C++ fundamentals encompass the core concepts and syntax that form the foundation of programming in C++, enabling developers to write efficient and effective code.
Here's a simple example illustrating the usage of variables and basic input/output in C++:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old!" << endl;
return 0;
}
History of C++
C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s, evolving from the C programming language. It was designed to add object-oriented features to C while still maintaining low-level capabilities. Understanding the history of C++ is essential to appreciate its evolution and versatility.
Over the years, C++ has undergone several significant updates. The primary standards include:
- C++98: The first standardized version of C++, introduced fundamental features such as templates and exceptions.
- C++11: This version revolutionized C++ with features like auto type declarations, nullptr, range-based for loops, and lambda expressions.
- C++14 and C++17: These updates improved the language further, adding features like generic lambdas and structured bindings.
- C++20: The latest significant standard introduced concepts, modules, and coroutines, marking a new era in C++ programming.
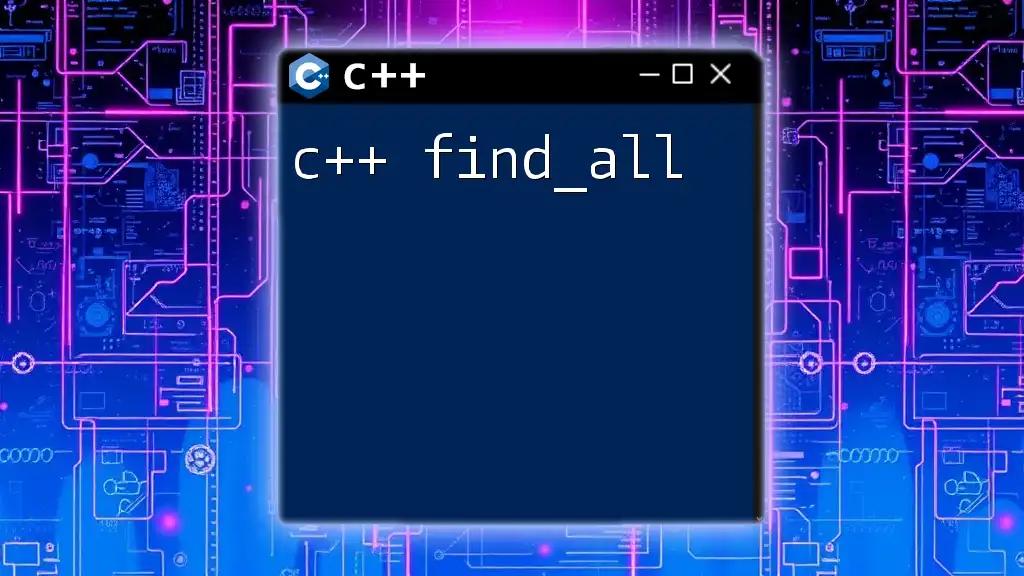
Basic Syntax
Structure of a C++ Program
A fundamental understanding of the structure of a C++ program is vital for beginners. The most basic C++ program consists of the `main` function, which serves as the entry point for execution.
The essential components of a C++ program are:
- Preprocessor Directives: Statements that begin with `#`, which are processed before compilation.
- Function Definitions: C++ programs are organized into functions, with the `main` function acting as the starting point.
- Statements: Commands that instruct the program to perform tasks.
Code Example: Basic C++ Program
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Data Types
C++ supports a variety of data types, which categorize the specific type of value a variable can hold. These data types are classified as:
-
Fundamental Data Types:
- `int`: Represents integer values.
- `float`: Used for single-precision floating-point numbers.
- `char`: Stores a single character.
- `double`: For double-precision floating-point numbers.
- `bool`: Represents a boolean value (true or false).
-
User-Defined Data Types:
- `struct`: A collection of different data types grouped together.
- `union`: Similar to structures but shares memory space.
- `enum`: A user-defined type consisting of named integral constants.
- `class`: Defines objects that encapsulate data and functions.
Explanations and Examples:
The `int` type can hold various integer values, and using it effectively involves knowing its size and range. For a user-defined type, consider the following structure defining a person:
struct Person {
std::string name;
int age;
};
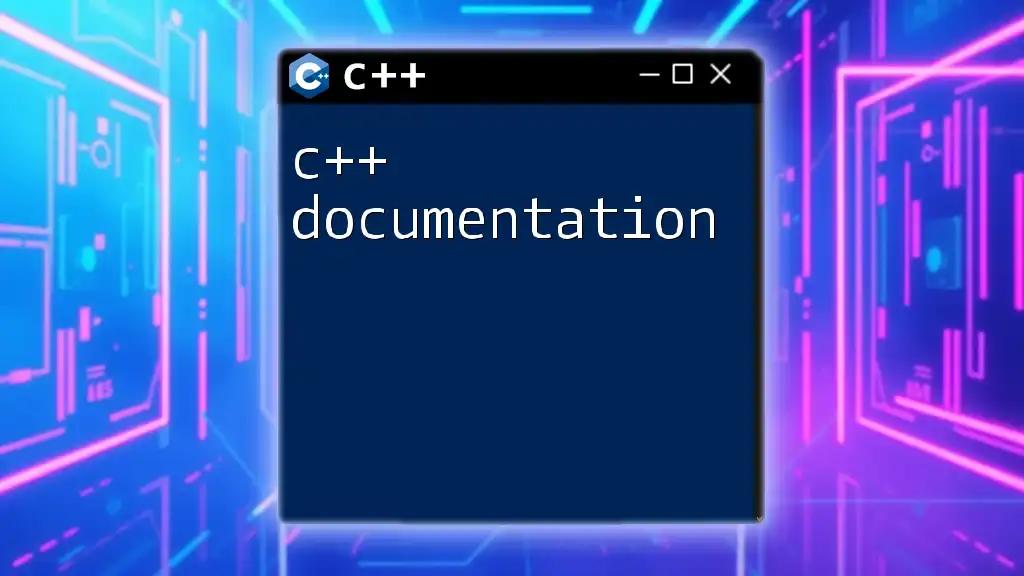
Control Flow
Conditional Statements
Conditional statements control the flow of a program by executing certain blocks of code based on specified conditions.
