cases.
Practicing these rounding techniques will enable you to manipulate and analyze numerical data successfully. If you have any questions or experiences you'd like to share, feel free to leave a comment!
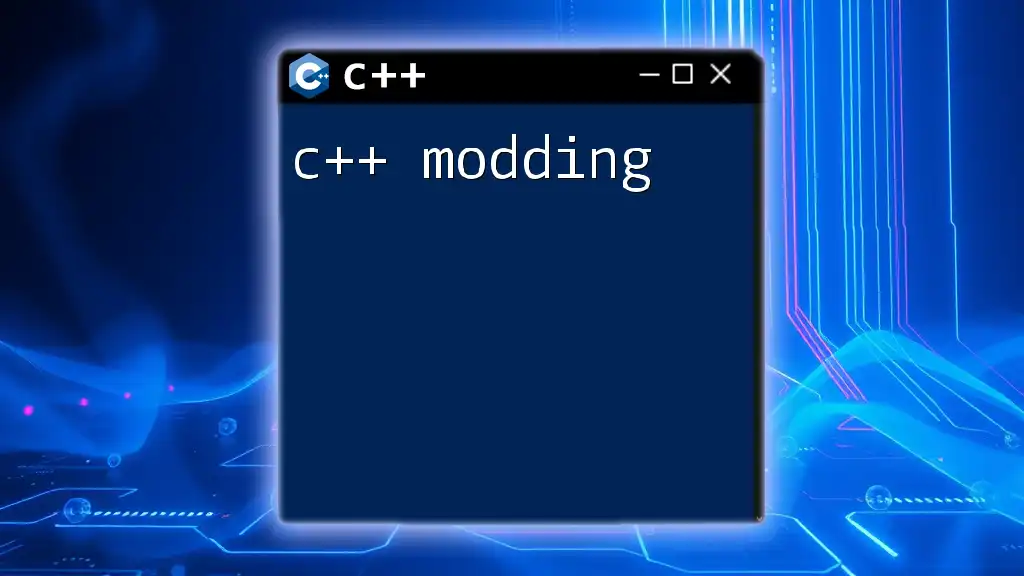
Additional Resources
For further exploration, consider referring to the official C++ documentation or additional programming resources. Engaging with practical examples will enhance your understanding of rounding techniques in C++.
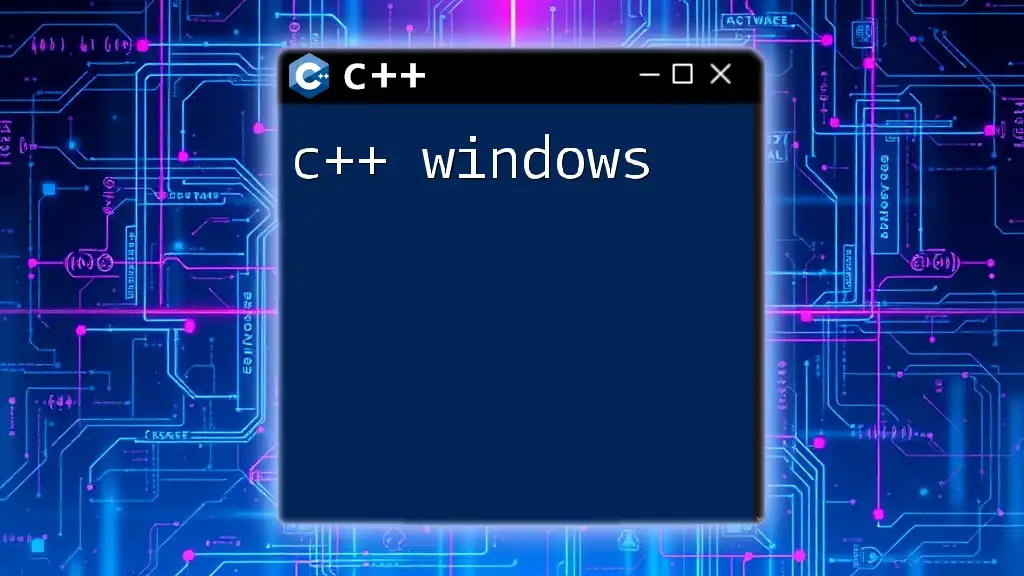
Call to Action
Make sure to subscribe for more C++ programming tips and tricks! Share this article with peers who might find it useful, and let’s build a community focused on mastering C++ together!