The `fopen_s` function is a safer alternative to `fopen` in C++, which helps prevent file access errors by using a secure method to open files.
Here’s a code snippet demonstrating how to use `fopen_s`:
#include <cstdio>
int main() {
FILE* file;
errno_t err = fopen_s(&file, "example.txt", "r");
if (err == 0) {
// File opened successfully
// Perform file operations here
fclose(file);
} else {
// Handle error
}
return 0;
}
What Is `fopen_s`?
`fopen_s` is a function introduced in the C11 standard to help manage file operations more safely. It facilitates the opening of files while reducing the risk of common errors associated with file handling. Unlike the traditional `fopen`, which returns a `NULL` pointer in case of failure, `fopen_s` returns an error code, allowing for better error management.
Comparison with `fopen`
While both `fopen` and `fopen_s` serve the same primary function—opening a file—there are some critical differences:
- Error Handling: `fopen` returns `NULL` if it fails, and the programmer must check the error using `errno`. In contrast, `fopen_s`'s error handling is more explicit, returning an `errno_t` type that indicates success or type of failure.
- Safety: The function mandates better programming practices, as it forces you to provide a pointer to a pointer, thereby reducing the chance of dangling pointers.

Syntax of `fopen_s`
The syntax for `fopen_s` is as follows:
errno_t fopen_s(FILE** stream, const char* filename, const char* mode);
Parameters Explained:
- `FILE** stream`: A pointer to a pointer, where the file handle will be stored upon successful opening of the file.
- `const char* filename`: A C-string representing the name of the file you wish to open.
- `const char* mode`: Specifies the file access mode (e.g., "r" for reading, "w" for writing, "a" for appending).
Return Value: The function returns an `errno_t` value:
- 0: Success
- Non-zero: An error code that indicates the type of failure.

How to Use `fopen_s`
Here's a simple example demonstrating how to use `fopen_s`:
#include <cstdio>
int main() {
FILE* file;
errno_t err = fopen_s(&file, "example.txt", "r");
if (err == 0) {
// File opened successfully
// You can now perform read/write operations on the file
fclose(file); // Always ensure to close the file
} else {
// Handle error (e.g., file not found)
}
return 0;
}
Error Handling with `fopen_s`
When using `fopen_s`, it’s essential to handle errors effectively:
- If `err` is 0, the file has opened successfully. Proceed with your file operations.
- If `err` is non-zero, perform error handling. You may print an error message or take corrective measures based on the nature of the error.
Example of basic error handling:
if (err != 0) {
printf("Error opening file. Error code: %d\n", err);
}

Common Use Cases for `fopen_s`
Reading from Files
To read data from a text file, you would typically use `fopen_s` with the "r" mode:
#include <cstdio>
int main() {
FILE* file;
errno_t err = fopen_s(&file, "data.txt", "r");
if (err == 0) {
char buffer[256];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
// Process buffer (e.g., print it)
printf("%s", buffer);
}
fclose(file);
} else {
printf("Error opening file. Error code: %d\n", err);
}
return 0;
}
Writing to Files
Similarly, if you want to write to a file, you can open it in "w" mode:
#include <cstdio>
int main() {
FILE* file;
errno_t err = fopen_s(&file, "output.txt", "w");
if (err == 0) {
fprintf(file, "Hello, World!\n");
fclose(file);
} else {
printf("Error opening file. Error code: %d\n", err);
}
return 0;
}
Appending to Files
To add content to an already existing file, use the "a" mode:
#include <cstdio>
int main() {
FILE* file;
errno_t err = fopen_s(&file, "append.txt", "a");
if (err == 0) {
fprintf(file, "Adding another line.\n");
fclose(file);
} else {
printf("Error opening file. Error code: %d\n", err);
}
return 0;
}
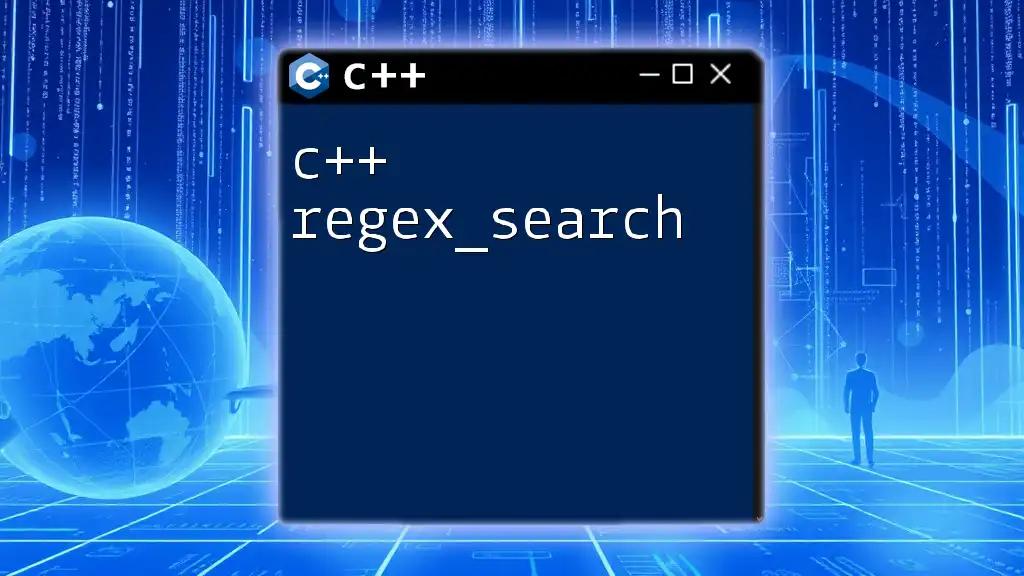
Best Practices for Using `fopen_s`
-
Always Check Return Values: Always check the return value of `fopen_s` to ensure that the file was successfully opened before proceeding with file operations. This safeguards against potential issues like segmentation faults and undefined behavior.