If-Else Statements:
The `if-else` statement allows branching logic, executing different code based on different conditions.
Code Example:
int a = 5, b = 10;
if (a > b) {
std::cout << "A is greater than B.";
} else {
std::cout << "A is not greater than B.";
}
Switch Statements:
Switch statements offer an alternative to multiple `if` checks. They are particularly useful when evaluating a single variable against many constants.
Code Example:
int day = 3;
switch (day) {
case 1: std::cout << "Monday"; break;
case 2: std::cout << "Tuesday"; break;
case 3: std::cout << "Wednesday"; break;
default: std::cout << "Invalid day";
}
Loops
Loops are crucial for iterating over sets of data or repeatedly executing a block of code.
For Loop:
The `for` loop is widely used for iterating with a known number of iterations.
Code Example:
for(int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
While Loop:
The `while` loop continues executing as long as a specified condition remains true.
Code Example:
int i = 0;
while (i < 5) {
std::cout << i << std::endl;
i++;
}
Do-While Loop:
The `do-while` loop is similar to the `while` loop, but guarantees at least one execution of the loop body.
Code Example:
int j = 0;
do {
std::cout << j << std::endl;
j++;
} while (j < 5);
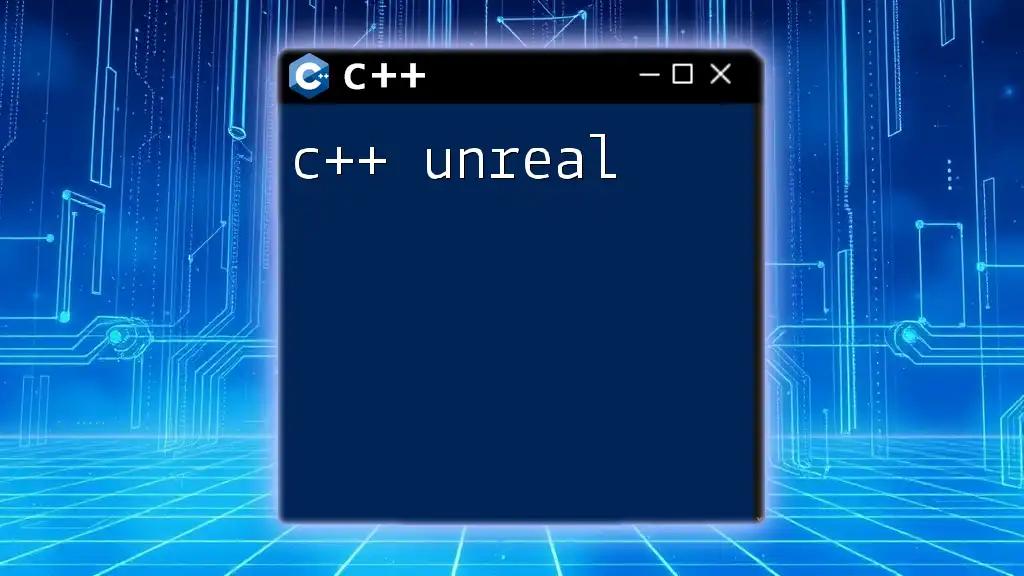
Functions
Definition and Syntax
Functions break down complex problems into smaller, manageable parts, enhancing code readability and reusability.
To define a function, use the following syntax:
return_type function_name(parameters) {
// function body
}
Code Example: Basic Function
int add(int a, int b) {
return a + b;
}
Parameters and Return Types
C++ allows passing parameters either by value or by reference, which enables flexibility in how data is managed.
Code Example:
void greet() {
std::cout << "Hello!" << std::endl;
}
Function Overloading
Function overloading permits the same function name to be used for different purposes based on varying parameter types or counts.
Code Example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
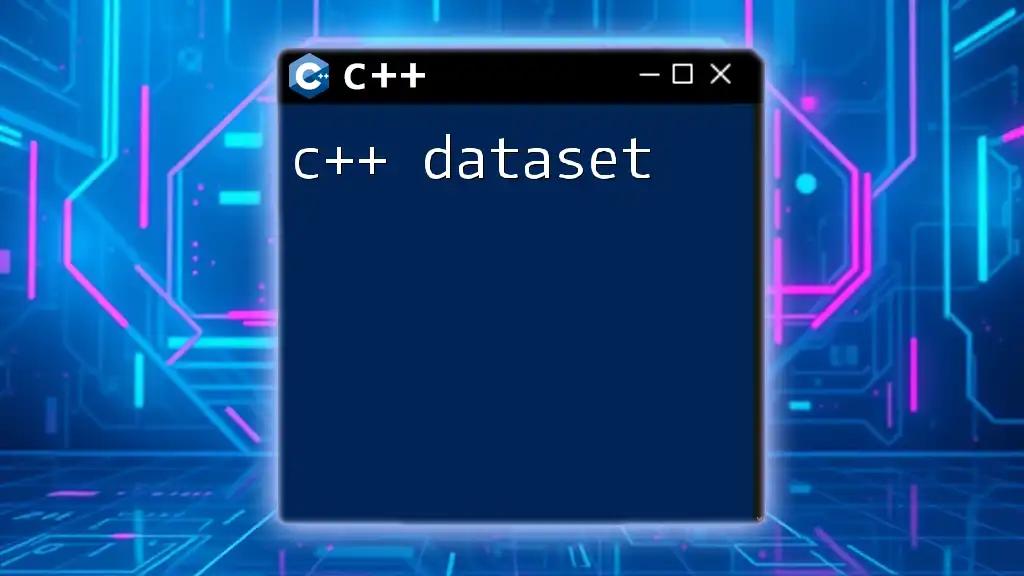
Object-Oriented Programming (OOP) Concepts
Classes and Objects
Classes serve as blueprints for creating objects, encapsulating data and behavior.
Code Example: Defining a Class
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep beep!" << std::endl;
}
};
Encapsulation
Encapsulation is the bundling of data and methods operating on that data within a single unit, restricting access to some components. The use of `public`, `protected`, and `private` access specifiers is crucial for data hiding.
Inheritance
Inheritance enables new classes to inherit attributes and behaviors from existing classes, promoting code reusability and logical hierarchy.
Code Example:
class Vehicle {
public:
void drive() {
std::cout << "Driving a vehicle" << std::endl;
}
};
class Car : public Vehicle {
public:
void honk() {
std::cout << "Car horn!" << std::endl;
}
};
Polymorphism
Polymorphism allows for methods to perform different tasks based on the object that calls them, enhancing flexibility in the program.
Code Example: Virtual Functions
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
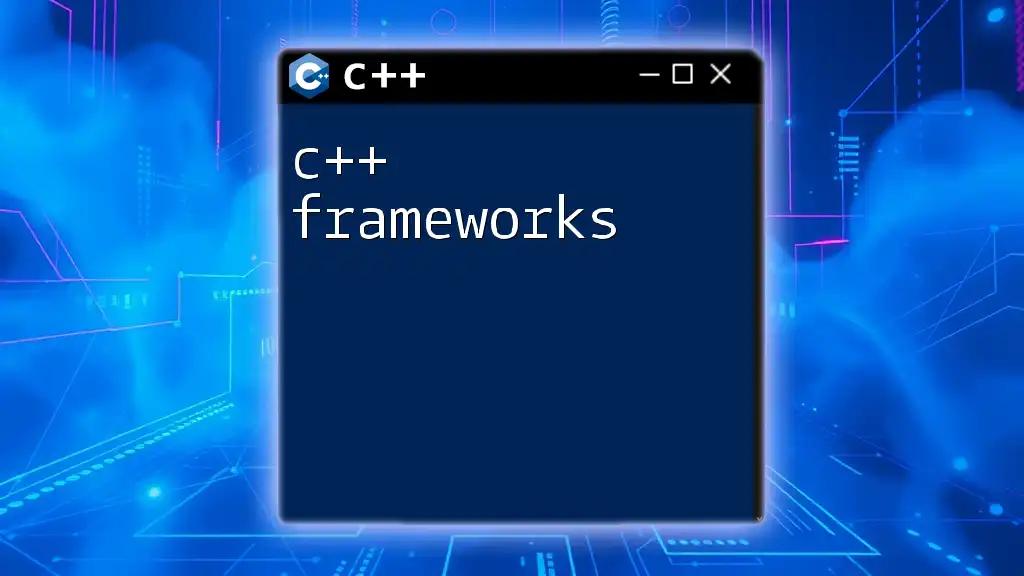
Conclusion
Mastering C++ fundamentals is foundational for becoming an effective programmer. Understanding the programming language's core structures—its types, control flow, functions, and object-oriented programming principles—will empower you to tackle increasingly advanced programming tasks.
The journey does not end here. While this guide provides a robust overview, continuing education in C++ through practice, real-world projects, and higher-order concepts will fortify your skills. Keep pushing the boundaries of what you can achieve with C++, and embrace the ever-evolving landscape of programming.