-
Properly Closing Files: Closing files is crucial in C++ programming. Neglecting to call `fclose()` will lead to memory leaks and data corruption.
-
Using Modes Effectively: Familiarize yourself with the different modes available for `fopen_s`. Whether you need to read, write, or append to a file, using the appropriate mode prevents logical errors in your program.
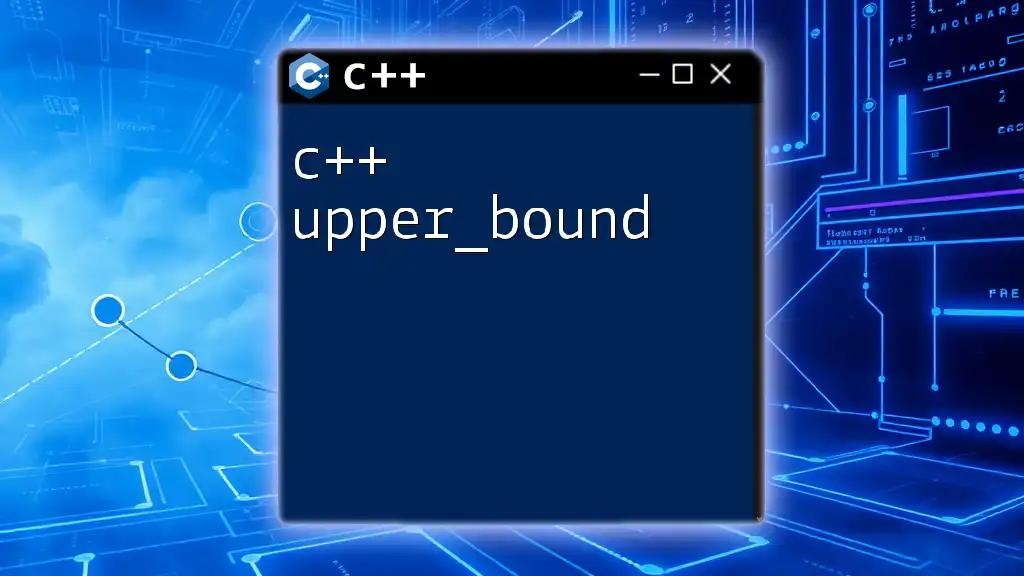
Common Mistakes When Using `fopen_s`
-
Pointers Mismanagement: One common issue is forgetting to use a pointer to a pointer. Ensure that the declaration of your `FILE* stream` variable is correctly handled.
-
Incorrect Modes: Using the wrong mode when opening a file can lead to unexpected behavior. For instance, attempting to read from a file while it's opened in "w" mode will result in an error.
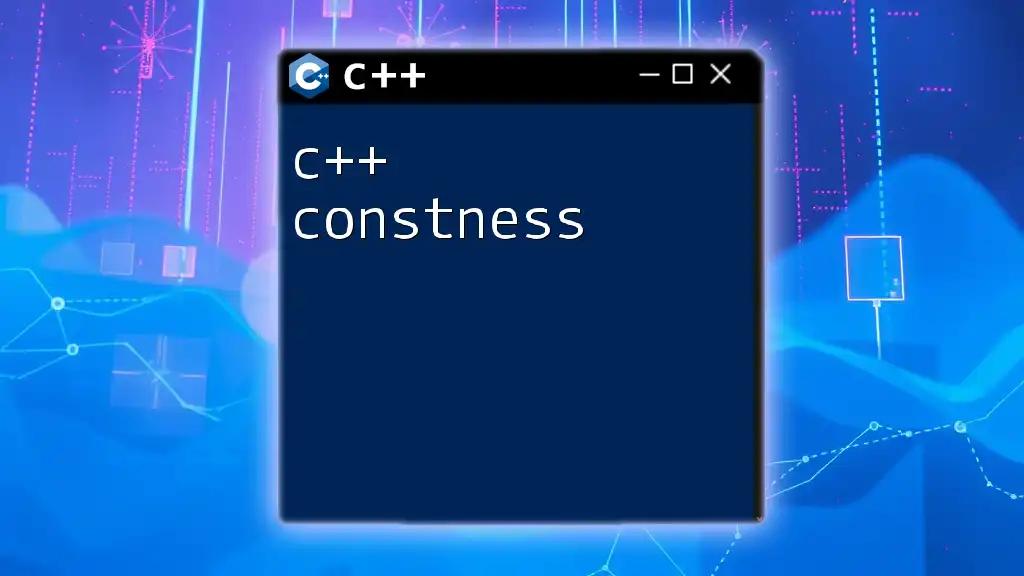
Conclusion
Using `fopen_s` in C++ for file handling provides an interface that helps prevent many common errors associated with file operations. Its improved error handling and safety features make it a superior choice compared to `fopen`. By following best practices, checking error codes, and practicing different file operations, you can maximize the benefits of this function. Embrace `fopen_s` in your file operations, and you'll develop a more robust and error-resistant codebase.
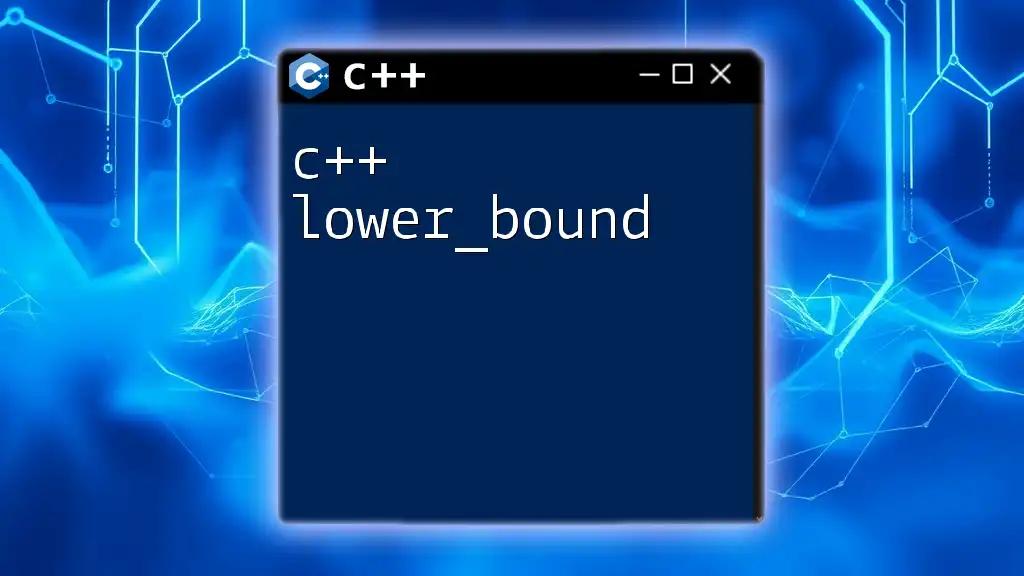
Additional Resources
For those seeking to delve deeper into file handling, consider exploring the official C++ documentation on `fopen_s` and similar functions. Numerous tutorials and videos are also available online for a comprehensive understanding of file operations in C++.
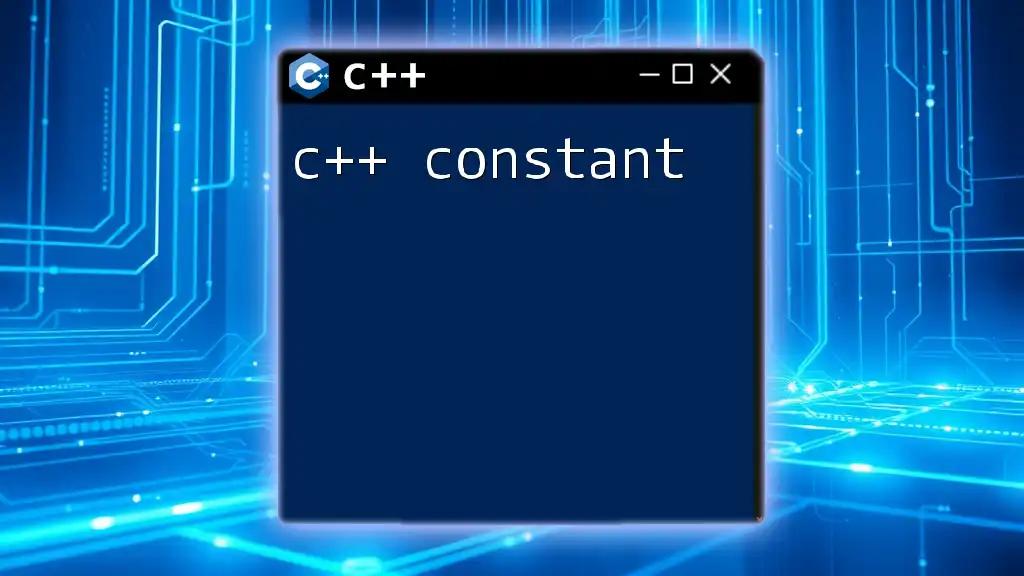
Call to Action
Share your experiences with `fopen_s` in the comments below, and don’t forget to follow our company for more concise and practical tips on mastering C++. Happy coding!